Why Am I Seeing ‘Could Not Find or Load Main Class?’ and How Can I Fix It?
Have you ever sat down to run your Java application, only to be met with the frustrating message: “Could not find or load main class”? This common error can feel like a roadblock, halting your coding progress and leaving you scratching your head. Whether you’re a seasoned developer or a newcomer to the world of Java, understanding the nuances of this error is crucial for a smooth coding experience. In this article, we will unravel the mystery behind this perplexing message, exploring its causes, implications, and solutions to get you back on track.
Overview
The “could not find or load main class” error typically arises when the Java Virtual Machine (JVM) is unable to locate the entry point of your application. This can occur due to a variety of reasons, including misconfigured classpaths, missing files, or even simple typographical errors in your code. Understanding the underlying mechanics of how Java locates and executes classes can empower you to troubleshoot this issue effectively.
In addition to addressing the technical aspects, we will also delve into best practices for setting up your Java environment to minimize the chances of encountering this error in the first place. By the end of this article, you’ll not only be equipped to resolve the “could not find or load main class”
Common Causes of “Could Not Find or Load Main Class”
The error message “could not find or load main class” typically indicates that the Java Virtual Machine (JVM) is unable to locate the specified class file or that there is an issue with the classpath configuration. Understanding the common causes can help in troubleshooting this error effectively.
- Incorrect Class Name: Ensure that the class name specified in the command line is correct, including case sensitivity. Java is case-sensitive, so `MyClass` and `myclass` are treated as different classes.
- Class Not Compiled: Verify that the Java file has been compiled successfully. The `.class` file must exist in the specified directory. Use the `javac` command to compile the `.java` file, and check for any compilation errors.
- Classpath Issues: The classpath is a parameter that tells the JVM where to look for user-defined classes and packages. If the required class is not in the classpath, the JVM cannot load it. Make sure to include the current directory (.) in your classpath if the class is in the same directory as your command prompt.
- File Structure: If your class is part of a package, ensure that the directory structure matches the package declaration. For example, if your class is in the package `com.example`, the file must be located in `com/example/MyClass.class`.
- JAR File Problems: If you’re trying to run a class from a JAR file, ensure that the JAR file is not corrupted and that it contains the required class. Use the command `jar tf yourfile.jar` to list the contents of the JAR file.
- Environment Variables: Ensure that the JAVA_HOME environment variable is set correctly, pointing to your JDK installation, and that the PATH variable includes the JDK’s `bin` directory.
Troubleshooting Steps
To resolve the “could not find or load main class” error, follow these troubleshooting steps:
- Verify that the class name and package structure are correct.
- Ensure that the `.class` file exists and is compiled properly.
- Check the classpath for correctness:
- Use the `-cp` option to specify the classpath explicitly.
- For example: `java -cp . com.example.MyClass`
- If using a JAR file, confirm that the JAR contains the expected class:
- Run `jar tf yourfile.jar` to check its contents.
- Review environment variables and ensure they are configured properly.
Issue | Solution |
---|---|
Class name incorrect | Double-check spelling and case sensitivity. |
Class not compiled | Compile the Java file using `javac`. |
Classpath not set | Add the correct path using `-cp` option. |
JAR file issues | Verify the JAR content and integrity. |
Environment variable misconfiguration | Check and update JAVA_HOME and PATH variables. |
By systematically addressing each of these potential issues, you can effectively resolve the “could not find or load main class” error and ensure that your Java application runs smoothly.
Understanding the Error
The error message “could not find or load main class” typically indicates that the Java Virtual Machine (JVM) is unable to locate the specified class file required for executing a Java application. This can arise due to various reasons, including incorrect classpath settings, missing files, or typographical errors in class names.
Common Causes
Several factors can contribute to this error. Understanding these can help in troubleshooting the issue effectively:
- Incorrect Classpath: The classpath is a parameter that tells the JVM where to look for user-defined classes and packages. If it is set incorrectly, the JVM may not find the necessary classes.
- Typographical Errors: Mistakes in the class name or package name can lead to this issue. Java is case-sensitive, and any mismatch can result in an error.
- File Not Found: The specified class file may not exist in the expected directory. Ensure that the file is in the correct location.
- Compiling Issues: If the class was not compiled correctly, it may not produce the `.class` file needed by the JVM.
- JAR File Issues: When using JAR files, if the JAR is not included in the classpath or does not contain the required class, this error will occur.
Troubleshooting Steps
To resolve the “could not find or load main class” error, follow these troubleshooting steps:
- Check Classpath Configuration:
- Ensure that the classpath is set correctly.
- Use the `-cp` or `-classpath` option when running your Java application.
- Verify Class Name and Package:
- Double-check the class name for any typographical errors.
- Ensure the package structure matches the directory structure.
- Ensure File Exists:
- Confirm that the `.class` file is present in the specified directory.
- Use commands such as `ls` (Unix/Linux) or `dir` (Windows) to list files.
- Compile the Class:
- If the class is not compiled, use `javac ClassName.java` to compile it.
- Check for any compilation errors that may prevent the `.class` file from being generated.
- Check for JAR File Issues:
- If using a JAR, ensure it is included in the classpath.
- Use the command `java -jar yourfile.jar` for execution if appropriate.
Example Scenarios
The following table outlines common scenarios and their solutions:
Scenario | Solution |
---|---|
Classpath not set | Add the classpath using `-cp` or `-classpath`. |
Typo in class name | Correct the spelling in the command line. |
Class file missing | Ensure the `.class` file exists in the directory. |
Compilation failure | Compile the Java file again and check for errors. |
JAR file not containing the class | Verify that the required class is inside the JAR. |
Best Practices
To minimize the chances of encountering this error, consider the following best practices:
- Use IDEs: Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse can manage classpaths automatically, reducing human error.
- Consistent Naming Conventions: Follow consistent naming conventions for packages and classes to avoid confusion.
- Version Control: Utilize version control systems to keep track of changes and ensure that files are not inadvertently deleted or moved.
- Documentation: Maintain clear documentation of project structure and dependencies to aid in troubleshooting.
By following these guidelines, developers can mitigate the occurrence of the “could not find or load main class” error and streamline the execution of Java applications effectively.
Resolving Java Class Loading Issues
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The error message ‘could not find or load main class’ typically indicates that the Java Virtual Machine (JVM) is unable to locate the specified class file. This often occurs due to incorrect classpath settings or a missing .class file. Ensuring that the classpath is correctly set and that the necessary files are present in the specified directories is crucial for resolving this issue.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When encountering the ‘could not find or load main class’ error, it is essential to verify that the main class is correctly defined in your code and that it matches the file name. Additionally, checking for typos in the class name and ensuring that the package structure aligns with the directory structure can help eliminate this common pitfall.”
Sarah Patel (Java Programming Instructor, Online Learning Academy). “Students often face the ‘could not find or load main class’ error when they are new to Java. I advise them to familiarize themselves with the command line options for compiling and running Java applications. Understanding how to set the classpath and using the correct syntax can significantly reduce the likelihood of encountering this error during development.”
Frequently Asked Questions (FAQs)
What does “could not find or load main class” mean?
This error indicates that the Java Virtual Machine (JVM) is unable to locate the specified main class to execute. This can occur due to incorrect class names, missing class files, or issues with the classpath configuration.
What are common causes of this error?
Common causes include misspelled class names, incorrect directory structure, missing .class files, and improper classpath settings that do not point to the directory containing the compiled classes.
How can I fix the “could not find or load main class” error?
To resolve this error, ensure that the class name is spelled correctly, verify that the .class file exists in the expected directory, and check that the classpath is correctly set to include the directory containing the class files.
What is the role of the classpath in Java?
The classpath is a parameter that tells the JVM where to look for user-defined classes and packages. It can include directories, JAR files, and ZIP files that contain the necessary class files for execution.
Can this error occur with JAR files?
Yes, this error can occur with JAR files if the manifest file does not specify the correct main class or if the JAR file is not included in the classpath when executing the Java application.
Is there a way to debug this error effectively?
Yes, debugging can be done by checking the command line used to run the Java application, ensuring the correct classpath is set, and using verbose mode with the `-verbose:class` option to see which classes are being loaded and from where.
The error message “could not find or load main class” is a common issue encountered by Java developers when attempting to run Java applications. This error typically arises due to several reasons, including incorrect classpath settings, the absence of the specified class file, or issues with the Java installation itself. Understanding the underlying causes of this error is crucial for effective troubleshooting and ensuring that Java applications run smoothly.
One of the primary factors contributing to this error is the misconfiguration of the classpath. The classpath is a parameter that tells the Java Virtual Machine (JVM) where to look for user-defined classes and packages. If the classpath is not set correctly, the JVM will be unable to locate the main class, resulting in the error message. Developers should verify their classpath settings and ensure that they point to the correct directories containing the necessary class files.
Additionally, the absence of the main class file in the specified location can lead to this error. It is essential to ensure that the compiled class files are present and correctly named. Furthermore, developers should check for typos in the class name or package structure, as these can also prevent the JVM from locating the main class. Lastly, issues with the Java installation, such as corrupted files or
Author Profile
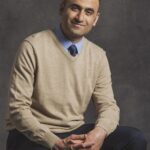
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?