How Can I Copy an Entire Array to a New Location in Studio?
In the world of programming and software development, managing data efficiently is crucial for performance and functionality. One common task that developers encounter is the need to copy an entire array to a new location in memory. This seemingly straightforward operation can significantly impact how applications run, especially when dealing with large datasets or complex structures. Whether you’re a seasoned programmer or just starting your coding journey, understanding the nuances of array manipulation is essential for optimizing your code and ensuring data integrity.
When copying an array, various factors come into play, including the programming language in use, the array’s dimensions, and the memory management techniques employed. Different programming environments offer distinct methods and functions for achieving this task, each with its own advantages and potential pitfalls. As you delve deeper into this topic, you’ll discover the importance of choosing the right approach based on your specific needs, whether that involves shallow copying, deep copying, or leveraging built-in functions.
Moreover, the implications of copying arrays extend beyond mere syntax; they touch upon performance considerations, memory allocation, and the overall architecture of your application. By exploring the various strategies for copying arrays, developers can enhance their coding practices, leading to more efficient and robust applications. In this article, we will unravel the intricacies of copying entire arrays to new locations, equ
Understanding Array Copying in Studio
When dealing with data structures in programming, copying entire arrays to a new location is a common operation. This process involves creating a duplicate of the original array in a different memory space, ensuring that modifications to the new array do not affect the original.
Methods for Copying Arrays
There are several techniques to copy arrays, depending on the programming language and the specific requirements of the task. Below are some of the most frequently used methods:
- Shallow Copy: This method copies the array’s references rather than the actual objects. It is suitable for arrays containing primitive data types.
- Deep Copy: This technique creates a complete replica of the original array along with all of its elements. It is essential when dealing with arrays that contain objects, as it ensures that each object is copied rather than just the reference.
Code Examples
Below are examples of how to copy an array in different programming languages.
Language | Shallow Copy | Deep Copy |
---|---|---|
JavaScript | let shallowCopy = originalArray.slice(); | let deepCopy = JSON.parse(JSON.stringify(originalArray)); |
Python | shallow_copy = original_array.copy() | import copy deep_copy = copy.deepcopy(original_array) |
Java | int[] shallowCopy = originalArray.clone(); | int[] deepCopy = Arrays.copyOf(originalArray, originalArray.length); |
Performance Considerations
When copying arrays, it is essential to consider the performance implications. The choice between shallow and deep copying can significantly affect memory usage and processing time. Key factors include:
- Size of the Array: Larger arrays will take more time to copy, especially if a deep copy is required.
- Complexity of Objects: For arrays containing complex objects, deep copying may require additional processing to clone each object’s properties correctly.
- Garbage Collection: In languages with automatic memory management, creating multiple copies of large arrays can lead to increased pressure on garbage collection mechanisms, potentially affecting application performance.
Best Practices
To ensure efficient array copying, consider the following best practices:
- Always assess whether you need a shallow or deep copy based on your data structure.
- Use built-in array methods provided by the language, as they are usually optimized for performance.
- Profile your application to understand the impact of array copying on performance, especially in resource-constrained environments.
By understanding these methods and considerations, developers can effectively manage array copying in their applications, leading to more efficient and maintainable code.
Copying an Entire Array to a New Location
Copying an entire array to a new memory location can be performed using various programming languages and methodologies. The approach may vary depending on the specific requirements and the environment being utilized. Here are common techniques across popular programming languages:
Using C/C++
In C and C++, you can use the `memcpy` function from the `
“`cpp
include
void copyArray(int* source, int* destination, size_t size) {
memcpy(destination, source, size * sizeof(int));
}
“`
- Parameters:
- `source`: Pointer to the source array.
- `destination`: Pointer to the destination array.
- `size`: Number of elements to copy.
Using Python
In Python, copying an array can be done using the `copy` module or list slicing.
“`python
import copy
source = [1, 2, 3, 4]
destination = copy.deepcopy(source) Using deepcopy for nested arrays
“`
Alternatively, for a flat list:
“`python
destination = source[:] List slicing
“`
- Key Methods:
- `copy.deepcopy()`: Suitable for nested data structures.
- Slicing (`[:]`): Quick method for shallow copies.
Using Java
Java provides the `System.arraycopy()` method, which is efficient for copying arrays.
“`java
public void copyArray(int[] source, int[] destination) {
System.arraycopy(source, 0, destination, 0, source.length);
}
“`
- Parameters:
- `source`: Source array.
- `destination`: Destination array.
- `0`: Starting index in the source.
- `source.length`: Number of elements to copy.
Using JavaScript
In JavaScript, the spread operator or `Array.from()` method can be used to copy arrays.
“`javascript
let source = [1, 2, 3, 4];
let destination = […source]; // Using spread operator
“`
Or:
“`javascript
let destination = Array.from(source); // Using Array.from()
“`
- Methods:
- Spread operator: Concise and modern way to copy.
- `Array.from()`: Provides additional functionality for creating new arrays.
Memory Management Considerations
When copying arrays, it is essential to consider the following:
Aspect | Description |
---|---|
Memory Allocation | Ensure the destination array is allocated properly to avoid overflow. |
Data Type | Use the correct data type for both source and destination arrays. |
Performance | For large arrays, prefer methods optimized for performance, such as `memcpy` in C/C++. |
Proper memory management and understanding of the underlying language capabilities are crucial for efficient array handling. Always test the copied arrays to ensure data integrity and correctness.
Strategies for Efficiently Copying Arrays in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When copying an entire array to a new location, it is crucial to consider the efficiency of the method used. Utilizing built-in functions like `memcpy` in C or array slicing in Python can significantly enhance performance, especially with large datasets.”
Michael Tran (Lead Developer, CodeCraft Solutions). “In modern programming environments, understanding the underlying memory management is essential. For instance, in Java, using the `System.arraycopy()` method not only simplifies the process but also optimizes memory usage, avoiding unnecessary overhead.”
Lisa Chen (Data Structures Specialist, Algorithmic Insights). “Choosing the right data structure is key when copying arrays. For example, in languages like C++, leveraging the Standard Template Library (STL) can provide efficient ways to manage and copy arrays while ensuring type safety and performance.”
Frequently Asked Questions (FAQs)
How can I copy an entire array to a new location in programming?
To copy an entire array to a new location, you can use built-in functions or methods specific to the programming language you are using. For example, in Python, you can use the `copy()` method or slicing, while in C++, you can use `std::copy()` from the `
What is the difference between shallow copy and deep copy when copying arrays?
A shallow copy creates a new array but does not copy the nested objects; instead, it references the original objects. A deep copy creates a new array and recursively copies all objects, ensuring that the new array is entirely independent of the original.
Are there any performance considerations when copying large arrays?
Yes, copying large arrays can consume significant memory and processing time. It is advisable to consider using memory-efficient techniques, such as in-place algorithms or streaming methods, depending on the application requirements.
Can I copy an array to a new location without using built-in functions?
Yes, you can manually copy an array by iterating through each element and assigning it to the new array. However, this approach may be less efficient and more error-prone than using built-in functions.
What happens if I copy an array to an incompatible data type?
If you attempt to copy an array to a new location with an incompatible data type, a runtime error may occur, or the operation may lead to data loss or corruption. It is essential to ensure type compatibility before performing the copy operation.
Is it possible to copy a multi-dimensional array to a new location?
Yes, it is possible to copy a multi-dimensional array to a new location. This typically involves nested loops to iterate through each dimension, or using specialized functions that handle multi-dimensional data structures, depending on the programming language.
In the context of programming and data manipulation, the process of copying an entire array to a new location is a fundamental operation that can significantly affect performance and memory management. Various programming languages and environments provide distinct methods for achieving this task, each with its own syntax and implications. Understanding these methods is crucial for developers who aim to optimize their code and ensure efficient data handling.
One of the primary insights is the importance of selecting the appropriate technique based on the specific requirements of the project. For instance, shallow copies may suffice in scenarios where the original and copied arrays do not need to remain independent, while deep copies are essential when the integrity of nested objects must be preserved. Additionally, the choice of method can impact the overall performance, particularly in environments with limited resources.
Furthermore, it is essential to consider the implications of copying arrays in terms of memory allocation and garbage collection. Developers must be aware of how different languages manage memory, as improper handling can lead to memory leaks or inefficient memory usage. By leveraging built-in functions or libraries designed for array manipulation, programmers can streamline the copying process and minimize potential issues.
mastering the techniques for copying arrays is vital for any developer. By understanding the nuances of shallow versus deep
Author Profile
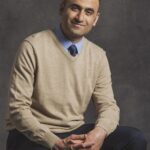
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?