How Can I Use Regular Expressions in Ruby to Parse CSV Files?
In the world of data manipulation and analysis, CSV (Comma-Separated Values) files have emerged as a staple format for storing and exchanging information. Their simplicity and versatility make them a go-to choice for developers and data scientists alike. However, as the complexity of data increases, so does the need for precise extraction and validation techniques. Enter regular expressions, a powerful tool for pattern matching and string manipulation. In the Ruby programming language, combining CSV file handling with regular expressions can unlock a new level of efficiency and accuracy in data processing. This article delves into the synergy between CSV files and Ruby’s regex capabilities, equipping you with the knowledge to streamline your data workflows.
Regular expressions are a formidable ally when it comes to parsing and validating the contents of CSV files. Whether you’re looking to extract specific fields, validate data formats, or clean up messy entries, regex provides the flexibility and precision needed to tackle these tasks. Ruby, known for its elegant syntax and robust libraries, offers built-in support for both CSV file operations and regex, making it an ideal choice for developers seeking to enhance their data manipulation skills. By leveraging these tools, you can transform raw data into structured, usable information with minimal effort.
As we explore the intersection of CSV files and regular expressions in
Understanding Regular Expressions in Ruby for CSV Files
Regular expressions (regex) are powerful tools for string matching and manipulation, essential for processing CSV files in Ruby. A CSV (Comma-Separated Values) file typically consists of data organized in rows and columns, making it vital to accurately parse and validate the content. Here, we will explore how to effectively utilize regular expressions in Ruby for handling CSV data.
Common Use Cases for Regex in CSV Processing
When dealing with CSV files, regular expressions can be employed for various tasks:
- Validation: Ensuring that the data conforms to expected formats (e.g., email addresses, phone numbers).
- Extraction: Pulling specific data from a string (e.g., extracting dates or numerical values).
- Replacement: Modifying data entries according to certain patterns (e.g., formatting dates).
Basic Regex Patterns for CSV Handling
Here are some basic regular expression patterns that can be useful when working with CSV files:
Use Case | Regex Pattern | Description | |
---|---|---|---|
Match Email | `\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z | a-z]{2,}\b` | Validates standard email formats. |
Match Phone | `\(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4}` | Matches various phone number formats. | |
Match Dates | `\b\d{1,2}[/-]\d{1,2}[/-]\d{2,4}\b` | Matches dates in MM/DD/YYYY or DD-MM-YYYY formats. | |
Match CSV Line | `^([^,]+(,[^,]+)*)$` | Matches a complete line in a CSV file. |
Implementing Regex in Ruby for CSV Files
In Ruby, you can use the `CSV` library along with regex to effectively parse and validate CSV data. Here is a basic example of how to read a CSV file and validate email addresses in each row:
“`ruby
require ‘csv’
CSV.foreach(“data.csv”, headers: true) do |row|
email = row[‘Email’]
if email =~ /\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b/
puts “{email} is valid.”
else
puts “{email} is invalid.”
end
end
“`
In this example, the `CSV.foreach` method iterates over each row of the CSV file, checking if the email address matches the specified regex pattern.
Complex Regex Patterns for Advanced CSV Validation
For more complex CSV validation scenarios, you may need to combine multiple regex patterns. For instance, validating a CSV line that contains an email and a phone number can be done as follows:
“`ruby
csv_line = “[email protected], (123) 456-7890”
if csv_line =~ /^([^,]+),\s*(\(?\d{3}\)?[-.\s]?\d{3}[-.\s]?\d{4})$/
puts “Valid CSV line with email: {$1} and phone: {$2}”
else
puts “Invalid CSV line.”
end
“`
This regex checks if the line contains both an email and a phone number, extracting them for further processing.
Best Practices for Using Regex with CSV Files
To ensure effective use of regular expressions in your CSV processing, consider the following best practices:
- Test Your Regex: Use tools like regex101.com to test your regex patterns before implementation.
- Keep Patterns Simple: Complex patterns can lead to unexpected behavior. Start with simple expressions and build complexity as needed.
- Handle Edge Cases: Always account for potential variations in data format and ensure your regex can handle them.
By leveraging regular expressions effectively, you can enhance your data processing capabilities in Ruby, ensuring that your CSV handling is both robust and efficient.
Understanding CSV File Structure
CSV (Comma-Separated Values) files are plain text files that use specific structuring to organize data. Each line in a CSV file corresponds to a record, and fields within a record are typically separated by commas.
Key characteristics of CSV files include:
- Delimiter: Commonly a comma, but other characters (like semicolons) may be used.
- Header Row: The first row often contains column names.
- Text Qualifiers: Text fields may be enclosed in quotes, particularly if they contain commas.
Regular Expressions in Ruby
Regular expressions (regex) in Ruby are powerful tools for pattern matching and data extraction. Ruby provides built-in support for regex through the `Regexp` class.
To create a regex pattern in Ruby:
- Use slashes to define the pattern: `/pattern/`
- Utilize various regex constructs, such as:
- `.`: Matches any character.
- `*`: Matches zero or more occurrences of the preceding element.
- `+`: Matches one or more occurrences of the preceding element.
- `?`: Matches zero or one occurrence of the preceding element.
- `[]`: Matches any one of the characters inside the brackets.
Extracting Data from CSV with Regex
When working with CSV files in Ruby, regex can be used to validate the format of data or extract specific information. Here are common scenarios:
- Validating Email Addresses:
To ensure a field contains a valid email format:
“`ruby
email_regex = /\A[\w+\-.]+@[a-z\d\-.]+\.[a-z]+\z/i
“`
- Extracting Numbers:
To find and extract numeric values from a string:
“`ruby
number_regex = /[\d]+/
“`
- Finding Specific Patterns:
For instance, to capture dates in the format YYYY-MM-DD:
“`ruby
date_regex = /\d{4}-\d{2}-\d{2}/
“`
Implementing Regex with CSV Parsing
Ruby’s standard library includes the `CSV` module, which simplifies reading and writing CSV files. Below is a sample implementation using regex:
“`ruby
require ‘csv’
CSV.foreach(‘data.csv’, headers: true) do |row|
if row[’email’] =~ email_regex
puts “Valid email: {row[’email’]}”
end
numbers = row[‘data’].scan(number_regex)
puts “Extracted numbers: {numbers.join(‘, ‘)}”
end
“`
In this example:
- The `CSV.foreach` method reads each row in the CSV file.
- The email field is validated against the regex.
- Numeric values are extracted and printed.
Best Practices for Using Regex with CSV
When utilizing regex in conjunction with CSV files, adhere to the following best practices:
- Keep Patterns Simple: Complex regex can become difficult to maintain and debug.
- Test Regular Expressions: Use tools or libraries that allow you to test regex patterns before implementation.
- Handle Edge Cases: Consider variations in data formats, such as leading/trailing spaces or unexpected delimiters.
- Use Named Groups: For better readability, employ named capture groups in your regex when applicable.
Practice | Description |
---|---|
Simplicity | Keep regex patterns straightforward. |
Testing | Validate patterns with test cases. |
Edge Case Handling | Prepare for data format variations. |
Named Groups | Enhance readability of regex. |
Expert Insights on Using Regular Expressions in Ruby for CSV Files
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). Regular expressions in Ruby provide a powerful tool for parsing CSV files. They allow for flexible pattern matching, which is essential when dealing with inconsistent data formats commonly found in CSVs. By leveraging Ruby’s built-in regex capabilities, developers can efficiently validate and extract specific fields from large datasets.
Mark Thompson (Software Engineer, Open Source Contributor). When working with CSV files in Ruby, utilizing regular expressions can greatly enhance data cleaning processes. For instance, regex can be used to identify and remove unwanted characters or to enforce specific formats, such as dates or email addresses, ensuring that the data conforms to expected standards before further processing.
Lisa Chen (Ruby on Rails Developer, CodeCraft Solutions). Regular expressions are invaluable when it comes to CSV file manipulation in Ruby. They enable developers to perform complex searches and replacements, which can streamline the process of transforming raw data into a structured format. However, it’s crucial to balance regex complexity with readability to maintain code maintainability.
Frequently Asked Questions (FAQs)
What is a regular expression in Ruby?
A regular expression in Ruby is a sequence of characters that forms a search pattern. It is used for string matching and manipulation, enabling developers to identify, replace, or validate text based on specific criteria.
How can I use regular expressions to parse a CSV file in Ruby?
To parse a CSV file in Ruby using regular expressions, you can read the file line by line and apply a regex pattern to match the desired fields. The `CSV` library in Ruby can also be used in conjunction with regex to handle complex data extraction.
What are some common regular expressions for CSV validation?
Common regex patterns for CSV validation include matching quoted strings, ensuring commas separate fields, and validating numeric formats. For example, a pattern like `^”([^”]+)”,(\d+)$` can match a quoted string followed by a comma and a number.
Can I use regex to handle embedded commas in CSV fields?
Yes, you can use regex to handle embedded commas in CSV fields by accounting for quotes. A regex pattern can be constructed to match fields enclosed in quotes, allowing for commas within those fields without breaking the structure.
What Ruby libraries support regular expressions for CSV processing?
The primary library for CSV processing in Ruby is the `CSV` standard library, which provides methods for parsing and generating CSV data. Additionally, you can use the `Regexp` class to implement regular expressions for more complex data manipulation.
How do I test a regular expression in Ruby?
You can test a regular expression in Ruby using the `=~` operator or the `match` method. For example, `if string =~ /pattern/` checks if the string matches the regex pattern and returns the index of the match, or `string.match(/pattern/)` returns a MatchData object if a match is found.
In the context of working with CSV files in Ruby, regular expressions serve as a powerful tool for parsing and manipulating data. CSV, or Comma-Separated Values, is a common format for data exchange, and Ruby provides built-in support for reading and writing CSV files through its standard library. Regular expressions can enhance this functionality by allowing developers to validate, search, and transform data within these files efficiently.
One of the main advantages of using regular expressions with CSV files is the ability to handle complex data patterns. For instance, when dealing with inconsistent data formats or needing to extract specific fields from a CSV row, regular expressions can be employed to match patterns and isolate relevant information. This capability is particularly useful when the CSV data does not adhere strictly to expected formats, enabling developers to implement robust data validation and cleaning processes.
Moreover, Ruby’s integration of regular expressions allows for concise and readable code. By utilizing regex within Ruby’s CSV handling methods, developers can streamline their data processing workflows. Key takeaways include the importance of understanding both CSV structure and regex syntax to maximize the effectiveness of data manipulation tasks. Ultimately, leveraging regular expressions in Ruby for CSV file processing can lead to more efficient, maintainable, and error-resistant code.
Author Profile
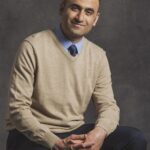
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?