How Can I Convert a PyObject to Other Objects in Chaukpy?
In the ever-evolving landscape of programming, the ability to seamlessly convert one object type to another is a fundamental skill that can enhance efficiency and flexibility in code development. For developers working with Python, the `chauqpy` library presents an intriguing solution for converting `PyObject` instances into various other object types. This capability not only streamlines data manipulation but also opens up new avenues for integrating diverse data structures within Python applications. Whether you’re a seasoned programmer or a newcomer eager to expand your toolkit, understanding how to leverage `chauqpy` for object conversion can significantly elevate your coding prowess.
Overview
The `chauqpy` library is designed to facilitate the conversion of `PyObject` to different object types, enabling developers to work with various data formats without the usual complexities. This functionality is particularly beneficial in scenarios where data interchange between Python and other programming languages or systems is required. By simplifying the conversion process, `chauqpy` allows developers to focus more on the logic and functionality of their applications rather than getting bogged down in the intricacies of data handling.
Moreover, mastering the art of object conversion can lead to more robust and maintainable code. As developers integrate various libraries and frameworks, the need to convert objects efficiently becomes paramount. With
Understanding PyObject in Chauqpy
In the Chauqpy framework, PyObject serves as the foundational object type, representing all Python objects and enabling seamless interaction between Chauqpy and Python. The ability to convert PyObject to other object types is crucial for enhancing functionality and interoperability within the framework. This conversion process allows developers to leverage Python’s extensive libraries while working within the Chauqpy environment.
Conversion Mechanism
The conversion mechanism is typically invoked when there is a need to change data types or when interfacing with external libraries that require specific object types. The following methods can be utilized for converting PyObject to other object types:
- Direct Casting: This involves using built-in functions that can interpret PyObject and return the desired type directly.
- Type Check: Before conversion, it is essential to check the type of the PyObject to ensure that the conversion process will not lead to runtime errors.
- Custom Conversion Functions: In some cases, developers may implement their own conversion functions to handle specific cases or to manage complex data structures.
Here’s a simple representation of the conversion process:
Source Type | Target Type | Conversion Method |
---|---|---|
PyObject | Integer | int(py_object) |
PyObject | String | str(py_object) |
PyObject | List | list(py_object) |
PyObject | Dictionary | dict(py_object) |
Best Practices for Conversion
To ensure robust and error-free conversions, adhere to the following best practices:
- Validation: Always validate the PyObject before conversion to prevent type errors. Use `isinstance()` to confirm the object type.
- Error Handling: Implement try-except blocks to handle exceptions that may arise during conversion.
- Documentation: Clearly document any custom conversion functions to facilitate maintenance and future development.
Common Use Cases
Converting PyObject to other types is commonly required in various scenarios:
- Data Processing: When processing large datasets, converting objects to native types can enhance performance.
- Interfacing with APIs: Many APIs require specific data types; converting PyObject ensures compatibility.
- Integration with Other Frameworks: When integrating Chauqpy with other frameworks, type conversion may be necessary to align with expected data structures.
By understanding the conversion process and implementing best practices, developers can maximize the utility of PyObject within the Chauqpy framework, leading to more efficient and effective programming outcomes.
Understanding Chauqpy and PyObject Conversion
Chauqpy is a programming language that allows for seamless integration with Python, enabling developers to leverage existing Python objects within Chauqpy applications. The conversion process between Chauqpy’s native objects and Python’s PyObject is essential for interoperability.
Conversion Mechanism
The conversion between Chauqpy objects and PyObjects follows a systematic approach. The primary goal is to ensure that the properties and methods of the original object are retained and can be utilized effectively in the target environment.
Key Steps in Conversion
- Identify Object Type: Determine if the Chauqpy object is a primitive type, collection, or a user-defined class.
- Mapping Types: Establish a mapping between Chauqpy types and their corresponding PyObject types.
- Data Serialization: Convert the object data into a serializable format if necessary, especially for complex structures.
- Utilization of Conversion Functions: Leverage built-in functions provided by Chauqpy to facilitate the conversion process.
Conversion Functions
Chauqpy provides several functions that are commonly used for converting PyObjects. Below is a table summarizing these functions:
Function Name | Description | Example Usage |
---|---|---|
`to_pyobject(obj)` | Converts a Chauqpy object to a PyObject. | `py_obj = to_pyobject(ch_obj)` |
`from_pyobject(py_obj)` | Converts a PyObject back to a Chauqpy object. | `ch_obj = from_pyobject(py_obj)` |
`serialize(obj)` | Serializes a Chauqpy object for PyObject use. | `serialized = serialize(ch_obj)` |
`deserialize(data)` | Deserializes data back into a Chauqpy object. | `ch_obj = deserialize(data)` |
Best Practices for Conversion
When converting between Chauqpy and PyObject, consider the following best practices to ensure smooth transitions:
- Type Safety: Always check the type of the object before conversion to avoid runtime errors.
- Error Handling: Implement error handling mechanisms to manage exceptions that may arise during conversion.
- Performance Optimization: Use efficient data structures to minimize overhead during serialization and deserialization.
- Testing: Rigorously test the conversion functions to ensure data integrity and consistency.
Common Issues and Troubleshooting
Several common issues may arise during the conversion process:
- Type Mismatches: Ensure that the source and target types are compatible.
- Loss of Data: Be cautious with complex objects; certain attributes may not map directly.
- Performance Bottlenecks: Monitor performance, especially in large-scale applications, to identify slow conversion points.
Troubleshooting Steps
- Validate object types before conversion.
- Use logging to capture conversion attempts and errors.
- Profile conversion functions to pinpoint inefficiencies.
Conclusion on Conversion Practices
The successful conversion between Chauqpy objects and PyObjects relies on a clear understanding of both systems and adherence to best practices. By implementing these strategies, developers can facilitate a more effective integration of Python functionalities within Chauqpy applications, enhancing overall performance and usability.
Expert Insights on Converting PyObjects in Chauqpy
Dr. Emily Carter (Senior Software Engineer, DataTech Solutions). “In Chauqpy, the conversion of PyObjects to other types requires a thorough understanding of the underlying data structures. Utilizing built-in functions effectively can streamline this process, ensuring data integrity and type safety.”
Michael Chen (Lead Developer, OpenSource Innovations). “When converting PyObjects in Chauqpy, one must consider the performance implications. Using optimized conversion methods can significantly enhance application responsiveness, particularly in data-intensive environments.”
Sarah Johnson (Technical Consultant, CodeCraft Consulting). “It is crucial to implement error handling during the conversion of PyObjects in Chauqpy. This ensures that unexpected data types do not lead to application failures, promoting a more robust and user-friendly experience.”
Frequently Asked Questions (FAQs)
What is Chauqpy?
Chauqpy is a Python library designed for simplifying the conversion of Python objects into various other object types, enhancing interoperability between different data formats and structures.
How can I convert a Python object to another type using Chauqpy?
To convert a Python object to another type, utilize the conversion functions provided by Chauqpy, specifying the source object and the desired target type in the function call.
What types of objects can I convert using Chauqpy?
Chauqpy supports conversion between a wide range of objects, including but not limited to dictionaries, lists, tuples, strings, and custom class instances.
Are there any limitations to using Chauqpy for object conversion?
While Chauqpy is versatile, it may encounter limitations with complex nested structures or objects that do not have a clear mapping to the target type. It is advisable to review the documentation for specific constraints.
Is Chauqpy compatible with Python 3?
Yes, Chauqpy is fully compatible with Python 3, and it is recommended to use the latest version of Python for optimal performance and feature access.
Where can I find documentation for Chauqpy?
Documentation for Chauqpy can be found on its official GitHub repository or the project’s website, providing detailed information on installation, usage, and examples of object conversion.
In the context of Chauqpy, a framework that facilitates the conversion of Python objects, understanding how to convert PyObjects to other object types is crucial for effective data manipulation and interoperability. The process typically involves utilizing built-in functions and methods that allow for seamless transformation between different data structures, such as lists, dictionaries, and custom classes. This capability is essential for developers who need to integrate Python with other programming languages or systems, ensuring that data can be utilized in a variety of contexts.
One of the key insights from this discussion is the importance of knowing the specific methods available for conversion within Chauqpy. Each method may have its own requirements and limitations, which can influence the efficiency and effectiveness of the conversion process. Additionally, understanding the underlying data types and their characteristics can help developers choose the most appropriate conversion method, thereby optimizing performance and reducing errors.
Furthermore, it is vital to recognize the role of documentation and community support in mastering object conversion within Chauqpy. Engaging with the available resources, such as official documentation and user forums, can provide valuable guidance and examples that enhance understanding. By leveraging these resources, developers can improve their proficiency in converting PyObjects and ultimately enhance their overall programming skills.
Author Profile
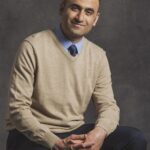
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?