How Can You Convert a Hardcoded String to a Date in C?
In the world of programming, handling dates and times is a fundamental skill that can significantly enhance the functionality of applications. For developers working with the C programming language, converting hardcoded strings into date formats is a common yet crucial task. Whether you’re dealing with user inputs, reading data from files, or simply formatting dates for display, mastering this conversion can streamline your code and improve its readability. In this article, we will delve into the techniques for transforming hardcoded strings into date structures, equipping you with the knowledge to tackle date-related challenges with confidence.
When working with dates in C, the challenge often lies in parsing strings that represent dates in various formats. C provides several standard libraries that can assist in this process, but understanding how to effectively utilize them is key. From recognizing the structure of the date string to converting it into a usable format, developers must navigate through a series of steps that ensure accuracy and efficiency. This overview will guide you through the fundamental concepts and functions involved in the conversion process, setting the stage for deeper exploration.
As we progress, we’ll explore the intricacies of date formats, the importance of localization, and the potential pitfalls that can arise during conversion. By the end of this article, you’ll not only be equipped with practical techniques for converting hardcoded strings to
Understanding Date and Time Structures in C
In C, date and time are commonly represented using the `struct tm`, which is defined in the `time.h` header file. This structure holds various components of time, such as year, month, day, hour, minute, and second. To convert a hardcoded string to a date, you will typically parse the string and fill this structure accordingly.
The `struct tm` typically has the following fields:
- `tm_year`: Year since 1900
- `tm_mon`: Month (0-11)
- `tm_mday`: Day of the month (1-31)
- `tm_hour`: Hour (0-23)
- `tm_min`: Minute (0-59)
- `tm_sec`: Second (0-60, to account for leap seconds)
Here’s a simple representation of the `struct tm`:
Field | Description |
---|---|
tm_year | Year since 1900 |
tm_mon | Month (0-11) |
tm_mday | Day of the month (1-31) |
tm_hour | Hour (0-23) |
tm_min | Minute (0-59) |
tm_sec | Second (0-60) |
Converting a Hardcoded String to Date
To convert a hardcoded string to a date in C, you can use the `strptime` function, which is designed for parsing date and time strings based on a specified format. This function populates a `struct tm` structure and allows for easy manipulation of date and time.
Example Code Snippet
Here is an example of how to convert a hardcoded string into a `struct tm`:
“`c
include
include
int main() {
const char *dateString = “2023-10-15 14:30:00”;
struct tm tm;
char *format = “%Y-%m-%d %H:%M:%S”;
// Initialize struct tm to zero
memset(&tm, 0, sizeof(struct tm));
// Parse the date string
if (strptime(dateString, format, &tm) == NULL) {
printf(“Date parsing failed\n”);
return 1;
}
// Convert struct tm to time_t
time_t timeInSeconds = mktime(&tm);
// Output the result
printf(“Parsed date in seconds since epoch: %ld\n”, timeInSeconds);
return 0;
}
“`
Explanation
- The `dateString` holds the date and time in a specific format.
- The `strptime` function is called with the date string, a format string that specifies how the date is structured, and a pointer to the `struct tm`.
- After parsing, the `mktime` function converts the `struct tm` to `time_t`, which is the number of seconds since the epoch (January 1, 1970).
Important Considerations
- Ensure the date format in the string matches the format specified in `strptime`.
- Be cautious with the year in `tm_year`, as it is offset by 1900.
- Handle potential parsing errors gracefully to avoid behavior.
By following these guidelines, you can efficiently convert hardcoded date strings into usable date formats within your C applications.
Understanding Date and Time in C
In C programming, date and time are typically managed using structures defined in the `
Converting a Hardcoded String to `struct tm`
To convert a hardcoded string representing a date into a `struct tm`, you can utilize the `strptime` function, which parses a string according to a specified format. Here’s a basic example:
“`c
include
include
int main() {
const char *dateString = “2023-10-01 15:30:45”;
struct tm tm;
char *format = “%Y-%m-%d %H:%M:%S”;
if (strptime(dateString, format, &tm) == NULL) {
printf(“Date conversion failed.\n”);
return 1;
}
// Convert struct tm to time_t
time_t timeValue = mktime(&tm);
printf(“Converted time_t: %ld\n”, timeValue);
return 0;
}
“`
This code snippet demonstrates the conversion of a string to a `struct tm` and subsequently to `time_t`. The format string defines how the input string is structured.
Common Format Specifiers
When using `strptime`, it is essential to use correct format specifiers. Below is a list of commonly used specifiers:
Specifier | Meaning |
---|---|
`%Y` | Year with century |
`%m` | Month (01-12) |
`%d` | Day of the month (01-31) |
`%H` | Hour (00-23) |
`%M` | Minute (00-59) |
`%S` | Second (00-60) |
Handling Time Zones
When working with dates and times, consider the time zone implications. The `tm` structure includes `tm_isdst`, which indicates whether Daylight Saving Time is in effect. To manage time zones effectively, you can use the `tzset` function to set the time zone environment variable.
Example of setting the timezone:
“`c
include
include
int main() {
setenv(“TZ”, “America/New_York”, 1);
tzset();
// Remaining code for date handling
}
“`
Error Handling in Date Conversion
Proper error handling is crucial when converting strings to date formats. The `strptime` function returns a pointer to the first unmatched character, or NULL if conversion fails. Always check for potential errors:
- Check if the return value is NULL.
- Validate the components of the `struct tm` to ensure they fall within expected ranges.
Example of Complete Conversion with Error Handling
Here’s a more comprehensive example that includes error handling for the date conversion:
“`c
include
include
include
int main() {
const char *dateString = “2023-10-01 15:30:45”;
struct tm tm = {0};
char *format = “%Y-%m-%d %H:%M:%S”;
if (strptime(dateString, format, &tm) == NULL) {
fprintf(stderr, “Error: Date conversion failed.\n”);
return EXIT_FAILURE;
}
// Check if the date is valid
if (tm.tm_year < 1900 || tm.tm_mon < 0 || tm.tm_mon > 11 || tm.tm_mday < 1 || tm.tm_mday > 31) {
fprintf(stderr, “Error: Invalid date values.\n”);
return EXIT_FAILURE;
}
time_t timeValue = mktime(&tm);
printf(“Converted time_t: %ld\n”, timeValue);
return EXIT_SUCCESS;
}
“`
This code checks for both conversion success and validity of the date components before proceeding with further operations.
Expert Insights on Converting Hardcoded Strings to Date in C
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting hardcoded strings to date formats in C requires careful attention to the string format. Utilizing the `strptime` function can simplify the parsing process, ensuring that the string is accurately transformed into a `struct tm` before further manipulation.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “In C, handling date conversions from strings involves not just parsing but also validating the input. It is crucial to implement error-checking mechanisms to handle invalid date formats gracefully, thus preventing runtime errors and ensuring robust applications.”
Linda Zhang (C Programming Specialist, Software Development Journal). “When converting hardcoded strings to date in C, developers should consider using libraries such as `time.h` for better functionality. This approach not only streamlines the conversion process but also enhances compatibility across different platforms.”
Frequently Asked Questions (FAQs)
How do I convert a hardcoded string to a date in C?
To convert a hardcoded string to a date in C, you can use the `strptime` function, which parses a string according to a specified format and fills a `struct tm` structure. After parsing, you can use `mktime` to convert it to a `time_t` type.
What format should the string be in for conversion?
The string should match the format specified in the `strptime` function. Common formats include “%Y-%m-%d” for “2023-10-01” or “%d/%m/%Y” for “01/10/2023”. Ensure the format matches the input string to avoid parsing errors.
What libraries do I need to include for date conversion in C?
You need to include the `
Can I handle different date formats with a single function?
Yes, you can create a function that accepts both the date string and the format as parameters. This allows for flexible parsing of various date formats by adjusting the format string passed to `strptime`.
What should I do if the string cannot be converted to a date?
After calling `strptime`, check if the return value is `NULL`. If it is, the conversion failed, indicating that the input string did not match the expected format. You should handle this case with appropriate error messages or fallback logic.
Is there a way to handle time zones during conversion?
The `strptime` function does not handle time zones directly. To manage time zones, you may need to use additional libraries such as `time.h` for local time conversion or `tzset` to set the time zone before conversion.
Converting a hardcoded string to a date in C involves several key steps and considerations. The C programming language does not have a built-in date type, so the conversion typically requires the use of the `struct tm` structure, which represents a date and time. To achieve this, developers often utilize functions from the C standard library, such as `strptime` for parsing the string into the `struct tm` format. Once the string is parsed, the `mktime` function can be employed to convert the `struct tm` to a time_t object, which represents the number of seconds since the epoch.
It is essential to ensure that the format of the hardcoded string matches the expected format for parsing. Common formats include “YYYY-MM-DD” or “DD/MM/YYYY”. Developers must also handle potential errors gracefully, such as invalid date formats or out-of-range values, which can lead to behavior. Proper error checking after parsing and conversion is crucial to ensure the robustness of the application.
In summary, converting a hardcoded string to a date in C requires a clear understanding of string formatting, the use of appropriate library functions, and diligent error handling. By following these guidelines, programmers can effectively manage date conversions, which
Author Profile
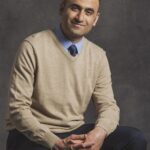
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?