Does the Comparison Method Really Violate Its General Contract?
In the world of programming, particularly in Java, the concept of comparison methods is pivotal to maintaining order and consistency within data structures. However, a seemingly innocuous oversight in the implementation of these methods can lead to significant issues, most notably when a comparison method violates its general contract. This violation can result in unexpected behavior in sorting algorithms, data retrieval processes, and even the integrity of collections. Understanding the implications of this violation is essential for developers who aim to write robust and reliable code.
At its core, the general contract for comparison methods dictates that they must adhere to specific rules to ensure consistent and predictable outcomes. These rules encompass reflexivity, antisymmetry, and transitivity, among others, which together create a framework that allows data structures to function correctly. When a comparison method strays from these principles, it can cause chaos in how objects are compared and sorted, leading to potential errors and inefficiencies in applications.
Moreover, the ramifications of violating this contract extend beyond mere technical glitches; they can also impact user experience and application performance. Developers must be vigilant in crafting their comparison methods, as overlooking these fundamental principles can result in a cascade of issues, from incorrect sorting to failure in data retrieval. As we delve deeper into this topic, we will explore the nuances of comparison
Understanding the General Contract of Comparison Methods
The general contract of comparison methods, particularly in programming and algorithms, refers to the expectations regarding how objects are compared to one another. This is crucial for data structures like trees, heaps, or sorting algorithms, where the behavior of comparisons dictates the structure and performance of these systems.
When implementing comparison methods, developers must ensure that they adhere to specific properties:
- Antisymmetry: If one object is considered less than another, then the reverse cannot be true.
- Transitivity: If object A is less than object B, and object B is less than object C, then A must be less than C.
- Consistency: The comparison should yield the same result each time it is called on the same pair of objects.
A comparison method that violates these principles can lead to unpredictable behavior in data structures and algorithms, resulting in errors or inefficient processing.
Examples of Violations
Violations of the general contract can manifest in various ways. Some examples include:
- Incorrect Implementation: If a comparison method is designed to compare two objects but mistakenly introduces conditions that contradict antisymmetry or transitivity.
- Mutable Objects: When objects being compared can change state during the comparison process, leading to inconsistent results.
Here is a table summarizing common violations and their implications:
Type of Violation | Description | Potential Impact |
---|---|---|
Antisymmetry Violation | Returns true for both A < B and B < A | Data structures may become corrupted; infinite loops may occur. |
Transitivity Violation | Returns inconsistent results such that A < B, B < C but A >= C | Sorting algorithms may fail to sort correctly. |
Mutable State | Objects change during comparison, altering outcomes | Unexpected behavior in collections; data integrity issues. |
Consequences of Violating the General Contract
The consequences of violating the general contract can be severe. Inconsistent comparisons lead to:
- Incorrect Sorting: Algorithms like QuickSort or MergeSort rely on consistent comparison methods. Inconsistent results can lead to an incorrectly sorted dataset.
- Data Structure Corruption: Data structures like priority queues or binary search trees may become corrupted, leading to errors in retrieval or insertion.
- Performance Issues: Algorithms may perform poorly or inefficiently if they cannot rely on the expected behavior of comparison methods.
By ensuring that comparison methods adhere to their general contract, developers can avoid these pitfalls and create robust, reliable software systems.
Understanding the General Contract of Comparison Methods
The general contract for comparison methods, particularly in programming and algorithms, refers to the rules that govern how objects are compared. Violating this contract can lead to unpredictable behavior in data structures such as sorted collections and can cause issues like incorrect sorting or retrieval of elements.
Key Principles of the General Contract
The general contract stipulates three main principles that must be adhered to:
- Antisymmetry: If one object is considered less than another, the reverse must also hold true.
- Transitivity: If object A is less than object B, and object B is less than object C, then object A must be less than object C.
- Consistency: The comparison results must remain consistent across multiple invocations, given that the objects are not modified.
Common Violations of the Comparison Method Contract
Violations can occur in various scenarios, leading to significant issues. Here are some common types of violations:
- Inconsistent Comparisons: An object is compared differently in different contexts or calls, leading to unpredictable results. For example, if two objects are equal in one comparison but not in another.
- Breaking Transitivity: If A < B and B < C, but A is not less than C, the transitive property is violated.
- Null Handling Issues: Improper handling of null values can lead to exceptions or inconsistent comparisons, particularly in languages that do not allow null comparisons.
Consequences of Violating the Contract
The repercussions of not adhering to the general contract are far-reaching:
Consequence | Description |
---|---|
Incorrect Sorting | Data structures that rely on sorting algorithms may not function correctly, leading to an unsorted collection. |
Unexpected Behavior | Applications may exhibit erratic behavior when relying on the results of comparisons for logic, such as searching or filtering. |
Inefficiency | Algorithms that depend on comparison can become inefficient, leading to increased resource consumption and slower performance. |
Data Integrity Risks | The integrity of data may be compromised, especially if comparisons are used in critical processes like transactions or data validation. |
Best Practices for Implementing Comparison Methods
To ensure compliance with the general contract, consider the following best practices:
- Consistent Logic: Develop comparison logic that is consistently applied across all instances.
- Unit Testing: Implement thorough unit tests to validate comparison methods against the contract principles. Tests should cover edge cases, including null values and extreme input scenarios.
- Documentation: Clearly document the intended behavior of comparison methods, including any assumptions about the input data.
- Use Built-in Methods: When available, utilize language-provided comparison methods that are designed to comply with the general contract, reducing the risk of violations.
By adhering to these principles and practices, developers can create robust comparison methods that maintain consistency and reliability in their applications.
Understanding the Implications of Comparison Method Violations
Dr. Emily Carter (Computer Scientist, Algorithm Research Institute). “The violation of the general contract in comparison methods can lead to unpredictable behavior in sorting algorithms. This compromise not only affects the integrity of the data but also undermines the reliability of applications that depend on accurate ordering.”
Michael Thompson (Software Engineer, Tech Innovations Corp). “When a comparison method violates its general contract, it can result in inconsistent sorting outcomes. This inconsistency can introduce critical bugs in software systems, especially in scenarios requiring stable sorting, such as database management and user interface design.”
Laura Chen (Data Scientist, Analytics Solutions Group). “In data analysis, the integrity of comparison methods is paramount. A violation of the general contract can skew results, leading to erroneous conclusions and decisions based on flawed data interpretations. Ensuring adherence to these contracts is essential for maintaining analytical rigor.”
Frequently Asked Questions (FAQs)
What does it mean when a comparison method violates its general contract?
A comparison method violates its general contract when it fails to adhere to the principles of consistency and transitivity required by the comparison operation, leading to unpredictable or erroneous results.
What are the consequences of a violation of the comparison method’s general contract?
Consequences include incorrect sorting or ordering of elements, which can result in failures in algorithms that rely on these comparisons, such as sorting algorithms or data structures like priority queues.
How can one identify if a comparison method is violating its general contract?
One can identify violations by testing the comparison method with various input values to check for inconsistencies, such as if `compare(a, b)` returns a different result than `compare(b, a)` or if it fails to maintain transitive relationships.
What are the key principles of a valid comparison method?
The key principles include reflexivity (an element is equal to itself), antisymmetry (if two elements are equal, they cannot be unequal), and transitivity (if one element is greater than a second, and the second is greater than a third, then the first must be greater than the third).
Can a comparison method be fixed if it violates its general contract?
Yes, a comparison method can be fixed by revising its logic to ensure it adheres to the principles of consistency, reflexivity, antisymmetry, and transitivity, thus restoring its reliability for sorting and comparison tasks.
What are common examples of comparison methods that can violate their general contract?
Common examples include improperly implemented `compareTo` methods in Java, where the logic may inadvertently allow for inconsistencies, such as failing to handle edge cases or using non-deterministic criteria for comparison.
The comparison method in programming, particularly in the context of sorting algorithms and data structures, is crucial for maintaining the expected behavior of collections and ensuring that they function correctly. When a comparison method violates its general contract, it can lead to inconsistent results, unpredictable behavior, and potential errors in data processing. The general contract typically requires that comparisons be consistent, transitive, and reflexive, meaning that if an object A is considered less than object B, and object B is less than object C, then object A must also be less than object C. Any violation of these principles can disrupt the integrity of algorithms that rely on these comparisons.
Moreover, the implications of a flawed comparison method extend beyond immediate functionality. They can affect the performance of sorting operations, lead to incorrect ordering of elements, and ultimately result in failures in applications that depend on precise data handling. It is essential for developers to rigorously test and validate their comparison methods to ensure they adhere to the expected contract. This includes checking for edge cases and ensuring that the method behaves consistently across different scenarios.
the importance of adhering to the general contract of comparison methods cannot be overstated. Developers must prioritize the integrity of these methods to maintain the reliability and efficiency of their applications.
Author Profile
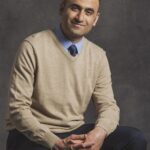
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?