Why Am I Seeing ‘commands community publish.js: Cannot Find Module’? Troubleshooting Tips!
In the fast-paced world of software development, encountering errors can often feel like an inevitable rite of passage. One such frustrating hurdle is the dreaded “cannot find module” error, particularly when working with JavaScript files like `publish.js`. This issue can halt your progress, leaving you scratching your head and questioning your setup. Whether you’re a seasoned developer or just starting your coding journey, understanding the nuances of module resolution in JavaScript is crucial for maintaining a smooth workflow.
In this article, we will delve into the common causes and solutions for the “cannot find module” error, specifically in the context of `publish.js`. We’ll explore how module paths, dependencies, and project structure can impact your ability to successfully run your commands. By the end, you’ll not only grasp the underlying reasons for this error but also gain practical strategies to troubleshoot and resolve it, empowering you to tackle similar issues with confidence in the future.
Join us as we navigate the intricacies of JavaScript module management, demystifying the error messages that can often seem cryptic. Whether you’re working on a personal project or contributing to a larger community, understanding how to effectively manage your modules will enhance your development experience and improve your coding skills.
Understanding the Error: Cannot Find Module
When you encounter the error message `commands community publish.js: cannot find module`, it usually indicates that the Node.js runtime is unable to locate the specified JavaScript file or module. This situation can arise from various causes, and understanding these can help you resolve the issue effectively.
The following are common reasons for this error:
- Incorrect File Path: The path specified in your command may not correctly point to the desired file. Double-check the relative or absolute path to ensure accuracy.
- Module Not Installed: If you are trying to import a module that hasn’t been installed in your project, you will need to add it via npm or yarn.
- Typographical Errors: Simple typos in the filename or path can lead to this error. Verify that the spelling and casing of the file name match exactly.
- File Permissions: Insufficient permissions on the file or directory can prevent the module from being accessed. Ensure that your user has the necessary permissions.
- Node Modules Not Found: If your script relies on other local modules that are missing, you will need to install them as well.
Troubleshooting Steps
To resolve the `cannot find module` error, consider following these troubleshooting steps:
- Check the File Path:
- Confirm that the file exists at the specified location.
- Use commands such as `ls` or `dir` to list the directory contents and verify.
- Install Missing Modules:
- If a third-party module is missing, run:
“`bash
npm install
“`
- For globally installed modules, ensure they are accessible in your PATH.
- Verify Typographical Accuracy:
- Review your import statements and file references for any mistakes.
- Adjust Permissions:
- On Unix-based systems, you can change file permissions using:
“`bash
chmod 644 publish.js
“`
- Reinstall Node Modules:
- If issues persist, you can clear and reinstall your node modules:
“`bash
rm -rf node_modules
npm install
“`
Example of a Correct File Structure
To prevent such errors, it’s helpful to maintain a clear project structure. Below is an example of a well-organized Node.js project:
Directory/File | Description |
---|---|
/src | Source files, including scripts and modules. |
/node_modules | Installed npm packages. |
package.json | Project metadata and dependencies. |
publish.js | The main script for publication commands. |
By adhering to a logical directory structure and following best practices in file naming, you can significantly reduce the chances of encountering module-related errors.
Troubleshooting the “Cannot Find Module” Error
When encountering the error message `commands community publish.js: cannot find module`, it’s essential to perform a systematic troubleshooting process. This error typically indicates that Node.js cannot locate a specified module required by your script. Below are steps and considerations to address this issue effectively.
Check Module Installation
Ensure that the module you are trying to use is installed correctly. You can verify this by:
- Checking the `node_modules` directory for the module.
- Running the following command in your project directory:
“`bash
npm list
“`
- If the module is missing, install it using:
“`bash
npm install
“`
Verify File Paths
Incorrect file paths can lead to module not found errors. Confirm the following:
- Check that the path specified in your `require` or `import` statement is correct.
- Ensure that file names and paths are case-sensitive, especially on UNIX-based systems.
Update Node.js and NPM
Sometimes, outdated versions of Node.js or npm may cause compatibility issues. To update, you can use:
“`bash
npm install -g npm
“`
For Node.js, visit the official [Node.js website](https://nodejs.org/) to download the latest version.
Review Package.json Configuration
Examine your `package.json` file for any discrepancies. Key aspects to review include:
- Ensure that all dependencies are listed correctly.
- Run `npm install` to install missing modules as per the `package.json`.
Check Global vs. Local Installation
Modules can be installed globally or locally. If you require a module globally, ensure it is installed as such:
“`bash
npm install -g
“`
Conversely, if the script is looking for a local module, confirm that it exists in the project directory.
Utilize Node.js Resolution Mechanism
Node.js resolves modules in a specific order. Understanding this resolution can aid in troubleshooting:
- Built-in Modules: These are part of Node.js and do not need installation.
- Local Modules: Look in the current directory and in the `node_modules` folder.
- Global Modules: Search in the global `node_modules` directory if installed globally.
Debugging with Verbose Output
To gain more insight into what is happening during module resolution, you can run your script with verbose logging. This can be achieved by modifying your command as follows:
“`bash
node –trace-warnings
“`
This option will provide additional context on where the issue might be occurring.
Common Issues and Solutions
Issue | Solution |
---|---|
Module not installed | Install the module using `npm install`. |
Incorrect file path | Verify and correct the path in your `require` statement. |
Version incompatibility | Update Node.js and npm to the latest versions. |
Dependency conflicts | Check for and resolve version conflicts in `package.json`. |
Misconfigured project structure | Ensure the module is located in the expected directories. |
By following these steps and employing a methodical approach, you should be able to resolve the `commands community publish.js: cannot find module` error effectively.
Resolving the ‘Cannot Find Module’ Error in JavaScript Projects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘cannot find module’ error typically arises from incorrect file paths or missing dependencies. It is essential to verify that the module you are trying to import is correctly installed and that your import statements accurately reflect the directory structure of your project.”
Michael Chen (JavaScript Framework Specialist, CodeMaster Academy). “When encountering this error in a Node.js environment, I recommend checking your package.json file to ensure all necessary modules are listed under dependencies. Additionally, running ‘npm install’ can often resolve issues related to missing packages.”
Sarah Thompson (Full-Stack Developer, Dev Solutions Group). “This error can also occur due to issues with module resolution settings in your project configuration. Make sure that your build tools, such as Webpack or Babel, are properly configured to recognize the paths to your modules, as misconfigurations can lead to these errors.”
Frequently Asked Questions (FAQs)
What does the error “commands community publish.js: cannot find module” indicate?
This error indicates that the Node.js runtime cannot locate the specified module, in this case, `publish.js`, which is necessary for executing the desired command.
How can I resolve the “cannot find module” error?
To resolve this error, ensure that the module is correctly installed in your project. You can check your `node_modules` directory or reinstall the module using npm or yarn.
What should I check if the module is installed but still shows the error?
Verify that the path to the module is correct in your code. Additionally, check for any typos in the module name and ensure that the module is compatible with your current Node.js version.
Can this error occur due to incorrect file structure?
Yes, if the file structure does not align with the expected paths in your code, it can lead to this error. Ensure that the `publish.js` file is located in the correct directory as referenced in your import or require statement.
Is there a way to debug this issue further?
You can use console logging or debugging tools to trace the execution flow and identify where the module is being called. Additionally, using `npm ls` can help you check for any missing dependencies.
What are some common reasons for a module not being found?
Common reasons include the module not being installed, incorrect file paths, typos in the module name, or issues with the package manager not resolving dependencies correctly.
The error message “commands community publish.js: cannot find module” typically indicates that the Node.js runtime is unable to locate the specified module required for executing a script. This issue often arises due to various reasons, such as incorrect file paths, missing dependencies, or improperly configured project structures. Understanding the underlying causes of this error is essential for developers to effectively troubleshoot and resolve the problem.
One of the primary insights from this discussion is the importance of verifying the file path and ensuring that the module is correctly installed. Developers should check the directory structure of their project and confirm that the module exists in the specified location. Additionally, utilizing package managers like npm or yarn can help in managing dependencies and ensuring that all necessary modules are installed correctly.
Another key takeaway is the value of consulting documentation and community forums when encountering such errors. The developer community often shares solutions and best practices that can expedite the debugging process. Engaging with these resources can provide insights into common pitfalls and effective strategies for module management in Node.js projects.
Author Profile
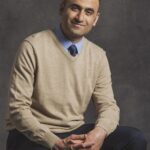
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?