How Can You Convert a java.lang.String to a JSON Object?
In the ever-evolving landscape of software development, the ability to seamlessly transition between different data formats is paramount. One common challenge developers face is converting a `java.lang.String`—often representing structured data—into a JSON object. As JSON (JavaScript Object Notation) has become the de facto standard for data interchange, understanding how to manipulate strings to create valid JSON structures is an essential skill for any Java programmer. Whether you’re working with APIs, configuring data for web applications, or simply managing data serialization, mastering this conversion process can significantly streamline your development workflow.
At its core, converting a Java string to a JSON object involves parsing the string to ensure it adheres to JSON syntax, which includes proper formatting and the correct use of data types. This process not only enhances data readability but also facilitates easier data manipulation and transfer between systems. With Java’s robust libraries and frameworks, developers have a variety of tools at their disposal to perform this conversion efficiently.
As we delve deeper into this topic, we will explore the various methods and libraries available in Java that can assist in transforming strings into JSON objects. From the popular Gson and Jackson libraries to native approaches, understanding these techniques will empower you to handle JSON data with confidence and precision. Whether you’re a seasoned developer or just starting your
Understanding JSON Objects
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. In Java, converting a `java.lang.String` representation of a JSON object into an actual JSON object can be accomplished using libraries such as Jackson or Gson.
When converting a `String` to a JSON object, it is important to ensure that the `String` is properly formatted as valid JSON. Invalid formatting will lead to parsing errors.
Using Jackson for Conversion
Jackson is a popular library for processing JSON in Java. To convert a `String` to a JSON object using Jackson, follow these steps:
- Include the Jackson dependency in your project (Maven example):
“`xml
“`
- Use the `ObjectMapper` class to parse the `String`:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
String jsonString = “{\”key\”:\”value\”}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
“`
This code snippet takes a JSON formatted `String` and converts it into a `JsonNode`, which is a Jackson representation of a JSON object.
Using Gson for Conversion
Gson is another widely used library for converting Java objects to JSON and vice versa. Here’s how to convert a `String` to a JSON object using Gson:
- Add the Gson dependency to your project (Maven example):
“`xml
“`
- Use the `Gson` class to convert the `String`:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
String jsonString = “{\”key\”:\”value\”}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
“`
This will parse the `String` and produce a `JsonObject` that can be used for further processing.
Common Pitfalls
When converting a `String` to a JSON object, developers should be aware of the following common pitfalls:
- Invalid JSON Format: Ensure that the `String` adheres to JSON standards. Common issues include:
- Missing quotes around keys or string values.
- Trailing commas after the last element in an object or array.
- Handling Exceptions: Always handle exceptions such as `JsonParseException` or `IOException` that may arise during parsing.
- Data Type Mismatch: Be cautious about the data types in the JSON. Ensure that the expected data types in Java match those in the JSON structure.
Library | Dependency | Conversion Method |
---|---|---|
Jackson | com.fasterxml.jackson.core:jackson-databind | ObjectMapper.readTree() |
Gson | com.google.code.gson:gson | JsonParser.parseString() |
By utilizing either Jackson or Gson, developers can seamlessly convert a `java.lang.String` representation of a JSON object into a usable JSON object in Java, enabling efficient data manipulation and interaction.
Understanding JSON Conversion in Java
To convert a `java.lang.String` to a JSON object in Java, the most commonly used libraries are Gson and Jackson. Both libraries provide straightforward methods to achieve this, facilitating the manipulation and storage of JSON data.
Using Gson for Conversion
Gson is a popular Java library developed by Google. It allows for easy conversion between Java objects and JSON format.
- Adding Gson Dependency: If you are using Maven, add the following dependency to your `pom.xml`:
“`xml
“`
- Conversion Example:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class StringToJsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
This example demonstrates how to parse a JSON string into a `JsonObject` using Gson.
Using Jackson for Conversion
Jackson is another powerful library for processing JSON in Java, known for its speed and extensive features.
- Adding Jackson Dependency: Include the following in your `pom.xml` for Maven:
“`xml
“`
- Conversion Example:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class StringToJsonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
In this case, the `ObjectMapper` reads the JSON string and converts it into a `JsonNode` object.
Key Differences Between Gson and Jackson
Feature | Gson | Jackson |
---|---|---|
Performance | Generally slower | Faster, especially with larger datasets |
Streaming API | Limited streaming support | Excellent streaming support |
Annotations | Fewer annotations | Rich set of annotations |
Data Binding | Simpler data binding | More complex but flexible data binding |
Common Use Cases
- Data Exchange: Sending and receiving JSON objects over APIs.
- Configuration Files: Storing application configurations in JSON format.
- Data Serialization: Converting Java objects to JSON for storage or transmission.
By utilizing either Gson or Jackson, developers can efficiently convert strings to JSON objects, enhancing their applications’ data handling capabilities.
Expert Insights on Converting Java Strings to JSON Objects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To convert a `java.lang.String` to a JSON object in Java, one can utilize libraries such as Jackson or Gson. These libraries provide straightforward methods to parse the string and transform it into a JSON object, ensuring efficient handling of data structures.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “Using the Gson library is particularly advantageous for this conversion. By leveraging the `fromJson` method, developers can easily convert a well-formed JSON string into a corresponding Java object, thus streamlining data manipulation in applications.”
Sarah Patel (Technical Architect, FutureTech Labs). “When converting a string to a JSON object, it is crucial to ensure that the string is valid JSON. Tools like JSONLint can assist in validating the string before conversion, preventing runtime errors and enhancing application stability.”
Frequently Asked Questions (FAQs)
What is the process to convert a `java.lang.String` to a JSON object?
To convert a `java.lang.String` to a JSON object in Java, you can use libraries such as Gson or Jackson. For Gson, use `new Gson().fromJson(jsonString, JsonObject.class)`, and for Jackson, use `new ObjectMapper().readTree(jsonString)`.
What libraries are commonly used for JSON processing in Java?
Common libraries for JSON processing in Java include Gson, Jackson, and org.json. Each library offers different features and methods for parsing and converting JSON data.
Can I convert a malformed JSON string to a JSON object?
No, a malformed JSON string cannot be converted to a JSON object. You must ensure that the JSON string is properly formatted according to JSON syntax rules before conversion.
What exceptions should I handle when converting a string to a JSON object?
When converting a string to a JSON object, handle exceptions such as `JsonSyntaxException` (for Gson) or `JsonProcessingException` (for Jackson). These exceptions indicate issues with the JSON format.
Is there a performance difference between using Gson and Jackson for JSON conversion?
Yes, there can be performance differences between Gson and Jackson. Jackson is generally faster and more efficient for large data sets, while Gson is simpler and easier to use for smaller tasks.
How can I validate a JSON string before converting it to a JSON object?
To validate a JSON string, you can use a JSON schema validator or simply attempt to parse it using a library like Gson or Jackson. If parsing fails, the string is not valid JSON.
Converting a `java.lang.String` to a JSON object is a common task in Java programming, especially when dealing with APIs or data interchange formats. The process typically involves using libraries such as Jackson or Gson, which provide convenient methods to parse strings formatted as JSON into Java objects. Understanding the structure of the JSON string is crucial, as it dictates how the conversion will be handled and what data types will be expected in the resulting object.
One of the key insights is the importance of error handling during the conversion process. JSON parsing can fail due to various reasons, such as malformed strings or unexpected data types. Implementing robust exception handling mechanisms ensures that your application can gracefully manage such errors, providing users with meaningful feedback rather than crashing unexpectedly.
Additionally, it is essential to consider the performance implications of JSON parsing, especially when dealing with large datasets or frequent conversions. Optimizing the conversion process by reusing object mappers or minimizing the number of conversions can lead to significant performance improvements. By leveraging the features of libraries like Jackson and Gson, developers can streamline their code and enhance overall application efficiency.
Author Profile
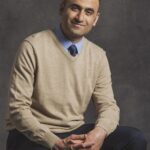
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?