Why Could I Not Open JPA EntityManager for Transaction?
In the world of Java Persistence API (JPA), developers often encounter a variety of challenges that can disrupt the flow of their applications. One such challenge is the perplexing error message: “could not open JPA EntityManager for transaction.” This issue can be a significant roadblock, hindering the ability to interact with the database and manage entities effectively. For developers, understanding the root causes of this error is crucial, as it can lead to frustrating debugging sessions and delays in project timelines. In this article, we will explore the common pitfalls associated with this error, providing insights and solutions to help you navigate these turbulent waters with confidence.
At its core, the error message indicates a failure in establishing a connection to the JPA EntityManager, which is essential for executing database operations. This can stem from a variety of factors, including configuration issues, incorrect transaction management, or even problems with the underlying database itself. As developers dive deeper into the intricacies of JPA, they may find themselves grappling with the nuances of persistence contexts, transaction boundaries, and the lifecycle of entities—all of which can contribute to this frustrating error.
Understanding the context in which this error arises is vital for effective troubleshooting. By examining the common scenarios that lead to this issue, developers can better equip themselves with
Common Causes of EntityManager Issues
One of the frequent problems developers encounter with JPA (Java Persistence API) is the inability to open an `EntityManager` for transactions. Understanding the underlying causes can significantly help in troubleshooting these issues. Below are some common reasons:
- Incorrect Persistence Unit Configuration: The persistence.xml file may have incorrect settings, such as the wrong database connection URL, username, or password.
- Contextual Issues: If the `EntityManager` is not being created within the appropriate context, such as a managed environment (like a Java EE container), it could lead to failures.
- Resource Exhaustion: Database connection limits can be reached, which prevents new connections from being established.
- Transaction Management Problems: Misconfigured transaction management, either through annotations or XML configuration, can prevent the `EntityManager` from functioning correctly.
Debugging Steps for EntityManager Issues
When faced with the problem of opening an `EntityManager`, it’s essential to follow a systematic approach to debugging. Here are key steps to consider:
- Check Configuration Files:
- Verify the `persistence.xml` for correct settings.
- Ensure the database driver is correctly specified.
- Review Application Logs:
- Look for stack traces or error messages in the application logs that could indicate the root cause.
- Test Database Connectivity:
- Use a database client to ensure the application can connect to the database using the same credentials.
- Examine Resource Allocation:
- Monitor database connections to ensure that the connection pool is not exhausted.
- Validate Transaction Settings:
- Ensure that transaction management is correctly configured for your environment, whether using JTA or resource-local transactions.
Configuration Example
Here is an example of how a typical `persistence.xml` might look:
“`xml
Best Practices for Managing EntityManager
To avoid issues with `EntityManager`, consider the following best practices:
- Use Dependency Injection: Leverage frameworks like Spring or CDI to manage `EntityManager` instances, promoting better lifecycle management.
- Handle Transactions Properly: Always ensure that transactions are properly started, committed, or rolled back to avoid resource leaks.
- Close EntityManager: If using resource-local transactions, always close the `EntityManager` after use to free resources.
- Monitor and Optimize Performance: Regularly review performance metrics and optimize queries and connection pooling configurations.
Issue | Potential Solution |
---|---|
Incorrect Configuration | Review persistence.xml for correctness |
Connection Pool Exhausted | Increase connection pool size |
Transaction Not Managed | Ensure proper transaction annotations are used |
Common Causes of EntityManager Issues
The inability to open a JPA EntityManager for transactions can stem from various issues. Understanding these causes can help in troubleshooting effectively.
- Configuration Errors:
- Incorrect persistence.xml settings.
- Missing or misconfigured data source properties.
- EntityManager Factory Issues:
- Not initializing the EntityManagerFactory properly.
- Resource leaks or failure to close EntityManager instances.
- Transaction Management Problems:
- Using an unsupported transaction management strategy.
- Misconfigurations in Spring or Java EE transaction management.
- Database Connectivity:
- Database server downtime or network issues.
- Incorrect database URL, username, or password.
Troubleshooting Steps
To resolve the issue of being unable to open an EntityManager, follow these troubleshooting steps:
- Check Configuration Files:
- Review `persistence.xml` for accuracy.
- Ensure all required properties are defined correctly.
- Validate EntityManagerFactory Initialization:
- Confirm that the EntityManagerFactory is being created and initialized correctly in your application.
- Verify that you are not attempting to use the EntityManager before the factory is fully set up.
- Examine Transaction Management:
- Ensure the transaction management annotations or XML configurations align with your chosen framework (e.g., Spring).
- Check for proper use of `@Transactional` where needed.
- Test Database Connection:
- Use standalone tools (like DBeaver or SQL Workbench) to test the database connection independently.
- Check for any SQLExceptions that might indicate connectivity issues.
Example Configuration
A typical `persistence.xml` for a JPA application might look as follows:
“`xml
Best Practices for Managing EntityManager
To avoid issues with EntityManager, consider implementing the following best practices:
- Scope and Lifecycle Management:
- Use dependency injection to manage the lifecycle of the EntityManager.
- Close EntityManager instances in a `finally` block or use try-with-resources.
- Error Handling:
- Implement robust error handling to catch and log exceptions related to EntityManager operations.
- Performance Optimization:
- Avoid opening and closing EntityManager instances frequently. Use a context or session-based approach where appropriate.
- Testing:
- Write unit and integration tests to ensure EntityManager operations work as expected in various scenarios.
By adhering to these strategies, you can significantly reduce the likelihood of encountering issues with your JPA EntityManager during transaction handling.
Expert Insights on JPA EntityManager Transaction Issues
Dr. Emily Carter (Senior Software Architect, Java Development Institute). “The error ‘could not open JPA EntityManager for transaction’ typically arises due to misconfigurations in the persistence context. It is crucial to ensure that the EntityManagerFactory is properly initialized and that the transaction management settings align with the application server’s requirements.”
Michael Chen (Lead Backend Developer, Enterprise Solutions Corp). “In my experience, this issue often stems from a failure to establish a connection to the database. Verifying the database URL, credentials, and network accessibility can resolve the problem. Additionally, checking the transaction boundaries in your code is essential to prevent this error.”
Sarah Thompson (JPA Expert Consultant, Tech Innovations Group). “It’s important to consider the context in which the EntityManager is being used. If the application is running in a multi-threaded environment, ensure that the EntityManager is not being shared across threads, as this can lead to transaction conflicts and the inability to open the EntityManager.”
Frequently Asked Questions (FAQs)
What does “could not open JPA EntityManager for transaction” mean?
This error indicates that the Java Persistence API (JPA) EntityManager could not be initialized, preventing the application from starting a transaction. This can occur due to misconfiguration, resource unavailability, or issues with the persistence context.
What are common causes of this error?
Common causes include incorrect database connection settings, failure to load the persistence unit, issues with the underlying database (e.g., server down), or misconfigured transaction management settings in the application.
How can I troubleshoot this issue?
To troubleshoot, verify the database connection details in the persistence.xml file, ensure the database server is running, check for exceptions in the logs, and confirm that the JPA provider is correctly set up in your application.
Is this error related to my database configuration?
Yes, this error is often related to database configuration. Ensure that the database URL, username, password, and driver class are correctly specified in your configuration files.
Can this error occur in a Spring Boot application?
Yes, this error can occur in a Spring Boot application if the JPA configuration is incorrect, the application context fails to load, or there are issues with the database connection pool.
What steps can I take to prevent this error in the future?
To prevent this error, regularly review and test your database configurations, ensure proper exception handling in your code, and monitor the health of your database server. Additionally, keep your dependencies up to date to avoid compatibility issues.
The issue of being unable to open a JPA EntityManager for a transaction is a common challenge faced by developers working with Java Persistence API (JPA). This problem typically arises due to misconfigurations in the persistence context, issues with the underlying database connection, or incorrect transaction management settings. Understanding the root causes of this issue is crucial for effective troubleshooting and resolution.
One of the primary factors contributing to this problem is the configuration of the EntityManagerFactory. Developers must ensure that the persistence.xml file is correctly set up with the appropriate database connection properties. Additionally, issues such as network connectivity problems, database server downtime, or incorrect credentials can prevent the EntityManager from being opened successfully.
Another important aspect to consider is the transaction management strategy being employed. Inconsistent transaction boundaries or improper handling of transactions can lead to failures when attempting to open an EntityManager. It is essential to adhere to best practices for managing transactions, such as using container-managed transactions or properly configuring the EntityManager in a Spring application.
In summary, addressing the inability to open a JPA EntityManager for a transaction requires a thorough examination of both configuration settings and transaction management practices. By ensuring that all components are correctly configured and that best practices are followed,
Author Profile
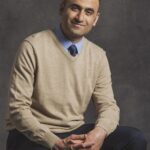
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?