How Can I Convert a String to a JSON Object?
In today’s digital landscape, the ability to seamlessly handle data is more crucial than ever. As developers and data enthusiasts navigate the complexities of programming, one common task they often encounter is the need to convert strings into JSON objects. JSON, or JavaScript Object Notation, has emerged as a universal format for data interchange, prized for its simplicity and readability. However, transforming a plain string into a structured JSON object can be a daunting challenge for many, especially those new to the world of coding. This article will unravel the intricacies of this process, equipping you with the knowledge to efficiently manage and manipulate data in your applications.
Understanding how to convert a string to a JSON object is not just a technical skill; it’s a gateway to unlocking the potential of your data. Whether you’re working with APIs, handling user input, or processing data from various sources, mastering this conversion can streamline your workflow and enhance your application’s functionality. The process typically involves parsing the string and ensuring it adheres to the JSON format, which can vary based on the programming language you are using.
As we delve deeper into this topic, we’ll explore the various methods and best practices for converting strings to JSON objects across different programming environments. From common pitfalls to advanced techniques, you’ll gain insights that will empower you to tackle
Understanding JSON Format
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is commonly used for transmitting data in web applications between a server and a client. JSON represents data as key-value pairs, arrays, and nested objects, making it highly flexible.
Converting a String to a JSON Object
To convert a string into a JSON object, programming languages provide built-in methods or functions that parse the string and return the corresponding JSON object. The process is crucial in scenarios where data is received in string format, such as API responses or configuration files.
Common Methods for Conversion
- JavaScript: The `JSON.parse()` method is used to convert a JSON string into an object.
- Python: The `json.loads()` function is used for the same purpose.
- Java: The `JSONObject` class from the `org.json` package can be utilized.
Example Conversions
Below are examples demonstrating how to convert a string to a JSON object in various programming languages.
Language | Code Example |
---|---|
JavaScript | let jsonObject = JSON.parse('{"name": "John", "age": 30}'); |
Python | import json |
Java | import org.json.JSONObject; |
Error Handling
When converting strings to JSON objects, it is crucial to handle potential errors, such as malformed JSON. Common practices include:
- Try-Catch Blocks: Encapsulating the conversion in a try-catch block to gracefully handle exceptions.
- Validation: Using methods to validate JSON syntax before parsing.
For example, in JavaScript, you can do:
“`javascript
try {
let jsonObject = JSON.parse(‘{“name”: “John”, “age”: 30}’);
} catch (error) {
console.error(“Invalid JSON string:”, error);
}
“`
Conclusion
Converting a string to a JSON object is a fundamental operation in software development, especially when dealing with APIs and data storage. By utilizing the appropriate methods and handling errors effectively, developers can ensure robust data manipulation and application functionality.
Methods to Convert String to JSON Object
The conversion of a string to a JSON object can be accomplished using various programming languages, each offering its own syntax and methods. Below are examples from popular languages.
JavaScript
In JavaScript, the `JSON.parse()` method is utilized to convert a JSON string into a JavaScript object. The syntax is straightforward:
“`javascript
const jsonString = ‘{“name”: “John”, “age”: 30}’;
const jsonObject = JSON.parse(jsonString);
“`
Key Points:
- Ensure the string is properly formatted JSON.
- Handling exceptions is crucial; use `try…catch` for error management.
“`javascript
try {
const jsonObject = JSON.parse(jsonString);
} catch (error) {
console.error(“Invalid JSON string:”, error);
}
“`
Python
In Python, the `json` module provides methods for parsing JSON strings. The `json.loads()` function is specifically designed for this purpose.
“`python
import json
json_string = ‘{“name”: “John”, “age”: 30}’
json_object = json.loads(json_string)
“`
Considerations:
- Ensure that the string adheres to JSON formatting rules.
- Handle exceptions with try-except blocks:
“`python
try:
json_object = json.loads(json_string)
except json.JSONDecodeError as e:
print(“Error decoding JSON:”, e)
“`
Java
Java provides various libraries, such as `org.json` and `Gson`, to convert strings to JSON objects. Using `org.json`, the process is as follows:
“`java
import org.json.JSONObject;
String jsonString = “{\”name\”: \”John\”, \”age\”: 30}”;
JSONObject jsonObject = new JSONObject(jsonString);
“`
Important Notes:
- Ensure to include the required library in your project.
- Exception handling is essential to catch potential errors.
“`java
try {
JSONObject jsonObject = new JSONObject(jsonString);
} catch (JSONException e) {
System.out.println(“Invalid JSON string: ” + e.getMessage());
}
“`
C
In C, the `JsonConvert` class from the Newtonsoft.Json library is commonly used to convert a string to a JSON object.
“`csharp
using Newtonsoft.Json;
string jsonString = “{\”name\”: \”John\”, \”age\”: 30}”;
var jsonObject = JsonConvert.DeserializeObject
“`
Tips:
- Install the Newtonsoft.Json package via NuGet.
- Implement error handling using try-catch:
“`csharp
try {
var jsonObject = JsonConvert.DeserializeObject
} catch (JsonException e) {
Console.WriteLine(“JSON conversion error: ” + e.Message);
}
“`
Common Errors and Troubleshooting
When converting strings to JSON objects, various issues may arise. Here are some common errors and their solutions:
Error Type | Description | Solution |
---|---|---|
Syntax Error | Improperly formatted JSON string | Validate the JSON structure using tools. |
Unexpected Token | Non-JSON characters present | Ensure only valid JSON syntax is used. |
Null Reference Exception | Trying to access properties on null object | Check for null before accessing properties. |
Type Mismatch | Incorrect data types for expected properties | Verify the expected data types match. |
Best Practices:
- Always validate JSON strings before conversion.
- Use robust error handling to manage exceptions effectively.
- Regularly review and test your JSON structures to ensure compatibility with your application logic.
Expert Insights on Converting Strings to JSON Objects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting a string to a JSON object is a fundamental skill for developers. It allows for seamless data interchange between systems. Utilizing libraries like JSON.parse() in JavaScript or the json module in Python can simplify this process significantly.”
Michael Chen (Data Scientist, Analytics Hub). “Understanding how to convert strings to JSON objects is crucial for data manipulation. It enables analysts to transform raw data into structured formats, facilitating easier analysis and visualization.”
Lisa Patel (Lead Backend Developer, Web Solutions Group). “When working with APIs, converting strings to JSON objects is often necessary. Proper error handling during this conversion is essential to ensure that malformed JSON does not cause application failures.”
Frequently Asked Questions (FAQs)
What is the process to convert a string to a JSON object?
To convert a string to a JSON object, use the `JSON.parse()` method in JavaScript. This method takes a valid JSON string as input and returns the corresponding JavaScript object.
What are the requirements for a string to be valid JSON?
A string must adhere to specific formatting rules to be valid JSON. It must be enclosed in double quotes, use proper key-value pairs, and support data types such as strings, numbers, arrays, objects, booleans, and null.
Can I convert a string that is not properly formatted into a JSON object?
No, a string must be properly formatted to convert into a JSON object. If the string contains syntax errors, `JSON.parse()` will throw a `SyntaxError`, indicating that the input is not valid JSON.
What programming languages support string to JSON object conversion?
Many programming languages support this conversion, including JavaScript, Python, Java, C, and PHP. Each language has its own methods or libraries for parsing JSON strings into objects.
How can I handle errors during the conversion process?
To handle errors during conversion, use try-catch blocks in your code. This allows you to catch exceptions thrown by `JSON.parse()` and implement appropriate error handling measures.
Is there a way to convert a JSON object back into a string?
Yes, you can convert a JSON object back into a string using the `JSON.stringify()` method in JavaScript. This method takes a JavaScript object and returns a JSON-formatted string.
Converting a string to a JSON object is a fundamental operation in programming, particularly when dealing with data interchange formats. JSON (JavaScript Object Notation) is widely used due to its simplicity and readability. The process typically involves parsing a string that adheres to JSON syntax into a structured object that can be easily manipulated within programming environments. Various programming languages provide built-in functions or libraries to facilitate this conversion, making it accessible for developers across different platforms.
One of the key takeaways is the importance of ensuring that the string being converted is well-formed according to JSON standards. This includes proper use of quotation marks, commas, and brackets. Failure to adhere to these standards can result in parsing errors, which can disrupt the functionality of applications. Additionally, understanding the structure of the resulting JSON object is crucial for effective data handling and manipulation.
Moreover, developers should be aware of the security implications when converting strings to JSON objects, especially when dealing with user-generated content. Implementing validation and sanitization techniques is essential to prevent potential security vulnerabilities, such as code injection attacks. Overall, mastering the conversion of strings to JSON objects is an invaluable skill that enhances data processing capabilities in modern software development.
Author Profile
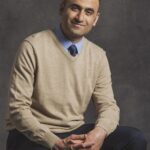
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?