How Can Code Snippets Help You Hide the Header on Scroll?
In the fast-paced digital landscape, user experience is paramount. As websites evolve, so do the techniques to enhance navigation and usability. One such technique that has gained traction among developers and designers alike is the ability to hide headers on scroll. This dynamic feature not only maximizes screen real estate but also creates a more immersive browsing experience. If you’ve ever wondered how to implement this functionality seamlessly, you’re in the right place. In this article, we’ll explore the concept of hiding headers on scroll, providing you with practical code snippets and insights to elevate your web projects.
Overview
Hiding headers on scroll is a popular design choice that allows users to focus on content without the distraction of a persistent navigation bar. By making the header disappear as users scroll down the page, and reappear when they scroll up, websites can create a fluid and engaging experience. This technique is particularly beneficial for mobile users, where screen space is limited and every pixel counts.
Implementing this feature involves a combination of CSS for styling and JavaScript (or jQuery) for functionality. The basic premise revolves around detecting the user’s scroll direction and adjusting the header’s visibility accordingly. With a few lines of code, you can transform your website’s header behavior, making it more intuitive and user-friendly.
Implementing Header Hide on Scroll
To achieve a dynamic header that hides on scroll, we can utilize JavaScript or jQuery along with CSS for styling. This technique enhances user experience by maximizing screen space when users scroll down a webpage. Here’s a step-by-step approach to implement this feature.
CSS for Header Style
Before implementing the JavaScript functionality, it is essential to define the initial styles for the header. The following CSS code snippet sets the basic look and feel of the header:
“`css
.header {
position: fixed;
top: 0;
width: 100%;
background-color: 333;
color: white;
padding: 10px 0;
transition: top 0.3s;
z-index: 1000;
}
“`
This CSS ensures that the header remains fixed at the top of the viewport and includes a smooth transition effect when it hides and shows.
JavaScript for Scroll Detection
Next, we need to implement JavaScript to detect the scroll event and toggle the visibility of the header. Below is a simple code snippet that can be used:
“`javascript
let lastScrollTop = 0;
const header = document.querySelector(‘.header’);
window.addEventListener(‘scroll’, function() {
let scrollTop = window.pageYOffset || document.documentElement.scrollTop;
if (scrollTop > lastScrollTop) {
// Scrolling down
header.style.top = “-60px”; // Hide header
} else {
// Scrolling up
header.style.top = “0”; // Show header
}
lastScrollTop = scrollTop;
});
“`
In this code, we listen for the scroll event on the window. Depending on whether the user scrolls up or down, the header’s position is adjusted accordingly.
jQuery Alternative
For those who prefer jQuery, the implementation becomes even more concise. Here’s how you could achieve the same functionality:
“`javascript
$(document).ready(function() {
let lastScrollTop = 0;
const header = $(‘.header’);
$(window).scroll(function() {
let scrollTop = $(this).scrollTop();
if (scrollTop > lastScrollTop) {
header.css(‘top’, ‘-60px’); // Hide header
} else {
header.css(‘top’, ‘0’); // Show header
}
lastScrollTop = scrollTop;
});
});
“`
This jQuery version accomplishes the same as the vanilla JavaScript example, providing a more succinct syntax for those familiar with the library.
Performance Considerations
While implementing a header that hides on scroll can enhance user experience, it is essential to consider performance implications. Here are some best practices:
- Debouncing the Scroll Event: To minimize performance overhead, consider debouncing the scroll event to limit how often your function runs.
- Use CSS Transitions: Rely on CSS transitions for smoother animations, as they are often less resource-intensive than JavaScript animations.
- Test Across Browsers: Ensure that the functionality works consistently across different browsers and devices.
Technique | Pros | Cons |
---|---|---|
JavaScript | More control over functionality | More code to manage |
jQuery | Simpler syntax | Requires jQuery library |
This table summarizes the two primary techniques for hiding a header on scroll, outlining their advantages and disadvantages, helping developers choose the best approach for their project.
Implementing Header Hide on Scroll
To achieve a dynamic header that hides upon scrolling down and reappears when scrolling up, a combination of HTML, CSS, and JavaScript is required. Below is a structured guide to implement this feature effectively.
HTML Structure
The HTML structure is simple and consists of a header and a content area. Here is a basic example:
“`html
“`
CSS Styling
The CSS will ensure the header is styled appropriately and positioned correctly. Below is a sample CSS code snippet:
“`css
body {
margin: 0;
font-family: Arial, sans-serif;
}
header {
position: fixed;
top: 0;
width: 100%;
height: 60px;
background-color: 333;
color: fff;
display: flex;
align-items: center;
justify-content: center;
transition: top 0.3s;
z-index: 1000;
}
.content {
margin-top: 70px; /* To avoid content being hidden behind the header */
padding: 20px;
height: 2000px; /* For demonstration purposes */
background: linear-gradient(to bottom, f0f0f0, e0e0e0);
}
“`
JavaScript Functionality
The JavaScript code will handle the scroll events and toggle the header’s visibility accordingly. Below is an example of how to implement this:
“`javascript
let lastScrollTop = 0;
const header = document.getElementById(“header”);
window.addEventListener(“scroll”, function() {
const currentScroll = window.pageYOffset || document.documentElement.scrollTop;
if (currentScroll > lastScrollTop) {
// Scroll Down
header.style.top = “-60px”; // Hide header
} else {
// Scroll Up
header.style.top = “0”; // Show header
}
lastScrollTop = currentScroll <= 0 ? 0 : currentScroll; // For Mobile or negative scrolling
});
```
Considerations for Mobile Devices
When implementing this feature, it is essential to consider mobile user experience. Here are some recommendations:
- Touch Sensitivity: Adjust the threshold for hiding and showing the header to account for touch gestures.
- Performance Optimization: Use `requestAnimationFrame` for better performance during scroll events.
- Accessibility: Ensure that the header remains accessible for screen readers.
Testing and Debugging
After implementing the code, thorough testing is necessary:
- Test on different browsers to ensure compatibility.
- Check responsiveness on various screen sizes.
- Monitor performance to avoid jank during scrolling.
By following the above guidelines and code snippets, developers can effectively implement a header that hides on scroll, enhancing the overall user experience on their web applications.
Expert Insights on Hiding Headers on Scroll in Web Development
Emily Chen (Front-End Developer, CodeCraft Solutions). “Implementing code snippets to hide headers on scroll can significantly enhance user experience by maximizing screen real estate. Utilizing CSS properties such as ‘position: sticky’ in conjunction with JavaScript for scroll detection can create a seamless interaction.”
Michael Thompson (UI/UX Designer, Digital Innovations). “The technique of hiding headers on scroll not only declutters the interface but also encourages users to focus on content. It is essential to ensure that the transition is smooth to avoid disrupting the user’s engagement.”
Sarah Patel (Web Performance Analyst, SpeedyWeb Analytics). “From a performance standpoint, using efficient code snippets to manage header visibility during scrolling can reduce layout shifts and improve overall site responsiveness. This is crucial for maintaining high user satisfaction and SEO rankings.”
Frequently Asked Questions (FAQs)
What is the purpose of hiding a header on scroll?
Hiding a header on scroll enhances user experience by maximizing screen space for content while maintaining easy access to navigation when needed.
How can I implement a header that hides on scroll using JavaScript?
You can achieve this by adding an event listener for the scroll event, checking the scroll position, and toggling the visibility of the header based on the direction of the scroll.
Are there any CSS-only solutions for hiding a header on scroll?
Yes, you can use CSS transitions combined with the `position: sticky;` property, but JavaScript is typically required for more complex behaviors like detecting scroll direction.
What libraries can assist in creating a hide-on-scroll header?
Libraries such as jQuery or frameworks like React and Vue.js offer plugins and components that simplify the implementation of hide-on-scroll functionality.
Is it possible to customize the hide behavior of the header?
Yes, you can customize the hide behavior by adjusting the scroll threshold, animation speed, and visibility triggers based on user interactions or specific scroll positions.
What are common pitfalls to avoid when implementing a hide-on-scroll header?
Common pitfalls include not accounting for varying screen sizes, failing to optimize for performance, and neglecting accessibility considerations for users relying on keyboard navigation.
implementing code snippets to hide a header on scroll is a widely used technique in web development that enhances user experience. This feature allows for more screen space to be utilized for content as users scroll down a page, making it particularly beneficial for mobile devices and content-heavy websites. Various methods can be employed, including CSS properties and JavaScript event listeners, to achieve this effect effectively.
Key takeaways from the discussion include the importance of user experience in web design, as hiding the header can minimize distractions and allow users to focus on the content. Additionally, developers should consider performance implications when implementing such features, ensuring that the solution is optimized for different devices and browsers. The choice between CSS and JavaScript approaches often depends on the specific requirements of the project and the desired level of customization.
Furthermore, it is essential to test the implementation across various scenarios to ensure that the header behaves as expected under different conditions. This includes responsiveness to different scroll speeds and ensuring that the header reappears smoothly when scrolling back up. Overall, mastering the technique of hiding headers on scroll can contribute significantly to creating a more engaging and user-friendly web experience.
Author Profile
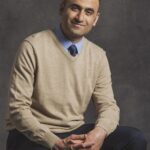
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?