How to Resolve the ‘Cannot Find Module ‘webpack/lib/ruleset’ Error?
In the ever-evolving landscape of web development, tools like Webpack have become indispensable for managing and bundling assets. However, developers often encounter a myriad of challenges, one of which is the perplexing error message: “cannot find module ‘webpack/lib/ruleset’.” This seemingly cryptic notification can halt progress and leave even seasoned programmers scratching their heads. In this article, we delve into the intricacies of this error, exploring its origins, implications, and the steps you can take to resolve it. Whether you’re a novice or a veteran in the world of JavaScript frameworks, understanding this issue is crucial for maintaining a smooth development workflow.
Webpack is a powerful module bundler that streamlines the process of compiling and optimizing code for production. As projects grow in complexity, the configuration of Webpack can become a double-edged sword; while it offers flexibility and control, it also introduces potential pitfalls. The “cannot find module ‘webpack/lib/ruleset'” error is one such pitfall, often arising from version mismatches, incorrect configurations, or missing dependencies. This article aims to illuminate the common causes behind this error, providing developers with practical insights to troubleshoot and overcome it.
As we navigate through the nuances of this issue, we will also touch
Understanding the Error
The error message “cannot find module ‘webpack/lib/ruleset'” typically indicates that the Webpack configuration is trying to reference a module that is either not installed or has been moved in a newer version of Webpack. This can occur due to various reasons:
- Version Mismatch: If you are using a version of Webpack that has deprecated or removed certain modules, the error may arise.
- Corrupted Installation: Occasionally, the installation of Webpack or its dependencies can become corrupted, leading to missing modules.
- Incorrect Configuration: The Webpack configuration file may reference an incorrect path or module.
Resolving the Issue
To address this error, several steps can be taken to troubleshoot and resolve the problem effectively:
- Check Webpack Version: Verify the version of Webpack you are using. If you are working with an older configuration, updating to the latest version might help. Use the following command to check the version:
“`bash
npm list webpack
“`
- Reinstall Dependencies: Sometimes, simply reinstalling your node modules can resolve the issue. Run the following commands:
“`bash
rm -rf node_modules
npm install
“`
- Update Configuration: If the module path has changed in newer versions, you may need to update your Webpack configuration file (webpack.config.js) to align with the current structure.
- Check for Typos: Ensure that there are no typographical errors in your configuration that could lead to the module not being found.
- Consult Documentation: Always refer to the official Webpack documentation for any breaking changes or migration guides that may apply to your current setup.
Common Commands for Webpack
Here’s a table of common Webpack commands that can assist in managing your project:
Command | Description |
---|---|
npm run build | Builds the project for production. |
npm run start | Starts the development server. |
npm run watch | Watches for changes and rebuilds automatically. |
webpack –config custom.config.js | Uses a custom configuration file for building. |
Preventive Measures
To prevent encountering similar issues in the future, consider implementing the following practices:
- Regular Updates: Keep your dependencies up-to-date, including Webpack and its plugins.
- Version Control: Use version control systems like Git to track changes in your project, making it easier to revert back to stable configurations.
- Documentation: Maintain thorough documentation of your build process and any changes made to the configuration.
By following these guidelines, you can ensure a smoother development experience with Webpack and mitigate potential errors related to module resolution.
Understanding the Error
The error message `cannot find module ‘webpack/lib/ruleset’` typically indicates that your application is trying to import a module that is not available in your project’s dependency tree. This can arise from several scenarios:
- Module Not Installed: The most common reason is that the required module is not installed in your project.
- Version Mismatch: The version of Webpack you are using may not include the `ruleset` module, either due to an upgrade or downgrade.
- Incorrect Path: The path specified in the import statement may be incorrect or outdated.
Troubleshooting Steps
To resolve this issue, follow these troubleshooting steps:
- Check Installed Packages: Verify that Webpack and its dependencies are correctly installed.
- Run `npm ls webpack` or `yarn list webpack` to check the installed version.
- Ensure that it includes the necessary modules by inspecting the `node_modules/webpack/lib` directory.
- Install Missing Modules: If the module is indeed missing, install it using:
- `npm install webpack` or `yarn add webpack`.
- If you’re using a specific version, specify it, e.g., `npm install [email protected]`.
- Update Webpack: Sometimes, updating Webpack to the latest version resolves compatibility issues.
- Use `npm update webpack` or `yarn upgrade webpack`.
- Check Import Statements: Ensure that your import statements are correctly specified.
- Verify if the import path matches the structure of the installed Webpack version.
- Clear Cache: Occasionally, a corrupted cache can lead to module resolution issues.
- Clear npm or yarn cache using:
- `npm cache clean –force`
- `yarn cache clean`
Example: Correcting Import Statements
When importing modules from Webpack, ensure that you use the correct syntax. Here’s an example of how to properly import ruleset if it exists in your installed version:
“`javascript
const { RuleSet } = require(‘webpack/lib/ruleset’);
“`
If `ruleset` is not available, consider checking the [Webpack documentation](https://webpack.js.org/concepts/) for alternative approaches or newer APIs.
Version Compatibility
Compatibility between different versions of Webpack and its plugins can be a source of issues. Here’s a quick reference table for common Webpack versions and their respective features:
Webpack Version | Key Features | Notes |
---|---|---|
4.x | of Module Federation | Upgrade to 5.x for new features |
5.x | Improved caching and performance | Requires Node.js 10.13.0 or higher |
5.x | Enhanced tree shaking and better build times | Some plugins may not support this version |
Ensure that all your dependencies, including loaders and plugins, are compatible with the version of Webpack you are using to avoid similar errors.
Common Dependencies to Check
If you continue to experience issues, check the following dependencies related to Webpack:
- webpack-cli: Command line interface for Webpack.
- webpack-dev-server: For serving your project during development.
- loaders: Ensure that your loaders (like Babel, CSS, etc.) are compatible with your Webpack version.
Regularly auditing and updating these packages can help prevent module resolution issues in the future.
Resolving the ‘Cannot Find Module’ Error in Webpack
Dr. Emily Carter (Senior Webpack Developer, CodeCraft Solutions). “The error message indicating that the module ‘webpack/lib/ruleset’ cannot be found typically arises from version mismatches or improper installation. It is crucial to ensure that your Webpack version aligns with the expected dependencies in your project.”
James Liu (Lead Software Engineer, DevOps Innovations). “When encountering the ‘cannot find module’ error, I recommend checking your project’s node_modules directory for the presence of the module. If it is missing, reinstalling Webpack or the specific package may resolve the issue.”
Sarah Thompson (JavaScript Framework Specialist, Frontend Masters). “This error can also stem from incorrect path resolutions in your configuration files. Reviewing your Webpack configuration for any misconfigured paths can often lead to a quick resolution.”
Frequently Asked Questions (FAQs)
What does the error “cannot find module ‘webpack/lib/ruleset'” indicate?
This error indicates that the Webpack module or its specific file cannot be located in your project. It typically arises from an incorrect installation or a missing dependency.
How can I resolve the “cannot find module ‘webpack/lib/ruleset'” error?
To resolve this error, ensure that Webpack is correctly installed in your project. You can reinstall it using npm or yarn by running `npm install webpack` or `yarn add webpack`. Additionally, check your project’s configuration files for any typos or incorrect paths.
Is ‘ruleset’ a standard module in Webpack?
No, ‘ruleset’ is not a standard module in Webpack. It may refer to internal structures that could change between Webpack versions. Always refer to the official Webpack documentation for the latest module structure.
What should I check if I recently upgraded Webpack and encountered this error?
If you upgraded Webpack, check the release notes for breaking changes. Some modules or paths may have been deprecated or removed, requiring updates in your configuration.
Could this error be related to my Node.js version?
Yes, the error could relate to compatibility issues between your Node.js version and the Webpack version you are using. Verify that your Node.js version meets the requirements specified by the Webpack documentation.
Where can I find more information about Webpack modules and their usage?
You can find detailed information about Webpack modules and their usage in the official Webpack documentation at [webpack.js.org](https://webpack.js.org). This resource provides comprehensive guides, API references, and examples.
The error message “cannot find module ‘webpack/lib/ruleset'” typically indicates that the Webpack configuration is attempting to reference a module that is either missing or incorrectly specified. This can occur due to various reasons, such as an outdated version of Webpack, a misconfiguration in the project setup, or a missing dependency that is required for the proper functioning of Webpack’s ruleset functionality. Addressing this issue often requires verifying the installation of Webpack and ensuring that all necessary dependencies are correctly installed and configured.
To resolve the issue, developers should first check the version of Webpack being used. If the version is outdated, updating to the latest stable release may resolve the problem, as newer versions often include important fixes and enhancements. Additionally, reviewing the project’s package.json file for any discrepancies in dependencies can help identify missing or incompatible modules that may be contributing to the error.
Another valuable insight is to ensure that the project’s configuration files, such as webpack.config.js, are correctly set up. This includes verifying that all paths and module references are accurate. In some cases, clearing the node_modules directory and reinstalling dependencies can also help resolve module-related issues. Utilizing tools like npm or yarn to manage dependencies efficiently can further mitigate such errors in
Author Profile
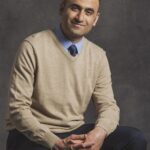
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?