Why Do I Get the Error ‘Cannot Convert Undefined or Null to Object’ and How Can I Fix It?
In the ever-evolving landscape of web development, encountering errors is an inevitable part of the journey. One particularly perplexing error that many developers face is the cryptic message: “cannot convert or null to object.” This seemingly innocuous phrase can halt your progress and leave you scratching your head, especially when it appears unexpectedly in your code. Understanding the roots of this error is essential for troubleshooting and refining your programming skills, ensuring that your applications run smoothly and efficiently.
At its core, this error arises when JavaScript attempts to perform operations on a value that is either “ or `null`, both of which signify the absence of a value. This can occur in various scenarios, such as when trying to access properties of an object that hasn’t been properly initialized or when passing incorrect parameters to functions. As developers, recognizing the conditions that lead to this error can empower us to create more robust and error-resistant code.
In this article, we will delve into the common causes of the “cannot convert or null to object” error, explore practical strategies for identifying and resolving it, and share best practices to prevent it from occurring in the first place. Whether you’re a seasoned developer or just starting your coding journey, understanding this error will enhance your problem-solving toolkit and
Understanding the Error Message
The error message “cannot convert or null to object” typically arises in JavaScript when a method or function attempts to operate on an or null value, treating it as an object. This often occurs with functions like `Object.keys()`, `Object.values()`, or when using spread syntax.
Common Causes
- Null or Variables: Attempting to access properties or methods on variables that are either null or .
- Incorrect Function Arguments: Passing an incorrect argument to functions that expect an object.
- Asynchronous Operations: Timing issues in asynchronous code can lead to situations where variables are not yet defined when accessed.
How to Diagnose the Issue
To effectively diagnose the cause of this error, consider the following steps:
- Check Variable Initialization: Ensure that all variables are initialized before being passed to functions or methods.
- Use Console Logging: Implement console logs to track the state of variables leading up to the error.
- Debugging Tools: Utilize debugging tools available in browsers (like Chrome DevTools) to inspect variables at runtime.
Example Code Snippet
“`javascript
let user = null;
console.log(Object.keys(user)); // This will throw an error
“`
Preventing the Error
To avoid encountering this error, follow best practices for coding:
– **Use Default Values**: When destructuring or assigning values, use default parameters to prevent values.
“`javascript
const getUserInfo = (user = {}) => {
console.log(user.name); // This will not throw an error
};
“`
- Type Checking: Implement checks to ensure that the variables are not null or before attempting to use them.
“`javascript
if (user) {
console.log(Object.keys(user));
}
“`
Handling the Error Gracefully
In situations where the error may still occur, implement error handling to manage the situation without crashing the application.
Example of Error Handling
“`javascript
try {
console.log(Object.keys(user));
} catch (error) {
console.error(“An error occurred:”, error.message);
}
“`
Table of Methods and Their Behavior
Method | Behavior with null/ |
---|---|
Object.keys() | Throws an error |
Object.values() | Throws an error |
Object.entries() | Throws an error |
Object.assign() | Ignores null/ |
By understanding the context in which this error arises, you can implement effective strategies to prevent it, leading to more robust and resilient JavaScript code.
Understanding the Error Message
The error message “cannot convert or null to object” typically arises in JavaScript when attempting to perform an operation on a value that is either “ or `null`. This situation often occurs in scenarios where an object is expected, but the actual value is not valid.
Common Scenarios Leading to the Error
- Object Methods: Using methods that require an object as an argument, such as `Object.keys()`, `Object.values()`, or `Object.entries()`.
- Destructuring: Attempting to destructure properties from an object that is “ or `null`.
- Function Parameters: Passing “ or `null` as an argument to functions that expect an object.
Identifying the Source of the Error
To troubleshoot this error effectively, follow these steps:
- Check Variable Initialization: Ensure that variables expected to be objects are properly initialized.
- Debugging: Use debugging tools or `console.log()` statements to inspect the values before they are used.
- Conditional Checks: Implement checks to verify that variables are not “ or `null`.
Example Code Snippet
Here’s an example that demonstrates the issue:
“`javascript
let obj = null;
console.log(Object.keys(obj)); // Throws error: cannot convert or null to object
“`
To fix this, you can add a condition:
“`javascript
if (obj) {
console.log(Object.keys(obj));
} else {
console.log(“Object is null or “);
}
“`
Best Practices to Avoid the Error
To prevent encountering the “cannot convert or null to object” error, consider the following best practices:
- Default Parameters: Use default parameters in functions to ensure an object is provided.
“`javascript
function processObject(data = {}) {
console.log(Object.keys(data));
}
“`
- Optional Chaining: Utilize optional chaining (`?.`) to safely access properties.
“`javascript
let user = null;
console.log(user?.name); // Returns without throwing an error
“`
- Type Checking: Implement type checks to verify data types before processing.
“`javascript
function isObject(value) {
return value !== null && typeof value === ‘object’;
}
if (isObject(obj)) {
console.log(Object.keys(obj));
}
“`
Handling Asynchronous Data
In scenarios involving asynchronous data fetching, such as API calls, it’s crucial to handle cases where the expected data might not yet be available:
- Promises and Async/Await: Ensure that data is available before attempting to access it.
“`javascript
async function fetchData() {
const response = await fetch(‘api/data’);
const data = await response.json();
if (isObject(data)) {
console.log(Object.keys(data));
}
}
“`
- Fallback Values: Use fallback values when the data is not available.
“`javascript
let responseData = await fetchData() || {};
console.log(Object.keys(responseData));
“`
Addressing the “cannot convert or null to object” error requires careful programming practices, including proper initialization, type checking, and handling asynchronous data effectively. By adhering to these guidelines, developers can enhance code robustness and reduce the occurrence of such errors.
Understanding the Error: “Cannot Convert or Null to Object”
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘cannot convert or null to object’ typically arises when developers attempt to manipulate data that has not been properly initialized. It is crucial to ensure that variables are defined before they are used in operations that require an object, such as Object.keys() or Object.assign().”
Michael Chen (JavaScript Developer Advocate, CodeCraft Academy). “This error serves as a reminder of the importance of type checking in JavaScript. Utilizing tools like TypeScript or employing runtime checks can help prevent such issues by enforcing stricter type rules, thereby reducing the likelihood of encountering or null values in object manipulations.”
Lisa Patel (Lead Frontend Developer, Web Solutions Group). “To effectively handle this error, developers should implement defensive programming techniques. This includes using default parameters, optional chaining, and nullish coalescing to safeguard against or null values, ensuring that the application remains robust and user-friendly.”
Frequently Asked Questions (FAQs)
What does the error “cannot convert or null to object” mean?
This error indicates that a piece of code is attempting to convert a value that is either “ or `null` into an object, which is not permissible in JavaScript.
What causes the “cannot convert or null to object” error?
The error typically arises when a function or method expects an object as an argument but receives “ or `null` instead, often due to missing or improperly initialized variables.
How can I troubleshoot this error in my code?
To troubleshoot, check the variables being passed to functions or methods, ensuring they are properly initialized and not “ or `null`. Use console logging to inspect values before they are processed.
Are there specific functions that commonly trigger this error?
Yes, functions such as `Object.keys()`, `Object.assign()`, and `JSON.stringify()` can trigger this error if they receive “ or `null` as their argument.
What are some best practices to avoid this error?
Implement checks to validate variables before usage, such as using conditional statements or default values. Additionally, consider using TypeScript for type safety, which can help catch such issues during development.
Can this error occur in asynchronous code?
Yes, it can occur in asynchronous code if a variable is expected to be set by a promise or callback but is still “ or `null` at the time of access. Proper error handling and checks are essential in such cases.
The error message “cannot convert or null to object” typically arises in JavaScript when attempting to use a method that expects an object, but instead receives either “ or `null`. This often occurs in scenarios involving object manipulation, such as when using functions like `Object.keys()`, `Object.assign()`, or destructuring assignments. Understanding the context in which this error appears is crucial for effective debugging and code maintenance.
One of the primary causes of this error is the failure to initialize variables properly. Developers must ensure that variables are assigned valid object values before invoking methods that manipulate or read properties from them. Additionally, careful validation of input data and the use of default parameters can help mitigate this issue. Employing techniques such as optional chaining or nullish coalescing can also provide more robust solutions to handle potentially or null values gracefully.
addressing the “cannot convert or null to object” error requires a proactive approach to variable management and input validation. By implementing best practices in coding, developers can significantly reduce the occurrence of this error. Furthermore, understanding the underlying principles of JavaScript objects and their manipulation will enhance overall code quality and reliability.
Author Profile
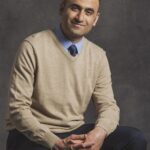
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?