Why Can’t This Element Be Used as a JSX Component?
In the ever-evolving landscape of web development, React has emerged as a powerhouse framework, enabling developers to build dynamic user interfaces with ease. However, as with any technology, it comes with its own set of challenges. One common issue that developers encounter is the perplexing error message: “cannot be used as a JSX component.” This seemingly cryptic warning can halt progress in its tracks, leaving both novice and seasoned developers scratching their heads. Understanding the nuances behind this error is crucial for anyone looking to harness the full potential of React and create seamless, interactive applications.
The “cannot be used as a JSX component” error often arises from a mismatch between the expected and actual types of components in your code. It can stem from various sources, including improper imports, incorrect component definitions, or even issues with TypeScript typings. As you dive deeper into the world of React, recognizing the common pitfalls that lead to this error can save you time and frustration, allowing you to focus on what you do best: building amazing user experiences.
In this article, we will explore the underlying causes of the “cannot be used as a JSX component” error and provide insights into how to troubleshoot and resolve it effectively. Whether you’re working on a small project or a large-scale application, understanding
Understanding JSX Component Errors
When working with JSX in React, developers may encounter the error message stating that a certain element “cannot be used as a JSX component.” This error typically arises due to various reasons related to how components are defined and used within the application. To troubleshoot and resolve these issues, it is crucial to understand the common causes.
Common Causes of JSX Component Errors
Several factors can lead to the aforementioned error. Here are some prevalent causes:
- Component Naming Conventions: React components must start with an uppercase letter. If a component is defined with a lowercase name, React treats it as a regular HTML element rather than a component.
- Incorrect Imports: If a component is not imported correctly, it may result in the error. Ensure that the component path is correct and that the component is exported properly.
- Functional vs. Class Components: If a component is defined as a class but is used as a functional component, or vice versa, it may lead to confusion and errors.
- Return Value Issues: Components must return valid JSX. If a component returns “ or a non-JSX value, it can cause this error.
- TypeScript and Props Validation: In TypeScript projects, using types incorrectly can lead to issues. Ensure that the component’s props align with the expected types.
Resolving JSX Component Errors
To effectively resolve these errors, consider the following steps:
- Check Component Naming: Ensure all components are named with an uppercase first letter.
- Verify Imports and Exports: Confirm that components are imported from the correct file and that they are exported correctly.
- Review Component Definitions: Double-check whether the component is defined as a functional or class component and ensure it is used accordingly.
- Inspect Return Statements: Make sure that the component returns valid JSX or `null`.
- Validate Props: In TypeScript, ensure the props passed to the component match the expected types.
Example of JSX Component Usage
Here’s an example of a correctly defined and used React component:
“`javascript
// Component definition
const MyComponent = (props) => {
return
;
};
// Usage
“`
In this example, `MyComponent` is defined as a functional component, and it is used correctly with an uppercase name and valid props.
Component Export and Import
Understanding how to export and import components is crucial for avoiding JSX component errors. Below is a table summarizing different methods of exporting components:
Export Type | Usage |
---|---|
Named Export | export const MyComponent = () => { … } |
Default Export | export default MyComponent; |
When importing, ensure you use the correct syntax based on the export type:
- For named exports: `import { MyComponent } from ‘./MyComponent’;`
- For default exports: `import MyComponent from ‘./MyComponent’;`
By following these guidelines, developers can effectively troubleshoot and resolve issues related to JSX component usage in React applications.
Understanding JSX Component Limitations
When working with JSX in React, developers may encounter the error message stating that a certain element “cannot be used as a JSX component.” This indicates that the component in question does not adhere to the expected structure or definition required for JSX rendering.
Common Causes of the Error
Several factors can lead to this issue:
- Incorrect Import Statements: Ensure that the component is properly imported from its respective file.
- Default vs. Named Exports: Verify whether the component is a default export or a named export. Usage inconsistencies can lead to this error.
- Invalid Component Definition: The component must be a function or class. If it’s a primitive value (like a string or number), JSX will not recognize it as a valid component.
- Missing Return Statement: Functional components must return valid JSX. A missing return can lead to rendering failures.
- Capitalization: Component names must start with an uppercase letter. JSX treats lowercase names as HTML tags.
Resolving the Error
To address the “cannot be used as a JSX component” error, consider the following steps:
- **Check Import Statements**:
- Ensure the component is correctly imported:
“`javascript
import MyComponent from ‘./MyComponent’; // Default export
// or
import { MyComponent } from ‘./MyComponent’; // Named export
“`
- **Review Component Definition**:
- Confirm that the component is defined as a function or class:
“`javascript
const MyComponent = () => {
return
;
};
“`
- Use Proper Capitalization:
- Ensure that the component name starts with an uppercase letter:
“`javascript
“`
- **Validate Return Statement**:
- Ensure the functional component returns JSX:
“`javascript
const MyComponent = () => {
return
; // Valid return
};
“`
- Check for Circular Dependencies:
- Circular dependencies can lead to components not being recognized. Refactor if necessary to avoid such situations.
Example Table of Common Issues and Fixes
Issue | Resolution |
---|---|
Component not imported | Ensure proper import statement is used. |
Incorrect export type | Match import style with export type (default vs named). |
Component defined incorrectly | Check that it is a function or class component. |
Lowercase component name | Rename the component to start with an uppercase letter. |
Missing return in functional component | Add a return statement that outputs valid JSX. |
Best Practices for Defining Components
To minimize the occurrence of this error, adhere to these best practices:
- Consistent Naming Conventions: Always use PascalCase for component names.
- Organized File Structure: Maintain a clear directory structure to simplify imports.
- Component Prop Validation: Utilize PropTypes or TypeScript for component prop validation to catch errors early.
- Documentation: Comment and document components to clarify their purpose and usage.
By following these guidelines, developers can avoid many common pitfalls associated with JSX components, ensuring smoother development and fewer runtime errors.
Understanding JSX Component Limitations
Jessica Lin (Senior Frontend Developer, CodeCraft Solutions). “The error message indicating that a certain element ‘cannot be used as a JSX component’ often arises from issues such as incorrect imports or the component not being defined properly. Ensuring that the component is exported correctly and that its name adheres to React’s naming conventions is crucial for successful integration.”
Michael Chen (React Specialist, DevInsights). “When a component cannot be used as a JSX component, it typically signifies that the component is either a plain JavaScript function or an object that does not return valid JSX. Developers should verify that the component returns a React element and is not mistakenly treated as a primitive value.”
Sarah Patel (Software Architect, Tech Innovations Inc.). “The inability to use a component as a JSX element may also stem from TypeScript type definitions. If the component is typed incorrectly or lacks the necessary props, TypeScript may prevent its use in JSX. Ensuring that type definitions align with the expected structure is essential for seamless integration.”
Frequently Asked Questions (FAQs)
What does it mean when a component cannot be used as a JSX component?
A component cannot be used as a JSX component when it does not meet the requirements set by React, such as being a valid function or class that returns JSX or when it is not properly defined or imported.
What are common reasons for a component not being recognized as a JSX component?
Common reasons include incorrect import statements, the component not being exported properly, or the component being defined as a non-callable entity, such as an object or a string.
How can I troubleshoot a component that cannot be used as a JSX component?
To troubleshoot, check the import paths, ensure the component is exported correctly, verify that it is a function or class, and confirm that it returns valid JSX.
Can functional components be used as JSX components?
Yes, functional components can be used as JSX components as long as they are correctly defined as functions that return JSX and are properly imported into the file.
Are there specific naming conventions that affect JSX component usage?
Yes, JSX components must start with an uppercase letter. If a component starts with a lowercase letter, React treats it as a DOM tag rather than a component, leading to the “cannot be used as a JSX component” error.
What should I do if I encounter a TypeScript error related to JSX components?
If you encounter a TypeScript error, ensure that your component types are correctly defined, check for any type mismatches, and verify that your TypeScript configuration supports JSX.
The phrase “cannot be used as a JSX component” typically arises in the context of React development when a developer attempts to render a value that is not a valid React component. This situation often occurs due to various reasons, such as attempting to use a primitive value, an variable, or a function that does not return a valid React element. Understanding the nature of JSX and how it interprets components is crucial for troubleshooting these issues effectively.
One of the key insights is the importance of ensuring that all components are defined correctly and adhere to the conventions of React. Components should either be class-based or functional components, and they must return a valid JSX structure. Additionally, developers should be cautious about the scope and context in which components are defined to avoid referencing issues that lead to the “cannot be used as a JSX component” error.
Another takeaway is the significance of proper imports and exports in React applications. If a component is not imported correctly or if it is exported as a default when it should be a named export, this can lead to confusion and errors during rendering. Therefore, maintaining a clear and organized structure for components can mitigate these issues and enhance overall code quality.
In summary, addressing the “cannot be used as
Author Profile
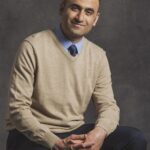
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?