How Can You Convert a String to an Integer in Go (Golang)?
In the world of programming, data types play a crucial role in how we manipulate and interact with information. Among these types, strings and integers are fundamental, each serving distinct purposes in the realm of software development. For developers working with Go, commonly known as Golang, the need to convert a string representation of a number into an integer is a frequent task. Whether you’re parsing user input, reading from a file, or processing data from an API, understanding how to seamlessly transition between these data types is essential for building efficient and robust applications.
Converting a string to an integer in Go might seem straightforward, but it involves more than just a simple cast. The Go programming language provides built-in functions that handle this conversion, ensuring that developers can manage potential errors and edge cases effectively. This process not only enhances the reliability of your code but also helps in maintaining clarity and readability, which are hallmarks of Go’s design philosophy.
As we delve deeper into this topic, we will explore the various methods available for converting strings to integers in Go, including best practices and common pitfalls to avoid. Whether you’re a seasoned Go developer or just starting your journey, mastering this conversion will empower you to handle data more adeptly and elevate your programming skills to new heights.
Converting String to Integer in Go
In Go, converting a string to an integer can be accomplished using the `strconv` package, which provides various functions for string conversions. The most commonly used function for this purpose is `strconv.Atoi`, which stands for “ASCII to integer”.
To use `strconv.Atoi`, follow this syntax:
“`go
import “strconv”
value, err := strconv.Atoi(“123”)
“`
Here, `value` will hold the converted integer, while `err` will capture any errors that occur during the conversion. If the string cannot be converted (for example, if it contains non-numeric characters), `err` will not be `nil`, and you should handle this accordingly.
Handling Errors
When converting strings to integers, it is crucial to handle potential errors. The conversion might fail for various reasons, including:
- The string is empty.
- The string contains non-numeric characters.
- The integer value exceeds the range of an `int`.
Here’s an example of how to handle errors during conversion:
“`go
value, err := strconv.Atoi(“abc”)
if err != nil {
fmt.Println(“Error converting string to int:”, err)
} else {
fmt.Println(“Converted value:”, value)
}
“`
Alternative Methods
Apart from `strconv.Atoi`, Go offers other functions in the `strconv` package that can be used for more specific conversion needs:
- `strconv.ParseInt`: Converts a string to an `int64` with a specified base.
- `strconv.ParseUint`: Converts a string to an `uint64` with a specified base.
Here’s an example using `strconv.ParseInt`:
“`go
value, err := strconv.ParseInt(“123”, 10, 0) // base 10, bit size 0 (int)
“`
This function allows for more control over the conversion process. The parameters are:
- The string to convert.
- The base for the conversion (e.g., 10 for decimal).
- The bit size (0 means it will return an int).
Performance Considerations
When converting strings to integers in performance-sensitive applications, consider the following:
- Avoid unnecessary conversions: If you know the data type ahead of time, avoid repeated conversions.
- Batch processing: If converting a large number of strings, consider processing them in batches to minimize overhead.
Here’s a quick comparison of the two functions:
Function | Returns | Base | Usage |
---|---|---|---|
strconv.Atoi | int | Base 10 | Basic conversion |
strconv.ParseInt | int64 | Custom | More control over conversion |
These considerations will help ensure that string to integer conversions in Go are both effective and efficient.
Converting String to Integer in Go
In Go, converting a string to an integer can be accomplished using the `strconv` package, which provides various functions for string conversions. The most commonly used function for this purpose is `strconv.Atoi`.
Using strconv.Atoi
The `strconv.Atoi` function converts a string to an `int`. It returns the converted integer and an error if the conversion fails.
Example:
“`go
package main
import (
“fmt”
“strconv”
)
func main() {
str := “123”
num, err := strconv.Atoi(str)
if err != nil {
fmt.Println(“Error:”, err)
} else {
fmt.Println(“Converted integer:”, num)
}
}
“`
Key Points:
- `strconv.Atoi` takes a single string argument.
- It returns two values: the converted integer and an error.
- If the string cannot be converted, the error will indicate the reason.
Handling Different Integer Types
If you need to convert a string to other integer types, such as `int64` or `uint`, you can use `strconv.ParseInt` and `strconv.ParseUint` respectively.
Using strconv.ParseInt:
The `strconv.ParseInt` function allows you to specify the base of the number system (e.g., base 10 for decimal).
Example:
“`go
package main
import (
“fmt”
“strconv”
)
func main() {
str := “123456789”
num, err := strconv.ParseInt(str, 10, 64) // base 10, bit size 64
if err != nil {
fmt.Println(“Error:”, err)
} else {
fmt.Println(“Converted int64:”, num)
}
}
“`
Using strconv.ParseUint:
For converting strings to unsigned integers, `strconv.ParseUint` can be used in a similar manner.
Example:
“`go
package main
import (
“fmt”
“strconv”
)
func main() {
str := “123456789”
num, err := strconv.ParseUint(str, 10, 64) // base 10, bit size 64
if err != nil {
fmt.Println(“Error:”, err)
} else {
fmt.Println(“Converted uint64:”, num)
}
}
“`
Common Errors and Edge Cases
When converting strings to integers, several common errors may occur:
- Invalid Format: If the string contains non-numeric characters, the conversion will fail.
- Out of Range: The resulting integer may exceed the limits of the target type, causing an error.
- Empty String: An empty string will also lead to an error during conversion.
Error Handling:
Always check for errors after conversion to handle these scenarios gracefully.
Performance Considerations
While string to integer conversions in Go are generally efficient, consider the following when dealing with large volumes of data:
- Batch Processing: If converting many strings, process them in batches to minimize overhead.
- Avoiding Repeated Conversions: Store converted values if the same strings are used multiple times to reduce unnecessary conversions.
Utilizing the appropriate functions from the `strconv` package ensures effective and robust string-to-integer conversions in Go applications.
Expert Insights on Converting Strings to Integers in Golang
Dr. Emily Carter (Senior Software Engineer, GoLang Innovations). “In Go, converting a string to an integer is straightforward using the `strconv` package. The `Atoi` function is commonly employed for this purpose, but developers must handle potential errors effectively to ensure robust applications.”
Mark Thompson (Technical Lead, Cloud Solutions Inc.). “When converting strings to integers in Go, it is crucial to validate the input string beforehand. This can prevent runtime errors and ensure that your application behaves predictably, especially when dealing with user inputs.”
Linda Zhang (Go Language Specialist, Tech Insights). “Utilizing `strconv.Atoi` is not only efficient but also idiomatic in Go. However, developers should be aware of the implications of using `ParseInt` for more control over base conversions and error handling, especially in complex applications.”
Frequently Asked Questions (FAQs)
How can I convert a string to an integer in Go?
You can convert a string to an integer in Go using the `strconv.Atoi` function, which parses a string and returns the corresponding integer value.
What package do I need to import for string conversion in Go?
You need to import the `strconv` package to access the functions required for converting strings to integers.
What happens if the string cannot be converted to an integer?
If the string cannot be converted to an integer, `strconv.Atoi` will return an error indicating that the conversion failed. Always check for errors to handle such cases appropriately.
Can I convert a string with non-numeric characters to an integer?
No, attempting to convert a string with non-numeric characters will result in an error. The string must represent a valid integer format.
Is there a way to specify the base when converting a string to an integer?
Yes, you can use `strconv.ParseInt` to specify the base for conversion. This function allows you to define the base (e.g., base 10 for decimal) along with the bit size.
What is the difference between `strconv.Atoi` and `strconv.ParseInt`?
`strconv.Atoi` is a convenience function that converts a string to an int, assuming base 10. In contrast, `strconv.ParseInt` provides more flexibility, allowing you to specify the base and the bit size of the resulting integer.
In Go (Golang), converting a string to an integer is a straightforward process that can be accomplished using the `strconv` package, specifically the `strconv.Atoi` function. This function takes a string as input and returns the corresponding integer value along with an error value. If the string is not a valid representation of an integer, the function will return an error, which is essential for robust error handling in applications. Understanding this conversion process is crucial for developers working with user input or data parsing.
Another method to convert strings to integers in Go is by using `strconv.ParseInt`, which provides additional flexibility by allowing developers to specify the base of the number system and the bit size of the resulting integer. This method is particularly useful when dealing with strings that represent numbers in different bases, such as binary or hexadecimal. Both methods emphasize the importance of error checking to ensure that the conversion is successful and that the program can handle unexpected input gracefully.
In summary, converting strings to integers in Go involves utilizing the `strconv` package, with `strconv.Atoi` and `strconv.ParseInt` being the primary functions for this task. Developers should always be mindful of error handling when performing these conversions to maintain the integrity of their applications. By mastering these
Author Profile
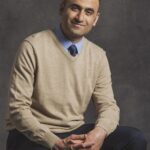
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?