How Can You Change the Center Position of a Rectangle?
In the world of graphic design and digital art, precision is key. Whether you’re creating a stunning visual for a website, developing a game, or designing an app interface, the placement of elements can significantly impact the overall aesthetics and functionality of your project. One common challenge that designers face is the need to adjust the center position of rectangles—an essential shape used in countless applications. Understanding how to effectively change the center position of a rectangle can enhance your design workflow, allowing for greater creativity and efficiency. In this article, we will explore the various methods and techniques to manipulate the center position of rectangles, ensuring that your designs are both visually appealing and perfectly aligned.
When working with rectangles, especially in graphic design software or programming environments, the center position often serves as a crucial reference point. This position determines how the rectangle interacts with other elements on the canvas and can affect animations, transitions, and overall layout. By mastering the techniques to change the center position, designers can easily reposition rectangles without compromising their intended design or layout.
Moreover, understanding the mathematical principles behind center positioning can empower designers to create more dynamic and responsive layouts. From simple adjustments in design software to more complex calculations in coding environments, the ability to manipulate the center position of rectangles opens up a world
Understanding Rectangles in Graphics Programming
In graphics programming, a rectangle (or “rect”) is typically defined by its position and dimensions, consisting of width and height. The position of a rectangle is commonly represented by its top-left corner coordinates. However, in many applications, it can be more intuitive to think in terms of the rectangle’s center point. This shift in perspective allows for easier calculations when positioning the rectangle relative to other graphical elements.
Calculating the Center Position
To change the center position of a rectangle, one must first understand how to calculate the center based on its current position and dimensions. The center of a rectangle can be determined using the following formula:
- Center X = Top-Left X + (Width / 2)
- Center Y = Top-Left Y + (Height / 2)
To reposition the rectangle to a new center, the new top-left coordinates can be calculated as follows:
- New Top-Left X = New Center X – (Width / 2)
- New Top-Left Y = New Center Y – (Height / 2)
This ensures that the rectangle’s dimensions remain unchanged while its center moves to the desired coordinates.
Example Calculations
Consider a rectangle defined by the following parameters:
- Width: 100 pixels
- Height: 50 pixels
- Current Top-Left Position: (30, 20)
To find the current center:
- Current Center X = 30 + (100 / 2) = 80
- Current Center Y = 20 + (50 / 2) = 45
If we want to move the center to a new position, say (100, 100), the new top-left coordinates would be:
- New Top-Left X = 100 – (100 / 2) = 50
- New Top-Left Y = 100 – (50 / 2) = 75
Now, the rectangle would be positioned at (50, 75) with its center at (100, 100).
Implementation in Code
When implementing this logic in code, it is essential to encapsulate the calculations into functions for reusability. Here’s a simple example in Python:
“`python
class Rectangle:
def __init__(self, top_left_x, top_left_y, width, height):
self.top_left_x = top_left_x
self.top_left_y = top_left_y
self.width = width
self.height = height
def center(self):
center_x = self.top_left_x + (self.width / 2)
center_y = self.top_left_y + (self.height / 2)
return (center_x, center_y)
def move_center(self, new_center_x, new_center_y):
self.top_left_x = new_center_x – (self.width / 2)
self.top_left_y = new_center_y – (self.height / 2)
“`
Common Use Cases
Adjusting the center position of rectangles is particularly useful in various scenarios, such as:
- Game Development: Positioning characters, obstacles, or UI elements relative to the center.
- User Interface Design: Aligning graphical components based on user interactions.
- Data Visualization: Adjusting the placement of graphical representations in charts or graphs.
Summary of Key Points
Aspect | Details |
---|---|
Center Calculation | Top-Left X + (Width / 2), Top-Left Y + (Height / 2) |
New Position Calculation | New Center X – (Width / 2), New Center Y – (Height / 2) |
Common Applications | Game development, UI design, data visualization |
By understanding these calculations and their applications, developers can efficiently manage the positioning of rectangles within their graphical environments.
Changing the Center Position of a Rectangle
Modifying the center position of a rectangle is a common task in graphic design and programming. Depending on the context, there are different methods to achieve this. Below are various approaches based on the environment or framework being used.
Mathematical Approach
When adjusting the center position of a rectangle, it is essential to understand its properties. A rectangle can be defined by its center point (Cx, Cy), width (W), and height (H). The corners of the rectangle can be calculated as follows:
- Top-left corner: (Cx – W/2, Cy – H/2)
- Top-right corner: (Cx + W/2, Cy – H/2)
- Bottom-left corner: (Cx – W/2, Cy + H/2)
- Bottom-right corner: (Cx + W/2, Cy + H/2)
To change the center position, simply update the coordinates of Cx and Cy.
Implementation in Programming Languages
Different programming environments have distinct methods for changing the center position of rectangles. Below are examples in popular frameworks.
HTML5 Canvas
In HTML5 Canvas, you can adjust the center of a rectangle using the `fillRect` method after translating the context:
“`javascript
function drawRectangle(ctx, centerX, centerY, width, height) {
ctx.save(); // Save the current state
ctx.translate(centerX, centerY); // Move the origin to the center
ctx.fillRect(-width / 2, -height / 2, width, height); // Draw the rectangle centered
ctx.restore(); // Restore the state
}
“`
CSS for Web Development
In CSS, centering a rectangle can be achieved using the `transform` property. Here’s how you can do this:
“`css
.rectangle {
width: 200px;
height: 100px;
background-color: blue;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%); /* Centering */
}
“`
Python with Pygame
In Pygame, the rectangle can be centered by adjusting its `topleft` attribute based on the desired center:
“`python
import pygame
def draw_rectangle(screen, center_x, center_y, width, height):
rect = pygame.Rect(0, 0, width, height)
rect.center = (center_x, center_y) Set the center
pygame.draw.rect(screen, (0, 128, 255), rect) Draw the rectangle
“`
Using Graphics Software
For software like Adobe Illustrator or Inkscape, you can center a rectangle through the following steps:
- Select the rectangle.
- Open the alignment panel.
- Choose the option to align to the artboard or parent object.
- Click the center alignment buttons for both horizontal and vertical alignment.
Common Pitfalls
When changing the center position of a rectangle, consider the following potential issues:
- Coordinate System: Be aware of the coordinate system in use (e.g., top-left origin vs. center origin).
- Aspect Ratio: Ensure that the width and height remain consistent if the rectangle needs to maintain its aspect ratio.
- Overlapping Objects: Be mindful of other graphical elements that may overlap with the rectangle after repositioning.
Example Scenarios
Scenario | Method Used | Code Snippet Example |
---|---|---|
Web Development | CSS | `.rectangle { transform: translate(-50%, -50%); }` |
Game Development | Pygame | `rect.center = (center_x, center_y)` |
HTML5 Canvas | JavaScript | `ctx.translate(centerX, centerY)` |
Graphic Design Software | Alignment Tools | Use alignment panel for centering |
By applying the appropriate techniques according to the specific context, changing the center position of a rectangle can be executed efficiently and effectively.
Adjusting Rectangle Center Positions: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Vector Dynamics Inc.). “To change the center position of a rectangle programmatically, one must first understand the coordinate system in use. By adjusting the x and y coordinates of the rectangle’s center, while recalculating the top-left corner based on the rectangle’s width and height, one can effectively reposition the rectangle without altering its dimensions.”
Michael Chen (UI/UX Designer, Creative Solutions Agency). “In user interface design, changing the center position of a rectangle is crucial for achieving balance and visual harmony. Utilizing CSS properties such as ‘transform’ and ‘translate’, designers can easily adjust the center position of elements, ensuring they align with other components on the page.”
Sarah Thompson (Computer Graphics Researcher, Advanced Visual Technologies). “From a computational graphics perspective, modifying the center position of a rectangle involves matrix transformations. By applying translation matrices, one can shift the rectangle’s center efficiently, which is essential for animations and dynamic rendering in real-time applications.”
Frequently Asked Questions (FAQs)
How can I change the center position of a rectangle in a graphics application?
To change the center position of a rectangle, you typically need to adjust its x and y coordinates based on the desired center point. This involves recalculating the rectangle’s top-left corner by subtracting half the width and height from the center coordinates.
What programming functions can be used to modify a rectangle’s center position?
Most graphics libraries provide functions to set the position of shapes. For example, in libraries like p5.js or Processing, you can use methods like `rectMode(CENTER)` to draw a rectangle based on its center rather than its top-left corner.
Does changing the center position affect the rectangle’s dimensions?
No, changing the center position does not affect the rectangle’s dimensions. The width and height remain constant; only the coordinates of the rectangle’s position are altered.
Are there any performance implications when changing the center position of rectangles frequently?
Frequent changes to the center position can lead to performance issues, especially in real-time applications. Minimizing redraws and optimizing the rendering process can help mitigate these impacts.
Can I animate the center position of a rectangle?
Yes, animating the center position of a rectangle is possible by incrementally adjusting its coordinates over time. This can be achieved using animation loops or frame updates in your graphics framework.
What is the difference between changing the center position and the position of the rectangle’s corner?
Changing the center position adjusts the rectangle based on its midpoint, while changing the corner position modifies the rectangle’s top-left corner. The center position is more intuitive for rotation and alignment tasks.
In graphical programming and design, the ability to change the center position of a rectangle (rect) is a fundamental operation that impacts the overall layout and alignment of elements within a visual composition. Adjusting the center position allows for greater control over the placement of shapes, enabling designers to create more visually appealing and balanced designs. This adjustment can be achieved through various methods, depending on the programming environment or graphic design software being utilized.
One key insight is that understanding the coordinate system of the canvas or workspace is crucial when altering the center position of a rectangle. Different environments may use different origin points and coordinate systems, which can affect how position adjustments are made. Familiarity with these systems ensures precise placement and alignment, ultimately enhancing the quality of the design.
Another important takeaway is the significance of transformations in graphic design. By employing transformation techniques such as translation, rotation, and scaling, designers can manipulate the center position of rectangles dynamically. This flexibility not only facilitates creative expression but also allows for responsive designs that adapt to various screen sizes and orientations.
changing the center position of a rectangle is a vital skill in graphic design and programming. Mastery of this concept not only improves the aesthetic quality of designs but also enhances functionality
Author Profile
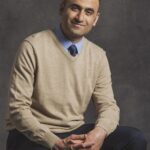
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?