How Can You Cast a String to Date in SQL?
In the world of database management, the ability to manipulate and convert data types is crucial for effective data analysis and reporting. One common challenge that developers and analysts face is how to cast a string to a date in SQL. This seemingly straightforward task can become complex, especially when dealing with various date formats, regional settings, and database systems. Understanding the intricacies of date conversion not only enhances data integrity but also ensures that your queries yield accurate results.
Casting a string to a date in SQL involves transforming text representations of dates into a format that the database can recognize and work with. Different SQL dialects offer a variety of functions and methods to achieve this, each with its own syntax and capabilities. Whether you’re working with MySQL, SQL Server, PostgreSQL, or Oracle, grasping the nuances of date formatting is essential for avoiding errors and ensuring seamless data operations.
As you delve into the specifics of casting strings to dates, you’ll discover the importance of understanding date formats, handling exceptions, and leveraging built-in functions to streamline the process. This knowledge not only empowers you to write more efficient SQL queries but also enhances your overall data manipulation skills, paving the way for more robust data-driven decision-making. Prepare to unlock the secrets of date conversion and elevate your SQL proficiency!
Common SQL Functions for Casting Strings to Dates
In SQL, converting a string to a date format is commonly achieved using specific functions that vary by database system. Below are some of the most prevalent SQL functions across different platforms:
- CAST(): This standard SQL function is used to convert a value from one data type to another, including converting strings to dates.
- CONVERT(): Particularly used in SQL Server, this function allows for more specific formatting options when casting strings to dates.
- TO_DATE(): A function utilized in Oracle databases, which requires a format string to parse the input correctly.
- STR_TO_DATE(): This MySQL function allows the conversion of strings to dates using a specified format.
Using the CAST Function
The `CAST()` function can be employed to convert a string to a date in a straightforward manner. The syntax is as follows:
“`sql
CAST(expression AS data_type)
“`
For example, to convert a string to a date:
“`sql
SELECT CAST(‘2023-10-01’ AS DATE);
“`
This command will convert the string ‘2023-10-01’ into a date format.
Using the CONVERT Function in SQL Server
In SQL Server, the `CONVERT()` function provides additional formatting options:
“`sql
CONVERT(data_type, expression [, style])
“`
The `style` parameter allows you to define the output format. For instance:
“`sql
SELECT CONVERT(DATE, ’10/01/2023′, 101);
“`
This converts the string ’10/01/2023′ to a date using the MM/DD/YYYY format.
Using the TO_DATE Function in Oracle
Oracle’s `TO_DATE()` function requires both the string and the format model:
“`sql
TO_DATE(string, format)
“`
Example usage:
“`sql
SELECT TO_DATE(’01-OCT-2023′, ‘DD-MON-YYYY’) FROM dual;
“`
This converts the string ’01-OCT-2023′ into an Oracle date type.
Using STR_TO_DATE in MySQL
In MySQL, the `STR_TO_DATE()` function is essential for converting strings to dates with a specified format:
“`sql
STR_TO_DATE(string, format)
“`
Example:
“`sql
SELECT STR_TO_DATE(’31-12-2023′, ‘%d-%m-%Y’);
“`
This command will convert ’31-12-2023′ to a date type based on the provided format.
Considerations for Date Conversion
When converting strings to dates, consider the following:
- Format Compatibility: Ensure the string format matches the expected date format of the function you are using.
- Database Settings: Some databases may have specific settings that affect date formats.
- Error Handling: Be aware of potential errors when the input string does not conform to the expected format.
Database | Function | Example |
---|---|---|
SQL Server | CONVERT() | CONVERT(DATE, ’10/01/2023′, 101) |
Oracle | TO_DATE() | TO_DATE(’01-OCT-2023′, ‘DD-MON-YYYY’) |
MySQL | STR_TO_DATE() | STR_TO_DATE(’31-12-2023′, ‘%d-%m-%Y’) |
Standard SQL | CAST() | CAST(‘2023-10-01’ AS DATE) |
Methods to Cast String to Date in SQL
Casting a string to a date in SQL can vary based on the specific SQL dialect being used. Below are some common methods and functions across different database systems.
Using CAST Function
The `CAST` function is a standard SQL function that converts one data type into another.
Syntax:
“`sql
CAST(expression AS target_data_type)
“`
Example:
“`sql
SELECT CAST(‘2023-10-01’ AS DATE) AS converted_date;
“`
This will convert the string `’2023-10-01’` to a date format.
Using CONVERT Function
The `CONVERT` function is often used in SQL Server and Sybase for type conversion.
Syntax:
“`sql
CONVERT(target_data_type, expression, style)
“`
Example:
“`sql
SELECT CONVERT(DATE, ’10/01/2023′, 101) AS converted_date;
“`
In this example, `101` specifies the style format (MM/DD/YYYY).
Using STR_TO_DATE Function
In MySQL, the `STR_TO_DATE` function is used to convert strings into date values based on a specified format.
Syntax:
“`sql
STR_TO_DATE(string, format)
“`
Example:
“`sql
SELECT STR_TO_DATE(’01-10-2023′, ‘%d-%m-%Y’) AS converted_date;
“`
Here, the format specifies that the input string is in the day-month-year format.
Using TO_DATE Function
In Oracle, the `TO_DATE` function is used to convert a string to a date.
Syntax:
“`sql
TO_DATE(string, format)
“`
Example:
“`sql
SELECT TO_DATE(‘2023-10-01’, ‘YYYY-MM-DD’) AS converted_date FROM dual;
“`
This example converts the string to a date using the specified format.
Common Format Specifiers
When converting strings to dates, it is crucial to use the correct format specifiers. Below is a table of common specifiers used in various SQL dialects:
Specifier | Description |
---|---|
`%Y` | Year (4 digits) |
`%y` | Year (2 digits) |
`%m` | Month (01-12) |
`%d` | Day of the month (01-31) |
`%H` | Hour (00-23) |
`%i` | Minutes (00-59) |
`%s` | Seconds (00-59) |
Handling Invalid Date Formats
When casting strings to dates, invalid formats can lead to errors or null values. Consider the following approaches:
- Using TRY_CAST/TRY_CONVERT in SQL Server: These functions return null instead of throwing an error.
“`sql
SELECT TRY_CAST(‘invalid_date’ AS DATE) AS converted_date;
“`
- Using Exception Handling in PL/SQL (Oracle): Wrap the conversion in a block to handle exceptions gracefully.
“`sql
BEGIN
SELECT TO_DATE(‘invalid_date’, ‘YYYY-MM-DD’) INTO v_date FROM dual;
EXCEPTION
WHEN OTHERS THEN
v_date := NULL;
END;
“`
Ensure proper validation of input strings before attempting to convert them to avoid runtime errors and data inconsistencies.
Expert Insights on Casting Strings to Dates in SQL
Dr. Emily Carter (Database Architect, Data Solutions Inc.). “When casting strings to dates in SQL, it is crucial to ensure that the string format matches the expected date format of the database system being used. For instance, SQL Server uses the CONVERT function, while PostgreSQL employs the TO_DATE function. Understanding these nuances can prevent data integrity issues.”
James Liu (Senior SQL Developer, Tech Innovations Group). “One common pitfall when converting strings to dates is not accounting for locale-specific formats. Always validate your string data before conversion, as discrepancies in date formats can lead to unexpected errors or incorrect data entries in your database.”
Sarah Thompson (Data Analyst, Insight Analytics). “Using the correct casting function is essential for successful string-to-date conversions. In MySQL, the STR_TO_DATE function is particularly useful, allowing for flexible date formats. Properly leveraging these functions can significantly enhance data manipulation and reporting capabilities.”
Frequently Asked Questions (FAQs)
How can I cast a string to a date in SQL?
You can cast a string to a date in SQL using the `CAST` or `CONVERT` functions. For example, `CAST(‘2023-10-01’ AS DATE)` or `CONVERT(DATE, ‘2023-10-01’, 120)` will convert the string to a date format.
What formats can be used when casting strings to dates?
The acceptable formats for casting strings to dates depend on the SQL database system. Common formats include ‘YYYY-MM-DD’, ‘MM/DD/YYYY’, and ‘DD-MM-YYYY’. Always refer to the specific database documentation for supported formats.
What happens if the string is not in a valid date format?
If the string is not in a valid date format, SQL will typically return an error or NULL, depending on the database system and the settings for error handling.
Can I use the `TO_DATE` function for casting strings to dates?
Yes, in databases like Oracle, you can use the `TO_DATE` function. For example, `TO_DATE(‘2023-10-01’, ‘YYYY-MM-DD’)` converts the string to a date using the specified format.
Is it possible to cast a string with a time component to a date?
Yes, you can cast a string with a time component to a date. The time portion will be ignored when casting to a date type. For example, `CAST(‘2023-10-01 12:30:00’ AS DATE)` will result in ‘2023-10-01’.
Are there any performance implications when casting strings to dates?
Casting strings to dates can have performance implications, especially in large datasets. It is advisable to ensure that the strings are in the correct format before casting to minimize errors and optimize query performance.
In SQL, casting a string to a date is a common operation that allows for the conversion of textual representations of dates into a date data type. This process is essential for performing date-related operations, comparisons, and calculations. Different SQL databases have their specific functions and syntax for casting strings to dates, such as the `CAST()` and `CONVERT()` functions in SQL Server, or the `TO_DATE()` function in Oracle. Understanding the correct format for the string is crucial, as it must align with the expected date format of the database system to ensure successful conversion.
One of the key insights is that the format of the date string must be compatible with the database’s date format settings. For instance, while some databases may accept date strings in the ‘YYYY-MM-DD’ format, others might require ‘MM/DD/YYYY’ or ‘DD-MON-YYYY’. Additionally, using the appropriate functions to handle different formats can prevent errors and improve the efficiency of queries. It is also important to consider the impact of locale settings, as these can influence how dates are interpreted and displayed.
Another takeaway is the importance of error handling when casting strings to dates. Invalid date formats can lead to runtime errors, which can disrupt the execution of SQL queries
Author Profile
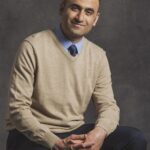
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?