Why Am I Seeing ‘Could Not Autowire: No Beans Of?’ in My Spring Application?
In the world of Spring Framework, developers often encounter a range of challenges, one of the most perplexing being the dreaded error message: “could not autowire. no beans of…”. This cryptic notification can halt progress and leave even seasoned programmers scratching their heads. Understanding the nuances of dependency injection and bean management in Spring is crucial for any developer aiming to build robust applications. In this article, we will demystify this common issue, exploring its causes and offering practical solutions to ensure your Spring application runs smoothly.
When Spring’s autowiring feature fails, it can stem from a variety of factors, including misconfigurations, missing components, or incorrect annotations. These issues can arise in different contexts, whether you’re working with a simple application or a complex microservices architecture. As we delve into the intricacies of Spring’s dependency injection mechanism, we will highlight key concepts and best practices that can help prevent such errors from occurring in the first place.
Moreover, we will examine the implications of encountering this error in real-world applications and how it can affect your development workflow. By understanding the underlying principles of Spring’s bean lifecycle and the autowiring process, you’ll be better equipped to troubleshoot and resolve these issues efficiently. Join us as we unravel the mystery behind
Understanding Autowiring in Spring
Autowiring is a powerful feature in the Spring Framework that allows developers to automatically inject dependencies into beans without explicitly defining them in the configuration files. However, the error message “could not autowire. no beans of…” indicates that Spring could not find a suitable bean to inject into the dependent class.
This issue typically arises from one of the following reasons:
- Bean Not Defined: The required bean is not defined in the Spring context.
- Incorrect Qualifier: If multiple beans of the same type exist, a specific bean needs to be qualified using the `@Qualifier` annotation.
- Component Scanning Issues: The package containing the bean is not scanned by Spring due to configuration settings.
- Scope Mismatch: The scope of the beans does not align, causing incompatibility during injection.
Common Causes of Autowiring Failures
To effectively troubleshoot autowiring failures, consider these common causes:
- Missing Bean Definition: Ensure that the bean is defined with the correct annotations, like `@Component`, `@Service`, or `@Repository`.
- Misconfigured Component Scanning: Verify that your Spring configuration is set up to scan the necessary packages. For example:
“`java
@ComponentScan(basePackages = “com.example.project”)
“`
- Ambiguous Dependencies: When multiple beans of the same type exist, use `@Qualifier` to specify which bean should be injected:
“`java
@Autowired
@Qualifier(“specificBean”)
private MyBean myBean;
“`
Best Practices for Resolving Autowiring Issues
To avoid autowiring issues, follow these best practices:
- Define all beans clearly and use annotations appropriately.
- Use `@Configuration` classes to manage bean creation and dependencies explicitly.
- Utilize `@Primary` for a default bean when multiple candidates exist.
- Regularly review component scanning settings to ensure all necessary packages are included.
Example of Autowiring
Here’s a simple example of how to correctly use autowiring in a Spring application:
“`java
@Component
public class UserService {
@Autowired
private UserRepository userRepository; // Autowired dependency
}
@Repository
public class UserRepository {
// Repository methods here
}
“`
In this example, `UserService` will automatically have `UserRepository` injected into it by Spring, provided that both classes are correctly annotated and included in the component scanning.
Troubleshooting Table
Issue | Solution |
---|---|
Bean Not Found | Check that the bean is annotated and included in the context. |
Multiple Beans of the Same Type | Use `@Qualifier` to specify which bean to inject. |
Component Scanning Not Configured | Ensure that the package containing the bean is scanned. |
Scope Issues | Align the scopes of the beans involved in the injection. |
By adhering to these practices and understanding the common pitfalls associated with autowiring, developers can mitigate issues and create more robust Spring applications.
Understanding the Autowiring Mechanism
In Spring Framework, autowiring is a feature that allows developers to automatically inject dependencies into beans. This mechanism simplifies configuration by reducing the need for explicit wiring in XML or Java configuration files. However, issues can arise, such as the “could not autowire. no beans of…” error, which indicates that the framework cannot find a suitable bean to inject.
Common Causes of the Autowiring Error
Several factors can lead to the “could not autowire” error. Understanding these causes can help in troubleshooting:
- Missing Bean Definition: The required bean may not be defined in the application context.
- Incorrect Qualifier: When multiple beans of the same type exist, the correct one may not be specified.
- Scope Mismatch: Beans with incompatible scopes (e.g., singleton vs. prototype) can lead to issues.
- Component Scanning: The package containing the bean may not be included in the component scan.
- Circular Dependencies: A cycle in dependency resolution can prevent the autowiring process from completing.
Resolving the Error
To address the autowiring issues, consider the following strategies:
- Check Bean Definitions: Ensure that the required beans are correctly defined in the context. Review configuration files or annotations.
- Use @Qualifier Annotation: Specify the intended bean when multiple candidates exist, using the @Qualifier annotation alongside @Autowired.
- Review Scopes: Verify that the scopes of the beans being injected are compatible.
- Adjust Component Scanning: Confirm that the necessary packages are included in the component scan configuration.
- Refactor Code: If circular dependencies are present, consider refactoring the code to eliminate these cycles.
Example of Autowiring with Annotations
Here’s an example of how to use autowiring effectively in a Spring application:
“`java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ServiceA {
// ServiceA’s implementation
}
@Component
public class ServiceB {
private final ServiceA serviceA;
@Autowired
public ServiceB(ServiceA serviceA) {
this.serviceA = serviceA; // Inject ServiceA
}
}
“`
In the above code snippet, ServiceB depends on ServiceA. The `@Autowired` annotation allows Spring to automatically inject the required dependency.
Using XML Configuration for Autowiring
For those using XML configuration, you can define beans and their dependencies as follows:
“`xml
“`
In this configuration, `serviceB` will automatically receive a `serviceA` instance based on type.
Best Practices for Autowiring
To avoid common pitfalls associated with autowiring, follow these best practices:
- Prefer Constructor Injection: This approach makes it clear what dependencies are required and facilitates easier testing.
- Use @Primary for Default Beans: If there are multiple beans of the same type, mark one with @Primary to indicate it should be preferred.
- Keep Context Clean: Limit the number of beans in the application context to reduce complexity and potential for errors.
- Enable Debugging: Use logging to trace dependency injection processes, which can help identify issues quickly.
By adhering to these practices, the likelihood of encountering autowiring issues can be minimized.
Understanding the Challenges of Autowiring in Spring Framework
Dr. Emily Tran (Senior Software Engineer, Spring Innovations). “The error message ‘could not autowire. no beans of…’ typically indicates that the Spring container is unable to find a suitable bean to inject. This often arises from misconfigurations in your Spring context or missing component scans.”
Mark Johnson (Lead Developer, Tech Solutions Inc.). “In many cases, this issue can be resolved by ensuring that all necessary beans are properly annotated with @Component, @Service, or similar annotations. Additionally, verifying that your configuration files are correctly set up is crucial.”
Linda Patel (Java Framework Consultant, CodeCraft). “It’s essential to be aware of the scope of your beans. A ‘could not autowire’ error may also occur if there are conflicting bean definitions or if a bean is defined in a different context than expected.”
Frequently Asked Questions (FAQs)
What does the error “could not autowire. no beans of” mean?
This error indicates that the Spring framework is unable to find a bean definition for the specified type that is required for dependency injection. It suggests that the application context does not contain a bean of the expected type.
How can I resolve the “could not autowire. no beans of” error?
To resolve this error, ensure that the bean is properly defined in your Spring configuration. Check for correct component scanning, ensure that the class is annotated with `@Component`, `@Service`, or similar, and verify that the required dependencies are included in your project.
What are common reasons for “could not autowire. no beans of”?
Common reasons include missing bean definitions, incorrect package scanning, misconfigured application context, or the absence of required libraries in the classpath. Also, ensure that the bean is not marked as `@Primary` if multiple candidates exist.
Can this error occur in a Spring Boot application?
Yes, this error can occur in a Spring Boot application if there are issues with component scanning, missing configurations, or if the bean is not instantiated correctly. Ensure that your application is set up to scan the correct packages.
How can I check if a bean is defined in the application context?
You can check if a bean is defined in the application context by using the `ApplicationContext` interface in your code. Use the `getBeanNamesForType(Class
What should I do if the bean is defined but still causes this error?
If the bean is defined but still causes the error, check for potential circular dependencies, ensure that the bean’s scope is appropriate, and verify that all required properties are correctly set. Additionally, review the logs for any initialization errors that may provide further insights.
The phrase “could not autowire. no beans of?” typically arises in the context of Spring Framework applications, particularly when dealing with dependency injection. This error indicates that the Spring container is unable to find a suitable bean to inject into a component. The issue often stems from misconfigurations, such as missing bean definitions, incorrect package scanning, or the absence of required annotations like @Component, @Service, or @Repository on the classes intended for autowiring.
Understanding the root causes of this error is essential for developers working with Spring. Common solutions include ensuring that all necessary beans are defined in the application context, verifying that component scanning is correctly set up, and checking for typos or incorrect package structures. Additionally, developers should familiarize themselves with the Spring documentation to better understand the lifecycle of beans and the autowiring process.
addressing the “could not autowire. no beans of?” error requires a systematic approach to diagnosing and resolving configuration issues within the Spring Framework. By ensuring that all components are properly annotated and that the application context is correctly configured, developers can effectively leverage Spring’s powerful dependency injection capabilities, leading to more robust and maintainable applications.
Author Profile
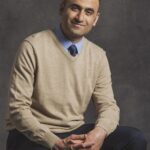
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?