Can You Really Use Python to Build a Website?
In the ever-evolving landscape of web development, the tools and languages available to creators have expanded dramatically, leading to exciting possibilities for building dynamic and interactive websites. Among these tools, Python stands out as a versatile and powerful programming language that has captured the attention of developers and entrepreneurs alike. But can you really use Python to make a website? The answer is a resounding yes! In this article, we’ll explore the various ways Python can be harnessed to create stunning web applications, from simple personal blogs to complex e-commerce platforms.
Python’s simplicity and readability make it an ideal choice for both beginners and seasoned developers. With a plethora of frameworks and libraries at its disposal, such as Django and Flask, Python streamlines the web development process, allowing developers to focus more on creativity and functionality rather than getting bogged down by intricate coding details. Whether you’re looking to build a robust backend or a user-friendly frontend, Python offers the flexibility to cater to diverse project requirements.
Moreover, the thriving community surrounding Python ensures that developers have access to a wealth of resources, tutorials, and support. This collaborative environment fosters innovation and encourages experimentation, making it easier than ever to bring your web development ideas to life. As we delve deeper into the capabilities of Python for web development, you’ll discover
Web Frameworks for Python
Python provides a variety of web frameworks that simplify the process of building websites. These frameworks come with built-in features that handle many of the repetitive tasks associated with web development, allowing developers to focus on creating unique functionalities. Some of the most popular frameworks include:
- Django: A high-level framework that emphasizes rapid development and clean, pragmatic design. It includes an ORM, authentication, and a robust admin interface out of the box.
- Flask: A lightweight and flexible micro-framework that is easy to use for small applications. Flask is highly extensible and allows developers to choose their components.
- FastAPI: Known for its high performance and ease of use, FastAPI is particularly well-suited for building APIs, leveraging Python type hints for better code quality.
- Pyramid: A versatile framework that can be used for both small and large applications, offering a lot of flexibility in terms of components.
Each framework has its own strengths and ideal use cases, allowing developers to select one based on their project requirements.
Building a Basic Web Application with Flask
To illustrate how to create a simple web application using Python, here’s a quick example using Flask.
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
In this example, a basic web server is set up, which responds with “Hello, World!” when accessed at the root URL. This minimal setup demonstrates Flask’s simplicity and effectiveness.
Frontend Integration with Python
While Python excels in backend development, it is essential to integrate it with frontend technologies to create a complete web experience. The following technologies are often used alongside Python:
- HTML/CSS: For structuring and styling web pages.
- JavaScript: To add interactivity and dynamic features.
- AJAX: For asynchronous requests to the server, improving user experience.
Python frameworks typically render HTML templates. Here’s a simple example using Flask with Jinja2 template rendering:
“`python
from flask import render_template
@app.route(‘/greet/
def greet(name):
return render_template(‘greet.html’, name=name)
“`
The corresponding HTML template (`greet.html`) might look like this:
“`html
Hello, {{ name }}!
“`
Deployment of Python Web Applications
Deploying a Python web application involves several steps to ensure that it runs smoothly in a production environment. Common deployment options include:
- Heroku: A cloud platform that supports various programming languages, including Python. It allows for easy deployment with Git.
- AWS Elastic Beanstalk: A service for deploying applications which automatically handles the infrastructure.
- Docker: Containerization allows for consistent environments across development and production.
The general deployment process can be summarized in the following steps:
- Prepare your application for deployment.
- Choose a hosting platform.
- Configure the server environment (e.g., web server, database).
- Deploy the application.
- Monitor and maintain the application post-deployment.
Platform | Pros | Cons |
---|---|---|
Heroku | Easy setup, free tier available | Limited control, scaling can get expensive |
AWS Elastic Beanstalk | Highly scalable, flexible configurations | Complex setup, steep learning curve |
Docker | Consistent environments, portable applications | Requires knowledge of containerization |
Understanding these frameworks, integration techniques, and deployment methods empowers developers to effectively use Python for web development.
Web Frameworks for Python
Python offers several frameworks that simplify the process of web development, enabling developers to create robust web applications efficiently. Some of the most popular frameworks include:
- Django: A high-level framework that promotes rapid development and clean, pragmatic design.
- Features: Built-in admin panel, ORM, and security features.
- Flask: A micro-framework that provides the essentials for web development, allowing for flexibility and scalability.
- Features: Lightweight, modular, and easy to extend with plugins.
- FastAPI: A modern framework for building APIs, designed for speed and performance.
- Features: Asynchronous support, automatic generation of OpenAPI documentation.
Frontend Development with Python
While Python is predominantly used for backend development, there are libraries and frameworks that allow for frontend development as well. Notable options include:
- PyScript: A framework that enables users to create rich web applications using Python directly in the browser.
- Anvil: A platform for building web apps with a drag-and-drop interface that uses Python for backend logic.
These tools bridge the gap between Python and frontend technologies, allowing developers to use a single programming language across the stack.
Database Integration
Integrating databases with Python web applications is straightforward, with several libraries available to facilitate this process. Common choices include:
Library | Description |
---|---|
SQLAlchemy | An ORM that provides a set of high-level API for database interaction. |
Django ORM | Integrated with Django, it abstracts database queries into Python objects. |
Peewee | A small, expressive ORM that is easy to use and supports multiple databases. |
These libraries support various databases, including SQLite, PostgreSQL, and MySQL, making it easier to manage data storage and retrieval.
Deployment Options
Once a Python web application is developed, deployment is the next crucial step. There are several options to consider:
- Platform-as-a-Service (PaaS): Services like Heroku, PythonAnywhere, and Google App Engine allow for easy deployment with minimal configuration.
- Virtual Private Servers (VPS): Providers such as DigitalOcean or AWS EC2 give more control over the server environment.
- Containerization: Docker can be used to create containers for applications, ensuring consistency across development and production environments.
APIs and Microservices
Python is well-suited for building APIs and microservices, allowing developers to create modular applications. Key considerations include:
- RESTful APIs: Using frameworks like Flask or FastAPI to build APIs that adhere to REST principles.
- GraphQL: Using libraries such as Graphene to implement GraphQL APIs, providing flexible query capabilities.
- Microservice architecture: Utilizing Docker and Kubernetes for container orchestration and management of microservices.
These approaches enable scalability and facilitate integration with other systems and services.
Testing and Debugging
Testing is vital to ensure the reliability of web applications. Python provides various tools for testing and debugging:
- Unit Testing: The `unittest` module is built into Python, allowing for the creation of test cases.
- Integration Testing: Tools like `pytest` and `nose` can be used for more complex testing scenarios.
- Debugging Tools: Libraries such as `pdb` for debugging code in a command-line interface.
Incorporating these tools into the development workflow can significantly enhance code quality and reduce the likelihood of bugs.
Can Python Power Your Next Website? Expert Insights
Dr. Emily Carter (Senior Software Engineer, WebTech Innovations). “Python is an incredibly versatile language that can indeed be used to create dynamic websites. Frameworks like Django and Flask simplify the development process, allowing developers to focus on building robust and scalable web applications.”
Michael Chen (Web Development Instructor, Code Academy). “Using Python for web development is not only feasible but also highly effective. Its readability and extensive libraries make it an excellent choice for both beginners and seasoned developers looking to implement complex functionalities.”
Sarah Thompson (Lead Developer, Tech Solutions Group). “While Python may not be the traditional choice for front-end development, its back-end capabilities are unmatched. By leveraging frameworks such as Django, developers can create powerful APIs that serve data to front-end technologies seamlessly.”
Frequently Asked Questions (FAQs)
Can you use Python to make a website?
Yes, Python can be used to create websites through various web frameworks such as Django and Flask, which facilitate rapid development and clean design.
What are the advantages of using Python for web development?
Python offers simplicity and readability, a vast ecosystem of libraries, strong community support, and frameworks that enhance productivity and reduce development time.
Which Python frameworks are best for web development?
Django and Flask are the most popular frameworks. Django is suited for larger applications with built-in features, while Flask is ideal for smaller projects due to its lightweight nature.
Can Python handle front-end development?
Python is primarily a back-end language. For front-end development, HTML, CSS, and JavaScript are typically used, although tools like Brython allow Python code to run in the browser.
Is Python suitable for building RESTful APIs?
Yes, Python is well-suited for building RESTful APIs. Frameworks like Flask and Django REST Framework provide tools to create robust APIs efficiently.
What hosting options are available for Python web applications?
Popular hosting options include Heroku, PythonAnywhere, and AWS. These platforms support Python applications and provide various deployment options.
Python can indeed be used to create websites, leveraging its versatility and the plethora of frameworks and libraries available. Frameworks such as Django and Flask provide robust solutions for web development, allowing developers to build scalable and maintainable web applications efficiently. These frameworks simplify the process of handling backend logic, database interactions, and user authentication, making Python a popular choice among developers.
Moreover, Python’s ease of learning and readability make it an attractive option for both novice and experienced developers. Its extensive ecosystem, which includes libraries for data manipulation, web scraping, and machine learning, enables the integration of advanced functionalities into web applications. This capability allows developers to create dynamic, data-driven websites that can cater to various user needs.
Additionally, the growing community support and resources available for Python web development contribute to its appeal. Developers can easily find documentation, tutorials, and forums to assist them in overcoming challenges they may encounter during the development process. Overall, Python’s combination of simplicity, power, and community support positions it as a strong candidate for web development projects.
Author Profile
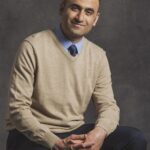
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?