Why Can’t I Create a File When It Already Exists?
In the digital age, where data management and file organization are paramount, encountering the error message “cannot create a file when that file already exists” can be a frustrating experience. This seemingly simple notification can halt your productivity and leave you grappling with the intricacies of file systems and software behavior. Whether you’re a seasoned developer, a casual user, or someone navigating the complexities of digital storage, understanding the nuances behind this error is essential for seamless file management.
At its core, this error message arises when an attempt is made to create a new file with a name that already exists in the specified directory. This situation can occur in various contexts, from programming environments to everyday applications like word processors and spreadsheets. The implications of this error extend beyond mere inconvenience; they can disrupt workflows, hinder data organization, and complicate collaborative efforts.
Understanding the underlying causes and potential solutions to this issue is vital for anyone who regularly interacts with digital files. By exploring the mechanics of file creation, naming conventions, and the role of operating systems, readers will gain valuable insights into how to effectively navigate and resolve this common hurdle. With the right knowledge, you can transform this frustrating encounter into an opportunity for improved file management practices.
Understanding File Creation Errors
When attempting to create a file that already exists in a specified location, the system generates a file creation error. This error typically arises in various operating systems and programming environments. Understanding the underlying mechanisms can help prevent and resolve these issues effectively.
Common reasons for this error include:
- File Existence: The file you are trying to create already exists in the specified directory.
- Permissions Issues: Lack of sufficient permissions to overwrite or modify existing files.
- File Locking: The existing file may be locked by another process, preventing modifications or deletions.
Handling File Creation Errors
To mitigate the error of creating a file that already exists, consider employing the following strategies:
- Check for Existence: Before creating a new file, check if it already exists. This can be done programmatically using file system functions.
- Use Unique Naming Conventions: When creating files, use unique identifiers (such as timestamps or GUIDs) to avoid name collisions.
- Prompt for Overwrite: If your application allows it, prompt the user for permission to overwrite an existing file.
Here is an example of a simple pseudocode approach to handle file creation:
“`pseudocode
if fileExists(“example.txt”):
promptUser(“File exists. Do you want to overwrite? (yes/no)”)
if userResponse == “yes”:
createFile(“example.txt”)
else:
createFile(“example.txt”)
“`
Best Practices for File Management
Implementing best practices can significantly reduce the likelihood of encountering file creation errors. Here are some key points to consider:
- Organize Files in Directories: Create a logical directory structure to manage files efficiently.
- Regular Backups: Maintain backups of important files to prevent loss during overwrites or deletions.
- Error Handling: Implement robust error handling in your code to gracefully manage file creation issues.
Error Handling Table
Error Type | Possible Cause | Resolution |
---|---|---|
FileExistsError | File with the same name exists | Check for existence and prompt user |
PermissionError | Insufficient permissions | Change permissions or run with elevated rights |
FileLockedError | File is in use by another process | Close the application using the file |
By applying these practices and understanding the common causes of file creation errors, you can enhance the reliability and efficiency of file management in your applications.
Understanding the Error Message
The phrase “cannot create a file when that file already exists” typically indicates that an attempt is being made to create a file in a location where a file with the same name already exists. This issue can arise in various contexts, including programming, file management systems, and software applications.
Key points to consider include:
- File System Behavior: Most file systems enforce unique filenames within the same directory. Attempting to create a file with a name that already exists will trigger an error.
- Overwrite Permissions: Depending on the application or operating system settings, users may or may not have permissions to overwrite existing files.
- Error Handling: Understanding how to handle such errors programmatically can lead to more robust applications.
Troubleshooting Steps
When faced with this error, several troubleshooting steps can be taken to resolve the issue:
- Check File Existence:
- Verify if the file already exists in the intended directory.
- Use file management tools or commands (like `ls` in Unix/Linux or `dir` in Windows) to list files.
- Rename the File:
- If creating a new file, consider renaming the new file to avoid conflicts.
- Use a naming convention that ensures uniqueness (e.g., appending timestamps).
- Delete or Move the Existing File:
- If the existing file is no longer needed, delete it or move it to a different location to free up the filename.
- Check Permissions:
- Ensure that your user account has the necessary permissions to overwrite existing files in the target directory.
- Use Version Control:
- Implement version control systems to manage file changes and avoid conflicts.
Programming Contexts
In programming, this error can manifest in various languages and frameworks. Here are some common scenarios:
Language/Framework | Common Cause | Resolution |
---|---|---|
Python | Using `open(‘filename.txt’, ‘x’)` where the file exists | Switch to `open(‘filename.txt’, ‘w’)` to overwrite |
Java | Using `File.createNewFile()` when the file exists | Check if the file exists before creation |
JavaScript | Using `fs.writeFileSync(‘filename.txt’, data)` | Use `fs.appendFileSync` to add data instead |
Best Practices for File Management
To minimize the occurrence of this error in file management and development, consider the following best practices:
- Implement File Checks: Always check if a file exists before attempting to create or write to it.
- Use Unique Identifiers: Generate unique identifiers or timestamps as part of the filename to prevent conflicts.
- Provide User Feedback: In applications, provide clear messages to users when a file cannot be created due to existing files.
- Graceful Handling: Implement error handling in your code to manage exceptions related to file creation gracefully.
Addressing the error “cannot create a file when that file already exists” involves understanding the context, employing troubleshooting steps, and adhering to best practices in file management. By following these guidelines, users can prevent future occurrences of this issue and streamline their workflows.
Understanding File Creation Errors in Software Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘cannot create a file when that file already exists’ typically indicates that the system is attempting to write a file to a location where a file with the same name already exists. Developers should implement checks to verify file existence before attempting to create new files to avoid this issue.”
Michael Chen (IT Support Specialist, Global Tech Solutions). “In many cases, this error arises from permission issues or file locks. Users should ensure they have the necessary permissions to overwrite existing files and check if any applications are currently using the file, which may prevent its modification.”
Sarah Thompson (Data Management Consultant, InfoSecure Corp.). “When dealing with file creation errors, it is essential to implement robust error handling in your code. This not only helps in diagnosing the issue but also provides users with clearer feedback on what went wrong, allowing for a smoother user experience.”
Frequently Asked Questions (FAQs)
What does it mean when a system says “cannot create a file when that file already exists”?
This message indicates that the file you are attempting to create has the same name and location as an existing file. The system prevents overwriting to avoid data loss.
How can I resolve the “cannot create a file when that file already exists” error?
To resolve this error, you can either rename the new file, delete or move the existing file, or choose to overwrite the existing file if that is acceptable.
Is there a way to force the creation of a file that already exists?
Yes, you can force the creation of a file by using specific programming functions or commands that allow overwriting. However, this may result in the loss of the existing file’s data.
What are the potential risks of overwriting an existing file?
Overwriting an existing file can lead to permanent data loss, as the original content will be replaced. Always ensure you have backups before proceeding with overwriting.
Can this error occur in cloud storage services?
Yes, cloud storage services can also display this error if you attempt to upload a file with the same name and location as an existing file. The resolution methods are similar to those on local systems.
Are there any programming languages that handle file creation differently?
Yes, different programming languages have varying methods for file handling. For instance, some languages allow for options to append or overwrite files, while others may require explicit commands to avoid this error.
In the realm of file management and programming, the issue of being unable to create a file that already exists is a common challenge. This situation often arises due to the inherent properties of file systems, which prevent overwriting existing files unless explicitly permitted. Different programming languages and systems have various methods to handle this scenario, including error handling mechanisms, file mode specifications, and user prompts for file replacement. Understanding these nuances is crucial for developers and users alike to navigate file creation effectively.
Moreover, the implications of this limitation extend beyond mere technicalities. It emphasizes the importance of implementing robust file management practices, such as checking for file existence before attempting to create a new file. This proactive approach not only prevents errors but also enhances the overall user experience by minimizing disruptions. Additionally, leveraging features like version control can help manage file iterations without conflict.
the inability to create a file when it already exists serves as a reminder of the complexities involved in file handling. By adopting best practices and utilizing the available tools and techniques, users can mitigate potential issues and streamline their workflows. Ultimately, a thorough understanding of file management principles is essential for effective data handling and programming success.
Author Profile
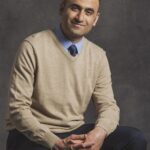
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?