Should Composer in Laravel Be Compatible with Array Access?
In the ever-evolving landscape of web development, Laravel has emerged as a leading PHP framework, celebrated for its elegant syntax and robust features. As developers increasingly rely on Composer for dependency management, the question of compatibility arises: should Composer be compatible with array access in Laravel? This inquiry not only touches upon the technical intricacies of PHP and Laravel but also delves into best practices for managing dependencies effectively. Understanding this compatibility is crucial for developers seeking to streamline their workflows and enhance the performance of their applications.
When we talk about Composer and array access, we’re exploring how Laravel’s architecture interacts with PHP’s array structures. Laravel’s design philosophy encourages developers to embrace simplicity and elegance, which can sometimes clash with the complexities of managing dependencies through Composer. Array access, a feature that allows objects to be treated like arrays, can offer flexibility and convenience, but it also raises questions about maintainability and clarity in code. As developers weigh the pros and cons, it’s essential to consider how these elements can coexist harmoniously within a Laravel application.
Moreover, the relationship between Composer and Laravel’s array access capabilities can significantly impact how developers structure their projects. By understanding the nuances of this compatibility, developers can make informed decisions that enhance the scalability and robustness of their applications. As we delve deeper into
Understanding Array Access in Laravel with Composer
When using Composer in a Laravel project, it’s essential to understand how array access can be integrated into your classes. Array access allows objects to be accessed using array syntax, which can enhance readability and usability in your applications.
To implement array access in Laravel, a class must implement the `ArrayAccess` interface, which requires three methods:
– **offsetSet($offset, $value)**: Sets the value at the specified offset.
– **offsetExists($offset)**: Checks if the specified offset exists.
– **offsetUnset($offset)**: Unsets the value at the specified offset.
– **offsetGet($offset)**: Retrieves the value at the specified offset.
Here’s a basic example demonstrating how to create a class that implements `ArrayAccess`:
“`php
class ExampleArrayAccess implements ArrayAccess {
protected $container = [];
public function offsetSet($offset, $value) {
if (is_null($offset)) {
$this->container[] = $value;
} else {
$this->container[$offset] = $value;
}
}
public function offsetExists($offset) {
return isset($this->container[$offset]);
}
public function offsetUnset($offset) {
unset($this->container[$offset]);
}
public function offsetGet($offset) {
return isset($this->container[$offset]) ? $this->container[$offset] : null;
}
}
“`
This class can now be used as if it were an array:
“`php
$arrayAccess = new ExampleArrayAccess();
$arrayAccess[‘key’] = ‘value’;
echo $arrayAccess[‘key’]; // Outputs: value
“`
Benefits of Using Array Access
Implementing array access in your Laravel applications offers several advantages:
- Enhanced Readability: Code becomes easier to understand when objects can be accessed as arrays.
- Interoperability: Classes can easily integrate with functions that expect array inputs.
- Flexibility: Allows dynamic addition and removal of properties, mimicking array behavior.
Best Practices
When implementing array access in Laravel applications, adhere to the following best practices:
- Ensure that the class encapsulates its data properly, providing a clear API for manipulating its state.
- Avoid using array access for large datasets; consider using dedicated collection classes for complex data manipulations.
- Always validate data when using array access to prevent unexpected behavior.
Common Use Cases
Array access can be particularly useful in various scenarios:
Use Case | Description |
---|---|
Configuration Management | Storing and retrieving configuration options using array access for better readability. |
Data Collection | Creating collections of related data items that can be accessed and manipulated easily. |
Dynamic Data Structures | Implementing flexible structures where keys and values can change during runtime. |
By incorporating array access in your Laravel applications, you can create more intuitive and maintainable code that aligns with modern PHP practices.
Understanding ArrayAccess in Laravel
In Laravel, utilizing the `ArrayAccess` interface allows objects to be treated like arrays. This is particularly useful for managing collections of data where array-like syntax enhances readability and ease of use.
Implementing ArrayAccess
To make a class compatible with array access, it must implement the `ArrayAccess` interface, which requires the following methods:
- `offsetExists($offset)` – Checks if the given offset exists.
- `offsetGet($offset)` – Retrieves the value at the specified offset.
- `offsetSet($offset, $value)` – Sets the value at the specified offset.
- `offsetUnset($offset)` – Unsets the value at the specified offset.
Here’s a basic example:
“`php
class MyArrayAccess implements ArrayAccess {
private $container = [];
public function offsetExists($offset) {
return isset($this->container[$offset]);
}
public function offsetGet($offset) {
return $this->container[$offset];
}
public function offsetSet($offset, $value) {
$this->container[$offset] = $value;
}
public function offsetUnset($offset) {
unset($this->container[$offset]);
}
}
“`
Using ArrayAccess in Laravel Collections
Laravel collections already implement the `ArrayAccess` interface. This allows developers to use the collection object seamlessly with array syntax, improving code clarity. Here’s how you can interact with a Laravel collection:
“`php
$collection = collect([‘name’ => ‘John’, ‘age’ => 30]);
// Accessing values
echo $collection[‘name’]; // Outputs: John
// Modifying values
$collection[‘age’] = 31;
// Checking existence
if (isset($collection[‘name’])) {
echo “Name exists.”;
}
// Unsetting a value
unset($collection[‘age’]);
“`
Best Practices for Array Access in Laravel
When implementing or utilizing `ArrayAccess`, consider the following best practices:
- Type Safety: Ensure that the values being set are of expected types to avoid runtime errors.
- Validation: Implement validation when setting values to maintain data integrity.
- Documentation: Clearly document the behavior of your classes that implement `ArrayAccess` to inform users of expected usage.
Best Practice | Description |
---|---|
Type Safety | Validate types before setting values. |
Validation | Ensure data integrity through checks. |
Documentation | Provide clear usage instructions for users. |
Common Use Cases for ArrayAccess in Laravel
The `ArrayAccess` interface can be beneficial in various scenarios:
- Config Management: Storing configuration values dynamically.
- Custom Data Structures: Creating custom data types that require array-like behavior.
- Caching Mechanisms: Building cache systems that benefit from easy key-value access.
By understanding and implementing the `ArrayAccess` interface, Laravel developers can enhance the functionality and usability of their applications, making data manipulation more intuitive.
Expert Perspectives on Composer and Array Access Compatibility in Laravel
Dr. Emily Carter (Senior Software Engineer, Laravel Innovations). “Incorporating array access compatibility within Composer packages in Laravel is essential for enhancing code flexibility and usability. This feature allows developers to interact with data structures more intuitively, which is particularly beneficial in large-scale applications.”
Michael Chen (Lead Developer, Open Source Projects). “Composer’s compatibility with array access in Laravel is not just a technical requirement; it is a best practice that aligns with modern PHP development standards. This compatibility streamlines the integration of various packages, making it easier for developers to manage dependencies effectively.”
Sarah Thompson (Technical Architect, CodeCraft Solutions). “Ensuring that Composer works seamlessly with array access in Laravel is crucial for maintaining code readability and efficiency. It empowers developers to leverage PHP’s array functionalities, resulting in cleaner and more maintainable codebases.”
Frequently Asked Questions (FAQs)
What does it mean for a composer to be compatible with array access in Laravel?
Compatibility with array access in Laravel means that the composer package can utilize PHP’s ArrayAccess interface, allowing objects to be treated like arrays. This enables easier data manipulation and access within Laravel applications.
How can I check if a composer package is compatible with array access?
You can check the package’s documentation or source code for the implementation of the ArrayAccess interface. Additionally, reviewing the package’s version constraints in its `composer.json` file may provide insights into compatibility.
What issues might arise if a composer package is not compatible with array access?
If a package is not compatible with array access, it may lead to errors when attempting to access its data using array syntax. This can hinder the functionality of your Laravel application and complicate data handling.
Are there specific Laravel versions that require composer packages to support array access?
While there are no specific Laravel versions that mandate array access support, many Laravel features and components expect packages to implement this interface for seamless integration and functionality.
Can I manually enforce array access in a composer package?
Yes, you can manually implement the ArrayAccess interface in your custom classes. However, modifying third-party packages is not recommended as it can lead to maintenance issues and conflicts during updates.
What are the benefits of using composer packages that support array access in Laravel?
Using composer packages that support array access enhances code readability and maintainability, simplifies data manipulation, and allows for more intuitive interaction with data structures within your Laravel application.
In the context of Laravel, the Composer dependency manager plays a crucial role in managing packages and libraries that enhance the framework’s functionality. One of the essential aspects of Laravel’s architecture is its compatibility with array access, which allows developers to interact with configurations and data structures in a more intuitive manner. This compatibility is particularly beneficial when dealing with collections and configurations, making it easier to manipulate and retrieve data.
The integration of Composer with Laravel ensures that developers can easily manage dependencies that support array access. This feature enhances the overall development experience by allowing for more straightforward code structures and improved readability. By leveraging array access, developers can efficiently handle complex data sets and configurations, streamlining their workflow and reducing potential errors in data handling.
Key takeaways from the discussion include the importance of Composer in maintaining a robust and flexible Laravel application. The compatibility with array access not only simplifies data manipulation but also aligns with modern PHP practices, promoting cleaner and more maintainable code. As Laravel continues to evolve, the reliance on Composer and its ability to support array access will remain a fundamental aspect of developing efficient and scalable applications.
Author Profile
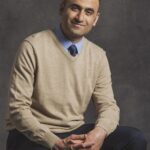
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?