Why Is My Click Event Not Firing in JavaScript?
In the dynamic world of web development, interactivity is key to creating engaging user experiences. JavaScript, the backbone of client-side scripting, plays a crucial role in enabling this interactivity, particularly through events like clicks. However, developers often encounter a perplexing issue: the click event not firing as expected. This seemingly minor hiccup can lead to significant frustration, derailing the user experience and complicating the development process. Understanding the reasons behind this issue is essential for any developer looking to create seamless and responsive web applications.
When a click event fails to trigger, it can stem from a variety of factors, ranging from simple coding errors to more complex issues related to event delegation or browser compatibility. Developers may find themselves grappling with problems such as elements being obscured by other layers, event listeners not being properly attached, or even conflicts with other scripts. Each of these scenarios requires a different approach to diagnose and resolve, making it imperative for developers to have a comprehensive understanding of how click events function within the broader context of JavaScript.
As we delve deeper into the intricacies of click events in JavaScript, we will explore common pitfalls, troubleshooting techniques, and best practices to ensure that your click events fire reliably. Whether you’re a seasoned developer or just starting out,
Common Reasons for Click Events Not Firing
Several factors can contribute to click events not firing in JavaScript. Identifying the root cause is essential for troubleshooting effectively. Below are some common reasons:
- Event Delegation Issues: If the event is bound to a parent element that is dynamically modified or not present at the time of binding, it may not work as expected.
- Incorrect Selector: The selector used to bind the click event may not match any elements on the page, leading to a failure in event registration.
- Preventing Default Behavior: If the default behavior of a click event is prevented (e.g., using `event.preventDefault()`), it might affect how subsequent events are triggered.
- JavaScript Errors: Any existing JavaScript errors can halt script execution, preventing click events from firing.
- CSS Pointer Events: If an element has CSS properties set to `pointer-events: none;`, it will not respond to click events.
Debugging Click Events
To diagnose why a click event is not firing, several debugging strategies can be employed:
- Console Logging: Insert `console.log()` statements within the click event handler to ensure it is being triggered.
- Inspect Element: Use browser developer tools to inspect the element and verify event bindings.
- Check Event Listeners: Use `getEventListeners()` in the console to see if the click event is correctly attached to the element.
Here’s a simple example to illustrate a click event binding:
“`javascript
document.querySelector(‘myButton’).addEventListener(‘click’, function() {
console.log(‘Button was clicked!’);
});
“`
To check if the event is properly attached, you can run:
“`javascript
console.log(getEventListeners(document.querySelector(‘myButton’)));
“`
Best Practices for Event Handling
Implementing best practices can help avoid issues with click events. Consider the following guidelines:
- Use Event Delegation: Bind events to a parent container instead of individual child elements, especially for dynamic content.
- Ensure Proper Timing: Use `DOMContentLoaded` or similar methods to ensure elements are available before binding events.
- Use `addEventListener`: This method is preferred over inline event handlers as it allows multiple handlers for the same event type.
Common Solutions
Here are some solutions to fix click event issues:
Issue | Solution |
---|---|
Event not firing | Check if the selector matches the correct element. |
Event attached but not triggered | Ensure the element is not covered by another element or has `pointer-events: none;` applied. |
Event handlers conflicting | Remove or adjust conflicting event handlers. |
By applying these solutions and best practices, developers can enhance the reliability of click events in their applications.
Common Causes of Click Event Issues
The failure of click events to fire in JavaScript can stem from various issues. Understanding these common pitfalls can help in diagnosing and resolving the problem efficiently.
- Event Listener Not Attached Properly: Ensure that the event listener is added to the correct element. If the listener is added before the element is rendered in the DOM, it will not work.
- Incorrect Selector Usage: Verify that the selector used to bind the event listener matches the intended element. Using the wrong selector can lead to no event being attached.
- Preventing Default Behavior: Sometimes, other event handlers might be calling `event.preventDefault()`, which can stop the click event from propagating.
- Event Delegation Misconfiguration: If using event delegation, ensure that the event listener is attached to a parent element that exists in the DOM at the time of binding.
- CSS Issues: Elements that are hidden using CSS (e.g., `display: none`) or covered by other elements (z-index issues) cannot be clicked.
Debugging Click Event Issues
When troubleshooting click events, a systematic approach is essential. Here are steps to identify the problem:
- Use Console Logs: Insert `console.log` statements before and after the event listener to check if the code is executing.
- Inspect Element: Utilize browser developer tools to inspect the element and verify if the listener is attached correctly.
- Check Event Listeners: In the developer tools, check the list of event listeners for the element to ensure the click event is registered.
- Test in Isolation: Create a simplified version of the code to isolate the issue, stripping away other complexities.
- Review JavaScript Errors: Check the console for any JavaScript errors that might be preventing subsequent scripts from running.
Example Code Snippets
Here are a few examples illustrating common mistakes and their fixes:
Incorrect Selector Example:
“`javascript
// Incorrect
document.querySelector(‘.button’).addEventListener(‘click’, function() {
console.log(‘Button clicked!’);
});
// Fix: Ensure the selector matches the actual element
document.querySelector(‘myButton’).addEventListener(‘click’, function() {
console.log(‘Button clicked!’);
});
“`
Event Delegation Example:
“`javascript
// Correct Event Delegation
document.querySelector(‘parent’).addEventListener(‘click’, function(event) {
if (event.target.matches(‘.child’)) {
console.log(‘Child clicked!’);
}
});
“`
Tools for Testing Click Events
Several tools and techniques can help to test and debug click events effectively:
Tool/Technique | Description |
---|---|
Browser Developer Tools | Inspect elements, view console logs, and monitor network requests. |
JSFiddle/CodePen | Create and share live examples to isolate issues in a controlled environment. |
Unit Testing Frameworks | Use frameworks like Jest or Mocha to automate tests for click events. |
Best Practices for Handling Click Events
To ensure robust click event handling, consider the following best practices:
- Use Event Delegation Wisely: Attach event listeners to parent elements when dealing with dynamic content.
- Minimize Global Variables: Keep your code modular to avoid conflicts with other scripts.
- Detach Listeners When Necessary: Remove event listeners when they are no longer needed to prevent memory leaks.
- Test Across Browsers: Ensure compatibility by testing click events in multiple browsers and devices.
Understanding Why Click Events May Not Fire in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovators Inc.). “One common reason for click events not firing in JavaScript is the improper binding of event listeners. Developers should ensure that the event listener is attached to the correct element and that the element is present in the DOM at the time of binding.”
Mark Thompson (Lead Front-End Engineer, CodeCraft Solutions). “Another frequent issue arises from event propagation. If a parent element has a click event handler that calls `event.stopPropagation()`, it can prevent child elements from firing their own click events. Understanding the event flow is crucial for debugging these scenarios.”
Lisa Nguyen (UX/UI Specialist, Digital Design Agency). “Sometimes, click events may not fire due to CSS issues, such as overlapping elements or z-index problems. It is essential to inspect the layout in the browser’s developer tools to ensure that the clickable element is not obscured by other elements.”
Frequently Asked Questions (FAQs)
What are common reasons for a click event not firing in JavaScript?
A click event may not fire due to several reasons, including incorrect event listener attachment, the element being covered by another element, or the element being disabled. Additionally, if JavaScript errors occur before the event listener is executed, the event may not trigger.
How can I check if my event listener is correctly attached?
You can verify if your event listener is attached by using browser developer tools. Place a `console.log` statement within the event listener function to see if it logs when you interact with the element. Alternatively, you can use the `getEventListeners()` method in the console to inspect attached events.
What should I do if my click event is being overridden by another event?
If another event is overriding your click event, ensure that your event listener’s priority is set correctly. You can use `stopPropagation()` in your event handler to prevent the event from bubbling up and triggering other listeners. Additionally, check for any conflicting event listeners on parent elements.
How can I ensure that my click event fires on dynamically created elements?
For dynamically created elements, use event delegation by attaching the event listener to a parent element that exists when the page loads. This way, the event listener can capture events from child elements even if they are added later.
What debugging techniques can I use to troubleshoot click event issues?
Utilize browser developer tools to inspect elements and view console logs. Check for JavaScript errors in the console, and use breakpoints to step through the code execution. Additionally, ensure that the element is not hidden or disabled, which would prevent the click event from firing.
Can CSS styles affect the firing of click events in JavaScript?
Yes, CSS styles can affect click events. If an element has a `pointer-events: none;` style, it will not respond to click events. Similarly, if an element is positioned off-screen or hidden using `display: none;`, it will also not trigger click events.
the issue of a click event not firing in JavaScript can stem from various factors, including incorrect event listener attachment, issues with event propagation, or the presence of overlapping elements that may intercept the click. It is essential to ensure that the event listener is correctly bound to the intended element and that the JavaScript code is executed after the DOM has fully loaded. Additionally, understanding the concept of event delegation can help manage click events more efficiently, especially in dynamic applications.
Another critical aspect to consider is the role of JavaScript frameworks and libraries, which can sometimes alter the default behavior of events. Developers should be aware of how these tools manage events and ensure compatibility with their custom scripts. Debugging tools, such as browser developer consoles, can provide valuable insights into whether the event listeners are firing as expected and help identify any potential errors in the code.
Ultimately, addressing the problem of click events not firing requires a systematic approach to troubleshooting. By verifying the event listener setup, checking for conflicts with other scripts or styles, and utilizing debugging techniques, developers can effectively resolve these issues. This understanding not only enhances the functionality of web applications but also contributes to a smoother user experience.
Author Profile
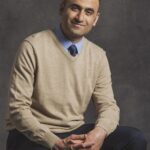
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?