Could Not Initialize Proxy – No Session? Understanding the Common Causes and Solutions
In the realm of software development, particularly when working with object-relational mapping (ORM) frameworks like Hibernate, encountering errors can be both frustrating and enlightening. One such error that developers often face is the perplexing message: “could not initialize proxy – no session?” This seemingly cryptic notification can halt progress and leave even seasoned programmers scratching their heads. Understanding this error is crucial for anyone looking to streamline their application performance and enhance their debugging skills. In this article, we will unravel the complexities behind this issue, exploring its causes, implications, and potential solutions.
At its core, the “could not initialize proxy – no session?” error arises when an attempt is made to access a lazily-loaded entity outside of an active Hibernate session. This situation typically occurs in scenarios involving detached entities or when the session has been closed prematurely. The implications of this error can be significant, leading to application crashes or unexpected behavior if not addressed properly. By delving into the mechanics of Hibernate sessions and the lifecycle of entities, developers can gain a clearer perspective on how to manage their data interactions effectively.
Moreover, the nuances of session management are vital for optimizing performance and ensuring data integrity within applications. As we explore the various aspects of this error, we will highlight best practices for session handling, strategies for
Understanding the Proxy Initialization Error
The error message “could not initialize proxy – no session?” typically occurs in ORM (Object-Relational Mapping) frameworks, such as Hibernate in Java or similar technologies in other programming languages. This issue arises when an attempt is made to access a lazy-loaded entity outside of a valid session context, which can lead to complications in data retrieval and application functionality.
When an ORM framework uses lazy loading, it defers the loading of an object’s data until it is explicitly requested. This is done to enhance performance by reducing unnecessary database queries. However, if the session that was responsible for loading the data is closed or not available, the framework will not be able to initialize the proxy object, resulting in this error.
Common Causes
Several factors contribute to the occurrence of this error:
- Session Management: The session may have been closed before the proxy object was accessed. Proper session management is crucial to avoid this issue.
- Transaction Boundaries: If a transaction is not properly scoped, accessing a lazy-loaded entity outside of the transaction can lead to this error.
- Detached Entities: An entity that was once managed within a session but has since been detached may not have access to its lazy-loaded properties.
Solutions and Best Practices
Addressing the “could not initialize proxy – no session?” error requires careful consideration of session management and entity lifecycle. Here are some strategies to mitigate this problem:
- Open Session in View Pattern: This pattern keeps the session open for the duration of the request, allowing lazy loading to function correctly.
- Eager Loading: Instead of relying on lazy loading, configure your ORM to eagerly fetch the required associations, thus avoiding the need for proxies altogether.
- Properly Scoped Transactions: Ensure that transactions are appropriately scoped to encompass the entire data retrieval process.
Strategy | Description | Pros | Cons |
---|---|---|---|
Open Session in View | Keeps the session open for the duration of the request. | Facilitates lazy loading without session issues. | Can lead to resource leaks if not managed properly. |
Eager Loading | Fetches associated entities immediately. | Avoids proxy initialization issues. | May lead to performance overhead by loading more data than necessary. |
Properly Scoped Transactions | Ensures transactions cover all necessary data operations. | Reduces the risk of detached entities. | Requires careful management of transaction boundaries. |
By implementing these strategies, developers can significantly reduce the occurrence of the “could not initialize proxy – no session?” error and enhance the overall stability of their applications. Understanding the underlying mechanics of session management and entity loading can lead to more effective troubleshooting and resolution of related issues.
Understanding the Error: “Could Not Initialize Proxy – No Session”
The error message “could not initialize proxy – no session” typically occurs in the context of object-relational mapping (ORM) frameworks, such as Hibernate. This error is indicative of a failed attempt to lazily load a proxy object due to an inactive or closed session.
Common Causes
- Session Management Issues: The session in which the proxy is being accessed has already been closed or was never opened.
- Lazy Loading: The application is trying to access an entity that is not loaded yet, and the session that could load it is no longer available.
- Transaction Boundaries: The boundaries of a transaction may not be properly defined, causing the session to close prematurely.
Resolution Strategies
To address the “could not initialize proxy – no session” error, consider the following strategies:
- Ensure Active Session: Always check that the session is active before accessing any proxy objects. This can be done by managing session lifecycle properly.
- Eager Fetching: Change the fetching strategy from lazy to eager if accessing the associated entity is unavoidable:
“`java
@OneToMany(fetch = FetchType.EAGER)
private Set
“`
- Open Session in View Pattern: Implement this pattern to keep the session open during the view rendering phase, particularly in web applications.
Best Practices for Session Management
Best Practice | Description |
---|---|
Use a Session Factory | Create a session factory that can be reused to obtain sessions. |
Close Sessions Properly | Always close sessions in a finally block to ensure they are released. |
Use Transaction Boundaries | Clearly define transaction boundaries to manage session lifespan effectively. |
Debugging Steps
When encountering this error, follow these debugging steps:
- Check Session State: Confirm if the session is still open when accessing the proxy object.
- Review Transaction Management: Ensure that transactions are properly defined and that the session is not being closed unexpectedly.
- Enable Logging: Enable detailed logging for the ORM tool to trace session and transaction states.
Example Scenario
Consider a scenario where a web application retrieves a user and then tries to access the user’s addresses after the session has been closed. The following code illustrates the potential issue:
“`java
Session session = sessionFactory.openSession();
User user = session.get(User.class, userId);
session.close(); // Session closed here
String firstAddress = user.getAddresses().get(0); // Error occurs here
“`
To fix this, ensure that the session remains open while accessing the addresses, or utilize eager loading.
Conclusion
Addressing the “could not initialize proxy – no session” error requires a solid understanding of session management and ORM behavior. By following the outlined strategies and best practices, developers can prevent and resolve this common issue effectively.
Understanding Proxy Initialization Issues in Software Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘could not initialize proxy – no session?’ typically arises in ORM frameworks when there is an attempt to access a lazy-loaded entity outside of an active session. It’s crucial for developers to ensure that their session management is robust and that they are properly handling the lifecycle of their entities to avoid such issues.”
Michael Chen (Lead Backend Developer, CodeCraft Solutions). “This error often indicates a misconfiguration in the database session handling. Developers should verify that their transaction boundaries are correctly defined and that the session is still active when attempting to access proxies. Implementing proper error handling can also help diagnose and resolve these issues more effectively.”
Lisa Patel (Database Architect, DataSecure Technologies). “In many cases, ‘could not initialize proxy – no session?’ is a symptom of a deeper architectural flaw. It is essential to review the design of the application to ensure that sessions are being managed appropriately, especially in distributed systems where session persistence can be challenging.”
Frequently Asked Questions (FAQs)
What does the error “could not initialize proxy – no session?” mean?
This error typically indicates that an attempt was made to access a proxy object in an ORM (Object-Relational Mapping) context, but there is no active session to initialize it. This often occurs when the session has been closed or was never opened.
What causes the “could not initialize proxy – no session?” error?
The error is usually caused by accessing a lazily loaded entity outside of an active database session. This can happen if the session is closed or if the entity is being accessed after the session has ended.
How can I resolve the “could not initialize proxy – no session?” error?
To resolve this error, ensure that the session is open when accessing the proxy object. You can either keep the session open longer or eagerly load the necessary data before closing the session.
Is this error specific to certain programming languages or frameworks?
Yes, this error is commonly associated with ORM frameworks such as Hibernate in Java, Entity Framework in .NET, and similar libraries in other languages that use session management for database interactions.
Can I prevent the “could not initialize proxy – no session?” error from occurring?
Yes, you can prevent this error by managing your session lifecycle properly. Always ensure that the session is active when working with entities, and consider using eager fetching strategies where appropriate.
Are there any best practices for handling sessions to avoid this error?
Best practices include using a session-per-request pattern, ensuring that all database operations are completed within the session’s scope, and implementing proper error handling to manage session states effectively.
The error message “could not initialize proxy – no session” typically arises in the context of object-relational mapping (ORM) frameworks, particularly when using Hibernate or similar technologies. This issue often indicates that an attempt is being made to access a lazily loaded entity outside of an active session. In ORM, lazy loading is a strategy that delays the loading of an object until it is needed, which can lead to complications if the session that manages the entity is already closed.
One of the primary causes of this error is the improper management of session lifecycle in the application. Developers must ensure that the session is open and active when attempting to access lazy-loaded properties. This can be achieved by either keeping the session open for the duration of the data access or by utilizing techniques such as Open Session in View, which allows the session to remain open during the rendering of the view.
Another valuable insight is the importance of understanding the implications of lazy loading and session management in ORM. Developers should be aware of the context in which entities are being accessed and consider using eager loading when necessary to avoid session-related issues. Additionally, implementing proper exception handling can help in gracefully managing situations where a session is not available, thereby improving the robustness of the application.
Author Profile
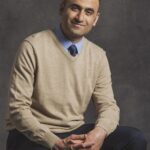
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?