How Can You Capture the HTML of a Link Without Opening It?
In an age where information is just a click away, the ability to efficiently gather data from the web has become increasingly essential. Whether you’re a developer looking to scrape data for analysis, a researcher compiling resources, or simply a curious individual wanting to explore content without the hassle of navigating through multiple tabs, the need to capture the HTML of a link without opening it has never been more relevant. This technique not only saves time but also enhances productivity by allowing users to extract and analyze web content seamlessly.
Capturing the HTML of a link without directly opening it involves leveraging various tools and methods that can fetch the underlying code of a webpage. This process can be particularly useful for those who want to avoid the distractions of a full browser experience or for situations where accessing a webpage may not be feasible due to restrictions or slow internet connections. By understanding the principles behind this technique, users can unlock a new level of efficiency in their web interactions.
In the following sections, we will explore the various approaches and tools available for capturing HTML content, from simple command-line utilities to more advanced programming techniques. We will also discuss the ethical considerations and best practices to keep in mind when scraping web data, ensuring that your efforts are both effective and responsible. Join us as we delve into the world of web scraping and discover
Methods to Capture HTML Without Opening a Link
Capturing the HTML content of a link without opening it can be achieved through various methods, including the use of programming languages, command-line tools, or web scraping libraries. Each method has its advantages and can be selected based on the user’s skill level and requirements.
Using Programming Languages
Programming languages like Python and JavaScript offer libraries that facilitate the retrieval of web page content without the need for a browser interface.
- Python: The `requests` library is a popular choice for fetching HTML content. Combined with `BeautifulSoup`, it allows for easy parsing and manipulation of the HTML.
“`python
import requests
from bs4 import BeautifulSoup
url = ‘http://example.com’
response = requests.get(url)
html_content = response.text
Parse HTML
soup = BeautifulSoup(html_content, ‘html.parser’)
print(soup.prettify())
“`
- JavaScript (Node.js): The `axios` library or `node-fetch` can be used to retrieve HTML from a URL.
“`javascript
const axios = require(‘axios’);
async function fetchHTML(url) {
const response = await axios.get(url);
console.log(response.data);
}
fetchHTML(‘http://example.com’);
“`
Command-Line Tools
For those who prefer command-line interfaces, tools like `curl` and `wget` are effective for capturing HTML.
- curl: This tool can be used to fetch HTML content easily.
“`bash
curl http://example.com -o output.html
“`
- wget: This command is handy for downloading entire web pages or sites.
“`bash
wget -q -O output.html http://example.com
“`
Web Scraping Libraries
Web scraping libraries offer powerful features for extracting HTML and data from web pages.
Library | Language | Key Features |
---|---|---|
BeautifulSoup | Python | HTML parsing, easy navigation |
Scrapy | Python | Framework for large-scale scraping |
Puppeteer | JavaScript | Headless browser for dynamic content |
These libraries enable developers to automate the process of HTML retrieval and manipulation.
Considerations for Ethical Scraping
When capturing HTML from websites, it is crucial to adhere to ethical guidelines:
- Check the robots.txt file: This file specifies which parts of the site can be crawled.
- Respect the website’s terms of service: Ensure compliance with legal and ethical standards.
- Limit the frequency of requests: Avoid overwhelming the server to prevent denial of service.
By following these methods and considerations, users can effectively capture the HTML of a link without the need for opening it in a web browser.
Methods to Capture HTML of a Link Without Opening It
Capturing the HTML of a link without directly opening it can be achieved through various methods. These methods utilize different tools and programming techniques to request the HTML content from a server.
Using Command Line Tools
Command line tools can be effective for fetching the HTML of a webpage. Here are a few popular options:
- cURL: A command-line tool for transferring data with URLs.
- Example command:
“`bash
curl -o output.html http://example.com
“`
- This command saves the HTML content of `http://example.com` to a file named `output.html`.
- Wget: A free utility for non-interactive download of files from the web.
- Example command:
“`bash
wget -O output.html http://example.com
“`
- Similar to cURL, this command retrieves the HTML and stores it in `output.html`.
Using Programming Languages
Programming languages such as Python and JavaScript can automate the process of capturing HTML.
– **Python with Requests**:
- The Requests library simplifies HTTP requests.
- Example code:
“`python
import requests
response = requests.get(‘http://example.com’)
html_content = response.text
with open(‘output.html’, ‘w’) as file:
file.write(html_content)
“`
– **JavaScript with Node.js**:
- The Axios library can be used to fetch HTML in a Node.js environment.
- Example code:
“`javascript
const axios = require(‘axios’);
const fs = require(‘fs’);
axios.get(‘http://example.com’)
.then(response => {
fs.writeFileSync(‘output.html’, response.data);
})
.catch(error => {
console.error(error);
});
“`
Using Web Scraping Libraries
For more complex tasks, web scraping libraries can handle HTML extraction while managing additional functionalities such as handling cookies or sessions.
– **Beautiful Soup (Python)**:
- Ideal for parsing HTML and XML documents.
- Example:
“`python
from bs4 import BeautifulSoup
import requests
response = requests.get(‘http://example.com’)
soup = BeautifulSoup(response.text, ‘html.parser’)
print(soup.prettify())
“`
– **Cheerio (Node.js)**:
- A fast and flexible library for manipulating HTML.
- Example:
“`javascript
const cheerio = require(‘cheerio’);
const axios = require(‘axios’);
axios.get(‘http://example.com’)
.then(response => {
const $ = cheerio.load(response.data);
console.log($.html());
});
“`
Utilizing Online Services
For users who prefer a no-code approach, online services can capture and display HTML content without requiring programming knowledge.
- Web-based services:
- Websites like `Fetch URL` allow users to input a URL and receive the HTML content.
- This method is straightforward and often user-friendly.
Service | Description |
---|---|
Fetch URL | Enter a URL to get HTML response. |
HTML Snapshot | Capture a snapshot of a webpage. |
Considerations and Best Practices
When capturing HTML without opening a link, consider the following:
- Respect Robots.txt: Always check the site’s `robots.txt` file to ensure that web scraping is allowed.
- Rate Limiting: Avoid sending too many requests in a short time to prevent being blocked by the server.
- User-Agent String: Some servers may block requests based on the user agent. Customize headers if necessary to mimic a browser.
By employing these methods, users can effectively capture HTML content from links without the need to open them directly.
Expert Insights on Capturing HTML Without Direct Access
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “Capturing the HTML of a link without opening it can be effectively achieved through the use of web scraping tools and libraries such as Beautiful Soup or Scrapy. These tools allow developers to fetch and parse HTML content programmatically, providing a robust solution for data extraction without the need for a browser interface.”
Michael Chen (Cybersecurity Analyst, SecureNet Solutions). “While it is technically feasible to capture HTML from a link without opening it, one must be cautious of ethical and legal implications. Implementing such techniques should always comply with the website’s terms of service and relevant laws to avoid potential violations of data privacy regulations.”
Sarah Thompson (Digital Marketing Strategist, MarketWise Agency). “Utilizing APIs provided by websites is an excellent method to retrieve HTML content without directly accessing the link. Many platforms offer public APIs that allow users to fetch data in a structured format, which can be more efficient and reliable than traditional scraping methods.”
Frequently Asked Questions (FAQs)
What methods can be used to capture HTML of a link without opening it?
You can use command-line tools like `curl` or `wget`, which allow you to fetch the HTML content of a URL without rendering it in a browser. Additionally, programming languages such as Python can utilize libraries like `requests` or `BeautifulSoup` to scrape HTML data programmatically.
Is it possible to capture HTML using browser developer tools?
While browser developer tools typically require the page to be opened, you can simulate a network request in some tools, such as Chrome’s DevTools, by using the “Fetch/XHR” panel. However, this still involves loading the page in some capacity.
Are there any online services that can capture HTML without opening a link?
Yes, there are online services and APIs, such as URL2PNG or Webpage to PDF converters, that can fetch and return the HTML content of a webpage without requiring you to open it in a browser.
Can I capture HTML from a link using a script?
Absolutely. You can write a script in languages like Python, JavaScript (Node.js), or PHP that sends an HTTP request to the URL and retrieves the HTML content. This method allows for automation and flexibility in handling multiple URLs.
What are the legal considerations when capturing HTML from a link?
It is essential to review the website’s terms of service and robots.txt file to ensure compliance with their policies regarding web scraping. Capturing HTML without permission may violate copyright laws or terms of use.
Does capturing HTML without opening a link affect website performance?
Generally, capturing HTML through automated requests can impact website performance, especially if done excessively. Websites may implement rate limiting or blocking mechanisms to prevent abuse, so it’s advisable to scrape responsibly and ethically.
Capturing the HTML of a link without opening it involves utilizing various techniques that allow users to retrieve web content programmatically. This can be achieved through methods such as using command-line tools like `curl` or `wget`, employing programming languages with HTTP libraries, or utilizing web scraping tools. Each of these methods enables users to access the HTML content of a webpage without the need for a browser interface, thereby preserving system resources and enhancing efficiency.
Moreover, it is crucial to consider the ethical implications and legalities associated with web scraping. Many websites have terms of service that prohibit automated access to their content. Therefore, users should ensure they are compliant with these regulations and respect robots.txt files, which provide guidelines on how and whether a site can be crawled. Understanding these aspects not only safeguards against potential legal issues but also fosters responsible usage of web data.
In summary, capturing HTML without opening a link is a valuable skill for developers, researchers, and data analysts. By leveraging the right tools and adhering to ethical standards, users can efficiently gather and analyze web content. This practice can significantly enhance productivity and facilitate the extraction of valuable insights from online resources.
Author Profile
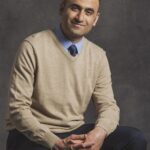
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?