How Can I Convert a String to JSON in Java?
In today’s data-driven world, the ability to seamlessly convert strings to JSON (JavaScript Object Notation) in Java is an essential skill for developers. JSON has become the de facto standard for data interchange due to its lightweight nature and ease of use. Whether you’re working on web applications, APIs, or mobile apps, understanding how to manipulate JSON data is crucial for effective communication between systems. This article will guide you through the process of converting strings to JSON in Java, unlocking the potential for efficient data handling and integration.
At its core, converting a string to JSON involves parsing the string representation of data into a structured format that Java can understand and manipulate. This process is vital for applications that need to read, write, or transmit data in a standardized format. Java provides several libraries that facilitate this conversion, making it easier for developers to work with JSON data without getting bogged down in the complexities of manual parsing.
In the following sections, we will explore the various methods and libraries available for converting strings to JSON in Java. From popular libraries like Jackson and Gson to built-in capabilities, you’ll discover how to choose the right approach for your specific needs. Whether you’re a seasoned Java developer or just starting your journey, mastering this skill will enhance your ability to work with modern data formats and improve
Using Jackson Library
One of the most popular libraries for converting strings to JSON in Java is the Jackson library. This library provides a straightforward way to handle JSON data, offering features for parsing, generating, and manipulating JSON. To utilize Jackson for converting a string to JSON, follow these steps:
- Add Jackson Dependency: Ensure you have the Jackson library included in your project. If you’re using Maven, add the following dependency to your `pom.xml`:
“`xml
“`
- Convert String to JSON: You can convert a string representation of JSON into a JSON object using the `ObjectMapper` class. Here’s a simple example:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
Person person = objectMapper.readValue(jsonString, Person.class);
System.out.println(person);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class Person {
private String name;
private int age;
// Getters and setters
}
“`
In this example, the `readValue` method of `ObjectMapper` is used to deserialize the JSON string into a `Person` object.
Using Gson Library
Another widely used library for JSON handling in Java is Google’s Gson. Gson is lightweight and easy to use for converting strings to JSON objects. To use Gson, follow these steps:
- Add Gson Dependency: Include Gson in your project. For Maven, add this dependency:
“`xml
“`
- Convert String to JSON: You can convert a JSON string to a Java object using Gson’s `fromJson` method. Here is an example:
“`java
import com.google.gson.Gson;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”Jane\”, \”age\”:25}”;
Gson gson = new Gson();
Person person = gson.fromJson(jsonString, Person.class);
System.out.println(person);
}
}
class Person {
private String name;
private int age;
// Getters and setters
}
“`
Gson automatically maps the JSON string to the specified class, allowing easy access to its fields.
Comparison of Libraries
When choosing between Jackson and Gson, consider the following factors:
Feature | Jackson | Gson |
---|---|---|
Performance | Generally faster for large datasets | Good performance, but slower than Jackson for big data |
Flexibility | Highly customizable with annotations | Less flexible, straightforward mappings |
Ease of Use | Requires understanding of more features | Simple and easy to use |
Both libraries are powerful tools for working with JSON in Java, and the choice between them often depends on specific project requirements and developer preferences.
Methods to Convert String to JSON in Java
In Java, converting a string to JSON can be accomplished using various libraries. The most popular libraries for this purpose are Jackson, Gson, and org.json. Each of these libraries provides convenient methods to parse strings into JSON objects.
Using Jackson
Jackson is a widely used library for handling JSON in Java. It provides a simple way to convert a string into a JSON object.
Example:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
public class JacksonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Key Points:
- `ObjectMapper` is the main class used for converting between Java objects and JSON.
- The method `readTree` parses the string and returns a `JsonNode`.
Using Gson
Gson, developed by Google, is another popular library for JSON processing. It allows easy conversion between JSON strings and Java objects.
Example:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonParser jsonParser = new JsonParser();
JsonObject jsonObject = jsonParser.parse(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Key Points:
- `JsonParser` is used to parse the JSON string.
- The `getAsJsonObject` method converts the parsed result into a `JsonObject`.
Using org.json
The `org.json` library is another option available for JSON manipulation. It provides straightforward methods to convert strings to JSON objects.
Example:
“`java
import org.json.JSONObject;
public class OrgJsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject);
}
}
“`
Key Points:
- The `JSONObject` class is used to create a JSON object from the string.
- The constructor of `JSONObject` directly accepts a string representation of JSON.
Comparison of Libraries
Feature | Jackson | Gson | org.json |
---|---|---|---|
Performance | High | Moderate | Moderate |
Ease of Use | Simple | Simple | Very simple |
Flexibility | Very flexible | Good flexibility | Basic |
Size | Larger | Smaller | Smaller |
Each library has its strengths and is suitable for different use cases. Selecting the right one depends on the project requirements and personal preference.
Expert Insights on Converting Strings to JSON in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting a string to JSON in Java, it is crucial to utilize libraries such as Jackson or Gson, as they provide robust mechanisms for parsing and serialization. These libraries not only simplify the process but also enhance the reliability of your data handling.”
Michael Thompson (Lead Java Developer, CodeCraft Solutions). “Understanding the structure of the string you wish to convert is essential. Ensuring that the string is properly formatted as JSON will prevent runtime exceptions and allow for seamless data manipulation within your Java applications.”
Sarah Lin (Technical Architect, FutureTech Systems). “Error handling is a vital aspect of converting strings to JSON. Implementing try-catch blocks around your parsing logic can help manage unexpected input formats and maintain application stability, which is often overlooked in initial implementations.”
Frequently Asked Questions (FAQs)
How can I convert a string to JSON in Java?
To convert a string to JSON in Java, you can use libraries such as Jackson or Gson. For example, with Gson, you can use `new Gson().fromJson(jsonString, YourClass.class)` to parse the string into an object of the specified class.
What is the difference between Gson and Jackson for JSON parsing?
Gson is generally simpler and easier to use for basic JSON parsing, while Jackson offers more advanced features and better performance for large datasets. Jackson also supports streaming and can handle more complex data structures.
Can I convert a JSON string to a Map in Java?
Yes, you can convert a JSON string to a Map in Java using Gson with `new Gson().fromJson(jsonString, new TypeToken
What happens if the JSON string is malformed?
If the JSON string is malformed, the parsing library will throw a `JsonSyntaxException` in Gson or a `JsonMappingException` in Jackson. Proper error handling should be implemented to manage these exceptions.
Is there a way to validate a JSON string before conversion?
Yes, you can validate a JSON string using libraries like JsonSchema or by attempting to parse it in a try-catch block. If parsing fails, the string is likely not valid JSON.
Are there any performance considerations when converting large JSON strings?
Yes, performance can be impacted when converting large JSON strings. Using streaming APIs provided by Jackson can improve performance by processing data in chunks rather than loading the entire JSON into memory.
Converting a string to JSON in Java is a common task that developers encounter when working with data interchange formats. The process typically involves using libraries such as Jackson or Gson, which provide robust methods for parsing strings and converting them into JSON objects. These libraries simplify the handling of JSON data structures, allowing for easy manipulation and access to the data contained within.
One of the key takeaways is the importance of selecting the right library based on the specific needs of the project. Jackson is known for its performance and extensive features, making it suitable for complex data structures. In contrast, Gson is often praised for its simplicity and ease of use, which can be beneficial for smaller applications or straightforward JSON parsing tasks. Understanding the strengths and weaknesses of each library can significantly impact the efficiency of data handling in Java applications.
Additionally, it is essential to handle exceptions properly during the conversion process. JSON parsing can throw various exceptions, such as JsonParseException or JsonMappingException, which should be anticipated and managed to ensure the robustness of the application. By implementing proper error handling, developers can create more resilient applications that gracefully handle unexpected data formats.
In summary, converting strings to JSON in Java is a straightforward process when utilizing the right tools and
Author Profile
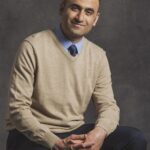
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?