How Can I Convert a Struct to a JSON String?
In the realm of programming, data interchange formats play a crucial role in how applications communicate with one another. Among these formats, JSON (JavaScript Object Notation) stands out for its simplicity and readability, making it a favorite among developers. However, as applications evolve, so too does the need to convert complex data structures, like structs in languages such as Go or C, into JSON strings that can be easily transmitted and understood. Understanding how to perform this conversion is not just a technical necessity; it’s a gateway to enhancing the interoperability of your applications and improving data handling efficiency.
Converting a struct to a JSON string is a fundamental skill that every developer should master. At its core, this process involves serializing the structured data, allowing it to be transformed into a format that can be easily parsed and utilized by other systems. This transformation is essential for APIs, data storage, and even configuration management, as it enables seamless communication between disparate systems. Whether you are working with a simple data structure or a complex nested object, the principles of conversion remain the same, providing a solid foundation for effective data manipulation.
As we delve deeper into the nuances of converting structs to JSON strings, we will explore various programming languages and their respective libraries that facilitate this process. We will also discuss best
Methods to Convert Struct to JSON String
To convert a struct to a JSON string in programming languages like Go, Python, or C, various built-in libraries and functions can be utilized. Each language offers unique methods to facilitate this conversion, ensuring that structured data can be easily serialized into a JSON format.
Go Language Example
In Go, the `encoding/json` package provides an efficient way to convert structs to JSON strings. By employing the `json.Marshal` function, you can serialize your struct as follows:
“`go
package main
import (
“encoding/json”
“fmt”
)
type Person struct {
Name string `json:”name”`
Age int `json:”age”`
}
func main() {
p := Person{Name: “Alice”, Age: 30}
jsonData, err := json.Marshal(p)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(jsonData))
}
“`
In this example, the struct `Person` is defined with JSON tags that dictate how the field names will appear in the resulting JSON string.
Python Example
Python simplifies struct-to-JSON conversion with the `json` module. For instance, using the `json.dumps` method allows you to convert a dictionary (which can be thought of as a struct) to a JSON string:
“`python
import json
person = {
“name”: “Alice”,
“age”: 30
}
json_data = json.dumps(person)
print(json_data)
“`
Here, the dictionary `person` is transformed into a JSON string using `json.dumps`.
CExample
In C, the `System.Text.Json` namespace provides functionality to convert objects to JSON strings. The `JsonSerializer.Serialize` method is frequently used for this purpose:
“`csharp
using System;
using System.Text.Json;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Program
{
public static void Main()
{
var person = new Person { Name = “Alice”, Age = 30 };
string jsonData = JsonSerializer.Serialize(person);
Console.WriteLine(jsonData);
}
}
“`
In this Cexample, the `Person` class is serialized into a JSON string, demonstrating a straightforward approach to object serialization.
Key Considerations
When converting structs or objects to JSON, several key considerations should be kept in mind:
- Field Visibility: Ensure that the fields of the struct or object are public or accessible based on the serialization rules of the chosen language.
- Data Types: Check the compatibility of data types within the struct and their corresponding JSON representations.
- Null Values: Handle null values appropriately to avoid unexpected results in the output JSON.
Comparison of Serialization Methods
The following table summarizes the main serialization methods for converting structs to JSON strings in different programming languages:
Language | Library/Method | Example Function |
---|---|---|
Go | encoding/json | json.Marshal |
Python | json | json.dumps |
C | System.Text.Json | JsonSerializer.Serialize |
Each method is tailored to the syntax and structure of the respective programming language, allowing for smooth serialization of structured data into JSON format.
Methods to Convert Struct to JSON String
To convert a struct to a JSON string, several programming languages provide built-in libraries or functions. Below are examples in popular programming languages, detailing how to perform this conversion.
Go
In Go, you can utilize the `encoding/json` package to convert a struct to a JSON string. The `json.Marshal` function serializes the struct.
“`go
package main
import (
“encoding/json”
“fmt”
)
type Person struct {
Name string `json:”name”`
Age int `json:”age”`
}
func main() {
p := Person{Name: “Alice”, Age: 30}
jsonData, err := json.Marshal(p)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(jsonData))
}
“`
Python
Python provides the `json` module for converting dictionaries and objects to JSON strings. For custom objects, you can define a method to convert the object to a dictionary.
“`python
import json
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def to_dict(self):
return {“name”: self.name, “age”: self.age}
p = Person(“Alice”, 30)
json_data = json.dumps(p.to_dict())
print(json_data)
“`
JavaScript
In JavaScript, you can utilize the `JSON.stringify` method to convert an object to a JSON string.
“`javascript
const person = {
name: “Alice”,
age: 30
};
const jsonData = JSON.stringify(person);
console.log(jsonData);
“`
C
Chas built-in support for JSON serialization via the `System.Text.Json` namespace. Use `JsonSerializer.Serialize` to convert a struct or class to a JSON string.
“`csharp
using System;
using System.Text.Json;
public struct Person
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
Person p = new Person { Name = “Alice”, Age = 30 };
string jsonData = JsonSerializer.Serialize(p);
Console.WriteLine(jsonData);
}
}
“`
Ruby
In Ruby, the `json` library can be used to convert a hash or object to JSON. You can define a method to convert the instance variables to a hash.
“`ruby
require ‘json’
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def to_hash
{ name: @name, age: @age }
end
end
p = Person.new(“Alice”, 30)
json_data = p.to_hash.to_json
puts json_data
“`
Common Considerations
When converting structs to JSON strings, consider the following:
- Field Visibility: Ensure that the fields intended for serialization are public or accessible.
- Data Types: Be mindful of how different data types are serialized (e.g., dates and custom objects).
- Null Values: Decide how to handle null or default values in the struct.
- Performance: Serialization can be resource-intensive; assess the impact on performance in large applications.
By leveraging the respective libraries and functions in your programming language of choice, converting a struct to a JSON string can be accomplished efficiently and effectively.
Expert Insights on Converting Structs to JSON Strings
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting a struct to a JSON string is a straightforward process in many programming languages. Utilizing libraries such as `json` in Python or `Newtonsoft.Json` in Ccan simplify this task significantly, allowing developers to serialize complex data structures with ease.”
Mark Thompson (Lead Data Scientist, Data Solutions Group). “When converting structs to JSON, it is crucial to consider the data types involved. Not all data types in a struct map directly to JSON types, which can lead to serialization issues. Ensuring compatibility through careful design can save time and prevent errors during data exchange.”
Linda Zhang (Software Architect, Cloud Tech Enterprises). “Performance can be a concern when converting large structs to JSON strings. It is advisable to benchmark different serialization methods and choose one that balances speed and memory usage, especially in high-load applications where efficiency is paramount.”
Frequently Asked Questions (FAQs)
What is the process to convert a struct to a JSON string?
To convert a struct to a JSON string, you typically use a serialization library or built-in functions provided by the programming language. For example, in Go, you can use the `json.Marshal()` function, while in Python, you can use `json.dumps()`.
Which programming languages support struct to JSON conversion?
Most modern programming languages support struct to JSON conversion, including but not limited to Go, Python, Java, C, and JavaScript. Each language has its own libraries or built-in functions to facilitate this process.
Are there any libraries specifically designed for struct to JSON conversion?
Yes, many libraries are designed for this purpose. For example, in Go, the `encoding/json` package is widely used. In Python, the `json` module serves this function, while in Java, libraries like Jackson and Gson are popular choices.
What are common issues encountered during struct to JSON conversion?
Common issues include handling unexported fields, circular references, and data type mismatches. Additionally, ensuring that the struct adheres to the JSON format specifications is crucial to avoid serialization errors.
How can I customize the JSON output when converting a struct?
Customization can often be achieved by using tags or annotations within the struct definition. For instance, in Go, you can use struct tags to specify field names and omit fields, while in Python, you can define a custom serialization method.
Is it possible to convert a JSON string back to a struct?
Yes, converting a JSON string back to a struct is commonly done using deserialization functions. In Go, you can use `json.Unmarshal()`, and in Python, you can use `json.loads()` to achieve this.
Converting a struct to a JSON string is a common requirement in programming, particularly when dealing with data interchange between systems. Structs, which are composite data types that group together variables under a single name, can be easily serialized into JSON format using various programming languages and libraries. This process allows for the structured representation of data, making it easier to transmit and store information in a human-readable format.
Different programming languages offer built-in functions or libraries to facilitate the conversion of structs to JSON. For instance, in languages like Go, the `encoding/json` package provides straightforward methods to marshal structs into JSON strings. Similarly, in Python, the `json` module allows for the serialization of dictionaries and custom objects. Understanding the specific methods available in your programming language of choice is crucial for effective implementation.
Key takeaways from this discussion include the importance of selecting the appropriate serialization method based on the programming environment and the structure of the data being converted. Additionally, developers should be aware of potential pitfalls, such as handling nested structs and ensuring that all data types are compatible with JSON format. By mastering the conversion process, developers can enhance data interoperability and streamline communication between different systems.
Author Profile
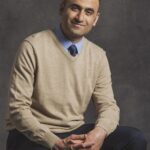
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?