How Can You Convert an Enum to an Int in Programming?
Enums, or enumerations, are a powerful feature in many programming languages that allow developers to define a set of named constants. They enhance code readability and maintainability by providing meaningful names to a collection of related values. However, there are times when you might need to convert these enums into integers, particularly when interfacing with systems that require numerical representations or when performing calculations. Understanding how to convert an enum to an int can streamline your code and improve its efficiency.
In this article, we will explore the process of converting enums to integers across various programming languages. We will delve into the reasons why such conversions are necessary, including data storage, serialization, and performance considerations. Additionally, we will highlight the nuances of enum representation, as the underlying integer values can vary depending on the language and the specific implementation of the enum type.
By the end of this discussion, you will have a clear understanding of the methods available for converting enums to integers, along with practical examples to illustrate these techniques. Whether you are a seasoned developer or just starting your coding journey, mastering this conversion will enhance your programming toolkit and empower you to write cleaner, more efficient code.
Understanding Enums in Programming
Enums, or enumerations, are a special data type that enables a variable to be a set of predefined constants. This can improve code readability and maintenance by giving meaningful names to sets of numeric values. In many programming languages, each constant in an enum is associated with an integer value, starting from zero by default and incrementing by one for each subsequent constant.
For example, consider the following enum in C:
“`csharp
enum Days
{
Sunday, // 0
Monday, // 1
Tuesday, // 2
Wednesday, // 3
Thursday, // 4
Friday, // 5
Saturday // 6
}
“`
In this case, `Days.Sunday` has an integer value of 0, `Days.Monday` has a value of 1, and so forth.
Converting an Enum to an Int
Converting an enum to an integer is straightforward in most programming languages. You can cast the enum value to its underlying integer type. Here’s how to accomplish this in several common programming languages:
- C: Use a simple cast.
- Java: Use the `ordinal()` method.
- Python: Use the `value` attribute in the Enum class.
- C++: Use a static cast.
Here is a comparison of how to convert an enum to an integer in these languages:
Language | Code Example |
---|---|
C | int dayValue = (int)Days.Monday; |
Java | int dayValue = Days.MONDAY.ordinal(); |
Python | day_value = Days.MONDAY.value; |
C++ | int dayValue = static_cast |
Best Practices for Enum Conversion
When converting enums to integers, consider the following best practices:
- Clarity: Always ensure that the intent of the conversion is clear in the code. Use descriptive variable names.
- Type Safety: Be cautious of type conversions and try to avoid situations that may lead to logical errors or loss of information.
- Consistency: Maintain consistent use of enums throughout your codebase to promote maintainability and readability.
- Error Handling: Implement error handling to manage scenarios where an invalid enum value might be encountered.
By adhering to these practices, developers can effectively manage enum conversions while minimizing potential errors in their applications.
Understanding Enum in Programming
Enums, or enumerations, are a special data type that allows a variable to be a set of predefined constants. Enums enhance code readability and maintainability by allowing developers to use meaningful names rather than numeric values. The underlying values of enums typically start from zero and increment by one for each subsequent value unless explicitly defined otherwise.
- Advantages of Using Enums:
- Improves code clarity and self-documentation.
- Prevents invalid values by restricting input to predefined constants.
- Simplifies refactoring since the names are easier to track than numeric constants.
Converting an Enum to an Integer
To convert an enum to an integer, you can use the casting feature available in many programming languages. The specific syntax may vary, but the fundamental principle remains consistent across languages like C, Java, and Python.
- CExample:
“`csharp
enum Colors { Red, Green, Blue }
Colors myColor = Colors.Green;
int colorValue = (int)myColor; // colorValue will be 1
“`
- Java Example:
“`java
enum Colors { RED, GREEN, BLUE }
Colors myColor = Colors.GREEN;
int colorValue = myColor.ordinal(); // colorValue will be 1
“`
- Python Example:
“`python
from enum import Enum
class Colors(Enum):
RED = 1
GREEN = 2
BLUE = 3
my_color = Colors.GREEN
color_value = my_color.value color_value will be 2
“`
In these examples, the conversion allows developers to leverage the advantages of enums while still being able to work with their integer representations when necessary.
Practical Considerations
When converting enums to integers, it is essential to keep in mind:
- Consistency: Ensure that the enum values are consistent across different parts of your application. Changing the order or values of enums can lead to bugs if the integer representations are hardcoded.
- Error Handling: Implement error handling when converting from integers back to enums, as invalid integer values can lead to exceptions.
- Performance: The conversion from enum to integer is generally efficient, but if performed in performance-critical sections, consider measuring the impact.
Common Use Cases
Enums are commonly used in scenarios such as:
- State Management: Representing the state of an object or process (e.g., `OrderStatus` with values like `Pending`, `Shipped`, `Delivered`).
- Configuration Settings: Defining options for configuration (e.g., `LogLevel` with values like `Debug`, `Info`, `Warning`, `Error`).
- User Permissions: Managing access levels in applications (e.g., `UserRole` with values like `Admin`, `Editor`, `Viewer`).
In these cases, converting enums to integers can facilitate storage in databases, transmission over networks, or integration with systems that require numerical representations.
Converting enums to integers is a straightforward process that varies slightly across programming languages but generally involves casting or using built-in methods. This conversion allows developers to maintain the benefits of enums while accommodating the needs of their applications.
Expert Insights on Converting Enums to Integers
Dr. Emily Carter (Lead Software Architect, Tech Innovations Inc.). “Converting an enum to an int is a common practice in programming that enhances performance and memory efficiency. It allows developers to use enums as integral values, making operations like comparisons and arithmetic straightforward.”
James Liu (Senior Developer, CodeCraft Solutions). “When converting enums to integers, it is essential to consider the underlying integer values assigned to each enum member. This ensures that the conversion aligns with the intended logic of the application and avoids unexpected behavior.”
Sarah Thompson (Programming Language Researcher, Global Tech Institute). “Enums provide a way to define named constants, and converting them to integers is often necessary for interoperability with systems that require numeric representations. Proper handling of these conversions is crucial to maintain type safety and prevent bugs.”
Frequently Asked Questions (FAQs)
How do you convert an enum to an int in C?
In C, you can convert an enum to an int by casting it directly. For example, if you have an enum named `MyEnum`, you can use `(int)MyEnum.Value` to obtain its underlying integer value.
Can you convert an enum to an int in Java?
Yes, in Java, you can convert an enum to an int by using the `ordinal()` method. This method returns the position of the enum constant, starting from zero. For example, `MyEnum.VALUE.ordinal()` gives you the integer representation.
Is it safe to convert an enum to an int in programming?
Converting an enum to an int is generally safe, but it is important to understand that the integer value corresponds to the enum’s definition order, which can change if the enum definition is modified. Always ensure that the enum constants are stable.
What happens if you convert an enum to an int and the value is not defined?
If you convert an enum to an int that does not correspond to any defined enum constant, it may lead to unexpected behavior or errors, depending on the programming language and context. It is advisable to validate the enum value before conversion.
Can you convert an enum back from an int?
Yes, you can convert an enum back from an int by using the `Enum.ToObject()` method in Cor by using a switch statement or a loop to match the integer value with the corresponding enum constant in other languages.
Are there any performance implications when converting enums to ints?
The performance implications of converting enums to ints are minimal and typically negligible in most applications. However, frequent conversions in performance-critical sections of code may warrant consideration of alternative approaches.
Converting an enum to an int is a common task in programming, particularly in languages such as C, Java, and C++. Enums, or enumerations, are a special data type that allows for a variable to be a set of predefined constants. Each constant in an enum is associated with an underlying integral value, typically starting from zero and incrementing by one for each subsequent constant. Understanding how to convert these enum values to integers is essential for tasks such as serialization, storage, and interoperability with systems that require integer representations.
To perform the conversion, most programming languages provide straightforward methods. For instance, in C, you can cast an enum to its underlying type directly using a simple cast operation. In Java, the ordinal() method can be used to retrieve the index of the enum constant, effectively giving its integer representation. This conversion is not only useful for debugging but also for scenarios where enums need to be stored in databases or transmitted over networks.
It is important to note that while converting an enum to an int is generally simple, developers should be cautious about the implications of such conversions. For example, relying solely on the integer values of enums can lead to issues if the enum definition changes, such as reordering constants or adding
Author Profile
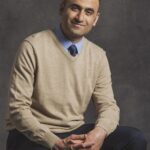
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?