How Can I Convert XLSX to CSV Using JavaScript?
In today’s data-driven world, the ability to efficiently manipulate and convert files is essential for businesses and developers alike. One common task that often arises is the need to convert Excel spreadsheets, typically saved in XLSX format, into CSV files. This conversion is crucial for various applications, from data analysis to integration with web services and databases. If you’re looking to streamline your workflow or enhance your project’s capabilities, understanding how to convert XLSX to CSV using JavaScript can be a game changer.
JavaScript, with its versatility and widespread use in web development, offers several powerful libraries and tools that facilitate this conversion process. Whether you’re working on a Node.js application or a client-side script, there are numerous options available that can help you seamlessly transform your data. By leveraging these resources, developers can easily handle large datasets, ensuring that the information is accessible and usable in a variety of contexts.
As we delve deeper into this topic, we will explore the various methods and libraries available for converting XLSX to CSV in JavaScript. From simple scripts to comprehensive libraries, you’ll discover the best practices and techniques that can enhance your data management tasks. Get ready to unlock the potential of your data and streamline your processes with effective conversion strategies!
Libraries for XLSX to CSV Conversion
When converting XLSX files to CSV in JavaScript, several libraries offer robust solutions. Each library has unique features, and the choice depends on specific project requirements. Below are some of the popular libraries:
- SheetJS (xlsx): This is one of the most widely used libraries for handling Excel files. It supports reading and writing various formats and is well-documented.
- PapaParse: While primarily a CSV parser, it can be utilized alongside other libraries to convert XLSX to CSV.
- ExcelJS: This library is designed for reading, manipulating, and writing Excel files in both XLSX and CSV formats.
Using SheetJS for Conversion
SheetJS provides a straightforward API for converting XLSX files to CSV. Here’s a step-by-step guide to achieve this:
- Installation: First, install the library using npm or include it in your HTML file.
“`bash
npm install xlsx
“`
- Reading the XLSX File: Use the `readFile` method to load your XLSX file.
- Converting to CSV: Utilize the `sheet_to_csv` method to convert the data to CSV format.
Here’s an example code snippet:
“`javascript
const XLSX = require(‘xlsx’);
function convertXlsxToCsv(filePath) {
const workbook = XLSX.readFile(filePath);
const sheetName = workbook.SheetNames[0]; // Access the first sheet
const worksheet = workbook.Sheets[sheetName];
const csv = XLSX.utils.sheet_to_csv(worksheet);
return csv;
}
const csvData = convertXlsxToCsv(‘path/to/your/file.xlsx’);
console.log(csvData);
“`
This code reads an XLSX file, selects the first sheet, and converts it to CSV format.
Handling Multiple Sheets
If your XLSX file contains multiple sheets, you may want to convert all sheets to CSV. This can be achieved by iterating through the `SheetNames` array. Below is an example:
“`javascript
function convertAllSheetsToCsv(filePath) {
const workbook = XLSX.readFile(filePath);
const csvData = {};
workbook.SheetNames.forEach(sheetName => {
const worksheet = workbook.Sheets[sheetName];
csvData[sheetName] = XLSX.utils.sheet_to_csv(worksheet);
});
return csvData;
}
const allCsvData = convertAllSheetsToCsv(‘path/to/your/file.xlsx’);
console.log(allCsvData);
“`
This function returns an object where each key is a sheet name, and the value is the corresponding CSV data.
Performance Considerations
When dealing with large XLSX files, performance can be a concern. Here are some strategies to enhance performance:
- Stream Processing: Use libraries that support streaming to handle large files without consuming much memory.
- Selective Sheet Conversion: Instead of converting all sheets, target only those that are necessary.
- Asynchronous Operations: Use asynchronous methods to avoid blocking the main thread during file reading.
Comparison Table of Libraries
Library | Features | Use Cases |
---|---|---|
SheetJS (xlsx) | Read/Write various formats, Supports large files | General purpose Excel handling |
PapaParse | Fast CSV parsing, Good for streaming | CSV specific tasks |
ExcelJS | Read/Write XLSX, Styling support | Creating and editing Excel files |
By choosing the appropriate library and following the outlined methods, converting XLSX files to CSV in JavaScript can be accomplished efficiently and effectively.
Understanding XLSX and CSV Formats
XLSX (Excel Spreadsheet) and CSV (Comma-Separated Values) are two prevalent file formats used for data storage. Understanding their differences can help in the conversion process.
- XLSX:
- Supports complex data structures.
- Can contain multiple sheets, formulas, charts, and rich formatting.
- Generally larger in file size due to embedded features.
- CSV:
- A plain text format that stores data in a tabular structure.
- Limited to a single sheet and does not support formulas or formatting.
- Smaller file size, making it easier to handle for simple datasets.
Libraries for Converting XLSX to CSV in JavaScript
Several libraries facilitate the conversion of XLSX files to CSV in JavaScript. Here are a few popular options:
- SheetJS (xlsx):
- A powerful library for parsing and writing various spreadsheet formats.
- Supports reading and converting XLSX to CSV seamlessly.
- PapaParse:
- Primarily a CSV parser but can work alongside SheetJS for conversion tasks.
- Offers robust parsing options and can handle large files efficiently.
- FileSaver.js:
- Useful for saving the generated CSV file to the user’s device after conversion.
Sample Code for Conversion
Using SheetJS, you can convert an XLSX file to CSV with the following code snippet:
“`javascript
const XLSX = require(‘xlsx’);
const fs = require(‘fs’);
// Load the XLSX file
const workbook = XLSX.readFile(‘path/to/file.xlsx’);
// Assuming you want the first sheet
const sheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[sheetName];
// Convert to CSV
const csv = XLSX.utils.sheet_to_csv(worksheet);
// Save the CSV file
fs.writeFileSync(‘output.csv’, csv);
“`
Handling Large Files and Performance Considerations
When working with large XLSX files, performance can be a concern. Consider the following strategies to optimize the conversion process:
- Streaming: Use libraries that support streaming to handle large files without exhausting memory.
- Chunking: Break the data into smaller chunks for processing, which can improve performance and reduce memory usage.
- Web Workers: For web applications, consider offloading the conversion task to a Web Worker to keep the UI responsive.
Common Issues and Troubleshooting
While converting XLSX to CSV, you may encounter some common issues:
Issue | Solution |
---|---|
Data Loss | Ensure that the data types and formatting are compatible. |
Special Characters | Use UTF-8 encoding to handle special characters properly. |
Large File Size | Implement chunking or streaming to process efficiently. |
Incorrect Formatting | Verify the original XLSX structure and validate the output. |
By addressing these potential problems and employing the right libraries and strategies, you can effectively convert XLSX files to CSV format in JavaScript.
Expert Insights on Converting XLSX to CSV Using JavaScript
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Converting XLSX to CSV in JavaScript can be efficiently accomplished using libraries like SheetJS. This allows developers to handle complex spreadsheet data while ensuring compatibility with various applications that require CSV formats.”
Michael Patel (Software Engineer, Data Solutions Corp.). “When implementing XLSX to CSV conversion in JavaScript, it’s crucial to consider the structure of your data. Properly managing nested data and ensuring that special characters are correctly encoded can prevent data loss during the conversion process.”
Sarah Johnson (Senior Web Developer, CodeCraft Labs). “Utilizing asynchronous functions in JavaScript can significantly enhance the performance of XLSX to CSV conversions. This approach allows for smoother user experiences, especially when dealing with large datasets that might otherwise block the main thread.”
Frequently Asked Questions (FAQs)
How can I convert an XLSX file to CSV using JavaScript?
You can convert an XLSX file to CSV in JavaScript by using libraries such as `xlsx` or `SheetJS`. These libraries allow you to read XLSX files and export the data in CSV format programmatically.
What is the `xlsx` library in JavaScript?
The `xlsx` library, also known as SheetJS, is a popular JavaScript library that enables reading, writing, and manipulating spreadsheet files in various formats, including XLSX and CSV.
Are there any limitations when converting XLSX to CSV?
Yes, converting XLSX to CSV may result in the loss of certain features such as formatting, formulas, and multiple sheets. CSV files only support plain text data in a single sheet format.
Can I convert XLSX to CSV in a web browser?
Yes, you can convert XLSX to CSV directly in a web browser using JavaScript. By utilizing the `xlsx` library, you can read the file input and convert it to CSV format without needing a server.
Is it possible to handle large XLSX files during conversion?
Handling large XLSX files can be challenging due to memory constraints. However, the `xlsx` library provides methods to read files in a streaming manner, which can help manage larger datasets more efficiently.
Do I need to install any packages to use the `xlsx` library?
Yes, you need to install the `xlsx` library using npm or include it via a CDN in your HTML file to utilize its functionalities for converting XLSX to CSV.
Converting XLSX files to CSV format using JavaScript is a common requirement for developers working with data. The process typically involves utilizing libraries designed to read and manipulate Excel files, such as SheetJS (xlsx), which provides robust functionality for parsing and converting spreadsheet data. By leveraging these libraries, developers can efficiently handle the complexities of Excel file structures and output the data in a universally accepted CSV format.
One of the key advantages of converting XLSX to CSV is the simplification of data handling. CSV files are plain text files that can be easily read and processed by various applications, making them ideal for data interchange. This conversion allows for easier integration with databases, data analysis tools, and other programming environments, enhancing the overall flexibility of data management workflows.
Moreover, the process can be automated using JavaScript in both client-side and server-side environments. This capability is particularly beneficial for applications that require real-time data processing or batch conversions. By implementing effective error handling and validation checks during the conversion process, developers can ensure data integrity and minimize potential issues that may arise from format discrepancies.
In summary, converting XLSX files to CSV using JavaScript is a practical solution that streamlines data management and enhances interoperability. By utilizing appropriate
Author Profile
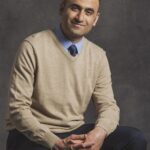
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?