How Can You Use a Case Statement in a Where Condition?
In the realm of SQL, the ability to manipulate and filter data is paramount for effective database management and analysis. One of the most powerful tools at a developer’s disposal is the `CASE` statement, which allows for conditional logic within queries. While many are familiar with its application in `SELECT` statements, its integration into the `WHERE` clause often remains an underutilized gem. Understanding how to leverage the `CASE` statement in `WHERE` conditions can significantly enhance the flexibility and precision of your queries, enabling you to filter data based on complex criteria.
The `CASE` statement serves as a versatile mechanism for implementing conditional logic directly in SQL queries. By incorporating it into the `WHERE` clause, developers can create dynamic filtering conditions that adapt based on the values of other fields. This capability not only simplifies complex queries but also enhances readability and maintainability, making it easier to understand the logic behind data retrieval.
Moreover, using a `CASE` statement in the `WHERE` condition allows for more nuanced data analysis. Instead of relying solely on straightforward comparisons, developers can craft intricate conditions that reflect business rules or specific analytical needs. This approach empowers users to derive insights that would be challenging to achieve through traditional filtering methods, paving the way for more informed decision-making and strategic planning
Using Case Statements in Where Conditions
In SQL, the `CASE` statement is a powerful tool that allows for conditional logic within queries. While `CASE` is commonly used in the `SELECT` clause to return different values based on conditions, it can also be employed within the `WHERE` clause to filter records conditionally. This method enhances the flexibility of your queries, enabling you to apply complex logic directly within your filtering criteria.
The basic syntax for using a `CASE` statement in the `WHERE` clause is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1
AND (CASE
WHEN condition2 THEN value1
WHEN condition3 THEN value2
ELSE default_value
END) = desired_value;
“`
Practical Examples
Consider a scenario where you have a sales database, and you want to filter records based on different sales regions. You can use a `CASE` statement to set the criteria depending on the region’s performance.
“`sql
SELECT sales_rep, total_sales
FROM sales_data
WHERE (CASE
WHEN region = ‘North’ AND total_sales > 10000 THEN ‘High’
WHEN region = ‘South’ AND total_sales > 15000 THEN ‘High’
ELSE ‘Low’
END) = ‘High’;
“`
In this example, the query retrieves sales representatives whose sales are classified as ‘High’ based on their region.
Benefits of Using Case Statements in Where Conditions
– **Dynamic Filtering**: Allows for more dynamic and complex filtering logic than simple boolean conditions.
– **Readability**: Can improve the readability of SQL queries by consolidating conditions.
– **Flexibility**: Provides the ability to evaluate multiple conditions in a single statement.
Considerations
While using `CASE` in the `WHERE` clause can be beneficial, it’s important to consider performance implications, especially in large datasets. Here are some key points to keep in mind:
– **Index Usage**: Complex conditions may prevent the use of indexes, leading to slower queries.
– **Readability**: Overusing `CASE` statements can make queries harder to read and maintain.
Example of Multiple Conditions
You can also use the `CASE` statement to handle multiple conditions more succinctly:
“`sql
SELECT employee_id, salary
FROM employees
WHERE (CASE
WHEN department = ‘Sales’ THEN salary * 0.1
WHEN department = ‘Engineering’ THEN salary * 0.2
ELSE 0
END) > 5000;
“`
This query filters employees based on their department and associated bonus conditions.
Summary Table of `CASE` in Where Clause
Condition | Action |
---|---|
region = ‘North’ AND total_sales > 10000 | Classify as High |
region = ‘South’ AND total_sales > 15000 | Classify as High |
Else | Classify as Low |
utilizing a `CASE` statement in the `WHERE` clause can significantly enhance the capability of SQL queries, allowing for intricate and conditional data retrieval strategies.
Understanding Case Statements in WHERE Conditions
Using a CASE statement within a WHERE condition allows for dynamic filtering based on various criteria. This approach is particularly useful when dealing with multiple potential conditions that dictate which records should be returned.
Syntax of CASE Statement in WHERE Clause
The general syntax for incorporating a CASE statement in a WHERE clause is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1
AND (CASE
WHEN condition2 THEN result1
WHEN condition3 THEN result2
ELSE result3
END) = some_value;
“`
In this structure, the CASE statement evaluates several conditions and returns a specific result based on the first condition that is met.
Examples of CASE Statements in WHERE Conditions
- **Basic Usage Example:**
Suppose you have a sales table with a column `sales_status`. You want to filter records based on different sales statuses:
“`sql
SELECT *
FROM sales
WHERE (CASE
WHEN sales_status = ‘completed’ THEN 1
WHEN sales_status = ‘pending’ THEN 2
ELSE 0
END) > 0;
“`
- Multiple Conditions Example:
In a scenario where you need to filter employees based on their department and status, you can use:
“`sql
SELECT employee_id, employee_name
FROM employees
WHERE (CASE
WHEN department = ‘HR’ AND status = ‘active’ THEN ‘HR Active’
WHEN department = ‘IT’ AND status = ‘inactive’ THEN ‘IT Inactive’
ELSE ‘Other’
END) = ‘HR Active’;
“`
Considerations When Using CASE in WHERE
- Performance: Using CASE statements can impact query performance, especially if the dataset is large. Always evaluate if simpler conditions could achieve the same result.
- Readability: While CASE statements can enhance flexibility, overcomplicating the WHERE clause may reduce readability. Aim for clarity in your SQL code.
- NULL Handling: Be mindful of how NULL values are treated within CASE statements. Explicitly check for NULLs if necessary to avoid unexpected results.
Common Use Cases
- Dynamic Filtering: Adjusting filters based on user input or application logic.
- Complex Logic: Handling intricate business rules that require multiple conditional checks.
- Data Transformation: When filtering based on transformed data values derived from existing columns.
Performance Optimization Tips
Technique | Description |
---|---|
Indexing | Ensure that the columns involved in the CASE statement are indexed to speed up searches. |
Simplifying Logic | Break complex conditions into simpler, separate queries if possible. |
Analyze Execution Plan | Use tools to analyze how the query is executed and make adjustments based on performance metrics. |
By understanding the implementation and implications of using CASE statements in WHERE clauses, SQL practitioners can enhance their querying capabilities to meet complex business requirements effectively.
Expert Insights on Using Case Statements in Where Conditions
Dr. Emily Carter (Database Architect, Data Solutions Inc.). “Utilizing a CASE statement within a WHERE condition can enhance the flexibility of SQL queries, allowing for more complex filtering based on conditional logic. This approach is particularly beneficial when dealing with multiple criteria that depend on varying conditions.”
Michael Tran (Senior Data Analyst, Insight Analytics). “Incorporating CASE statements in WHERE clauses can significantly streamline query logic. However, it is essential to ensure that performance is not adversely affected, especially with large datasets, as this can lead to slower execution times.”
Jessica Lee (SQL Consultant, Query Masters). “While CASE statements are powerful tools for conditional filtering, they should be used judiciously. Overcomplicating WHERE conditions can lead to maintenance challenges and reduced readability, making it harder for teams to understand and modify queries in the future.”
Frequently Asked Questions (FAQs)
What is a case statement in SQL?
A case statement in SQL is a conditional expression that allows you to evaluate a set of conditions and return a specific value based on the first condition that is met.
Can a case statement be used in a WHERE clause?
Yes, a case statement can be used in a WHERE clause to create complex filtering conditions based on multiple criteria.
How does a case statement in a WHERE clause work?
In a WHERE clause, the case statement evaluates conditions and returns a boolean value, which determines whether a row should be included in the result set based on the specified criteria.
What is the syntax for using a case statement in a WHERE clause?
The syntax typically follows this structure: `WHERE (CASE WHEN condition1 THEN result1 WHEN condition2 THEN result2 ELSE resultN END) = some_value`.
Are there performance considerations when using case statements in WHERE clauses?
Yes, using case statements can impact performance, especially with large datasets, as they may complicate query execution plans. It’s advisable to test performance and consider alternative approaches if necessary.
Can you provide an example of a case statement in a WHERE clause?
Certainly. An example would be: `SELECT * FROM employees WHERE (CASE WHEN department = ‘Sales’ THEN salary ELSE 0 END) > 50000;` This filters employees based on their salary in the Sales department.
The use of a CASE statement within a WHERE condition is a powerful SQL feature that allows for conditional logic to be applied when filtering records from a database. This capability enhances the flexibility of queries, enabling developers to create more dynamic and context-sensitive data retrieval processes. By incorporating a CASE statement, users can evaluate multiple conditions and return specific values that dictate whether a row should be included in the result set based on complex criteria.
One of the key advantages of utilizing a CASE statement in the WHERE clause is its ability to simplify complex queries. Instead of writing multiple OR conditions or subqueries, a single CASE statement can encapsulate the necessary logic, making the SQL code more readable and maintainable. This can significantly reduce the potential for errors and improve query performance by streamlining the evaluation process.
Moreover, the CASE statement enhances the versatility of SQL queries by allowing for dynamic comparisons based on varying input values. This is particularly useful in scenarios where the filtering criteria may change based on user input or application logic. As a result, developers can create more responsive applications that adapt to different contexts without the need for extensive query modifications.
the integration of a CASE statement in the WHERE condition is an invaluable technique for SQL developers. It not
Author Profile
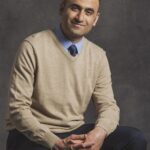
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?