Why Am I Getting the Error ‘Cannot Find Module or Its Corresponding Type Declarations’?
In the ever-evolving landscape of software development, encountering errors can often feel like navigating a maze. One common roadblock that many developers face is the dreaded message: “cannot find module or its corresponding type declarations.” This seemingly cryptic error can halt progress and lead to frustration, especially for those who are new to TypeScript or working with complex JavaScript projects. Understanding the roots of this issue is essential for anyone looking to streamline their development workflow and enhance their coding experience.
At its core, this error typically arises from a mismatch between the TypeScript compiler’s expectations and the actual structure of your project. Whether it’s due to missing type definitions, incorrect module paths, or even configuration mishaps, the implications can ripple through your codebase, affecting everything from functionality to maintainability. As developers, it’s crucial to grasp the nuances of module resolution and type declaration to avoid these pitfalls and ensure a smoother coding journey.
In the following sections, we will delve into the common causes of this error, explore effective troubleshooting strategies, and provide insights on best practices to prevent it from occurring in the future. By equipping yourself with this knowledge, you’ll be better prepared to tackle the challenges that arise in TypeScript development and keep your projects on track.
Understanding the Error Message
The error message “cannot find module or its corresponding type declarations” typically occurs in TypeScript projects when the TypeScript compiler cannot locate a specific module or its type definitions. This can hinder the development process, particularly when working with third-party libraries or custom modules.
Several factors can lead to this error:
- Missing Type Definitions: If a module lacks type definitions and TypeScript is unable to infer types, this error may arise.
- Incorrect Module Path: A typographical error in the module’s import path can prevent the compiler from locating the module.
- Node Modules Not Installed: If the required package is not installed in the `node_modules` directory, TypeScript will not be able to find it.
- Configuration Issues: Improper configuration in `tsconfig.json` may lead to TypeScript not recognizing certain directories or files.
How to Resolve the Error
Addressing the error can involve several approaches, depending on the underlying cause:
- Install Missing Type Definitions: For third-party libraries, ensure that appropriate type definitions are installed. This can often be done via DefinitelyTyped or the library’s own type definitions.
“`bash
npm install @types/library-name –save-dev
“`
- Check Import Path: Verify that the import statement accurately reflects the module’s path.
“`typescript
import { MyModule } from ‘./path/to/MyModule’; // Ensure the path is correct
“`
- Install the Module: If the module is not found in `node_modules`, install it using npm or yarn.
“`bash
npm install module-name
“`
- Update tsconfig.json: Make sure that the `tsconfig.json` file is correctly configured, particularly the `include` and `exclude` options.
“`json
{
“compilerOptions”: {
“target”: “es6”,
“module”: “commonjs”,
“strict”: true,
“esModuleInterop”: true
},
“include”: [“src/**/*”],
“exclude”: [“node_modules”, “**/*.spec.ts”]
}
“`
Common Scenarios and Solutions
In practice, developers often encounter specific scenarios that trigger this error. Below is a table summarizing common situations and their corresponding solutions:
Scenario | Solution |
---|---|
Missing type definitions for a library | Install the types using npm, e.g., npm install @types/library-name |
Incorrect file path in import statement | Verify and correct the import path in the code |
Module not installed | Run npm install module-name to install the missing module |
Exclusion of directories in tsconfig.json | Check and adjust the exclude settings in tsconfig.json |
By systematically addressing these factors, developers can effectively resolve the “cannot find module or its corresponding type declarations” error and enhance their TypeScript development experience.
Understanding the Error Message
The error message “cannot find module or its corresponding type declarations” typically indicates that TypeScript is unable to locate a specific module or its type definitions. This can occur in various situations:
- Module Not Installed: The required module may not be installed in the project.
- Incorrect Module Path: The path specified in the import statement may be incorrect.
- Missing Type Definitions: Type definitions for the module may not be available.
- TypeScript Configuration: The `tsconfig.json` file may not be correctly configured to include the module.
Troubleshooting Steps
To resolve this error, follow these troubleshooting steps:
- Verify Module Installation
Ensure that the module is installed in your project by running:
“`bash
npm list
“`
If the module is not listed, install it using:
“`bash
npm install
“`
- Check Import Path
Review the import statements in your TypeScript files to confirm that the paths are accurate. For example:
“`typescript
import { MyFunction } from ‘./path/to/module’;
“`
- Install Type Definitions
If the module does not include its own type definitions, install them separately. Many popular libraries have type definitions available in the DefinitelyTyped repository. You can install them using:
“`bash
npm install @types/
“`
- Update `tsconfig.json`
Ensure that your `tsconfig.json` file is correctly configured to include the necessary module types. You may need to adjust the `include` or `exclude` properties. For example:
“`json
{
“compilerOptions”: {
“moduleResolution”: “node”,
“esModuleInterop”: true,
“skipLibCheck”: true
},
“include”: [
“src/**/*”
]
}
“`
- Check for Local Type Declarations
If the module lacks type definitions, you can create a local declaration file (e.g., `declarations.d.ts`) and declare the module yourself:
“`typescript
declare module ‘
“`
Common Scenarios and Solutions
Scenario | Solution |
---|---|
Module not found | Install the module using npm |
Incorrect path in import statement | Correct the path in the import statement |
Missing type definitions | Install types from DefinitelyTyped or create a declaration file |
TypeScript configuration issues | Adjust `tsconfig.json` settings |
Best Practices to Avoid Future Issues
To minimize the occurrence of this error in the future, consider the following best practices:
- Use Absolute Imports: Configure your project to support absolute imports, which can help avoid path issues.
- Regularly Update Dependencies: Keep your dependencies and type definitions up to date to ensure compatibility.
- Use TypeScript’s Built-in Types: Leverage TypeScript’s built-in types whenever possible to reduce reliance on external modules.
- Document Dependencies: Maintain clear documentation of the dependencies used in your project, including their types.
By following these guidelines, you can effectively manage modules in TypeScript and reduce the likelihood of encountering “cannot find module or its corresponding type declarations” errors.
Expert Insights on Module Declaration Issues in TypeScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘cannot find module or its corresponding type declarations’ typically arises when TypeScript cannot locate the type definitions for a module. This can often be resolved by ensuring that the module is installed correctly and that the `@types` packages are available for any third-party libraries.”
James Liu (Lead TypeScript Developer, CodeCraft Solutions). “In my experience, this issue frequently occurs when the TypeScript configuration file (`tsconfig.json`) is not set up to include the paths to the node modules. Adjusting the `include` and `typeRoots` properties can significantly mitigate these errors.”
Sarah Thompson (Technical Consultant, DevExpert Advisors). “It is essential to verify that the module you are trying to import is correctly listed in your package.json and that it is compatible with your TypeScript version. Often, updating the module or TypeScript itself can resolve these declaration issues.”
Frequently Asked Questions (FAQs)
What does the error “cannot find module or its corresponding type declarations” mean?
This error indicates that the TypeScript compiler cannot locate the specified module or its type definitions. This often occurs when the module is not installed, or the TypeScript configuration does not include the necessary type declaration files.
How can I resolve the “cannot find module” error in TypeScript?
To resolve this error, ensure that the module is installed in your project. You can install it using npm or yarn. Additionally, check your `tsconfig.json` file for the correct paths and configurations.
What should I do if the module has no type definitions?
If the module lacks type definitions, you can create a declaration file (e.g., `module-name.d.ts`) in your project. Alternatively, you can use `declare module ‘module-name’;` to bypass type checking for that module.
Can I use JavaScript modules in a TypeScript project?
Yes, you can use JavaScript modules in a TypeScript project. However, you may encounter type-related errors. To mitigate this, you can either provide type definitions or use the `any` type for those modules.
How do I check if a module is installed correctly?
To verify if a module is installed correctly, check your `node_modules` directory and ensure the module folder exists. You can also run `npm list module-name` or `yarn list module-name` to confirm its installation.
What are type declaration files, and why are they important?
Type declaration files provide TypeScript with information about the types used in JavaScript modules. They are crucial for enabling type checking and IntelliSense features in TypeScript, improving code quality and developer experience.
The error message “cannot find module or its corresponding type declarations” typically arises in TypeScript when the compiler is unable to locate a specified module or its type definitions. This issue can occur due to several reasons, including incorrect module paths, missing type declaration files, or misconfigured TypeScript settings. Developers often encounter this error when integrating third-party libraries that lack proper type definitions or when the project’s directory structure does not align with the expected module resolution paths.
To resolve this error, developers should first verify the module’s path and ensure that it is correctly referenced in the import statement. If the module is a third-party library, checking for the existence of type declaration files, either within the library or through DefinitelyTyped, can be beneficial. Additionally, adjusting the `tsconfig.json` file to include the proper paths or enabling the `allowJs` option may help in recognizing JavaScript modules that do not have type declarations.
Key takeaways from this discussion include the importance of maintaining accurate module paths and ensuring that all necessary type declarations are present within a TypeScript project. Developers should also familiarize themselves with TypeScript’s configuration options to effectively manage module resolution. By addressing these aspects, one can significantly reduce the likelihood of encountering the “cannot find module
Author Profile
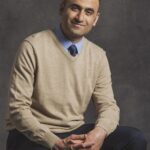
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?