How Can You Create a Contourf Plot in Matplotlib Using a Stretched Grid?
In the realm of data visualization, the ability to effectively represent complex datasets is paramount. One of the most powerful tools at a data scientist’s disposal is Matplotlib, a versatile plotting library in Python. Among its many features, the `contourf` function stands out, allowing users to create filled contour plots that vividly illustrate the relationships between variables. However, when working with irregularly spaced or stretched grids, achieving the desired visual representation can become a challenge. In this article, we will explore how to harness the capabilities of `contourf` in Matplotlib to create stunning visualizations that accommodate stretched grids, ensuring that your data is not only accurate but also aesthetically pleasing.
Understanding how to manipulate grids is essential for effective data visualization, especially when dealing with geographical data or simulations that require specific scaling. A stretched grid can distort the appearance of your data, making it crucial to apply the right techniques to maintain clarity and precision. This article will guide you through the intricacies of using `contourf` with stretched grids, highlighting key strategies and best practices that can elevate your plotting skills.
As we delve deeper into the topic, we will examine the fundamental concepts behind contour plotting and the specific adjustments needed for stretched grids. Whether you are a seasoned data analyst
Understanding Stretched Grids in Matplotlib
Stretched grids are a powerful tool in data visualization, particularly when dealing with non-uniform data distributions. In Matplotlib, employing a stretched grid can enhance the accuracy of contour plots by allowing for the representation of data that varies significantly over certain areas. This approach is particularly useful in fields such as meteorology, oceanography, and any other area where data may not be evenly distributed across a spatial domain.
A stretched grid is essentially a transformation of the coordinate system, where the spacing between grid points can vary. This allows for a more precise representation of data in regions where more detail is needed. To implement a stretched grid in a contour plot using Matplotlib, one can utilize the `numpy` library to create the grid coordinates and the `matplotlib.pyplot` module for plotting.
Creating a Contour Plot with a Stretched Grid
To create a contour plot with a stretched grid in Matplotlib, follow these steps:
- Define the grid: Use `numpy` to create a meshgrid that stretches in specified directions.
- Generate data: Create a function that defines the values at each point on the grid.
- Plot the data: Use `contourf` to visualize the data, specifying the grid and the data.
Here is an example of how to implement this:
“`python
import numpy as np
import matplotlib.pyplot as plt
Define the stretched grid parameters
x = np.linspace(-3, 3, 100)
y = np.linspace(-3, 3, 80)
X, Y = np.meshgrid(x, y)
Apply a transformation to stretch the grid
X_stretched = X ** 2
Y_stretched = Y ** 2
Define the function to plot
Z = np.sin(np.sqrt(X_stretched + Y_stretched))
Create the contour plot
plt.figure()
contour = plt.contourf(X, Y, Z, levels=20, cmap=’viridis’)
plt.colorbar(contour)
plt.title(‘Contour Plot with Stretched Grid’)
plt.xlabel(‘X-axis’)
plt.ylabel(‘Y-axis’)
plt.show()
“`
Key Considerations When Using Stretched Grids
When employing stretched grids in contour plots, several factors should be kept in mind to ensure effective visualization:
- Grid Resolution: The density of points in the stretched grid can significantly affect the smoothness and accuracy of the contours. Higher resolution may be necessary in areas of interest.
- Data Range: Ensure that the data values are within the range suitable for the chosen colormap, as this will impact the visual interpretation.
- Interpolation: Depending on the data distribution, consider whether interpolation is needed to fill in gaps or create a smoother contour representation.
Example of Stretched Grid Parameters
Below is a table summarizing possible transformations for creating stretched grids:
Transformation | Description |
---|---|
X^2 | Stretches the grid along the X-axis, emphasizing regions further from the origin. |
Y^2 | Stretches the grid along the Y-axis, emphasizing vertical regions. |
sin(X) | Creates a wavy effect, useful for periodic data. |
exp(X) | Increases density exponentially, suitable for data that grows rapidly. |
By carefully selecting the transformation and understanding the implications of a stretched grid, one can significantly enhance the quality and interpretability of contour plots in Matplotlib.
Creating Contour Plots with Stretched Grids in Matplotlib
To create contour plots in Matplotlib using a stretched grid, it is essential to understand how to manipulate the grid coordinates effectively. A stretched grid can provide better representation of data where variations are more pronounced in certain regions.
Defining the Stretched Grid
A stretched grid involves transforming the standard Cartesian grid into a non-uniform grid where spacing varies. This can be achieved using various techniques, such as linear interpolation or more complex transformations.
- Linear Transformation: Adjust the grid points linearly based on a function.
- Non-linear Transformation: Apply functions like sine, exponential, or polynomial for more complex stretching.
Here is a simple example of defining a stretched grid using NumPy:
“`python
import numpy as np
Define a standard grid
x = np.linspace(0, 1, 100)
y = np.linspace(0, 1, 100)
X, Y = np.meshgrid(x, y)
Apply a stretching function
X_stretched = X**2 Example of quadratic stretching
Y_stretched = Y**2
“`
Generating Data for Contour Plot
For the contour plot to be meaningful, it is necessary to compute a function over the stretched grid:
“`python
Z = np.sin(2 * np.pi * X_stretched) * np.cos(2 * np.pi * Y_stretched)
“`
This function combines sine and cosine functions, which can yield interesting contour patterns when visualized.
Plotting the Contour with Matplotlib
To visualize the stretched grid using contour plots, Matplotlib’s `contourf` function can be employed effectively. Below is a complete example of generating and displaying the contour plot:
“`python
import matplotlib.pyplot as plt
Create the contour plot
plt.figure(figsize=(8, 6))
contour = plt.contourf(X_stretched, Y_stretched, Z, levels=20, cmap=’viridis’)
plt.colorbar(contour)
plt.title(‘Contour Plot with Stretched Grid’)
plt.xlabel(‘Stretched X’)
plt.ylabel(‘Stretched Y’)
plt.show()
“`
Key Parameters in `contourf`:
- `X`, `Y`: These are the stretched grid arrays.
- `Z`: The computed values based on the function.
- `levels`: Defines the number of contour levels.
- `cmap`: The colormap to use for filling contours.
Customization Options
Customizing contour plots enhances visual appeal and clarity. Here are some options to consider:
- Colormap: Change the colormap to represent data differently (e.g., `cmap=’plasma’`).
- Contour Levels: Specify custom levels to highlight specific ranges of data.
- Line Styles: Use `contour` function to overlay contour lines on the filled contours for additional detail.
Example of customization:
“`python
Overlay contour lines
contour_lines = plt.contour(X_stretched, Y_stretched, Z, colors=’black’, linewidths=0.5)
plt.clabel(contour_lines, inline=True, fontsize=8)
“`
Utilizing a stretched grid in contour plots allows for more accurate representation of complex data distributions. The steps provided here facilitate the creation of visually appealing and informative contour plots with Matplotlib.
Expert Insights on Using Contourf in Matplotlib with Stretched Grids
Dr. Emily Carter (Data Visualization Specialist, TechViz Solutions). Contourf plots in Matplotlib are powerful for visualizing 2D data, but when working with stretched grids, it is crucial to understand how to manipulate the grid coordinates effectively. By using the `set_aspect` function, one can maintain the integrity of the data representation, ensuring that the contours accurately reflect the underlying data distribution.
Michael Chen (Senior Software Engineer, Data Science Innovations). When implementing contourf with stretched grids, I recommend utilizing the `matplotlib.tri` module. This allows for more flexibility with irregularly spaced data points. The triangulation process can help in creating a more accurate contour representation, especially when the grid is not uniform.
Dr. Sarah Thompson (Geospatial Analyst, GeoData Insights). It is essential to consider the interpolation methods when using contourf with stretched grids. Techniques such as linear or cubic interpolation can significantly impact the visual output. Choosing the right method based on the data characteristics will enhance the clarity and accuracy of the contour plots, making them more informative for analysis.
Frequently Asked Questions (FAQs)
What is a stretched grid in the context of contourf in Matplotlib?
A stretched grid refers to a non-uniform grid where the spacing between points varies, often used to represent data more accurately in regions of interest. In Matplotlib, this can enhance the visualization of contour plots by allowing for more detail in specific areas.
How can I create a contourf plot using a stretched grid in Matplotlib?
To create a contourf plot with a stretched grid, you must first define the grid points using functions like `numpy.meshgrid` with non-linear spacing. Then, you can use the `contourf` function to visualize the data on this grid.
What libraries are necessary to implement contourf with a stretched grid?
You primarily need Matplotlib for plotting and NumPy for handling numerical operations and creating the grid. Optionally, you may use SciPy for more advanced interpolation techniques if needed.
Can I customize the color map in a contourf plot with a stretched grid?
Yes, you can customize the color map by using the `cmap` parameter in the `contourf` function. Matplotlib provides several built-in colormaps, and you can also create a custom colormap if desired.
Are there any performance considerations when using a stretched grid for contourf plots?
Yes, using a stretched grid can increase computational complexity, especially with large datasets. It’s advisable to optimize the grid size and consider using interpolation techniques to balance performance and visualization quality.
How do I handle missing data when creating a contourf plot with a stretched grid?
You can handle missing data by using interpolation methods available in libraries like SciPy. Functions such as `griddata` can be useful for filling in gaps in your data before plotting with `contourf`.
In summary, utilizing the `contourf` function in Matplotlib to create contour plots on a stretched grid is a powerful technique for visualizing data that is not uniformly distributed. A stretched grid allows for more flexibility in representing complex geometries or varying densities of data points. This is particularly useful in fields such as meteorology, oceanography, and engineering, where the spatial distribution of data can significantly impact the interpretation of results.
Key insights include the importance of understanding the underlying grid structure when working with contour plots. By applying transformations to the grid, users can effectively highlight areas of interest or improve the clarity of the visual representation. Additionally, the combination of `numpy` for numerical operations and `matplotlib` for plotting facilitates the creation of sophisticated visualizations that can accommodate a variety of data configurations.
Furthermore, it is essential to consider the parameters of the `contourf` function, such as the levels of contours and colormaps, which can greatly influence the final output. Properly customizing these parameters enhances the interpretability of the plot, allowing for better communication of the data’s significance. Overall, mastering the use of `contourf` on stretched grids can greatly enhance data visualization capabilities in scientific and engineering applications.
Author Profile
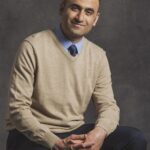
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?