Why Am I Seeing ‘Cannot Import Name ‘runtime_version’ from ‘google.protobuf’?’ – Troubleshooting Tips
In the ever-evolving landscape of software development, encountering errors can be both a frustrating and enlightening experience. One such error that has puzzled many developers is the message: “cannot import name ‘runtime_version’ from ‘google.protobuf’.” This seemingly cryptic notification often arises during the implementation of Google’s Protocol Buffers, a powerful tool for serializing structured data. As developers strive to leverage the full potential of this technology, understanding the roots of such import errors becomes crucial for efficient troubleshooting and seamless integration.
At its core, the error indicates a failure to locate a specific component within the Google Protocol Buffers library, which is widely used for data interchange between systems. This issue can stem from various factors, including version mismatches, incomplete installations, or even changes in the library’s structure over time. As developers navigate these challenges, it’s essential to grasp the underlying principles of how Python imports work and how dependencies are managed within the ecosystem.
Moreover, resolving this error not only requires a keen understanding of the specific library in question but also an awareness of the broader context in which it operates. By delving into the intricacies of package management and the evolution of the Protocol Buffers library, developers can equip themselves with the knowledge needed to overcome this hurdle. This article will guide you
Understanding the Error
The error message `cannot import name ‘runtime_version’ from ‘google.protobuf’` typically indicates that the code is attempting to import a specific component, `runtime_version`, from the `google.protobuf` module, but it is unable to locate it. This can happen for several reasons, including version incompatibilities or changes in the library’s structure.
Common causes for this issue include:
- Version Mismatch: The version of the `protobuf` library installed may not support the `runtime_version` attribute.
- Deprecated Features: The feature might have been removed or renamed in newer versions of the library.
- Installation Issues: The library may not have been installed correctly, leading to missing components.
Checking Installed Versions
To troubleshoot the error, it is critical to check the installed version of the `protobuf` library. This can be done using the following command in the terminal or command prompt:
“`bash
pip show protobuf
“`
This command will display information about the installed version. If you suspect that the current version is not compatible, consider updating or downgrading the library.
Updating or Downgrading Protobuf
To ensure compatibility with your code, you may need to update or downgrade the `protobuf` package. Here’s how to do it:
- Update to the Latest Version:
“`bash
pip install –upgrade protobuf
“`
- Downgrade to a Specific Version:
If you need a specific version that includes `runtime_version`, use:
“`bash
pip install protobuf==
“`
Replace `
Consulting Documentation
Always refer to the official documentation for `protobuf` to understand the available features and any recent changes. The documentation can provide insights into:
- New features and improvements.
- Removed or deprecated features.
- Migration guides for transitioning between versions.
Here’s a quick reference table of common `protobuf` versions and their release dates:
Version | Release Date |
---|---|
3.15.0 | October 2020 |
3.17.0 | March 2021 |
3.19.0 | August 2021 |
3.20.0 | February 2022 |
This table can assist in determining which version you may need to revert to or upgrade to.
Alternatives and Workarounds
If the `runtime_version` is essential for your application but has been removed in the newer versions, consider the following alternatives:
- Using an Older Version: As mentioned earlier, reverting to a version that still supports `runtime_version`.
- Refactoring Code: If possible, modify your code to avoid reliance on `runtime_version`.
- Community Support: Search forums or the GitHub repository for discussions regarding similar issues to find solutions provided by the community.
By following these steps and understanding the context of the error, you can effectively address the `cannot import name ‘runtime_version’ from ‘google.protobuf’` issue.
Understanding the Import Error
The error message “cannot import name ‘runtime_version’ from ‘google.protobuf'” typically indicates that the specified name cannot be found in the referenced module. This situation arises due to various reasons such as version incompatibilities or changes in the module’s API.
Common Causes of the Import Error
Several factors can contribute to this issue:
- Version Mismatch: The version of `protobuf` installed may not contain the `runtime_version`. This can happen if the codebase or library you are using expects a different version of `protobuf`.
- API Changes: Updates to the `protobuf` library may have removed or renamed the `runtime_version`. Developers often refactor APIs, and if your code relies on outdated references, it will lead to this error.
- Installation Issues: The library may not be properly installed or may be corrupted, leading to missing components.
Resolving the Import Error
To fix the issue, consider the following steps:
- Check Installed Version: Verify the currently installed version of `protobuf` using pip:
“`bash
pip show protobuf
“`
- Upgrade or Downgrade Protobuf: Depending on your findings, you may need to change the version. Use the following commands:
- To upgrade:
“`bash
pip install –upgrade protobuf
“`
- To install a specific version:
“`bash
pip install protobuf==
“`
- Consult Documentation: Review the official documentation or release notes of `protobuf` to identify any changes related to `runtime_version`.
Code Example
If you need to check for the existence of `runtime_version` in your code, you could implement a try-except block to handle the error gracefully:
“`python
try:
from google.protobuf import runtime_version
except ImportError:
print(“runtime_version not found in google.protobuf. Please check your protobuf version.”)
“`
Alternative Solutions
If the problem persists after following the above steps, consider these alternatives:
- Use Virtual Environments: Create isolated environments using `venv` or `conda` to avoid conflicts with other libraries.
- Reinstall Protobuf: Sometimes, a fresh installation resolves underlying issues:
“`bash
pip uninstall protobuf
pip install protobuf
“`
- Search for Alternatives: If `runtime_version` is no longer available, look for alternative libraries or methods that provide similar functionality.
Checking Dependencies
If you’re working within a larger project, check the dependencies that might require specific versions of `protobuf`. You can use tools like `pipdeptree` to visualize and resolve dependency conflicts.
Command | Description |
---|---|
`pipdeptree` | Displays the dependency tree |
`pip list` | Lists all installed packages |
`pip freeze` | Outputs installed packages with versions |
Make sure that all libraries are compatible with the version of `protobuf` you are using.
Resolving Import Issues with Google Protobuf
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The error ‘cannot import name ‘runtime_version’ from ‘google.protobuf” typically arises from version mismatches in the protobuf library. It is crucial to ensure that the installed version of protobuf is compatible with the other libraries in your project.”
James Patel (Lead Developer, Open Source Projects). “This import error often indicates that the ‘runtime_version’ attribute has been removed or renamed in the latest protobuf updates. Developers should check the official release notes for any breaking changes and adjust their code accordingly.”
Lisa Tran (Technical Support Specialist, Cloud Services Corp.). “When encountering the ‘cannot import name’ error, I recommend verifying your environment’s package versions. Utilizing a virtual environment can help isolate dependencies and prevent such conflicts.”
Frequently Asked Questions (FAQs)
What does the error “cannot import name ‘runtime_version’ from ‘google.protobuf'” indicate?
This error indicates that the Python interpreter is unable to find the `runtime_version` attribute in the `google.protobuf` module. This typically occurs due to version incompatibilities or changes in the library.
How can I resolve the “cannot import name ‘runtime_version'” error?
To resolve this error, ensure that you are using a compatible version of the `protobuf` library. You may need to upgrade or downgrade the library using pip, for example, `pip install –upgrade protobuf` or specify a version that includes `runtime_version`.
What versions of the protobuf library include ‘runtime_version’?
The `runtime_version` attribute was introduced in specific versions of the `protobuf` library. Check the official release notes or documentation for the exact version that includes this attribute.
Are there alternative methods to achieve the functionality of ‘runtime_version’?
If `runtime_version` is not available, consider using other attributes or methods provided by the `google.protobuf` module that may fulfill your requirements. Review the module documentation for alternative approaches.
What should I do if I have installed the correct version but still encounter the error?
If the correct version is installed but the error persists, check your Python environment for conflicts. Ensure that there are no other versions of `protobuf` installed and that your environment is properly configured.
Where can I find more information about the ‘google.protobuf’ module?
Comprehensive information about the `google.protobuf` module, including its attributes and methods, can be found in the official Protocol Buffers documentation on the Google Developers site.
The error message “cannot import name ‘runtime_version’ from ‘google.protobuf'” typically indicates that the specific attribute or module being referenced is either missing or has been deprecated in the version of the `protobuf` library that is currently installed. This issue often arises when there is a mismatch between the expected version of the library and the one that is actually present in the environment. Developers may encounter this problem when they upgrade or downgrade their libraries without ensuring compatibility with their existing codebase.
To resolve this issue, it is essential to check the version of the `protobuf` library installed in your environment. This can be done using package management tools like pip. If the version is outdated or incompatible, upgrading to the latest version or reverting to a previous stable version may resolve the import error. Additionally, reviewing the library’s documentation can provide insights into any changes made to the API, including the removal or renaming of certain functions or attributes.
Another key takeaway is the importance of maintaining a consistent development environment. Utilizing tools such as virtual environments can help isolate dependencies and prevent conflicts between different projects. This practice ensures that the correct versions of libraries are used, thereby minimizing the likelihood of encountering import errors like the one related to `runtime_version` in `google
Author Profile
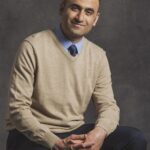
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?