How Can I Convert a Date from Y-M-D Format to String Month-Day in R?
In the world of data analysis and programming, the ability to manipulate and format dates is a crucial skill. Whether you’re working with datasets in R for statistical analysis, creating visualizations, or preparing reports, understanding how to convert dates into different formats can save you time and enhance your insights. One common task that many R users encounter is converting dates from the standard year-month-day (y-m-d) format into a more reader-friendly string format, such as month-day. This transformation not only improves the readability of your data but also allows for better communication of findings in presentations and reports.
When working with date data in R, you may often find yourself needing to change the way dates are displayed. The default y-m-d format is useful for many computational tasks, but it can be less intuitive for human readers. By converting dates into a string format that emphasizes the month and day, you can create outputs that are more accessible and engaging. This article will explore the methods and functions available in R for achieving this conversion, highlighting the simplicity and efficiency of the process.
As we delve into the details, you’ll discover how to leverage R’s powerful date handling capabilities to transform your date formats seamlessly. Whether you’re a beginner or an experienced programmer, the techniques discussed will equip you with the
Date Conversion Techniques in R
To convert dates from the `y-m-d` format to a string representation in the `month-day` format within R, you can utilize the `as.Date()` function along with the `format()` function. This process involves first converting your date string to a date object and then formatting it into the desired string format.
Here’s a step-by-step breakdown:
- Convert to Date Object: Use the `as.Date()` function to convert the date string from the `y-m-d` format to an R Date object.
- Format the Date: After conversion, apply the `format()` function to extract the month and day in the desired format.
Example Code
“`R
Sample date in y-m-d format
date_str <- "2023-10-15"
Convert to Date object
date_obj <- as.Date(date_str)
Format to month-day
formatted_date <- format(date_obj, "%m-%d")
Print the formatted date
print(formatted_date)
```
Explanation of Functions
- as.Date(): This function converts character representations of dates into Date objects. The default format is `”%Y-%m-%d”`, which corresponds to `y-m-d`.
- format(): This function formats a Date object according to a specified format string. The format string `”%m-%d”` specifies that the output should be in `month-day` format.
Additional Examples
You can also handle a vector of dates or convert various date formats using a similar approach.
“`R
Vector of dates
date_vec <- c("2023-01-01", "2023-02-14", "2023-12-31")
Convert and format
formatted_dates <- format(as.Date(date_vec), "%m-%d")
Print the formatted dates
print(formatted_dates)
```
Resulting Output
The resulting output for the above vector would be:
```
[1] "01-01" "02-14" "12-31"
```
Summary Table of Date Formatting Codes
Below is a table summarizing common format codes used in R for date formatting:
Format Code | Description |
---|---|
%Y | 4-digit year |
%y | 2-digit year |
%m | Month (01-12) |
%d | Day of the month (01-31) |
%B | Full month name |
%b | Abbreviated month name |
By using these functions and understanding the format codes, you can efficiently convert dates in R to meet your specific formatting needs.
Conversion Process
To convert a date from the format `year-month-day` (y-m-d) to a string format of `month-day` in R, the `as.Date()` function can be utilized in conjunction with `format()`. The following steps outline this process:
- Input Date: Ensure your date is in the correct format (y-m-d).
- Convert to Date Object: Use `as.Date()` to convert the string to a Date object.
- Format the Date: Utilize the `format()` function to convert the Date object to the desired string format.
Here is an example demonstrating these steps:
“`r
Example date in y-m-d format
date_string <- "2023-10-15"
Step 1: Convert to Date object
date_object <- as.Date(date_string)
Step 2: Format to month-day
formatted_date <- format(date_object, "%m-%d")
Output the result
formatted_date
```
This code will produce the output `10-15`, representing October 15th.
Function Explanation
- as.Date(): This function takes a character string and converts it into a Date object. The default format is `yyyy-mm-dd`, but custom formats can be specified if necessary.
- format(): The `format()` function takes a Date object and converts it into a character string based on the specified format. In this case, `%m-%d` indicates the desired output of month and day.
Handling Different Formats
If your input date format varies or is not in the standard `y-m-d` format, you can specify the format directly in the `as.Date()` function. For example:
“`r
Example date in d/m/y format
date_string <- "15/10/2023"
Convert to Date object with specified format
date_object <- as.Date(date_string, format="%d/%m/%Y")
Format to month-day
formatted_date <- format(date_object, "%m-%d")
formatted_date
```
In this case, the output will still be `10-15`, correctly interpreting the input date format.
Vectorized Operations
To convert multiple dates at once, you can use a vector of dates. The method remains the same but applied to the entire vector:
“`r
Vector of dates in y-m-d format
date_vector <- c("2023-10-15", "2023-11-20", "2023-12-25")
Convert and format
formatted_dates <- format(as.Date(date_vector), "%m-%d")
Output the result
formatted_dates
```
This will output:
```
[1] "10-15" "11-20" "12-25"
```
Considerations for Locale
Be aware that the locale may affect the output format when dealing with month names or translations. If your requirements involve localization, you can set the locale before formatting:
“`r
Set locale for month names
Sys.setlocale(“LC_TIME”, “en_US.UTF-8”) Example for English
Format with month names
formatted_dates_with_names <- format(as.Date(date_vector), "%B %d")
formatted_dates_with_names
```
This would produce full month names such as `October 15` for each date in the vector. Adjust the locale according to your needs for other languages.
Expert Insights on Date Conversion in R
Dr. Emily Chen (Data Scientist, R Analytics Group). “To convert a date from the format ‘y-m-d’ to a string format ‘month-day’ in R, one can utilize the `format()` function. This function allows for the specification of desired output formats, making it straightforward to achieve the desired string representation.”
Michael Thompson (Senior R Programmer, Data Insights Inc.). “Using the `as.Date()` function in conjunction with `format()` is an effective method for this conversion. By first ensuring the date is in the correct class, you can then easily manipulate the output to reflect the ‘month-day’ format.”
Lisa Patel (Statistical Consultant, Quantitative Solutions). “It’s essential to pay attention to the locale settings in R when formatting dates. If you want the month names to be displayed in a specific language, ensure that your R session is set to the appropriate locale before performing the conversion.”
Frequently Asked Questions (FAQs)
How can I convert a date from ‘y-m-d’ format to ‘month-day’ format in R?
You can use the `as.Date()` function to convert the date string to a Date object, followed by the `format()` function to convert it to the desired format. For example:
“`R
date_string <- "2023-10-05"
date_object <- as.Date(date_string)
formatted_date <- format(date_object, "%B-%d")
```
What does the format specifier ‘%B’ represent in R?
The format specifier ‘%B’ represents the full month name in R. When used in the `format()` function, it will convert the date to display the complete name of the month.
Can I convert multiple dates at once in R?
Yes, you can convert multiple dates by applying the conversion functions to a vector of date strings. Use the `sapply()` or `lapply()` function to iterate over the vector. For example:
“`R
date_strings <- c("2023-10-05", "2023-11-15")
formatted_dates <- sapply(date_strings, function(x) format(as.Date(x), "%B-%d"))
```
What will happen if the input date is not in ‘y-m-d’ format?
If the input date is not in the ‘y-m-d’ format, the `as.Date()` function may return `NA` or throw an error, indicating that the input could not be parsed. It is essential to ensure that the date strings are correctly formatted.
Is it possible to customize the output format further?
Yes, you can customize the output format by using different format specifiers in the `format()` function. For example, you can include the year by using `”%B-%d-%Y”` to display the full month name, day, and year.
What packages can assist with date formatting in R?
The `lubridate` package is highly recommended for date manipulation and formatting in R. It provides user-friendly functions for parsing and formatting dates, making it easier to work with various date formats.
Converting dates from the format year-month-day (y-m-d) to a string representation in the format month-day is a common task in data manipulation using R. This process typically involves utilizing R’s date handling capabilities, specifically the `as.Date()` function to parse the original date format and the `format()` function to convert it into the desired string format. By following these steps, users can efficiently transform their date data for better readability and presentation.
One of the key insights from this discussion is the importance of understanding date formats in R. Dates can be represented in various formats, and knowing how to manipulate these formats is essential for effective data analysis. The `format()` function is particularly useful, as it allows for customization of the output string, enabling users to specify exactly how they want their dates to appear. This flexibility is crucial for reporting and visualization purposes.
Additionally, it is worth noting that proper date conversion can prevent potential errors in data analysis. When dates are not formatted correctly, it can lead to misinterpretation of data, especially when performing operations that rely on chronological order. Therefore, mastering date conversions in R not only enhances data presentation but also ensures the integrity of the analysis being conducted.
Author Profile
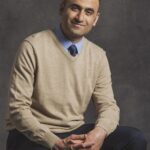
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?