Why Can’t I Consume a Scoped Service from a Singleton in My Application?
In the world of software development, particularly within the realm of dependency injection in .NET applications, developers often encounter a perplexing yet crucial challenge: the issue of service lifetimes. One common pitfall that many face is the error message stating that you “cannot consume scoped service from singleton.” This seemingly cryptic warning can lead to confusion and frustration, especially for those new to the intricacies of service lifetimes. Understanding this concept is essential for building robust, maintainable applications that leverage the power of dependency injection effectively.
At its core, the problem arises from the fundamental differences between service lifetimes—singleton, scoped, and transient. A singleton service is instantiated once and shared throughout the application’s lifetime, while a scoped service is created once per request within a specific scope, such as an HTTP request in a web application. This distinction is vital because it dictates how services interact with one another. When a singleton attempts to access a scoped service, it can lead to unintended consequences, including potential memory leaks or incorrect data states, as the singleton may outlive the scope of the scoped service.
Navigating the complexities of these service lifetimes is not just about avoiding errors; it’s about architecting your application in a way that promotes clarity and efficiency. By understanding the implications of consuming scoped services
Understanding Scoped Services
Scoped services in dependency injection are instantiated once per request within a specific scope. This means that every time a new request is initiated, a new instance of a scoped service is created. Scoped services are commonly used in web applications, where a new scope is created for each HTTP request.
Key characteristics of scoped services include:
- Instance Management: A new instance is created for each scope.
- Lifecycle: They are disposed of automatically when the scope ends.
- Use Case: Ideal for managing resources that are specific to a single request, such as database contexts.
Singleton Services Overview
Singleton services, on the other hand, are instantiated once and shared throughout the application’s lifetime. This means that there is only one instance of the singleton service, regardless of how many requests are made. Singleton services are useful for stateless services or shared resources that do not need to maintain state between requests.
Key characteristics of singleton services include:
- Instance Management: Only one instance exists for the entire application.
- Lifecycle: Remains in memory until the application is shut down.
- Use Case: Suitable for services that provide shared functionality, such as logging or configuration settings.
Problematic Interaction Between Scoped and Singleton Services
The error “cannot consume scoped service from singleton” arises when a singleton service tries to depend on a scoped service. This interaction is problematic due to the lifecycle differences between the two service types.
When a singleton service is created, it holds onto the instance of the scoped service, which may lead to several issues:
- Memory Leaks: If the singleton holds onto a scoped instance beyond its lifetime, it can lead to memory leaks.
- Inconsistent State: The singleton might end up using a disposed instance of the scoped service, leading to exceptions and inconsistent behavior.
Best Practices to Avoid This Issue
To prevent the “cannot consume scoped service from singleton” error, consider the following best practices:
- Use Factory Patterns: Implement a factory pattern that creates the scoped service instance on demand rather than holding onto it.
- Service Locator Pattern: Use a service locator to resolve the scoped service when needed, allowing for the correct instance to be provided without holding onto it.
- Refactor Service Lifetimes: If possible, refactor the singleton service to be scoped or transient if it requires a scoped service.
Service Type | Lifetime | Use Case |
---|---|---|
Scoped | Per request | Request-specific resources |
Singleton | Application lifetime | Shared functionality |
By adhering to these practices, developers can ensure that their applications maintain proper service lifetimes and avoid potential runtime issues related to service scope mismatches.
Understanding Scoped and Singleton Services
In the context of dependency injection in .NET, services can be registered with different lifetimes: singleton, scoped, and transient. Each lifetime defines how the service is instantiated and shared across components.
- Singleton: A service created once and shared throughout the application’s lifetime. It is ideal for stateless services or services that maintain global state.
- Scoped: A service created once per request (or per scope). This is suitable for services that should maintain state throughout a single operation but not beyond it.
- Transient: A service that is created each time it is requested. This is useful for lightweight, stateless services.
Why Scoped Services Cannot Be Consumed by Singletons
The fundamental reason why scoped services cannot be injected into singleton services is due to the lifecycle management of these services. When a singleton service is instantiated, it retains its instance for the application’s lifetime. In contrast, a scoped service is designed to be instantiated per request. Attempting to inject a scoped service into a singleton can lead to several issues:
- Lifetime Mismatch: The singleton may outlive the request that created the scoped service, leading to a situation where the singleton holds a reference to a disposed scoped service.
- State Management: Scoped services often hold state that is relevant only for the duration of the request. A singleton would not have access to this state across different requests, leading to unpredictable behavior.
- Thread Safety: Singleton services are often accessed by multiple threads simultaneously, while scoped services are intended to be used in a single thread context. This can lead to race conditions or data corruption.
Alternative Solutions
When faced with the need to use scoped services within singleton services, consider the following alternatives:
- Factory Pattern: Use a factory to create scoped services on demand. This allows the singleton to request a scoped service whenever it needs it without holding onto a reference.
“`csharp
public class MySingleton
{
private readonly IServiceScopeFactory _scopeFactory;
public MySingleton(IServiceScopeFactory scopeFactory)
{
_scopeFactory = scopeFactory;
}
public void UseScopedService()
{
using (var scope = _scopeFactory.CreateScope())
{
var scopedService = scope.ServiceProvider.GetRequiredService
// Use the scoped service here
}
}
}
“`
- Refactor Design: If feasible, consider redesigning the application architecture to avoid the need for a singleton to depend on a scoped service. This may involve moving shared logic out of the singleton or using events to communicate between services.
- Event Aggregator: Implement an event aggregator pattern that allows singletons to subscribe to events without directly depending on scoped services.
Best Practices for Managing Service Lifetimes
To effectively manage service lifetimes and avoid issues with dependency injection:
- Keep Lifetimes Aligned: Whenever possible, ensure that services with longer lifetimes do not depend on services with shorter lifetimes.
- Service Composition: Use composition to manage dependencies. Create a higher-level service that encapsulates both singleton and scoped services without direct dependency.
- Monitor Performance: Regularly review the application’s performance and service interactions to identify potential pitfalls or architectural concerns related to service lifetimes.
By adhering to these best practices, developers can maintain a clean and efficient dependency injection setup, ensuring that services operate within their intended scopes.
Understanding the Limitations of Scoped Services in Singleton Contexts
Dr. Emily Carter (Software Architecture Consultant, Tech Innovations Inc.). “The issue of consuming scoped services from a singleton arises from the fundamental lifecycle management in dependency injection. Singleton services are created once and live for the application’s lifetime, whereas scoped services are instantiated per request. Attempting to access a scoped service from a singleton can lead to unintended behavior and resource management problems, as the scoped instance may not exist in the singleton’s context.”
James Liu (Lead Software Engineer, Cloud Solutions Corp.). “In my experience, the inability to consume scoped services from singletons is a design decision that enforces separation of concerns. It ensures that singletons do not inadvertently hold onto transient or request-specific data, which could lead to memory leaks or stale data issues. Developers should consider redesigning their architecture to avoid such dependencies, possibly by injecting factories or using other patterns.”
Sarah Thompson (Principal Developer Advocate, Modern Dev Practices). “This limitation is crucial for maintaining application stability and scalability. Scoped services are intended to be short-lived and tied to a specific context, while singletons are long-lived and global. By preventing scoped services from being accessed in singletons, we encourage developers to think critically about their service lifetimes and the implications of their design choices, ultimately leading to cleaner and more maintainable code.”
Frequently Asked Questions (FAQs)
What does “cannot consume scoped service from singleton” mean?
This error indicates that a service with a scoped lifetime is being accessed from a singleton service. In dependency injection, scoped services are created per request, while singleton services are created once and reused throughout the application’s lifetime.
Why is it a problem to consume a scoped service in a singleton?
Consuming a scoped service within a singleton can lead to unintended behavior and resource management issues. The singleton may hold onto the scoped service beyond its intended lifespan, leading to potential memory leaks or stale data.
How can I resolve the “cannot consume scoped service from singleton” error?
To resolve this error, consider changing the lifetime of the service that requires the scoped service to also be scoped or transient. Alternatively, you can use dependency injection to pass the scoped service to methods or properties when needed instead of holding a reference to it.
What are the lifetime options available in dependency injection?
The primary lifetime options in dependency injection are singleton (one instance for the application’s lifetime), scoped (one instance per request), and transient (a new instance every time it is requested).
Can I use a factory pattern to access scoped services in a singleton?
Yes, you can implement a factory pattern to create instances of the scoped service within the singleton. This approach allows you to resolve the scoped service as needed without holding onto it, thus avoiding the error.
What should I consider when designing services with different lifetimes?
When designing services, consider the relationships between them, their lifetimes, and how they will be used. Ensure that services with shorter lifetimes are not referenced by those with longer lifetimes to maintain proper resource management and avoid runtime errors.
The issue of “cannot consume scoped service from singleton” arises from the fundamental principles of dependency injection in software development, particularly within the context of frameworks like ASP.NET Core. In these frameworks, services can be registered with different lifetimes: singleton, scoped, and transient. A singleton service is created once and shared throughout the application’s lifetime, while a scoped service is created once per request within a specific scope. This distinction is critical because it affects how dependencies are resolved and managed during the application’s execution.
Attempting to inject a scoped service into a singleton service leads to potential issues, primarily because the singleton service would retain a reference to the scoped service beyond its intended lifespan. When the scope ends, the scoped service may be disposed of, leaving the singleton service with a stale reference. This can result in runtime exceptions and unpredictable behavior, making it essential for developers to understand the implications of service lifetimes and their interactions.
To avoid this problem, it is advisable to rethink the design of the application. Developers can use factory patterns or service locators to resolve scoped services when needed, ensuring that the scoped service is created within the appropriate scope. Additionally, restructuring the application’s architecture to align with the intended lifetimes of services can lead to a more robust
Author Profile
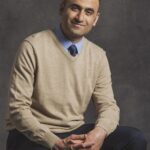
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?