Why Can’t We Compare Offset-Naive and Offset-Aware Datetimes in Python?
In the world of programming, particularly in Python, working with dates and times can often feel like navigating a labyrinth. Among the myriad of challenges developers face, one of the most perplexing is the distinction between offset-naive and offset-aware datetimes. This seemingly simple concept can lead to significant confusion and errors if not properly understood. Whether you’re managing timestamps for a global application or simply logging events in your local timezone, grasping the nuances of these two types of datetime objects is crucial for ensuring accurate and reliable time handling in your projects.
At the heart of the issue lies the fundamental difference between offset-naive and offset-aware datetimes. Offset-naive datetimes do not contain any timezone information, making them suitable for local contexts but potentially problematic when dealing with data from multiple time zones. On the other hand, offset-aware datetimes are equipped with timezone information, allowing for precise calculations and comparisons across different regions. This distinction is vital for developers who need to ensure that their applications behave consistently, regardless of where users are located.
As we delve deeper into this topic, we’ll explore the implications of using these two types of datetimes in Python, the common pitfalls that developers encounter, and best practices for managing time effectively. By the end of this article, you’ll be equipped with the knowledge
Understanding Offset-Naive and Offset-Aware Datetimes
Offset-naive and offset-aware datetimes are two distinct types of date and time representations in Python, specifically within the `datetime` module. Understanding the difference between these two types is crucial for effective time manipulation and comparison.
Offset-naive datetimes do not contain information about the time zone or offset from UTC (Coordinated Universal Time). They simply represent a specific point in time without any contextual information about its location in time zones. For example:
“`python
from datetime import datetime
naive_dt = datetime(2023, 10, 5, 15, 0) 3:00 PM on October 5, 2023
“`
In contrast, offset-aware datetimes include information about the time zone or UTC offset, which allows for accurate comparisons across different time zones. An example of an offset-aware datetime is:
“`python
from datetime import datetime, timezone, timedelta
aware_dt = datetime(2023, 10, 5, 15, 0, tzinfo=timezone.utc) 3:00 PM UTC on October 5, 2023
“`
Comparing Offset-Naive and Offset-Aware Datetimes
When attempting to compare offset-naive and offset-aware datetimes directly, Python raises a `TypeError`. This is because such comparisons are inherently ambiguous—an offset-naive datetime does not provide enough context to determine its relationship to an offset-aware datetime.
Key Points:
- TypeError: Attempting to compare a naive datetime with an aware datetime results in an error.
- Ambiguity: The lack of timezone information in naive datetimes creates potential for confusion in comparisons.
To avoid this error, you should either convert offset-naive datetimes to offset-aware or vice versa, ensuring both datetimes have the same level of timezone awareness.
Conversion Techniques
To perform comparisons without raising errors, you can convert naive datetimes to aware datetimes by specifying a timezone. Here’s how you can do this:
- Convert Naive to Aware:
“`python
from datetime import timezone
naive_dt = datetime(2023, 10, 5, 15, 0)
aware_dt = naive_dt.replace(tzinfo=timezone.utc) Make naive aware by assigning UTC
“`
- Convert Aware to Naive:
“`python
aware_dt = datetime(2023, 10, 5, 15, 0, tzinfo=timezone.utc)
naive_dt = aware_dt.astimezone(timezone.utc).replace(tzinfo=None) Remove timezone info
“`
Practical Example of Comparison
Here is a practical example demonstrating the comparison between naive and aware datetimes after converting them appropriately:
“`python
Naive datetime
naive_dt = datetime(2023, 10, 5, 15, 0)
Aware datetime
aware_dt = datetime(2023, 10, 5, 15, 0, tzinfo=timezone.utc)
Convert naive to aware
naive_as_aware = naive_dt.replace(tzinfo=timezone.utc)
Comparison
if naive_as_aware == aware_dt:
print(“The datetimes are equal.”)
else:
print(“The datetimes are not equal.”)
“`
Type | Example | Description |
---|---|---|
Naive | datetime(2023, 10, 5, 15, 0) | No timezone information |
Aware | datetime(2023, 10, 5, 15, 0, tzinfo=timezone.utc) | Includes timezone information |
By understanding the distinctions and employing the correct conversion methods, you can manage and compare datetimes effectively in Python.
Understanding Offset-Naive and Offset-Aware Datetimes
In Python, the `datetime` module provides two types of datetime objects: offset-naive and offset-aware.
- Offset-Naive Datetimes: These are datetime objects without any timezone information. They are treated as local time and can lead to ambiguity, especially when dealing with different time zones.
- Offset-Aware Datetimes: These datetime objects include timezone information, allowing for unambiguous representation of times across various time zones.
Common Issues with Comparison
When attempting to compare offset-naive and offset-aware datetime objects, Python raises a `TypeError`. This is because the operations between these two types are not directly compatible.
- TypeError Example:
“`python
from datetime import datetime, timezone
naive_dt = datetime(2023, 10, 1, 12, 0) Offset-naive
aware_dt = datetime(2023, 10, 1, 12, 0, tzinfo=timezone.utc) Offset-aware
result = naive_dt < aware_dt Raises TypeError ```
Converting Between Types
To compare these datetime objects, one must convert them to the same type. Below are methods for handling each case:
- Converting Offset-Naive to Offset-Aware:
- Use `replace(tzinfo=…)` to attach a timezone.
- Example:
“`python
from datetime import timezone, timedelta
naive_dt = datetime(2023, 10, 1, 12, 0)
aware_dt = naive_dt.replace(tzinfo=timezone.utc) Now offset-aware
“`
- Converting Offset-Aware to Offset-Naive:
- Use `astimezone()` to convert to a desired timezone and then get the naive version.
- Example:
“`python
aware_dt = datetime(2023, 10, 1, 12, 0, tzinfo=timezone.utc)
naive_dt = aware_dt.astimezone(timezone.utc).replace(tzinfo=None) Now offset-naive
“`
Comparison Best Practices
When comparing datetimes, follow these best practices to avoid errors:
- Always ensure both datetimes are of the same type before comparison.
- Use UTC as a common standard timezone for storing and comparing times.
- When converting, be aware of daylight saving time changes that may affect local times.
Example Code for Safe Comparison
Here is an example demonstrating safe comparison between naive and aware datetimes:
“`python
from datetime import datetime, timezone
naive_dt = datetime(2023, 10, 1, 12, 0)
aware_dt = datetime(2023, 10, 1, 12, 0, tzinfo=timezone.utc)
Convert naive to aware
naive_as_aware = naive_dt.replace(tzinfo=timezone.utc)
Now compare
if naive_as_aware < aware_dt:
print("Naive datetime is earlier than aware datetime.")
else:
print("Naive datetime is not earlier than aware datetime.")
```
This approach ensures that comparisons are valid and avoids the pitfalls of mixing datetime types.
Understanding the Limitations of Datetime Comparisons in Python
Dr. Emily Chen (Senior Data Scientist, Tech Innovations Inc.). “When working with datetime objects in Python, it’s crucial to recognize that offset-naive and offset-aware datetimes serve different purposes. Comparing them directly can lead to unexpected results, as offset-naive datetimes do not contain timezone information, while offset-aware datetimes do. This fundamental difference means that a direct comparison is not only illogical but also raises errors in many cases.”
Michael Thompson (Lead Software Engineer, Cloud Solutions Corp.). “In Python, the datetime module is designed to handle both offset-naive and offset-aware datetimes, but they cannot be compared directly. This is because the absence of timezone information in naive datetimes leads to ambiguity. Developers must convert naive datetimes to aware ones or vice versa before making comparisons to ensure accuracy and avoid runtime exceptions.”
Dr. Sarah Patel (Professor of Computer Science, University of Technology). “The inability to compare offset-naive and offset-aware datetimes in Python stems from the need for consistency in timezone representation. Without a common reference point, such comparisons can yield misleading results. It is advisable to standardize all datetimes to a single format, typically UTC, before performing any comparisons to maintain data integrity.”
Frequently Asked Questions (FAQs)
What are offset-naive and offset-aware datetimes in Python?
Offset-naive datetimes do not contain any timezone information, while offset-aware datetimes include a timezone offset that indicates their relation to UTC. This distinction is crucial for accurate date and time manipulations.
Why can’t I compare offset-naive and offset-aware datetimes directly?
Comparing offset-naive and offset-aware datetimes directly raises a `TypeError` in Python. This error occurs because the two types represent different concepts of time, making them incompatible for direct comparison.
How can I convert an offset-naive datetime to an offset-aware datetime?
You can convert an offset-naive datetime to an offset-aware datetime using the `pytz` library or the `datetime` module. Use the `localize()` method from the `pytz` library or the `replace(tzinfo=…)` method from the `datetime` module to assign a timezone.
What should I do if I need to compare an offset-naive datetime with an offset-aware datetime?
To compare them, convert the offset-naive datetime to an offset-aware datetime by assigning an appropriate timezone. Ensure both datetimes are in the same timezone before performing the comparison.
Can I use the `datetime` module’s `astimezone()` method for comparison?
Yes, the `astimezone()` method can convert an offset-aware datetime to a different timezone. However, it cannot be used directly on offset-naive datetimes. First, convert the naive datetime to aware, then use `astimezone()` for comparison.
What are the best practices for handling datetimes in Python?
Best practices include always using offset-aware datetimes when dealing with timezones, consistently applying timezone conversions, and utilizing libraries like `pytz` or `dateutil` for robust timezone handling.
In Python, datetime objects can be classified into two categories: offset-naive and offset-aware. Offset-naive datetime objects do not contain any timezone information, while offset-aware datetime objects include this information, allowing them to represent specific moments in time across different time zones accurately. Attempting to compare these two types of datetime objects directly will result in a TypeError, as Python does not allow comparisons between objects that lack a common basis for comparison.
The inability to compare offset-naive and offset-aware datetime objects arises from the fundamental differences in how they handle time. Offset-naive datetimes are treated as local times without any reference to a specific timezone, leading to ambiguity when interpreting their meaning in a global context. In contrast, offset-aware datetimes are explicitly tied to a timezone, making them more suitable for applications that require precise time calculations across different regions. This distinction is crucial for developers working with datetime data in Python, especially in applications involving scheduling, logging, or any functionality that relies on accurate timekeeping.
To effectively manage and compare datetime objects in Python, it is essential to ensure that both objects are either offset-naive or offset-aware before performing any operations. Developers can convert offset-naive datetimes to offset-aware by associating them
Author Profile
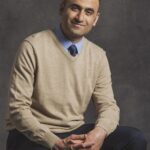
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?