How Can I Create a PR to Update a File’s Value in Go?
In the fast-paced world of software development, collaboration and version control are paramount. For developers working with Go (Golang), the process of updating files and managing changes can often feel daunting, especially when it comes to contributing to a project through pull requests (PRs). Whether you’re a seasoned Go developer or just starting out, understanding how to effectively create a PR to update the value of a file is a crucial skill that can enhance your workflow and improve team collaboration. In this article, we will delve into the intricacies of crafting a pull request that not only updates file values but also adheres to best practices in version control.
Creating a pull request in Golang involves a series of steps that ensure your changes are clearly communicated and easily integrated into the main codebase. From cloning the repository to making modifications and finally submitting your PR, each step plays a vital role in the development process. This article will guide you through the essential practices for preparing your changes, including how to write meaningful commit messages and provide context for reviewers.
Moreover, we will explore the importance of testing your changes before submission, which is critical in maintaining code quality and functionality. By the end of this article, you will have a solid understanding of how to create a pull request that not only updates the value
Creating a Pull Request in Go to Update a File
In order to create a pull request (PR) that updates the value of a file in a Go project, you need to follow a systematic approach. This involves modifying the file in your local repository, committing the changes, and then pushing the changes to a remote branch before creating the PR. Below are the steps involved in this process.
Step-by-Step Process
- Clone the Repository: Begin by cloning the repository to your local machine if you haven’t done so already. Use the following command:
“`bash
git clone https://github.com/username/repo.git
cd repo
“`
- Create a New Branch: It’s essential to create a new branch for your changes to keep the main branch stable. Use a descriptive name for the branch.
“`bash
git checkout -b update-file-value
“`
- Modify the File: Open the file you intend to update using your preferred text editor and make the necessary changes. For example, if you’re updating a configuration value in a Go file, locate the appropriate line and update it.
- Stage the Changes: After making the necessary modifications, stage the changes using:
“`bash
git add path/to/your/file.go
“`
- Commit the Changes: Next, commit your changes with a clear message that explains what you’ve done.
“`bash
git commit -m “Update value in file.go to reflect new configuration”
“`
- Push the Branch to Remote: Once you’ve committed your changes, push the branch to the remote repository.
“`bash
git push origin update-file-value
“`
- Create the Pull Request: Navigate to the repository on GitHub (or your preferred Git hosting service). You will often see an option to create a pull request for the newly pushed branch. Click on it, provide a title and description for your PR, and submit it.
Best Practices for Pull Requests
When creating a pull request, consider the following best practices to ensure clarity and facilitate the review process:
- Descriptive Titles: Use clear and concise titles that summarize the changes made.
- Detailed Descriptions: Provide a thorough description of the changes, why they were made, and any implications they may have.
- Link to Issues: If the PR addresses a specific issue, link it by using `issue_number` in the description.
- Review Code: Before creating the PR, review your own code for errors and ensure that it adheres to the project’s coding standards.
Example of a Pull Request Table
Field | Description |
---|---|
Title | Update value in file.go to reflect new configuration |
Description | This PR updates the configuration value in file.go from 10 to 20 to improve performance. |
Linked Issue | 42 |
Branch Name | update-file-value |
By following these steps and best practices, you can effectively create a pull request to update a file in a Go project, ensuring that your contributions are well-structured and easy to review.
Creating a Pull Request to Update a File in Go
To create a pull request (PR) for updating a file in a Go (Golang) project, follow these steps which include creating a branch, making changes, committing those changes, and finally creating the PR on your version control platform (e.g., GitHub).
Step-by-Step Process
- Clone the Repository
Use the following command to clone the repository to your local machine:
“`bash
git clone https://github.com/username/repository.git
cd repository
“`
- Create a New Branch
It’s a best practice to create a new branch for your changes. Use:
“`bash
git checkout -b update-file-value
“`
- Edit the File
Open the file you wish to update in your preferred text editor. Make your modifications as necessary. For example, if you are updating a configuration file, you might change a key-value pair in a `.go` file.
- Stage Your Changes
After making your edits, stage the changes using:
“`bash
git add path/to/your/file.go
“`
- Commit Your Changes
Commit your changes with a clear and concise message:
“`bash
git commit -m “Updated value in file.go for feature X”
“`
- Push Your Branch
Push your branch to the remote repository:
“`bash
git push origin update-file-value
“`
Creating the Pull Request
After pushing your branch, navigate to your repository on GitHub (or the version control system you are using) and follow these steps:
- Click on the “Pull Requests” tab.
- Click the “New Pull Request” button.
- Select your branch (e.g., `update-file-value`) as the compare branch.
- Ensure the base branch (typically `main` or `master`) is correctly set.
- Review your changes in the diff view.
- Add a title and description for your PR explaining the changes made.
- Click “Create Pull Request.”
Best Practices for Pull Requests
- Descriptive Titles: Use a clear and informative title for your PR.
- Detailed Descriptions: Provide context in the description, including why the change was made and any relevant issues it addresses.
- Labeling: Use labels to categorize your PR effectively if your platform supports them.
- Review Comments: Be open to feedback and suggestions from reviewers, and be prepared to make additional commits if necessary.
Example Pull Request Message
Here’s an example of a well-structured pull request message:
“`
Title: Update configuration value for API timeout
Description:
This PR updates the API timeout value in config.go from 30 seconds to 60 seconds.
This change is necessary to ensure that our application can handle slower responses
from the external service without timing out.
Changes:
- Updated the timeout value in config.go
- Adjusted related comments for clarity
Related Issues:
- Closes 123 (if applicable)
“`
By following these steps and guidelines, you can efficiently create a pull request to update a file in a Go project, ensuring clarity and collaboration within your development team.
Expert Insights on Creating Pull Requests to Update File Values in Golang
Dr. Emily Carter (Senior Software Engineer, GoLang Innovations). “When creating a pull request to update the value of a file in Golang, it is crucial to ensure that your changes are well-documented and follow the project’s coding standards. Additionally, including clear commit messages will help reviewers understand the context of your modifications.”
James Liu (Lead Developer, Cloud Solutions Inc.). “In Golang, leveraging the `git` command line effectively is essential for creating pull requests. Make sure to branch off the main codebase and test your changes locally before submitting your PR, as this minimizes integration issues and enhances code quality.”
Sarah Thompson (Open Source Contributor, Go Community). “Engaging with the community during the PR process can significantly improve the outcome. Seek feedback on your changes early and often, as this collaborative approach fosters better code practices and encourages knowledge sharing among developers.”
Frequently Asked Questions (FAQs)
What is a pull request (PR) in the context of updating a file in Golang?
A pull request (PR) is a method of submitting contributions to a project. It allows developers to propose changes to a codebase, such as updating a file, and facilitates review and discussion before merging those changes into the main branch.
How do I create a PR to update a file in a Golang project on GitHub?
To create a PR, first, fork the repository and clone it locally. Make the necessary changes to the file in your local copy, commit the changes, and push them to your forked repository. Then, navigate to the original repository on GitHub and click on the “Pull requests” tab to create a new PR, selecting your branch with the updates.
What are the best practices for writing a PR description when updating a file in Golang?
A good PR description should clearly explain the purpose of the changes, provide context about the file being updated, and outline any relevant issues or features addressed. It should also include information about testing performed and any potential impacts on the codebase.
How can I ensure my changes are compatible with the main branch before creating a PR?
To ensure compatibility, regularly sync your branch with the main branch by pulling the latest changes. Run tests locally to validate that your updates do not introduce any issues. Additionally, consider using continuous integration tools to automate testing.
What should I do if my PR gets feedback or requested changes?
If you receive feedback or requested changes, review the comments carefully, make the necessary adjustments in your code, and commit those changes. You can then push the updates to the same branch, and the PR will automatically reflect the new commits for further review.
How can I handle merge conflicts when creating a PR for a Golang file update?
To handle merge conflicts, first, pull the latest changes from the main branch into your feature branch. Resolve any conflicts in the affected files, test your changes to ensure everything works correctly, and then commit the resolved files before pushing your branch to the repository.
In summary, creating a pull request (PR) to update the value of a file in a Go (Golang) project involves several key steps that ensure the changes are properly reviewed and integrated into the main codebase. First, it is essential to fork the repository and create a new branch dedicated to the specific update. This practice not only isolates the changes but also facilitates easier collaboration and review by other developers.
Once the new branch is established, the necessary modifications to the file can be made. This may include changing configuration values, updating constants, or modifying any relevant data structures. After implementing the changes, it is crucial to commit the updates with a clear and descriptive commit message that outlines the purpose of the change. This transparency aids reviewers in understanding the context of the modifications.
Finally, submitting the pull request through the version control platform, such as GitHub or GitLab, allows for a formal review process. During this phase, other contributors can comment, suggest improvements, or approve the changes. This collaborative approach not only enhances code quality but also fosters a culture of shared responsibility and continuous improvement within the development team.
the process of creating a pull request to update a file value in Golang is
Author Profile
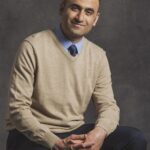
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?