Are You Facing Memory Issues When Converting CSV to String?
In today’s data-driven world, CSV (Comma-Separated Values) files have become a staple format for storing and sharing tabular data. Their simplicity and ease of use make them a popular choice among developers and data analysts alike. However, as datasets grow larger and more complex, the process of converting CSV files into strings can lead to unexpected challenges, particularly concerning memory management. This article delves into the intricacies of this seemingly straightforward task, exploring the potential pitfalls and offering insights on how to navigate them effectively.
When converting CSV files to strings, memory issues can arise due to the sheer volume of data being processed. Large datasets can consume significant amounts of RAM, leading to slow performance or even crashes in extreme cases. This is especially true in environments with limited resources or when using programming languages that do not manage memory efficiently. Understanding the underlying causes of these memory issues is crucial for developers who wish to maintain optimal performance while handling large CSV files.
Moreover, the methods used for conversion can greatly influence memory consumption. Different programming languages and libraries offer various approaches, each with its own strengths and weaknesses. By examining these methods and their implications, developers can make informed decisions that not only streamline the conversion process but also mitigate potential memory-related challenges. In the following sections, we will explore practical strategies
Understanding Memory Issues During CSV to String Conversion
When converting CSV files to strings, memory issues can arise due to the way data is processed and stored in memory. A CSV file, depending on its size, can contain a significant amount of data which, when loaded entirely into memory as a string, can lead to high memory consumption and potential crashes. Understanding the factors that contribute to these issues is crucial for efficient data handling.
Common causes of memory issues during conversion include:
- Large File Sizes: Large CSV files can consume substantial memory when loaded. If the file is several gigabytes, converting it to a string may exceed available memory limits.
- Inefficient Parsing: Using inefficient algorithms or libraries that do not optimize memory usage can exacerbate memory issues.
- Redundant Data: If the CSV contains redundant or unnecessary data, converting it without filtering can inflate the memory usage.
Strategies to Mitigate Memory Issues
Several strategies can be employed to mitigate memory issues when converting CSV files to strings. These methods help manage memory usage effectively:
- Streaming Processing: Instead of loading the entire CSV file into memory, use streaming techniques that read and process the file line by line or in chunks. This approach minimizes memory overhead.
- Data Filtering: Prioritize filtering unnecessary columns or rows before conversion. This reduces the amount of data that needs to be handled.
- Using Efficient Libraries: Opt for libraries designed for memory efficiency. For example, libraries like `pandas` in Python have built-in methods for handling large datasets more effectively.
- Increasing Memory Limits: In some programming environments, you can increase the memory limits available to your application, allowing for larger data handling, though this is often a temporary solution.
Example of Streaming Conversion in Python
Below is a simple example demonstrating how to convert a CSV file to a string using streaming in Python. This method helps avoid memory issues by processing data in manageable chunks.
“`python
import csv
def csv_to_string(file_path):
output_string = “”
with open(file_path, ‘r’) as file:
reader = csv.reader(file)
for row in reader:
output_string += ‘,’.join(row) + ‘\n’
return output_string
“`
This method reads the CSV file line by line, appending each row to the output string without loading the entire file into memory at once.
Performance Comparison: Different Approaches
The following table summarizes the memory usage and performance of different methods for converting CSV to string:
Method | Memory Usage | Speed |
---|---|---|
Full Load | High | Fast |
Streaming | Low | Moderate |
Filtered Load | Medium | Fast |
This comparison illustrates that while full loading is faster, it comes at a cost of high memory usage. Streaming is more memory-efficient but may result in longer processing times. Filtering provides a balance between speed and memory efficiency.
Understanding Memory Issues in CSV to String Conversion
When converting CSV files to strings, several factors can lead to memory issues. These issues primarily stem from the size of the CSV file, the method used for conversion, and the environment in which the conversion is executed.
- File Size: Large CSV files can consume significant amounts of memory when loaded entirely into memory.
- Data Complexity: CSV files with complex data types or large numbers of columns can exacerbate memory usage.
- Inefficient Algorithms: Using inefficient methods for reading and converting data can lead to excessive memory consumption.
Common Causes of Memory Issues
- Loading Entire File into Memory:
- Reading the entire CSV file into memory at once can lead to memory exhaustion, especially for large files.
- Inefficient Parsing Libraries:
- Some libraries may not be optimized for handling large datasets, causing higher memory usage.
- String Manipulation Overhead:
- Converting data types and manipulating strings can lead to temporary memory spikes.
- Garbage Collection Delays:
- In environments with automatic garbage collection, unreferenced objects may not be collected immediately, leading to increased memory usage.
Best Practices for Efficient Conversion
To minimize memory issues during CSV to string conversion, consider the following best practices:
- Stream Processing:
- Use libraries that support streaming to process data in chunks rather than loading the entire file.
- Optimized Libraries:
- Utilize libraries designed for performance, such as `pandas` in Python or `Apache Commons CSV` in Java.
- Incremental Processing:
- Convert rows to strings incrementally and write them to an output file or buffer rather than accumulating them in memory.
- Data Type Management:
- Ensure data types are managed efficiently to reduce overhead during conversion.
Example of Efficient CSV to String Conversion
Consider the following Python example using `pandas` for efficient CSV to string conversion:
“`python
import pandas as pd
Use iterator to read large CSV in chunks
chunk_size = 10000 Define chunk size
csv_file_path = ‘large_file.csv’
Initialize an empty string for the final result
result_string = “”
for chunk in pd.read_csv(csv_file_path, chunksize=chunk_size):
result_string += chunk.to_string(index=) + “\n” Append each chunk’s string representation
Result string contains the entire data, but managed in chunks
“`
Monitoring Memory Usage
To effectively manage memory usage during the conversion process, consider these monitoring techniques:
- Memory Profiling Tools:
- Use tools like `memory_profiler` in Python to track memory usage in real-time.
- System Monitoring:
- Utilize system monitoring tools to observe memory consumption patterns during execution.
- Adjusting Environment Settings:
- Configure environment variables or settings to allocate more memory if necessary, depending on the language or framework used.
Technique | Description |
---|---|
Memory Profiling | Track memory usage and identify bottlenecks. |
System Monitoring | Monitor overall system memory during execution. |
Environment Configuration | Adjust settings to allow more memory allocation. |
Expert Insights on Memory Issues When Converting CSV to String
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “When converting large CSV files to strings, memory management becomes critical. If the dataset exceeds available memory, it can lead to significant performance degradation or even application crashes. Utilizing streaming techniques or chunk processing can mitigate these issues effectively.”
Mark Thompson (Software Engineer, Cloud Solutions Corp.). “I have observed that inefficient data handling during CSV to string conversion often results in excessive memory consumption. It is advisable to implement garbage collection and optimize data structures to ensure that memory is utilized efficiently, especially with large datasets.”
Lisa Patel (Systems Architect, DataFlow Technologies). “In my experience, converting CSV files to strings can cause memory issues if not approached with caution. Leveraging libraries designed for efficient data parsing and conversion can significantly reduce the memory footprint, thus enhancing overall application stability.”
Frequently Asked Questions (FAQs)
What are common causes of memory issues when converting CSV to string?
Memory issues during CSV to string conversion often arise from large file sizes, inefficient parsing methods, or inadequate memory allocation in the programming environment. Using methods that load the entire file into memory can exacerbate these problems.
How can I optimize memory usage while converting CSV to string?
To optimize memory usage, consider processing the CSV file in smaller chunks instead of loading it entirely. Utilizing streaming libraries or iterating through the file line by line can significantly reduce memory consumption.
Are there specific programming languages that handle CSV conversion better than others?
Languages like Python and Java provide robust libraries for handling CSV files efficiently. Python’s `pandas` and Java’s `OpenCSV` are examples of libraries that can manage memory effectively during conversion tasks.
What tools or libraries can help prevent memory issues during conversion?
Tools such as `pandas` in Python, `csv` module in Python, and `Apache Commons CSV` in Java can help manage memory more effectively. These libraries often include built-in methods for handling large datasets without excessive memory usage.
Is there a way to monitor memory usage during the conversion process?
Yes, many programming environments offer profiling tools that allow you to monitor memory usage. In Python, for example, you can use the `memory_profiler` library to track memory consumption during the conversion process.
What should I do if I encounter memory errors during CSV to string conversion?
If memory errors occur, consider optimizing your code to process data in smaller segments, increasing available memory, or using a more efficient data handling library. Additionally, reviewing the data structure for unnecessary complexity can also help.
Converting CSV files to strings can lead to significant memory issues, particularly when dealing with large datasets. The process typically involves loading the entire CSV file into memory, which can consume substantial resources depending on the file size. This can result in performance degradation or even application crashes if the system runs out of available memory. It is essential to consider the limitations of the environment in which the conversion is taking place, as inadequate memory can severely impact the execution of such operations.
Another critical aspect to consider is the method used for the conversion. Different programming languages and libraries offer various approaches to read and manipulate CSV files. Some methods may be more memory-efficient than others, allowing for streaming or chunked processing of data instead of loading the entire file at once. Utilizing these more efficient techniques can mitigate memory issues and improve overall performance during the conversion process.
when converting CSV files to strings, it is crucial to be aware of the potential memory implications. By selecting appropriate methods and being mindful of the data size, one can effectively manage memory usage and ensure smoother operations. Implementing best practices in handling large datasets will not only enhance performance but also prevent system overloads, leading to more reliable software applications.
Author Profile
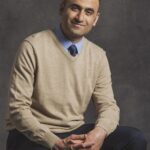
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?