Can You Use a Function Inside a Function in Python? Exploring Nested Functions!
In the world of programming, the ability to harness the power of functions is one of the most fundamental skills a developer can possess. Functions not only help in organizing code but also enhance readability and reusability. Among the myriad of possibilities within Python, one intriguing concept stands out: the ability to use a function inside another function. This technique opens the door to a world of creative coding solutions, allowing developers to break down complex problems into manageable pieces. Whether you’re a novice eager to learn or an experienced coder looking to refine your skills, understanding how to nest functions can significantly elevate your programming prowess.
When you think about functions in Python, you might picture them as standalone entities that execute specific tasks. However, the beauty of Python lies in its flexibility, enabling you to define a function within another function. This nesting capability not only allows for cleaner code but also facilitates the creation of closures and decorators, which are powerful tools in any programmer’s toolkit. By leveraging this structure, you can encapsulate functionality, maintain scope, and even create more dynamic and responsive applications.
As we delve deeper into the intricacies of using functions inside functions, we will explore various scenarios where this technique shines. From enhancing modularity to improving code organization, the implications are vast and varied. Join
Using Functions Inside Functions in Python
In Python, you can indeed use a function inside another function. This concept is known as a nested function or inner function. When you define a function within another function, the inner function can access the variables and parameters of the outer function, creating a closure. This can be particularly useful for encapsulating functionality and managing scope.
Here’s how it works:
- The inner function can be used to perform operations that are only relevant within the context of the outer function.
- The inner function can access variables from the outer function, allowing for more flexible and modular code.
Below is an example to illustrate this concept:
“`python
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
“`
In this example, `inner_function` is defined within `outer_function`. The inner function takes one parameter, `y`, and adds it to `x`, which is defined in the outer function.
To use the nested function, you would call `outer_function` and then the returned inner function:
“`python
add_five = outer_function(5)
result = add_five(10) This will return 15
“`
Benefits of Using Nested Functions
Utilizing nested functions offers several advantages:
- Encapsulation: Keeps related functionality together, improving readability and maintainability.
- Access to Outer Scope: Allows the inner function to access variables from the outer function without passing them explicitly.
- Avoiding Global Scope: Reduces the risk of name collisions in the global namespace, as inner functions are not accessible from outside the outer function.
Limitations and Considerations
While nested functions can be very useful, there are some limitations and considerations to keep in mind:
- Scope: Inner functions are only accessible within the outer function. Once the outer function exits, the inner function is no longer available.
- Performance: Excessive use of nested functions can lead to performance issues, especially if they are called repeatedly in a loop.
- Complexity: Overusing nested functions can make code harder to read and understand.
Example Use Cases
Nested functions can be advantageous in various scenarios, including:
- Callbacks: When defining callbacks that depend on parameters from an outer function.
- Decorators: A common use case in Python for modifying the behavior of functions.
- Factory Functions: Creating functions tailored to specific parameters without exposing them globally.
Use Case | Description |
---|---|
Callbacks | Functions that are passed as arguments to other functions. |
Decorators | Functions that modify the behavior of another function. |
Factory Functions | Functions that return other functions customized to their parameters. |
In summary, using functions inside functions in Python allows for greater modularity and encapsulation, making your code cleaner and more manageable while offering powerful capabilities in various programming scenarios.
Using Functions Inside Functions in Python
In Python, it is not only permissible but also a common practice to define and use functions within other functions. This concept is known as “nested functions” or “inner functions.”
Defining Inner Functions
Inner functions can be defined within the body of outer functions. They can access variables from the enclosing scope, which allows for encapsulation and helps in organizing code logically. Here’s an example:
“`python
def outer_function(x):
def inner_function(y):
return y + 1
return inner_function(x) * 2
“`
In this example:
- `outer_function` takes a parameter `x`.
- `inner_function`, defined inside `outer_function`, takes a parameter `y`.
- The `inner_function` adds one to `y` and returns the result.
- Finally, `outer_function` returns the result of `inner_function` multiplied by 2.
Benefits of Using Inner Functions
Utilizing inner functions provides several advantages:
- Encapsulation: Inner functions help encapsulate functionality, preventing it from being accessed globally.
- Avoiding Namespace Pollution: By keeping functions nested, you limit their scope, reducing the chance of naming conflicts.
- Access to Enclosing Variables: Inner functions have access to variables defined in the outer function, making it easier to manage state.
Returning Inner Functions
Inner functions can be returned from their enclosing functions, allowing them to be used elsewhere. This is particularly useful in scenarios involving decorators or factory functions. Here’s an example:
“`python
def make_multiplier(factor):
def multiply(x):
return x * factor
return multiply
“`
In this instance:
- `make_multiplier` returns the `multiply` inner function, which can now utilize the `factor` variable from the outer function.
Example of Using Returned Inner Functions
“`python
double = make_multiplier(2)
result = double(5) This will return 10
“`
Here:
- `double` becomes a function that multiplies its input by 2.
- Calling `double(5)` yields 10.
Limitations and Considerations
While inner functions are powerful, there are some considerations to keep in mind:
- Readability: Excessive use of nested functions can lead to code that is difficult to read and maintain.
- Performance: Defining functions inside loops may lead to performance issues due to the repeated creation of the inner function.
Practical Use Cases for Inner Functions
Inner functions are particularly useful in:
- Closures: They can close over variables from the outer function, preserving their state.
- Decorators: Inner functions are used extensively in decorators to modify or enhance the behavior of functions.
- Callback Functions: Nested functions can serve as callbacks, maintaining access to variables from the outer scope.
Using functions inside functions in Python enhances code organization and encapsulation. The ability to return inner functions allows for dynamic behavior and state retention, making this feature a valuable aspect of Python programming.
Understanding Nested Functions in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Using a function inside another function, commonly referred to as a nested function, is not only permissible in Python but also a powerful feature. It allows for better organization of code and encapsulation of functionality, making it easier to manage complex logic.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Nested functions in Python can access variables from their enclosing scope, which is a key aspect of closures. This capability is particularly useful for creating decorators or when you need to maintain state without using global variables.”
Sarah Patel (Python Instructor, LearnPythonFast Academy). “While nesting functions can enhance readability and modularity, it is essential to use them judiciously. Overusing nested functions can lead to code that is difficult to debug and maintain, so balance is crucial.”
Frequently Asked Questions (FAQs)
Can you use a function inside a function in Python?
Yes, you can define a function inside another function in Python. This is known as a nested function or inner function.
What is the purpose of using a function inside another function?
Using a function inside another function allows for better organization of code, encapsulation of logic, and can help avoid variable scope issues by keeping variables local to the inner function.
Are there any limitations when using nested functions in Python?
There are no strict limitations, but inner functions can only be called within the scope of the outer function. They cannot be accessed from outside the outer function.
Can inner functions access variables from the outer function?
Yes, inner functions can access variables from their enclosing outer function. This is known as a closure, which allows the inner function to remember the environment in which it was created.
How do decorators utilize functions inside functions in Python?
Decorators in Python are a common use case for nested functions. A decorator is a function that takes another function as an argument, allowing you to modify or enhance the behavior of that function.
Can you return a function from another function in Python?
Yes, you can return a nested function from an outer function. This allows the returned function to maintain access to the outer function’s scope, enabling powerful programming patterns.
In Python, it is entirely permissible to use a function inside another function, a practice commonly referred to as nested functions or inner functions. This feature allows for greater modularity and encapsulation of code, enabling developers to create more organized and manageable code structures. Inner functions can access variables from their enclosing function’s scope, which facilitates the creation of closures, where the inner function retains access to the outer function’s variables even after the outer function has completed execution.
Using functions within functions can enhance code readability and maintainability. It allows for the grouping of related functionality, which can be particularly beneficial in complex programs. Furthermore, this approach can help avoid polluting the global namespace with helper functions that are only relevant within a specific context. By keeping these functions nested, developers can ensure that they are only accessible where they are needed, thus promoting cleaner code practices.
Additionally, nested functions can improve performance in certain scenarios, as they can be defined and utilized only when necessary. This can lead to more efficient memory usage, especially when the inner function is computationally intensive and only required under specific conditions. Overall, the ability to use functions within functions in Python provides a powerful tool for developers, encouraging better design patterns and fostering a deeper understanding of scope
Author Profile
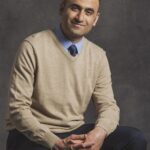
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?