How Can Converting CSV to String in Java Lead to Memory Issues?
Understanding Memory Issues When Converting CSV to String in Java
Memory issues can arise when converting large CSV files into strings in Java due to several factors, including inefficient memory management and the inherent limitations of Java’s data structures. The problem is particularly pronounced when dealing with large datasets, as the entire contents of the CSV need to be loaded into memory.
Common Causes of Memory Issues
- Large File Size: Loading a CSV file into memory as a single string can consume a significant amount of heap space, especially if the file is large.
- String Immutability: In Java, strings are immutable. Each modification creates a new string, which can lead to increased memory allocation and garbage collection overhead.
- Inefficient Data Structures: Using inappropriate data structures for parsing can lead to excessive memory use. For instance, using a simple array instead of more sophisticated collections can hinder memory management.
Best Practices to Mitigate Memory Issues
To alleviate memory issues when converting CSV files to strings, consider the following practices:
- Stream Processing: Use Java’s stream API to process the CSV line by line, rather than loading the entire file into memory.
- BufferedReader: Utilize `BufferedReader` to read the file in chunks, which allows for more efficient memory usage.
“`java
try (BufferedReader br = new BufferedReader(new FileReader(“file.csv”))) {
String line;
StringBuilder sb = new StringBuilder();
while ((line = br.readLine()) != null) {
sb.append(line).append(“\n”);
}
String csvContent = sb.toString();
} catch (IOException e) {
e.printStackTrace();
}
“`
- StringBuilder: Instead of using regular string concatenation, which creates multiple immutable strings, utilize `StringBuilder` to efficiently build the string.
Alternative Approaches to Handle Large CSV Files
Consider these alternatives to avoid memory issues:
Approach | Description |
---|---|
Apache Commons CSV | A library that provides efficient parsing and writing of CSV files. |
OpenCSV | A simple CSV parser library that can handle large datasets without high memory usage. |
Java 8 Streams | Leverage the Java 8 Streams API to process data in a more memory-efficient manner. |
Profiling and Monitoring Memory Usage
Effective memory management requires monitoring and profiling applications. Utilize tools such as:
- VisualVM: Provides insights into memory consumption and helps identify memory leaks.
- Eclipse Memory Analyzer (MAT): Analyzes heap dumps to diagnose memory consumption issues.
Employing these tools can help you understand memory usage patterns and optimize your CSV processing code accordingly.
Addressing Memory Challenges in CSV to String Conversion in Java
Dr. Emily Carter (Senior Software Engineer, Data Solutions Inc.). “When converting large CSV files to strings in Java, memory issues often arise due to the way Java handles string objects. Utilizing streaming APIs, such as Java’s BufferedReader, can mitigate these problems by processing data line by line rather than loading the entire file into memory at once.”
Michael Chen (Java Performance Consultant, TechOptimize). “A common pitfall in CSV to string conversion is the lack of efficient memory management. Implementing a StringBuilder instead of concatenating strings directly can significantly reduce memory overhead and improve performance, especially with large datasets.”
Sarah Thompson (Data Engineering Lead, CloudData Corp). “Memory issues during CSV to string conversion can often be resolved by adjusting the JVM’s memory settings. Increasing the heap size or using the G1 garbage collector can provide more resources for handling large data transformations without running into OutOfMemoryErrors.”
Frequently Asked Questions (FAQs)
What are common causes of memory issues when converting CSV to string in Java?
Memory issues often arise from large CSV files that require significant heap space to load and process. Inefficient data handling, such as reading the entire file into memory at once, can also contribute to excessive memory consumption.
How can I optimize memory usage when converting CSV to string in Java?
To optimize memory usage, consider using streaming techniques, such as BufferedReader, to read the file line by line instead of loading the entire file into memory. Additionally, using StringBuilder for string concatenation can help manage memory more efficiently.
Is there a specific library in Java that can help with CSV processing to avoid memory issues?
Yes, libraries like Apache Commons CSV or OpenCSV provide efficient methods for parsing CSV files, allowing for better memory management and reduced overhead during conversions.
What is the impact of using different character encodings on memory consumption during CSV conversion?
Different character encodings can affect memory usage significantly. For instance, UTF-8 can use more memory for certain characters compared to ASCII. Choosing the right encoding based on the data can help mitigate memory issues.
How can I monitor memory usage during the CSV to string conversion process in Java?
You can monitor memory usage using Java’s built-in tools like VisualVM or by programmatically checking memory usage with Runtime.getRuntime().totalMemory() and Runtime.getRuntime().freeMemory() methods during the conversion process.
What are some best practices to prevent memory overflow when handling large CSV files in Java?
Best practices include processing the file in smaller chunks, using efficient data structures, avoiding unnecessary object creation, and setting appropriate JVM memory limits. Additionally, consider using garbage collection tuning to manage memory more effectively.
Converting CSV files to strings in Java can lead to significant memory issues, particularly when dealing with large datasets. The process of reading a CSV file typically involves loading the entire file into memory, which can quickly exhaust available resources if the file size exceeds the system’s memory capacity. This can result in performance degradation, application crashes, or out-of-memory errors. Developers must be aware of these potential pitfalls when designing applications that handle CSV data.
To mitigate memory issues during the conversion process, it is advisable to implement strategies such as streaming the CSV data rather than loading it all at once. Utilizing libraries that support streaming, like Apache Commons CSV or OpenCSV, can help manage memory consumption effectively. Additionally, processing the CSV data in smaller chunks or using buffered readers can further reduce the memory footprint, allowing for more efficient handling of large files.
Another important consideration is the choice of data structures used to store the CSV data once it is converted to a string. Opting for more memory-efficient data structures can alleviate some of the strain on memory resources. Furthermore, developers should monitor memory usage during the conversion process and optimize their code to handle exceptions gracefully, ensuring that the application remains stable even under heavy loads.
Author Profile
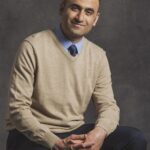
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?