How Can You Convert a String to a JSON Object in Java?
In the world of software development, data interchange formats are crucial for seamless communication between systems. Among these formats, JSON (JavaScript Object Notation) has emerged as a favorite due to its lightweight nature and ease of use. Java developers frequently encounter the need to convert strings into JSON objects, whether they’re parsing data from APIs, handling configuration files, or manipulating data structures. Understanding how to effectively perform this conversion is essential for building robust and efficient applications.
Converting a string to a JSON object in Java may seem straightforward, but it involves a few key considerations, such as ensuring the string is properly formatted and selecting the right libraries for the task. Popular libraries like Jackson and Gson provide powerful tools to facilitate this conversion, allowing developers to focus on building features rather than getting bogged down by data handling intricacies. Each library has its own set of functionalities and performance characteristics, making it important to choose the one that best fits your project’s needs.
As you delve deeper into this topic, you’ll discover various methods and best practices for converting strings to JSON objects in Java. From handling exceptions to optimizing performance, mastering this skill will empower you to manipulate data with confidence and efficiency, paving the way for more sophisticated applications. Whether you’re a seasoned developer or just starting out, understanding this fundamental process will
Using Gson to Convert String to JSON Object
Gson is a popular Java library provided by Google for converting Java objects into their JSON representation and vice versa. To convert a string into a JSON object using Gson, follow these steps:
- Add the Gson library to your project. If you’re using Maven, include the following dependency in your `pom.xml`:
“`xml
“`
- Use the `Gson` class to parse the string. Below is a simple example:
“`java
import com.google.gson.Gson;
import com.google.gson.JsonObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
Gson gson = new Gson();
JsonObject jsonObject = gson.fromJson(jsonString, JsonObject.class);
System.out.println(jsonObject);
}
}
“`
In this example, the `fromJson` method takes a string input and the class type to convert it into. The result is a `JsonObject` that can be manipulated as needed.
Using Jackson for JSON Conversion
Another widely used library for handling JSON in Java is Jackson. It provides a powerful way to convert strings to JSON objects.
- Include Jackson dependencies in your `pom.xml`:
“`xml
“`
- Use the `ObjectMapper` class for conversion:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”Jane\”, \”age\”:25}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
The `readTree` method parses the string and converts it into a `JsonNode`, which can be easily navigated.
Key Differences Between Gson and Jackson
Both Gson and Jackson are capable libraries for JSON manipulation, but they have distinct features and performance characteristics.
Feature | Gson | Jackson |
---|---|---|
Performance | Generally slower for large data sets | Faster, especially with large JSON |
Streaming API | No direct support | Supports streaming for large files |
Data Binding | Simple and easy to use | More complex but powerful |
Custom Serialization | Supports custom serializers | Highly customizable serialization and deserialization |
Choosing between Gson and Jackson often depends on the specific needs of your project, including performance requirements and ease of use.
To effectively convert strings to JSON objects in Java, both Gson and Jackson provide efficient methods and tools. Understanding the nuances and capabilities of each library will assist in selecting the appropriate one for your application.
Using Gson Library to Convert String to JSON Object
The Gson library, created by Google, is a popular choice for converting Java objects to JSON and vice versa. To convert a string to a JSON object using Gson, follow these steps:
- Add Gson Dependency: If you’re using Maven, include the following dependency in your `pom.xml`:
“`xml
“`
- Convert String to JSON Object:
Here is a sample code snippet demonstrating how to convert a JSON string into a JSON object:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Using org.json Library
The `org.json` library provides another straightforward way to handle JSON in Java. To convert a string to a JSON object, follow these steps:
- Add org.json Dependency: If you are using Maven, add the following dependency:
“`xml
“`
- Convert String to JSON Object:
Use the following code to perform the conversion:
“`java
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject);
}
}
“`
Using Jackson Library
Jackson is another widely-used library for processing JSON in Java. The steps to convert a string to a JSON object using Jackson are outlined below:
- Add Jackson Dependency: Include the following in your `pom.xml`:
“`xml
“`
- Convert String to JSON Object:
Here’s how you can convert a string into a JSON object:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) throws Exception {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
}
}
“`
Key Considerations
When converting strings to JSON objects in Java, consider the following:
- Error Handling: Always include error handling when parsing JSON. Use try-catch blocks to manage potential exceptions like `JsonParseException` or `IOException`.
- Valid JSON Format: Ensure that the string being converted is in valid JSON format; otherwise, parsing will fail.
- Library Choice: Choose a library based on your project requirements. Gson is lightweight, Jackson is feature-rich and fast, and org.json is simple and easy to use.
- Performance: For large datasets, consider the performance implications of the chosen library and approach.
Expert Insights on Converting Strings to JSON Objects in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting a string to a JSON object in Java, utilizing libraries like Jackson or Gson is essential. These libraries provide robust methods for parsing JSON data efficiently, ensuring that your application can handle complex data structures seamlessly.”
Michael Thompson (Java Development Lead, CodeCrafters LLC). “It is crucial to validate the string format before conversion. A malformed JSON string can lead to runtime exceptions. Implementing error handling and using try-catch blocks can significantly enhance the reliability of your code when working with JSON in Java.”
Lisa Chen (Technical Architect, FutureTech Solutions). “Understanding the differences between JSON and Java objects is vital. When converting, ensure that your Java classes are properly annotated if you are using frameworks like Jackson, as this will allow for smooth serialization and deserialization processes.”
Frequently Asked Questions (FAQs)
How do I convert a string to a JSON object in Java?
To convert a string to a JSON object in Java, you can use libraries such as `org.json` or `Gson`. For example, using `org.json`, you can create a `JSONObject` by passing the string to its constructor: `JSONObject jsonObject = new JSONObject(jsonString);`.
What libraries are commonly used for JSON manipulation in Java?
Common libraries for JSON manipulation in Java include `org.json`, `Gson`, and `Jackson`. Each library provides methods for parsing, generating, and manipulating JSON data effectively.
Can I convert a JSON string to a Java object?
Yes, you can convert a JSON string to a Java object using libraries like Gson or Jackson. For example, with Gson, you can use `Gson gson = new Gson(); MyClass obj = gson.fromJson(jsonString, MyClass.class);` to deserialize the JSON string into a Java object.
What exceptions should I handle when converting a string to a JSON object?
When converting a string to a JSON object, you should handle exceptions such as `JSONException` (for malformed JSON) and `NullPointerException` (if the input string is null). Proper exception handling ensures robustness in your application.
Is it necessary to validate a JSON string before converting it to a JSON object?
While it is not strictly necessary, validating a JSON string before conversion is a best practice. It helps to ensure that the string is well-formed and adheres to the expected structure, reducing the likelihood of runtime errors.
What is the difference between JSONObject and Gson in Java?
`JSONObject` is part of the `org.json` library and is primarily used for creating and manipulating JSON data in a more manual fashion. In contrast, Gson is a comprehensive library that provides automatic serialization and deserialization of Java objects to and from JSON, making it easier to work with complex data structures.
Converting a string to a JSON object in Java is a common task, especially when dealing with APIs or data interchange formats. The process typically involves utilizing libraries such as Jackson or Gson, which provide robust methods for parsing JSON strings into Java objects. These libraries simplify the conversion process and handle various data types and structures efficiently, allowing developers to focus on the application logic rather than the intricacies of JSON parsing.
One of the key takeaways is the importance of selecting the right library based on the specific needs of the project. Jackson is known for its speed and flexibility, making it suitable for high-performance applications, while Gson is praised for its ease of use and readability. Understanding the strengths and weaknesses of each library can significantly impact the development process and the performance of the application.
Furthermore, it is crucial to handle exceptions properly during the conversion process. JSON parsing can fail due to malformed strings or incompatible data types, so implementing robust error handling mechanisms is essential. This ensures that the application remains stable and provides meaningful feedback to users when issues arise.
In summary, converting a string to a JSON object in Java is a straightforward process when using the appropriate libraries. By choosing the right tool and implementing effective error handling, developers can
Author Profile
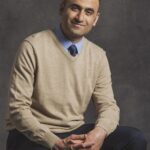
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?