Why Am I Getting ‘Could Not Locate Runnable Browser’ Error in Python?
In the ever-evolving landscape of software development, encountering roadblocks can be frustrating, especially when it comes to executing automated tasks in web browsers. One common issue that many developers face is the error message: “could not locate runnable browser.” This seemingly cryptic notification can halt progress and leave even seasoned programmers scratching their heads. Understanding the root causes of this problem and how to resolve it is essential for anyone looking to streamline their web automation processes using Python.
When working with web automation libraries like Selenium, the ability to launch a browser seamlessly is crucial. However, various factors can lead to the dreaded “could not locate runnable browser” error. From misconfigured paths to incompatible versions of web drivers and browsers, the reasons can vary widely. This article will delve into the common pitfalls that lead to this issue, providing insights into how to diagnose and fix them effectively.
Moreover, we will explore best practices for setting up your development environment to minimize the risk of encountering this error in the future. By equipping yourself with the knowledge and tools to address this challenge, you can enhance your productivity and focus on what truly matters—building robust and efficient web applications. Join us as we navigate the intricacies of browser automation in Python and uncover solutions that will keep your projects on
Troubleshooting Browser Location Issues
When encountering the error message “could not locate runnable browser” in Python, it typically indicates that the script is unable to find a web browser installation to execute automated tasks. This issue can arise due to several factors, including misconfiguration, missing dependencies, or environmental settings.
To troubleshoot this problem, consider the following steps:
- Verify Browser Installation: Ensure that a compatible web browser is installed on your machine. Common choices include Google Chrome, Mozilla Firefox, and Microsoft Edge.
- Check Path Environment Variable: The system needs to know where to find the browser executable. If the path is not set correctly, the script will fail to locate the browser.
- Use Browser Driver: For automation with Selenium or similar libraries, ensure that the appropriate browser driver (e.g., ChromeDriver for Chrome, GeckoDriver for Firefox) is installed and accessible in your system’s PATH.
- Install Required Packages: Make sure that you have installed all required Python packages, such as Selenium or Pyppeteer, which facilitate browser automation. Use pip to install any missing packages:
“`bash
pip install selenium
pip install pyppeteer
“`
- Check for Compatibility Issues: Ensure that the versions of the browser and the browser driver are compatible with each other. Mismatched versions can lead to execution failures.
Configuring Web Drivers
To set up a web driver correctly, follow these guidelines:
- **Download the Appropriate Driver**:
- For Chrome: [ChromeDriver](https://sites.google.com/chromium.org/driver/)
- For Firefox: [GeckoDriver](https://github.com/mozilla/geckodriver/releases)
- **Add Driver to PATH**:
- On Windows:
- Right-click on ‘This PC’ > Properties > Advanced system settings > Environment Variables.
- Under System Variables, find Path, and click Edit. Add the path to the driver executable.
- On macOS/Linux:
- Open terminal and edit your shell profile (e.g., `.bashrc`, `.zshrc`) to include:
“`bash
export PATH=$PATH:/path/to/driver
“`
- Confirm Driver Accessibility:
- Run a simple script to check if the driver is accessible. For example, in Python:
“`python
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(‘http://www.google.com’)
“`
Common Errors and Fixes
Below is a table outlining common errors related to browser automation and their respective fixes:
Error Message | Possible Cause | Solution |
---|---|---|
could not locate runnable browser | Browser not installed or not found in PATH | Install the browser and set the correct PATH |
SessionNotCreatedException | Browser version incompatible with driver | Update the browser or driver to compatible versions |
WebDriverException | Driver executable not found | Ensure the driver is in PATH and correctly referenced |
By systematically addressing these areas, you can resolve the “could not locate runnable browser” error and ensure that your Python scripts can successfully automate browser tasks.
Common Causes of “Could Not Locate Runnable Browser” Error
When encountering the “could not locate runnable browser” error in Python, it typically arises from several common issues. Understanding these causes can help in effectively troubleshooting the problem.
- Missing Browser Installation: The error may occur if the specified browser is not installed on your system.
- Incorrect Browser Path: If the path to the browser executable is incorrectly specified in your script or configuration, it can lead to this error.
- Environment Variables: The system may lack the necessary environment variables that point to the browser’s executable.
- Python Packages: Outdated or improperly installed packages, particularly those related to web automation (like Selenium), can also trigger this issue.
- Operating System Compatibility: Sometimes, the version of the browser may not be compatible with the Python package or the operating system being used.
Troubleshooting Steps
To resolve the “could not locate runnable browser” error, follow these troubleshooting steps:
- Check Browser Installation:
- Ensure that the browser you intend to use (e.g., Chrome, Firefox) is installed.
- Verify the browser version and update if necessary.
- Verify Browser Path:
- Confirm that the path to the browser executable is correctly specified in your code.
- Example for Chrome in Python:
“`python
from selenium import webdriver
driver = webdriver.Chrome(executable_path=’/path/to/chromedriver’)
“`
- Set Environment Variables:
- Check if the environment variables are set correctly:
- For Windows, add the browser’s installation path to the `PATH` variable.
- For macOS/Linux, you can export the path in your shell configuration file (e.g., `.bashrc` or `.zshrc`).
- Update Python Packages:
- Ensure that all relevant Python packages are up to date. You can use:
“`bash
pip install –upgrade selenium
“`
- Confirm Compatibility:
- Verify compatibility between the browser version, the WebDriver, and the operating system. Check the documentation for any specific requirements.
Example of Setting Up Selenium with Chrome
To provide a clear example, here’s how to set up Selenium with Chrome to avoid the “could not locate runnable browser” error:
“`python
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
Setup Chrome driver using webdriver_manager
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
Open a website
driver.get(“https://www.example.com”)
Perform your tasks…
Close the browser
driver.quit()
“`
This code ensures that the appropriate ChromeDriver is downloaded and utilized, reducing the chances of encountering path-related issues.
Tools for Verification
Utilizing certain tools can help verify your setup:
Tool | Purpose |
---|---|
WebDriver Manager | Automatically manages driver binaries for different browsers. |
Browser Version Check | Websites like [whatsmybrowser.org](https://www.whatsmybrowser.org/) can help verify your browser version and settings. |
Path Validation Scripts | Use scripts to validate if paths are set correctly in your environment. |
By methodically addressing the identified causes, you can effectively resolve the “could not locate runnable browser” error and ensure smooth execution of your Python automation scripts.
Resolving Browser Issues in Python Development
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “The error ‘could not locate runnable browser’ typically arises when the web driver is not properly configured or the browser itself is not installed. Ensuring that the correct version of the web driver matches your browser version is crucial for seamless execution.”
Mark Thompson (Lead Automation Tester, Quality Assurance Experts). “When encountering the ‘could not locate runnable browser’ error, it is essential to verify your environment variables. The path to the browser executable must be correctly set in your system’s PATH variable to allow Python scripts to locate and launch the browser.”
Linda Zhang (Python Developer, Open Source Advocate). “This issue can also stem from using an outdated or incompatible library. Regularly updating libraries like Selenium and ensuring compatibility with your browser version can prevent such errors from occurring during automated testing.”
Frequently Asked Questions (FAQs)
What does “could not locate runnable browser” mean in Python?
This error indicates that the Python script or application is unable to find a compatible web browser installed on the system to execute certain tasks, such as opening a URL or running automated tests.
How can I resolve the “could not locate runnable browser” error?
To resolve this error, ensure that a supported web browser, such as Chrome, Firefox, or Safari, is installed on your machine. Additionally, verify that the browser’s path is correctly set in your system’s environment variables.
Which Python libraries commonly require a browser to be runnable?
Libraries such as Selenium, Splinter, and Pyppeteer often require a runnable browser to perform web automation and testing tasks effectively.
How do I check if my browser is correctly configured for Python?
You can check your browser configuration by running a simple script that attempts to launch the browser. If the script fails with the “could not locate runnable browser” error, review the installation and path settings for the browser.
Can I use a headless browser to avoid this error?
Yes, using a headless browser, such as Headless Chrome or PhantomJS, can bypass the need for a graphical user interface. Ensure that the headless browser is installed and properly configured in your Python script.
What should I do if I have multiple browsers installed?
If multiple browsers are installed, specify the desired browser in your Python script or configuration file. This can often be done by setting the appropriate driver or executable path for the browser you intend to use.
The issue of “could not locate runnable browser” in Python typically arises when attempting to automate web browsing tasks using libraries such as Selenium. This error indicates that the script is unable to find a compatible web browser executable on the system. The root cause may stem from several factors, including an incorrect installation of the browser, the absence of the required browser driver, or misconfigured environment variables that prevent the script from locating the browser executable.
To resolve this issue, users should first ensure that the desired web browser, such as Chrome or Firefox, is installed correctly on their system. Following this, it is crucial to download and install the corresponding web driver that matches both the browser version and the operating system. For instance, if using Chrome, the ChromeDriver must be compatible with the installed version of Chrome. Additionally, users should verify that the path to the browser executable is correctly set in their environment variables or explicitly defined in their Python script.
Key takeaways from this discussion emphasize the importance of compatibility between the web browser and its driver, as well as the necessity of proper configuration. Users should always check for updates to both the browser and the driver to avoid version mismatches. Furthermore, consulting the documentation for the specific automation library being used can provide
Author Profile
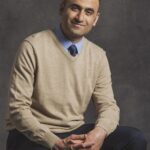
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?