How Can You Convert VARCHAR to DATE in SQL Effectively?
In the world of databases, managing data types effectively is crucial for ensuring accuracy and efficiency in data manipulation and retrieval. One common challenge that developers and database administrators face is converting `varchar` (variable character) strings into `date` formats in SQL. This conversion is not only essential for performing date-related calculations and comparisons but also for maintaining data integrity and consistency across applications. Whether you’re dealing with user input, legacy data, or external data sources, mastering this conversion can significantly enhance your SQL proficiency and streamline your database operations.
At its core, the process of converting `varchar` to `date` in SQL involves understanding the various date formats and how they can be interpreted by the database system. Different SQL dialects, such as MySQL, SQL Server, and Oracle, offer specific functions and methods for this conversion, each with its own syntax and nuances. As you navigate through these options, you’ll discover the importance of validating the input data to prevent errors and ensure that the conversion yields the expected results.
Moreover, this topic extends beyond mere syntax; it encompasses best practices for handling date formats, managing potential errors, and optimizing performance. Whether you’re a seasoned SQL developer or just starting your journey in database management, grasping the techniques for converting `varchar` to `date` will empower
Understanding Date Formats
When converting a `VARCHAR` to a `DATE` in SQL, it is crucial to understand the format of the date string you are working with. Different databases may interpret date formats differently. Common formats include:
- `YYYY-MM-DD` (ISO format)
- `MM/DD/YYYY`
- `DD/MM/YYYY`
Understanding the specific format of the string is essential to avoid errors during conversion.
Conversion Functions by Database
Different SQL databases offer various functions to convert `VARCHAR` to `DATE`. Below is a summary of some common databases and their corresponding functions:
Database | Function | Example |
---|---|---|
MySQL | STR_TO_DATE() | STR_TO_DATE(’01-12-2023′, ‘%d-%m-%Y’) |
SQL Server | CAST() or CONVERT() | CONVERT(DATE, ‘2023-12-01’, 120) |
PostgreSQL | TO_DATE() | TO_DATE(’12/01/2023′, ‘MM/DD/YYYY’) |
Oracle | TO_DATE() | TO_DATE(’01-DEC-2023′, ‘DD-MON-YYYY’) |
Handling Errors during Conversion
When converting `VARCHAR` to `DATE`, it is common to encounter errors. Here are some tips to handle these errors effectively:
- Validation: Always validate the date string format before attempting conversion. This can be achieved using regular expressions or specific functions within your SQL environment.
- Default Values: Implement default values for cases where conversion fails. This helps maintain data integrity.
- Error Handling: Utilize error handling mechanisms provided by your database system, such as `TRY…CATCH` in SQL Server, to gracefully manage conversion errors.
Example Conversions
Here are some practical examples for each database type:
- MySQL:
“`sql
SELECT STR_TO_DATE(’01-12-2023′, ‘%d-%m-%Y’) AS ConvertedDate;
“`
- SQL Server:
“`sql
SELECT CONVERT(DATE, ‘2023-12-01’, 120) AS ConvertedDate;
“`
- PostgreSQL:
“`sql
SELECT TO_DATE(’12/01/2023′, ‘MM/DD/YYYY’) AS ConvertedDate;
“`
- Oracle:
“`sql
SELECT TO_DATE(’01-DEC-2023′, ‘DD-MON-YYYY’) AS ConvertedDate FROM DUAL;
“`
Each of these examples illustrates how to convert a `VARCHAR` string into a `DATE` object, showcasing the specific syntax and functions utilized in each SQL dialect.
Methods for Converting Varchar to Date in SQL
In SQL, converting a `varchar` to a `date` type can be accomplished using several methods, depending on the database management system (DBMS) in use. Below are the most common approaches.
Using CAST and CONVERT Functions
Most SQL databases support the `CAST` and `CONVERT` functions to transform data types.
- CAST Function
- Syntax:
“`sql
SELECT CAST(column_name AS DATE) FROM table_name;
“`
- Example:
“`sql
SELECT CAST(‘2023-10-01’ AS DATE) AS ConvertedDate;
“`
- CONVERT Function (specific to SQL Server)
- Syntax:
“`sql
SELECT CONVERT(DATE, column_name, style) FROM table_name;
“`
- Example:
“`sql
SELECT CONVERT(DATE, ’01-10-2023′, 105) AS ConvertedDate; — Format DD-MM-YYYY
“`
Function | Database | Example Usage |
---|---|---|
CAST | SQL Server, MySQL, PostgreSQL | `CAST(‘2023-10-01’ AS DATE)` |
CONVERT | SQL Server | `CONVERT(DATE, ’01-10-2023′, 105)` |
Using TRY_PARSE and TRY_CONVERT Functions
These functions provide a way to safely attempt conversion, returning `NULL` if the conversion fails.
- TRY_PARSE (available in SQL Server)
- Syntax:
“`sql
SELECT TRY_PARSE(column_name AS DATE USING ‘en-US’) FROM table_name;
“`
- Example:
“`sql
SELECT TRY_PARSE(’10/01/2023′ AS DATE USING ‘en-US’) AS ConvertedDate;
“`
- TRY_CONVERT (available in SQL Server)
- Syntax:
“`sql
SELECT TRY_CONVERT(DATE, column_name) FROM table_name;
“`
- Example:
“`sql
SELECT TRY_CONVERT(DATE, ’01-10-2023′) AS ConvertedDate;
“`
Using Date Functions for Specific Formats
When dealing with non-standard date formats, date functions can help manipulate strings before conversion.
- Example with MySQL
- Using `STR_TO_DATE` to specify the format:
“`sql
SELECT STR_TO_DATE(’01-10-2023′, ‘%d-%m-%Y’) AS ConvertedDate;
“`
- Example with PostgreSQL
- Using `TO_DATE` to specify the format:
“`sql
SELECT TO_DATE(’01/10/2023′, ‘DD/MM/YYYY’) AS ConvertedDate;
“`
Function | Database | Example Usage |
---|---|---|
STR_TO_DATE | MySQL | `STR_TO_DATE(’01-10-2023′, ‘%d-%m-%Y’)` |
TO_DATE | PostgreSQL | `TO_DATE(’01/10/2023′, ‘DD/MM/YYYY’)` |
Handling Errors and Data Validation
It is essential to validate the data before conversion to avoid runtime errors. Here are some strategies:
- Check for Valid Dates:
- Use regular expressions or built-in validation functions to ensure the format is correct.
- Use CASE Statements:
- Implement `CASE` statements to handle different formats or possible errors:
“`sql
SELECT
CASE
WHEN ISDATE(column_name) = 1 THEN CAST(column_name AS DATE)
ELSE NULL
END AS ConvertedDate
FROM table_name;
“`
Utilizing these methods can streamline the process of converting `varchar` to `date` in SQL, making data handling more efficient and reliable.
Expert Insights on Converting Varchar to Date in SQL
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “When converting varchar to date in SQL, it is crucial to ensure that the string format matches the expected date format of the database. Utilizing the `CONVERT` function with the appropriate style code can significantly reduce errors during the conversion process.”
Marcus Lee (Senior SQL Developer, Data Insights Group). “One common pitfall when converting varchar to date is overlooking locale-specific date formats. Always validate the input data and consider using the `TRY_CONVERT` function to handle potential conversion failures gracefully.”
Laura Patel (Data Analyst, Analytics Pro). “It is advisable to clean and standardize your varchar data before conversion. Implementing checks for invalid dates or unexpected characters can streamline the process and enhance data integrity in your SQL queries.”
Frequently Asked Questions (FAQs)
What is the basic syntax for converting varchar to date in SQL?
To convert a varchar to date in SQL, use the `CAST` or `CONVERT` function. For example: `CAST(‘2023-10-01′ AS DATE)` or `CONVERT(DATE, ’10/01/2023’, 101)`.
What are the common formats for varchar dates in SQL?
Common formats include ‘YYYY-MM-DD’, ‘MM/DD/YYYY’, and ‘DD-MM-YYYY’. The format must match the expected date format of the SQL server to avoid conversion errors.
How do I handle conversion errors when converting varchar to date?
To handle conversion errors, use `TRY_CAST` or `TRY_CONVERT`, which return NULL instead of an error if the conversion fails. For example: `TRY_CAST(‘invalid-date’ AS DATE)`.
Can I specify a style when converting varchar to date in SQL Server?
Yes, you can specify a style using the `CONVERT` function. The third argument of `CONVERT` allows you to define the date format. For example: `CONVERT(DATE, ’01-10-2023′, 105)` specifies the Italian date format.
Is it possible to convert varchar to date in other SQL databases?
Yes, other SQL databases like MySQL and PostgreSQL also support similar conversion functions. In MySQL, use `STR_TO_DATE`, and in PostgreSQL, use `TO_DATE`.
What should I do if the varchar date format varies in my dataset?
If the varchar date format varies, consider using a combination of string manipulation functions to standardize the format before conversion. This may involve using `REPLACE`, `SUBSTRING`, or regular expressions.
Converting a varchar to a date in SQL is a common task that involves transforming string representations of dates into a date data type. This process is essential for performing date-based operations, comparisons, and calculations. Various SQL database systems, such as SQL Server, MySQL, and Oracle, offer different functions and methods to facilitate this conversion. Understanding the syntax and capabilities of these functions is crucial for ensuring accurate and efficient data manipulation.
One of the primary methods for converting varchar to date is using the `CAST` or `CONVERT` functions in SQL Server. These functions allow users to specify the desired output format, which is particularly useful when dealing with multiple date formats in the varchar data. In MySQL, the `STR_TO_DATE` function serves a similar purpose, enabling the conversion of strings to date values based on a specified format. Oracle uses the `TO_DATE` function, which also requires a format mask to interpret the input string correctly.
It is important to handle potential errors during conversion, especially when the varchar data may contain invalid date formats or values. Implementing error handling techniques, such as using conditional statements or validating input data before conversion, can prevent runtime errors and ensure data integrity. Additionally, understanding the locale and regional
Author Profile
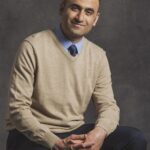
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?