How Can You Convert a String to JSON in Java?
In the world of programming, data interchange formats are vital for seamless communication between applications. Among these formats, JSON (JavaScript Object Notation) stands out for its simplicity and ease of use, making it a popular choice for developers working with web services and APIs. However, when it comes to integrating JSON data into Java applications, many developers encounter the challenge of converting strings into JSON objects. This seemingly straightforward task can be a source of confusion, especially for those new to Java or JSON. In this article, we will demystify the process of converting strings to JSON in Java, providing you with the tools and knowledge to handle data more effectively.
Understanding how to convert a string into a JSON object is essential for any Java developer who interacts with web-based services. This process involves parsing a string representation of JSON data and transforming it into a format that Java can manipulate. Various libraries, such as Jackson and Gson, have been developed to simplify this conversion, each offering unique features and benefits. By leveraging these libraries, developers can easily parse, serialize, and deserialize JSON data, streamlining their workflow and enhancing application performance.
As we delve deeper into the intricacies of string-to-JSON conversion in Java, we will explore the different libraries available, their respective functionalities, and best practices
Using JSON Libraries in Java
To convert a string to JSON in Java, you typically utilize libraries such as Jackson, Gson, or org.json. These libraries provide robust functionalities for parsing and manipulating JSON data. Below is a brief overview of each library.
- Jackson: Known for its performance and ease of use, Jackson can convert JSON to Java objects and vice versa seamlessly.
- Gson: Developed by Google, Gson offers a simple API for converting Java objects to JSON and back. It is particularly useful for working with complex Java objects.
- org.json: This library is straightforward and lightweight, making it suitable for simple JSON operations.
Example Code for Conversion
Here are examples demonstrating how to convert a string to JSON using the three mentioned libraries:
Using Jackson
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
Person person = objectMapper.readValue(jsonString, Person.class);
System.out.println(person);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class Person {
public String name;
public int age;
@Override
public String toString() {
return “Person{name='” + name + “‘, age=” + age + “}”;
}
}
“`
Using Gson
“`java
import com.google.gson.Gson;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”Jane\”, \”age\”:25}”;
Gson gson = new Gson();
Person person = gson.fromJson(jsonString, Person.class);
System.out.println(person);
}
}
class Person {
String name;
int age;
@Override
public String toString() {
return “Person{name='” + name + “‘, age=” + age + “}”;
}
}
“`
Using org.json
“`java
import org.json.JSONObject;
public class JsonOrgExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”Doe\”, \”age\”:40}”;
JSONObject jsonObject = new JSONObject(jsonString);
String name = jsonObject.getString(“name”);
int age = jsonObject.getInt(“age”);
System.out.println(“Name: ” + name + “, Age: ” + age);
}
}
“`
Comparison Table of Libraries
Library | Advantages | Disadvantages |
---|---|---|
Jackson | High performance, supports advanced features | More complex setup compared to others |
Gson | Simple API, well-documented | Performance may lag with large datasets |
org.json | Lightweight and easy to use | Limited functionality compared to others |
Each library has its strengths and weaknesses, and the choice of which to use largely depends on the specific needs of your project.
Using Gson Library to Convert String to JSON
The Gson library, developed by Google, is a popular choice for converting Java objects to JSON and vice versa. To use Gson for converting a string to JSON, follow these steps:
- Add Gson Dependency: If you are using Maven, include the following dependency in your `pom.xml`:
“`xml
“`
- Convert String to JSON: Use the `Gson` class to parse a JSON string into a Java object or directly into a `JsonElement`. Here’s an example:
“`java
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonParser;
public class StringToJsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
Gson gson = new Gson();
// Convert to JsonElement
JsonElement jsonElement = JsonParser.parseString(jsonString);
System.out.println(jsonElement);
}
}
“`
Using Jackson Library for JSON Conversion
Jackson is another powerful library for processing JSON in Java. It provides various methods to convert strings to JSON objects.
- Add Jackson Dependency: Include the following in your `pom.xml` for Maven projects:
“`xml
“`
- Convert String to JSON: Use the `ObjectMapper` class provided by Jackson:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
public class StringToJsonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
ObjectMapper objectMapper = new ObjectMapper();
// Convert String to JsonNode
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
Manual Conversion of String to JSON
In some cases, you may need to manually create a JSON object. This approach is useful for simple structures or when you do not want to rely on external libraries.
- Using `JSONObject` from org.json: If you have the org.json library, you can create JSON manually.
“`java
import org.json.JSONObject;
public class ManualJsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30, \”city\”:\”New York\”}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject.toString());
}
}
“`
- Creating JSON with Java Collections: Another way is to build a JSON structure using Java Maps and Lists.
“`java
import org.json.JSONObject;
public class ManualJsonCreation {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put(“name”, “John”);
jsonObject.put(“age”, 30);
jsonObject.put(“city”, “New York”);
System.out.println(jsonObject.toString());
}
}
“`
Choosing the Right Approach
When deciding which method to use for converting a string to JSON in Java, consider the following factors:
Factor | Gson | Jackson | Manual Creation |
---|---|---|---|
Ease of Use | Simple API | Flexible and powerful | More control, less overhead |
Performance | Good for most cases | High performance for large data | Depends on implementation |
Dependency Management | Requires Gson library | Requires Jackson library | No external dependencies |
Community Support | Strong community | Very strong community | Limited, as it’s less common |
By evaluating your project’s requirements and constraints, you can select the most suitable approach for converting strings to JSON in Java.
Expert Insights on Converting Strings to JSON in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting a string to JSON in Java, it is crucial to utilize libraries such as Jackson or Gson, which provide robust methods for serialization and deserialization. These libraries not only simplify the conversion process but also ensure that the resulting JSON is properly structured and adheres to the expected format.”
Michael Chen (Lead Java Developer, Cloud Solutions Group). “Understanding the nuances of JSON parsing in Java is essential for developers. Using the org.json library, for instance, allows for straightforward conversion of strings to JSON objects. However, developers must handle exceptions effectively to prevent runtime errors during the parsing process.”
Lisa Patel (Technical Architect, Enterprise Software Solutions). “It is advisable to validate the string before attempting to convert it to JSON. Implementing a pre-check can help identify malformed strings early, thus avoiding unnecessary exceptions. Additionally, leveraging the built-in features of libraries like Gson can streamline the conversion process significantly.”
Frequently Asked Questions (FAQs)
How can I convert a string to JSON in Java?
You can convert a string to JSON in Java using libraries such as Jackson or Gson. For example, with Gson, you can use `new Gson().fromJson(jsonString, YourClass.class)` to convert a JSON string into an object of a specified class.
What libraries are commonly used for JSON conversion in Java?
The most commonly used libraries for JSON conversion in Java are Jackson, Gson, and org.json. Each library has its own methods and advantages depending on the use case.
Is it necessary to create a class for JSON conversion?
While it is not strictly necessary, creating a class that represents the structure of the JSON data is recommended. This approach allows for easier mapping and manipulation of the data.
What exceptions should I handle when converting a string to JSON?
You should handle exceptions such as `JsonSyntaxException` and `JsonParseException`, which can occur during the parsing process. Additionally, consider handling `IOException` for input/output operations.
Can I convert a JSON string to a Map in Java?
Yes, you can convert a JSON string to a Map in Java using libraries like Gson. For example, you can use `new Gson().fromJson(jsonString, new TypeToken
What is the difference between Gson and Jackson for JSON conversion?
Gson is generally simpler and more lightweight, making it suitable for smaller projects. Jackson, on the other hand, is more powerful and flexible, offering advanced features like streaming and data binding, which are beneficial for larger applications.
Converting a string to JSON in Java is a fundamental task when working with data interchange formats. The process typically involves using libraries such as Jackson or Gson, which provide robust methods for serialization and deserialization. These libraries allow developers to easily parse JSON strings into Java objects and vice versa, facilitating seamless data manipulation and integration within applications.
One of the key takeaways is the importance of choosing the right library based on specific project requirements. Jackson is known for its performance and extensive features, making it suitable for complex scenarios, while Gson offers simplicity and ease of use for straightforward tasks. Understanding the differences between these libraries can significantly impact the efficiency and maintainability of the code.
Additionally, developers should be aware of the potential pitfalls when converting strings to JSON, such as handling malformed JSON strings and ensuring proper data types during the conversion process. Implementing error handling and validation mechanisms is crucial to prevent runtime exceptions and ensure data integrity.
In summary, converting strings to JSON in Java is a vital skill that enhances the capability of applications to interact with web services and APIs. By leveraging the right tools and practices, developers can efficiently manage JSON data, leading to improved application performance and user experience.
Author Profile
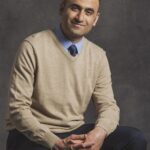
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?