How Can You Convert a Jupyter Notebook to a Python (.py) File?
Are you ready to unlock the full potential of your Jupyter notebooks? Whether you’re a data scientist, a researcher, or a student, the ability to convert Jupyter notebooks into Python scripts can significantly enhance your workflow. This transformation not only allows for easier sharing and version control but also opens the door to integrating your code into larger projects or applications. In this article, we will explore the ins and outs of converting Jupyter notebooks to Python files, ensuring you have the tools and knowledge to streamline your coding experience.
Jupyter notebooks are a powerful tool for interactive computing, combining code, visualizations, and narrative text in a single document. However, there are times when you may want to extract just the code for further development or collaboration. Converting a Jupyter notebook to a Python script simplifies this process, enabling you to focus on the code without the overhead of the notebook interface. This conversion can be particularly beneficial for those looking to refine their code, debug more effectively, or integrate it into a larger software project.
In this article, we will delve into various methods for achieving this conversion, highlighting the advantages and potential challenges you may encounter along the way. By the end, you’ll be equipped with the knowledge to seamlessly transition from a notebook environment to a more traditional coding framework, enhancing
Methods to Convert Jupyter Notebook to Python (.py) Files
To convert a Jupyter Notebook (.ipynb) into a Python script (.py), there are several methods you can utilize. Each approach may suit different workflows or preferences. Below are the most common techniques.
Using Jupyter Notebook Interface
Jupyter Notebooks come equipped with a built-in feature that allows you to export your notebook directly to a Python script. To do this:
- Open your Jupyter Notebook.
- Navigate to the “File” menu.
- Select “Download as”.
- Choose “Python (.py)”.
This method generates a .py file containing all the code cells from your notebook, maintaining the order in which they appear.
Using nbconvert
Another powerful way to convert Jupyter Notebooks is by using the `nbconvert` command-line tool. This method is particularly useful for batch processing or automation:
- Open your terminal or command prompt.
- Run the following command:
“`
jupyter nbconvert –to script your_notebook_name.ipynb
“`
This command converts the specified notebook into a .py file, which will be saved in the same directory as the original notebook.
Using Python Script
You can also programmatically convert a Jupyter Notebook to a .py file by using Python code. This method is beneficial for automation within larger Python scripts:
“`python
import nbformat
from nbconvert import PythonExporter
Load the notebook
with open(‘your_notebook_name.ipynb’, ‘r’, encoding=’utf-8′) as f:
notebook_content = nbformat.read(f, as_version=4)
Convert to Python script
exporter = PythonExporter()
script, _ = exporter.from_notebook_node(notebook_content)
Save the script
with open(‘your_script_name.py’, ‘w’, encoding=’utf-8′) as f:
f.write(script)
“`
This script loads a Jupyter Notebook, converts it to a Python script, and then saves it.
Considerations During Conversion
When converting notebooks, it is important to consider the following aspects to ensure the integrity and readability of the resulting Python file:
- Comments and Markdown: Markdown cells will be converted into comments within the .py file. Ensure that essential explanations are preserved.
- Cell Execution Order: The order of code execution in the notebook should be maintained in the script. Review the sequence to avoid logical errors.
- Output Cells: Output from code cells (like plots or printed statements) will not be included in the .py file.
Comparison of Conversion Methods
Method | Pros | Cons |
---|---|---|
Jupyter Notebook Interface | Simple and user-friendly | Manual process for each notebook |
nbconvert | Can process multiple notebooks in batch | Requires command line usage |
Python Script | Highly customizable and automatable | Requires coding knowledge |
By selecting the appropriate method based on your needs and familiarity with the tools, you can efficiently convert Jupyter Notebooks to Python scripts.
Methods to Convert Jupyter Notebook to Python Script
Converting a Jupyter Notebook (.ipynb) into a Python script (.py) can be accomplished using several methods. Below are the primary techniques available.
Using Jupyter Notebook Interface
- Open the Jupyter Notebook you wish to convert.
- Navigate to the “File” menu at the top left corner.
- Select “Download as” from the dropdown.
- Click on “Python (.py)”.
This method will download the notebook as a Python script directly to your local machine.
Using Command Line Interface
The command line provides an efficient way to convert Jupyter notebooks without opening the interface. You can use the `nbconvert` command:
“`bash
jupyter nbconvert –to script your_notebook.ipynb
“`
- Replace `your_notebook.ipynb` with the actual filename.
- This command generates a .py file in the same directory as the notebook.
Using Python Code
If you prefer to convert the notebook programmatically, you can use the following code snippet within a Python environment:
“`python
import nbformat
from nbconvert import PythonExporter
Load the notebook
with open(‘your_notebook.ipynb’) as f:
notebook = nbformat.read(f, as_version=4)
Convert to Python
exporter = PythonExporter()
source, _ = exporter.from_notebook_node(notebook)
Save to .py file
with open(‘your_notebook.py’, ‘w’) as f:
f.write(source)
“`
This method allows for more flexibility and integration within your Python scripts.
Using Third-Party Tools
Several third-party tools and libraries can assist in converting Jupyter Notebooks to Python scripts:
- JupyterLab: Similar to Jupyter Notebook, JupyterLab allows exporting notebooks directly.
- nbconvert: A robust tool for converting notebooks to various formats, including Python scripts.
- Papermill: Allows parameterization of notebooks and can output Python scripts.
Tool | Description |
---|---|
JupyterLab | GUI for Jupyter notebooks supporting export options |
nbconvert | Command-line tool for converting notebooks to scripts |
Papermill | Framework for parameterized notebook execution |
Considerations After Conversion
After converting a Jupyter Notebook to a Python script, ensure the following:
- Imports: Verify all necessary imports are present in the generated script.
- Markdown Cells: Text from markdown cells will be converted to comments; review for clarity.
- Cell Execution Order: The execution order may differ; test the script to ensure functionality.
- Dependencies: Ensure all libraries used in the notebook are installed in your Python environment.
These considerations will help maintain the integrity of your code and ensure smooth execution post-conversion.
Expert Insights on Converting Jupyter Notebooks to Python Scripts
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Converting Jupyter notebooks to Python scripts is essential for streamlining workflows. It allows for better version control and integration into larger software projects, enhancing collaboration among data teams.”
Michael Chen (Software Engineer, Open Source Advocate). “Utilizing tools like `nbconvert` can simplify the process of converting Jupyter notebooks to `.py` files. This not only preserves the code but also ensures that documentation and comments are retained, which is crucial for maintaining code readability.”
Sarah Patel (Educational Technology Specialist, LearnTech Solutions). “For educators, converting notebooks to Python scripts can facilitate the sharing of learning materials. It allows students to access clean, executable code without the additional overhead of notebook interfaces, promoting a more focused coding experience.”
Frequently Asked Questions (FAQs)
How can I convert a Jupyter Notebook to a Python (.py) file?
You can convert a Jupyter Notebook to a Python file by using the command line. Navigate to the directory containing your notebook and run the command `jupyter nbconvert –to script your_notebook.ipynb`. This will generate a `.py` file in the same directory.
Is there a way to convert Jupyter Notebooks to Python files directly from the Jupyter interface?
Yes, you can convert a Jupyter Notebook to a Python file directly from the Jupyter interface. Open the notebook, click on “File” in the menu, select “Download as,” and then choose “Python (.py).” This will download the notebook as a Python script.
What is the output format when converting a Jupyter Notebook to a Python file?
The output format is a plain text file with a `.py` extension. The code cells from the notebook will be converted into Python code, while markdown cells will be converted into comments in the script.
Can I automate the conversion of multiple Jupyter Notebooks to Python files?
Yes, you can automate the conversion by using a script that loops through all notebooks in a directory and calls the `nbconvert` command for each one. This can be done using Python or shell scripting.
Are there any limitations when converting Jupyter Notebooks to Python files?
Yes, certain features such as interactive widgets, plots, and rich media content may not be preserved in the Python script. The conversion primarily focuses on the code and markdown content.
Do I need to install any additional packages to convert Jupyter Notebooks to Python files?
Typically, no additional packages are required if you have Jupyter installed. However, ensure that `nbconvert` is included in your Jupyter installation, which is usually the case in standard installations.
Converting a Jupyter Notebook to a Python (.py) file is a straightforward process that can significantly streamline your workflow, particularly when transitioning from an exploratory coding environment to a more traditional programming setup. This conversion allows users to easily share code, integrate it into larger projects, or utilize version control systems more effectively. The process typically involves using built-in Jupyter commands or utilizing tools such as nbconvert, which facilitate the transformation of notebooks into scripts.
One of the key takeaways from this discussion is the importance of understanding the structure of Jupyter Notebooks. Notebooks contain not only code but also rich text elements, visualizations, and outputs. When converting to a .py file, it is essential to recognize that only the code cells will be preserved in the script. This means that any markdown or output will not be included, which may require additional documentation or comments in the Python file to maintain clarity and context for future users or collaborators.
Additionally, users should be aware of the potential need for modifications post-conversion. The resulting .py file may require adjustments to ensure that it runs smoothly outside the notebook environment. This includes addressing any dependencies, ensuring proper imports, and potentially refactoring code to enhance readability and maintainability. Overall
Author Profile
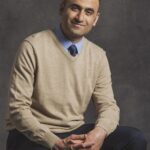
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?