How Can You Convert a Long to an Integer in Java?
In the world of programming, data types play a crucial role in determining how information is stored and manipulated. Java, a versatile and widely-used programming language, offers a variety of data types, including long and integer. While both are integral to handling numerical data, their differences can sometimes lead to confusion, especially when it comes to converting between them. Whether you’re a seasoned developer or a newcomer to Java, understanding how to effectively convert a long to an integer is essential for optimizing your code and ensuring data integrity.
When working with numerical values, the long data type is often used to store larger numbers that exceed the limits of the integer type. However, there are scenarios where you may need to convert a long to an integer, particularly when interfacing with APIs or libraries that require integer inputs. This conversion process, while straightforward, requires careful consideration of potential pitfalls, such as data loss or overflow, which can occur if the long value exceeds the range of the integer type.
In this article, we will explore the various methods available for converting long to integer in Java, highlighting best practices and common mistakes to avoid. By the end, you will have a solid understanding of how to perform this conversion safely and efficiently, empowering you to write cleaner, more reliable code in your Java applications. Get
Converting Long to Integer in Java
In Java, the `long` data type is a 64-bit signed integer, while the `int` data type is a 32-bit signed integer. When converting a `long` to an `int`, it’s essential to be aware of the potential for data loss if the `long` value exceeds the range that an `int` can represent. The range for `int` is from -2,147,483,648 to 2,147,483,647. If the `long` value falls outside this range, the result of the conversion will be incorrect.
To convert a `long` to an `int`, you can use a simple type cast. Here’s how this can be done in Java:
“`java
long longValue = 123456789L;
int intValue = (int) longValue;
“`
However, if you’re unsure whether the `long` value is within the `int` range, you should perform a check before casting:
“`java
if (longValue >= Integer.MIN_VALUE && longValue <= Integer.MAX_VALUE) {
int intValue = (int) longValue;
} else {
// Handle the case where longValue is out of int range
}
```
Potential Issues During Conversion
When converting a `long` to an `int`, there are specific issues to be aware of:
- Data Loss: If the `long` value exceeds the bounds of an `int`, the conversion will result in a truncated value.
- Negative Values: Care should be taken when dealing with negative `long` values to ensure that the conversion remains valid.
- Performance: While casting is generally efficient, excessive conversions in performance-critical applications can introduce overhead.
To illustrate the potential data loss, consider the following table:
Long Value | Converted Int Value |
---|---|
2147483648 | -2147483648 |
123456789 | 123456789 |
-2147483649 | 2147483647 |
As shown in the table, converting a `long` value that exceeds the limits of `int` can lead to unexpected results, demonstrating the importance of checking the range before performing the conversion.
Best Practices for Conversion
To ensure safe and effective conversion from `long` to `int`, consider the following best practices:
- Range Checking: Always verify that the `long` value is within the acceptable range of `int` before casting.
- Use of Wrapper Classes: Consider using `Long` and `Integer` wrapper classes for additional utility methods that might assist in conversion and handling.
- Error Handling: Implement error handling to manage cases where the conversion may not yield a valid result.
By adhering to these practices, developers can effectively manage the conversion process and minimize the risk of data loss or unexpected behavior in their applications.
Methods to Convert Long to Integer in Java
In Java, converting a `long` to an `int` can be accomplished using several methods. It’s essential to be aware that this conversion may lead to data loss if the `long` value exceeds the range of an `int`. The `int` type can hold values from -2,147,483,648 to 2,147,483,647.
Using Explicit Casting
The simplest way to convert a `long` to an `int` is through explicit casting. This method directly instructs the Java compiler to convert the `long` type to `int`.
“`java
long longValue = 100L;
int intValue = (int) longValue;
“`
- Caution: If `longValue` is larger than `Integer.MAX_VALUE` or smaller than `Integer.MIN_VALUE`, the resultant `intValue` will not represent the original `longValue` accurately.
Using the `Math.toIntExact()` Method
Java 8 introduced the `Math.toIntExact(long value)` method, which converts a `long` to an `int`. This method throws an `ArithmeticException` if the conversion results in overflow.
“`java
long longValue = 200L;
int intValue = Math.toIntExact(longValue);
“`
- Advantages: This method ensures that the value fits within the `int` range, providing a safer conversion.
Handling Data Loss
When converting a `long` to an `int`, it is crucial to handle potential data loss. Consider the following strategies:
– **Check Range Before Conversion**:
“`java
long longValue = 3000000000L; // Example long value
if (longValue >= Integer.MIN_VALUE && longValue <= Integer.MAX_VALUE) {
int intValue = (int) longValue;
} else {
// Handle the overflow scenario
}
```
- Use Try-Catch for Exception Handling:
“`java
try {
int intValue = Math.toIntExact(longValue);
} catch (ArithmeticException e) {
// Handle overflow exception
}
“`
Comparison of Methods
Method | Description | Exception Handling |
---|---|---|
Explicit Casting | Directly casts `long` to `int`. | No, potential data loss. |
`Math.toIntExact()` | Converts `long` to `int` with overflow check. | Yes, throws `ArithmeticException`. |
Choosing the appropriate method for converting a `long` to an `int` depends on the specific requirements of your application, particularly regarding data integrity and overflow management. Use explicit casting for simple cases but prefer `Math.toIntExact()` when you need to ensure the validity of the conversion.
Expert Insights on Converting Long to Integer in Java
Dr. Emily Carter (Senior Software Engineer, Java Innovations Inc.). “When converting a long to an integer in Java, it is crucial to be aware of potential data loss, as the long type can hold significantly larger values than an integer. Always validate the range before performing the conversion to avoid unexpected behavior.”
Michael Tran (Java Developer, Tech Solutions Group). “Utilizing explicit casting is the standard approach for converting long to integer in Java. However, developers should consider using methods like Math.toIntExact() for safer conversions, which throw an exception if the long value exceeds the integer range.”
Sarah Johnson (Lead Java Architect, CodeCraft Labs). “In performance-critical applications, the conversion from long to integer should be optimized. Avoid unnecessary conversions within loops and consider the implications of using boxed types versus primitive types to maintain efficiency.”
Frequently Asked Questions (FAQs)
How can I convert a long to an integer in Java?
You can convert a long to an integer in Java using explicit casting. For example: `int intValue = (int) longValue;`. This method truncates the long value to fit into an integer.
What happens if the long value exceeds the integer range?
If the long value exceeds the integer range (i.e., less than Integer.MIN_VALUE or greater than Integer.MAX_VALUE), the result will wrap around, leading to unexpected values due to overflow.
Is there a built-in method to convert long to integer in Java?
Java does not provide a built-in method specifically for converting long to integer. The conversion must be done using casting or by using the `Long.intValue()` method on a Long object.
What is the difference between casting and using Long.intValue()?
Casting directly converts the primitive long type to int, while `Long.intValue()` is a method that converts a Long object to an int. Both approaches yield the same result but differ in their usage context.
Can I safely convert a long to an integer without losing data?
You cannot guarantee safe conversion without potential data loss if the long value is outside the integer range. Always check the value before conversion to avoid truncation or overflow issues.
What should I do if I need to handle large numbers in Java?
If you need to handle large numbers, consider using the `BigInteger` class, which can represent integers of arbitrary size, thereby avoiding overflow issues associated with primitive types.
In Java, converting a long to an integer is a straightforward process, but it requires careful consideration due to the potential loss of data. The long data type can store values ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, while the integer type is limited to -2,147,483,648 to 2,147,483,647. Therefore, when performing this conversion, it is crucial to ensure that the long value falls within the integer range to avoid overflow and unintended results.
The conversion can be performed using a simple cast, as shown in the syntax: `int intValue = (int) longValue;`. However, if the long value exceeds the integer limits, the resulting integer may not represent the original long value accurately. To handle such cases, developers should implement checks to verify that the long value is within the acceptable range before conversion. This practice helps maintain data integrity and prevents runtime errors.
In summary, while converting a long to an integer in Java is technically simple, it necessitates a cautious approach to avoid data loss. Developers should always consider the range of the values being converted and implement necessary checks to
Author Profile
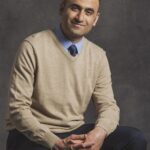
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?