How Can You Use a Case Statement in a Where Clause?
In the world of SQL, crafting precise queries is essential for extracting meaningful insights from vast datasets. One powerful tool that often goes unnoticed is the use of the CASE statement within the WHERE clause. This versatile feature allows developers to implement conditional logic directly in their queries, enabling them to filter records based on dynamic criteria. Whether you’re a seasoned database administrator or a budding data analyst, understanding how to leverage the CASE statement can significantly enhance your querying capabilities and streamline your data retrieval processes.
At its core, the CASE statement provides a way to introduce conditional logic into SQL queries, allowing for more complex and nuanced filtering of data. By integrating CASE into the WHERE clause, users can create conditions that adapt based on the values in the dataset, thus enabling more tailored and relevant results. This approach not only simplifies queries but also enhances their readability, making it easier for others to understand the logic behind the data selection.
Moreover, the ability to use CASE within the WHERE clause opens up a realm of possibilities for data analysis. It empowers users to address scenarios that would otherwise require multiple queries or cumbersome joins. By mastering this technique, you can elevate your SQL skills and gain deeper insights from your data, all while writing cleaner and more efficient code. As we delve deeper into the intricacies of the CASE statement,
Understanding Case Statements in Where Clauses
In SQL, the `CASE` statement is a powerful tool that allows for conditional logic to be implemented within queries. While it is commonly used in `SELECT` statements to derive calculated fields, it can also be effectively employed within `WHERE` clauses to filter records based on specific conditions.
The `CASE` statement evaluates a list of conditions and returns a value when the first condition is met. If no conditions are met, it can return a default value. This feature is particularly useful in scenarios where multiple filtering criteria are necessary.
Syntax of Case Statement in Where Clauses
The basic syntax for using a `CASE` statement in a `WHERE` clause is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE
column_name = CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END;
“`
This structure allows you to implement complex filtering logic in a concise manner.
Examples of Case Statements in Where Clauses
Consider a scenario where you want to filter records based on a status column that can have multiple values. Here are examples illustrating how to use a `CASE` statement in a `WHERE` clause.
Example 1: Filter based on customer status
“`sql
SELECT customer_id, customer_name
FROM customers
WHERE customer_status = CASE
WHEN membership_level = ‘Gold’ THEN ‘Active’
WHEN membership_level = ‘Silver’ THEN ‘Pending’
ELSE ‘Inactive’
END;
“`
In this example, customers are filtered based on their membership level, returning those with a status of ‘Active’, ‘Pending’, or ‘Inactive’.
Example 2: Filter based on sales figures
“`sql
SELECT sale_id, sale_amount
FROM sales
WHERE sale_amount > CASE
WHEN season = ‘Holiday’ THEN 1000
ELSE 500
END;
“`
This query filters sales records based on the season, using different thresholds for holiday and non-holiday periods.
Advantages of Using Case Statements in Where Clauses
Utilizing `CASE` statements in `WHERE` clauses offers several benefits:
- Flexibility: Allows for dynamic filtering based on various conditions without needing multiple `WHERE` clauses.
- Readability: Improves the clarity of SQL queries by consolidating logic into a single structure.
- Maintainability: Simplifies updates to conditional logic, as changes can be made in one location.
Common Use Cases
The use of `CASE` statements in `WHERE` clauses is prevalent in various scenarios, including:
- User Role-Based Filtering: Adjusting data visibility based on user permissions.
- Dynamic Reporting: Generating reports that reflect different criteria based on parameters provided by users.
Use Case | Description |
---|---|
User Role-Based Filtering | Filter data depending on user roles, allowing different views of the same dataset. |
Dynamic Reporting | Generate reports tailored to user-defined criteria, enhancing user experience. |
In summary, incorporating `CASE` statements within `WHERE` clauses enhances the ability to write more complex and dynamic SQL queries, making data retrieval more efficient and tailored to specific needs.
Understanding the Case Statement
The CASE statement in SQL allows for conditional logic within queries, enabling users to perform complex evaluations and transformations on data. Although typically used in SELECT clauses, it can also be effectively utilized in WHERE clauses, enhancing query flexibility.
Syntax of Case Statement in Where Clause
The syntax for incorporating a CASE statement within a WHERE clause generally follows this structure:
“`sql
SELECT column1, column2
FROM table_name
WHERE
(CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE result_default
END) = some_value;
“`
This format allows for conditional evaluations that determine whether a row should be included in the result set based on the specified conditions.
Examples of Case Statement in Where Clause
Here are some practical examples that illustrate the use of the CASE statement within a WHERE clause.
**Example 1: Filtering Based on Conditions**
In this example, we filter employees based on their position and the corresponding salary range.
“`sql
SELECT employee_id, name
FROM employees
WHERE
(CASE
WHEN position = ‘Manager’ THEN salary
WHEN position = ‘Developer’ THEN salary * 0.9
ELSE 0
END) > 50000;
“`
Example 2: Dynamic Filtering
This query demonstrates dynamic filtering based on a user-defined parameter.
“`sql
SELECT product_id, product_name
FROM products
WHERE
(CASE
WHEN category = ‘Electronics’ THEN stock
WHEN category = ‘Clothing’ THEN stock * 1.5
ELSE stock
END) < 100;
```
Use Cases for Case Statement in Where Clause
Utilizing the CASE statement in a WHERE clause can be beneficial in various scenarios:
- Dynamic Business Rules: Adjusting filters based on varying business criteria, allowing for more tailored query results.
- Conditional Aggregation: Enabling complex logic in filtering records for aggregation functions.
- Enhanced Readability: Improving query readability by consolidating multiple conditions into a single structure.
Considerations and Performance Implications
While incorporating a CASE statement in a WHERE clause provides flexibility, it is essential to consider the following aspects:
- Performance: The use of CASE statements can impact query performance, particularly on large datasets, as they may prevent the use of indexes effectively.
- Complexity: Overusing CASE statements can lead to complex queries that are harder to read and maintain.
- Database Compatibility: Ensure that the SQL dialect you are using supports the CASE statement in a WHERE clause, as some databases may have limitations.
Aspect | Consideration |
---|---|
Performance | May slow down queries on large datasets |
Complexity | Can lead to challenging readability |
Compatibility | Check SQL dialect support for CASE in WHERE clause |
Using a CASE statement in a WHERE clause enables advanced data manipulation and conditional filtering in SQL queries. Proper understanding and implementation can yield more efficient and dynamic data retrieval strategies.
Expert Insights on Using Case Statements in Where Clauses
Dr. Emily Chen (Database Architect, Data Solutions Inc.). “Incorporating a CASE statement within a WHERE clause can significantly enhance query flexibility. It allows for conditional logic directly in filtering, which can simplify complex queries and improve readability when used judiciously.”
Mark Thompson (Senior SQL Developer, Tech Innovations Group). “While using a CASE statement in a WHERE clause can be powerful, it is essential to consider performance implications. Depending on the database engine, this approach may lead to inefficient query plans if not optimized properly.”
Lisa Patel (Data Analyst, Insight Analytics). “The ability to use a CASE statement in a WHERE clause opens up new possibilities for data filtering. However, it is crucial to ensure that the logic remains straightforward to avoid confusion for those who may maintain the code later.”
Frequently Asked Questions (FAQs)
What is a case statement in SQL?
A case statement in SQL is a conditional expression that allows you to perform conditional logic within your queries. It evaluates a set of conditions and returns a specific value when the first condition is met.
Can a case statement be used in the WHERE clause?
Yes, a case statement can be used in the WHERE clause to create conditional filtering. It allows you to apply different conditions based on the values of columns in your database.
What is the syntax for using a case statement in the WHERE clause?
The syntax typically involves using the CASE keyword followed by conditions and their corresponding results. For example:
`WHERE column_name = CASE WHEN condition1 THEN result1 ELSE result2 END`
Are there any performance implications when using a case statement in the WHERE clause?
Using a case statement in the WHERE clause can impact performance, especially with large datasets, as it may require additional processing. It is advisable to test and optimize queries for efficiency.
Can you provide an example of a case statement in the WHERE clause?
Certainly. For instance:
`SELECT * FROM employees WHERE department_id = CASE WHEN job_title = ‘Manager’ THEN 1 ELSE 2 END;`
This example filters employees based on their job title.
What are the limitations of using a case statement in the WHERE clause?
Limitations include complexity in readability and potential performance issues. Additionally, case statements in the WHERE clause can make it harder to optimize queries for the database engine.
The use of a CASE statement in a WHERE clause is a powerful feature in SQL that allows for conditional logic within queries. By incorporating CASE, developers can create more dynamic and flexible filtering criteria. This capability is particularly useful when dealing with complex datasets where the filtering conditions may vary based on certain criteria or values within the data itself. The ability to evaluate multiple conditions and return specific results enhances the precision of data retrieval.
One of the key advantages of utilizing a CASE statement in the WHERE clause is the simplification of complex conditional logic. Instead of writing multiple OR conditions, a single CASE statement can encapsulate the logic, making the SQL query more readable and maintainable. This can lead to improved performance, as the database engine can optimize the execution of the query more effectively when the logic is clearly defined.
Moreover, using a CASE statement in the WHERE clause can significantly enhance the versatility of queries. It allows for the incorporation of business rules directly into the SQL statements, enabling developers to tailor their data retrieval processes to meet specific requirements. This versatility is essential in environments where data conditions frequently change, ensuring that queries remain relevant and effective over time.
the integration of a CASE statement in the WHERE clause is a valuable technique
Author Profile
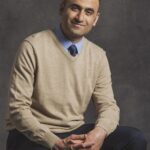
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?