Why Can’t I Resolve a Scoped Service from the Root Provider?
In the world of modern software development, particularly within the realm of ASP.NET Core, dependency injection (DI) stands as a cornerstone of building robust, maintainable applications. However, as developers dive deeper into the intricacies of DI, they often encounter a perplexing issue: the dreaded error message, “cannot resolve scoped service from root provider.” This seemingly cryptic notification can halt progress and lead to frustration, but understanding its roots is essential for any developer looking to master the art of DI in their applications.
At its core, this error arises from the way services are registered and resolved within the DI container. In ASP.NET Core, services can be registered with different lifetimes—transient, scoped, and singleton—each serving a unique purpose in the lifecycle of an application. The crux of the issue lies in the fact that scoped services are designed to operate within a specific context, typically tied to a single HTTP request. When developers attempt to access these scoped services from a singleton context, they inadvertently breach the intended lifecycle, resulting in the error message that raises eyebrows and questions.
Navigating this challenge requires a solid understanding of the DI framework and the implications of service lifetimes. By unpacking the nuances of service registration and resolution, developers can not only troubleshoot this error but
Understanding Scoped Services in Dependency Injection
Scoped services are instantiated once per request within the scope of an application. This means that when a request comes in, a new instance of the scoped service is created, and it remains alive for the duration of that request. However, when attempting to resolve a scoped service from the root provider, developers often encounter the error: “cannot resolve scoped service from root provider.”
This error typically occurs in applications using dependency injection frameworks, such as ASP.NET Core. The root provider, which is created at application startup, is designed to manage singleton services and does not have the context necessary to create instances of scoped services.
Why Scoped Services Cannot Be Resolved from the Root Provider
The inability to resolve scoped services from the root provider is primarily due to the lifecycle management of services in dependency injection. Here are the key reasons:
- Lifecycle Management: Scoped services have a lifecycle that is tied to individual requests. The root provider does not have knowledge of the current request context.
- Performance Considerations: Allowing scoped services to be resolved from the root provider could lead to inefficiencies and memory leaks, as instances could persist longer than intended.
- Thread Safety: Scoped services are not thread-safe across multiple requests; hence, if accessed from the root provider, it could lead to race conditions.
Best Practices to Avoid the Error
To avoid the “cannot resolve scoped service from root provider” error, consider the following best practices:
- Use Constructor Injection: Ensure that scoped services are injected into classes that are instantiated within the request scope, such as controllers or middleware.
- Service Factory: Use a service factory to create instances of scoped services when needed. This allows for the correct resolution of dependencies within the appropriate scope.
“`csharp
public class MyController : ControllerBase
{
private readonly IScopedService _scopedService;
public MyController(IScopedService scopedService)
{
_scopedService = scopedService;
}
}
“`
- Avoid Resolving Scoped Services in Singletons: If a singleton service requires access to a scoped service, consider redesigning the architecture. Pass the necessary data instead of the service itself.
Common Scenarios Leading to the Error
The following table outlines common scenarios that trigger the “cannot resolve scoped service from root provider” error:
Scenario | Explanation |
---|---|
Injecting Scoped Services into Singleton Services | Scoped services cannot be directly injected into singletons as they are not tied to the request lifecycle. |
Using Scoped Services in Static Methods | Static methods do not have access to the request context, leading to resolution issues. |
Accessing Scoped Services in Middleware | Middleware should be designed to handle request-scoped dependencies correctly. |
By understanding the lifecycle of services and adhering to best practices, developers can effectively manage scoped services within their applications without encountering resolution issues.
Understanding Scoped Services
Scoped services in dependency injection are designed to be instantiated once per request within the scope of a web application. This means that if a service is registered as scoped, it is created when a request starts and disposed of when the request ends. This is particularly useful for services that require a context, such as database connections, which can be expensive to create and manage.
Common Causes of the Error
The error message “cannot resolve scoped service from root provider” typically arises in scenarios where a scoped service is requested from a singleton or the root service provider. This situation leads to several issues:
- Incorrect Lifetimes: Mixing lifetimes of services can cause problems. Singleton services should not depend on scoped services.
- Service Resolution Context: Attempting to resolve a scoped service outside of an HTTP request or in a static context results in this error.
Best Practices to Resolve the Issue
To effectively handle the “cannot resolve scoped service from root provider” error, consider the following best practices:
- Use Dependency Injection Properly: Ensure that you inject services at the correct level. Scoped services should only be injected into other scoped or transient services.
- Avoid Static Contexts: Do not attempt to resolve scoped services in static methods or constructors as they do not have a request context.
- Service Factory: Utilize a factory pattern to create an instance of the scoped service when needed. This can be achieved by:
“`csharp
public class MyService
{
private readonly IServiceScopeFactory _scopeFactory;
public MyService(IServiceScopeFactory scopeFactory)
{
_scopeFactory = scopeFactory;
}
public void SomeMethod()
{
using (var scope = _scopeFactory.CreateScope())
{
var scopedService = scope.ServiceProvider.GetRequiredService
// Use scopedService as needed
}
}
}
“`
Example of Dependency Injection Configuration
When configuring services in an ASP.NET Core application, ensure that the lifetimes are appropriately set:
“`csharp
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped
services.AddSingleton
// Ensure no scoped service is injected into a singleton service directly
}
“`
Debugging Tips
If you encounter this error, consider the following debugging steps:
- Check Service Lifetimes: Review the registration of your services to ensure lifetimes are compatible.
- Examine Call Stack: Trace back to where the service is being requested, ensuring it’s within a valid scope.
- Logging: Implement logging to capture when and where the service resolution fails to gain insights into the context.
By adhering to these best practices and understanding the lifetimes of your services, you can mitigate the chances of encountering the “cannot resolve scoped service from root provider” error. Implementing these strategies not only enhances the stability of your application but also promotes a cleaner architecture.
Understanding the Challenges of Scoped Services in Dependency Injection
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The error ‘cannot resolve scoped service from root provider’ typically arises when attempting to resolve a service that is registered with a scoped lifetime from a singleton context. This is a fundamental aspect of dependency injection that developers must understand to avoid common pitfalls in application architecture.”
Michael Thompson (Senior Developer, CodeCraft Solutions). “When you encounter the ‘cannot resolve scoped service from root provider’ error, it’s crucial to revisit your service registration. Scoped services should only be resolved within the context of a request, and attempting to access them from a singleton or static context will lead to this error. Properly managing your service lifetimes is essential for a stable application.”
Jessica Lin (Lead Engineer, CloudTech Systems). “This error highlights the importance of understanding the lifecycle of services in dependency injection frameworks. Scoped services are designed for specific contexts, such as web requests, and cannot be accessed globally. Developers should consider using factory patterns or redesigning their service architecture to ensure proper service resolution and avoid this issue.”
Frequently Asked Questions (FAQs)
What does “cannot resolve scoped service from root provider” mean?
This error indicates that a service registered with a scoped lifetime is being requested from a singleton or root service provider, which does not have access to the scoped service.
How can I resolve the “cannot resolve scoped service from root provider” error?
To resolve this error, ensure that the scoped service is being requested within a valid scope, such as within an HTTP request context or by creating a new scope explicitly using the service provider.
What are the differences between singleton, scoped, and transient service lifetimes?
Singleton services are created once and shared throughout the application’s lifetime. Scoped services are created once per request or scope, while transient services are created each time they are requested.
When should I use scoped services in my application?
Scoped services are ideal for operations that require a consistent state throughout a single request, such as database contexts, where you want to maintain a single instance for the duration of that request.
Can I inject a scoped service into a singleton service?
Injecting a scoped service directly into a singleton service is not recommended. Instead, consider using a factory or service locator pattern to resolve the scoped service within a valid scope.
What is the impact of improperly managing service lifetimes in dependency injection?
Improper management of service lifetimes can lead to memory leaks, unintended behavior, and runtime exceptions, as services may not be disposed of correctly or may access invalid contexts.
The error message “cannot resolve scoped service from root provider” typically arises in dependency injection frameworks, particularly in ASP.NET Core. This issue occurs when a service that is registered with a scoped lifetime is being requested from a singleton or root service provider. Scoped services are designed to be created once per request, while singleton services are created once and shared throughout the application’s lifetime. Therefore, attempting to resolve a scoped service from a singleton context leads to this conflict.
To address this issue, developers should ensure that scoped services are only resolved within the appropriate context, such as within a controller or a middleware that is handling a request. If a singleton service needs to use a scoped service, it is advisable to pass the scoped service as a parameter to the singleton service’s methods or to use a factory pattern to create the scoped service instance as needed. This approach maintains the integrity of the service lifetimes and prevents runtime errors.
In summary, understanding the lifetimes of services in dependency injection is crucial for effective application design. Developers should be mindful of the context in which services are resolved and ensure that scoped services are not inadvertently accessed from singleton instances. By adhering to these principles, applications can avoid the “cannot resolve scoped service from root provider” error and maintain
Author Profile
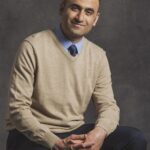
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?