Why Am I Getting the ‘Dataframe’ Object Has No Attribute ‘Iteritems’ Error?
In the world of data manipulation and analysis, Python’s Pandas library has emerged as a powerful tool that enables users to handle complex datasets with ease. However, even seasoned data scientists and analysts can encounter perplexing challenges when working with DataFrames. One such common issue is the error message: `’dataframe’ object has no attribute ‘iteritems’`. This seemingly cryptic notification can halt your workflow and leave you scratching your head, wondering what went wrong. In this article, we will unravel the mystery behind this error, explore its causes, and provide practical solutions to help you navigate these treacherous waters of data handling.
When working with DataFrames, the ability to iterate through columns is essential for data analysis tasks. The `iteritems()` method is typically used to loop over the columns of a DataFrame, allowing users to access both the column names and their corresponding data. However, if you encounter the error indicating that the DataFrame object lacks this attribute, it can be frustrating. Understanding the context of this error is crucial for troubleshooting and ensuring smooth data operations.
This article will delve into the reasons behind the `’dataframe’ object has no attribute ‘iteritems’` error, examining common pitfalls and misconceptions that lead to it. We will also provide
Understanding the Error
The error message `’dataframe’ object has no attribute ‘iteritems’` typically arises when attempting to use the `iteritems()` method on a DataFrame in pandas. This method is generally used to iterate over the (column name, Series) pairs in a DataFrame. However, the error suggests that either the object in question is not a pandas DataFrame or that there has been a typo or misuse of the method.
To clarify, the `iteritems()` method is available for pandas Series, not DataFrames. For iterating through the columns of a DataFrame, the appropriate method is `items()`.
Common Scenarios Leading to the Error
Several scenarios may lead to encountering this error:
- Incorrect Object Type: You may be trying to call `iteritems()` on an object that is not a DataFrame.
- Version Compatibility: Some features or methods might differ based on the version of pandas being used.
- Misleading Variable Names: Sometimes, variable names can be misleading, resulting in confusion about the object’s type.
Correct Methods for Iterating
To avoid this error, it is essential to use the correct methods for iteration. Here are the appropriate methods for different scenarios:
- For a DataFrame: Use the `items()` method.
- For a Series: Use the `iteritems()` method.
Here’s a comparison of the methods for clarity:
Object Type | Method | Usage Example |
---|---|---|
DataFrame | items() |
import pandas as pd df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]}) for column, data in df.items(): print(column, data) |
Series | iteritems() |
s = pd.Series([1, 2, 3]) for index, value in s.iteritems(): print(index, value) |
Troubleshooting the Error
If you encounter the error, consider the following troubleshooting steps:
- Check Object Type: Use the `type()` function to verify the type of the object you are working with.
“`python
print(type(your_object))
“`
- Review Documentation: Ensure that you are referencing the correct version of the pandas documentation for the methods you are using.
- Upgrade pandas: If you suspect a version issue, consider upgrading pandas using pip:
“`bash
pip install –upgrade pandas
“`
By following these guidelines, you can effectively resolve the issue and utilize the appropriate methods for iterating through your DataFrames and Series.
Understanding the Error Message
The error message `’dataframe’ object has no attribute ‘iteritems’` typically occurs in Python when using the Pandas library. It indicates that you are attempting to call the `iteritems()` method on a DataFrame, which is not a valid operation. Instead, `iteritems()` is a method used for Series objects.
Common Causes of the Error
- Using DataFrame Instead of Series: The `iteritems()` method is not available for DataFrame objects; it is specifically for Series.
- Typographical Errors: Misnaming the DataFrame variable or calling methods incorrectly can lead to this error.
- Version Compatibility: Different versions of Pandas may have variations in available methods, leading to unexpected behavior.
Correct Usage of iteritems()
To avoid this error, ensure you are using the `iteritems()` method on a Series. Here’s how to correctly use it:
“`python
import pandas as pd
Create a Series
s = pd.Series([1, 2, 3], index=[‘a’, ‘b’, ‘c’])
Correct usage of iteritems()
for index, value in s.iteritems():
print(f’Index: {index}, Value: {value}’)
“`
Alternative Methods for DataFrame
If your intention was to iterate over a DataFrame, consider using the following methods instead:
- iterrows(): Iterates over rows as (index, Series) pairs.
- itertuples(): Iterates over rows as named tuples.
- items(): Iterates over columns as (column name, Series) pairs.
Example of Using iterrows()
“`python
Create a DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6]
})
Correct usage of iterrows()
for index, row in df.iterrows():
print(f’Index: {index}, A: {row[“A”]}, B: {row[“B”]}’)
“`
Example of Using itertuples()
“`python
Correct usage of itertuples()
for row in df.itertuples(index=True):
print(f’Index: {row.Index}, A: {row.A}, B: {row.B}’)
“`
Best Practices for DataFrame Iteration
When working with DataFrames, it is generally advisable to avoid iteration when possible. Instead, utilize vectorized operations for performance improvement. Here are some best practices:
- Use Vectorized Operations: Leverage Pandas built-in functions that operate on entire columns.
- Apply Functions: Use `apply()` for row-wise or column-wise operations.
- Avoid Loops: Minimize the use of explicit loops for better performance with larger datasets.
Example of Using apply()
“`python
Apply a function to a column
df[‘C’] = df[‘A’].apply(lambda x: x * 2)
print(df)
“`
By following these guidelines, you can effectively manage DataFrame objects and avoid the common pitfalls associated with incorrect method usage.
Understanding the ‘dataframe’ Object Attribute Error
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The error message ‘dataframe’ object has no attribute ‘iteritems’ typically arises when a user attempts to call a method that is not available in the current version of the pandas library. It is crucial to ensure that the correct method is being used, as ‘iteritems’ is not a valid method for DataFrame objects; instead, ‘items’ should be utilized.”
Mark Thompson (Senior Software Engineer, Data Solutions Corp.). “When encountering the ‘dataframe’ object has no attribute ‘iteritems’ error, it is essential to check the data structure being manipulated. Users often confuse Series and DataFrame methods, leading to such issues. Understanding the distinctions between these two types is vital for effective data manipulation.”
Linda Zhang (Pandas Library Contributor, Open Source Community). “This error can also indicate that the pandas library is outdated. Users should ensure they are using the latest version of pandas, as methods and attributes can change over time. Regular updates and consulting the official documentation can prevent such errors.”
Frequently Asked Questions (FAQs)
What does the error message ‘dataframe’ object has no attribute ‘iteritems’ mean?
This error indicates that you are trying to use the `iteritems()` method on a DataFrame object in pandas, which does not support this method. Instead, you should use `items()` or `iterrows()` depending on your needs.
How can I iterate over columns in a pandas DataFrame?
To iterate over columns in a pandas DataFrame, use the `items()` method. This method returns an iterator that yields pairs of column names and Series objects.
What is the difference between ‘iteritems()’ and ‘items()’ in pandas?
In pandas, `items()` is the correct method to iterate over DataFrame columns, while `iteritems()` is used for Series objects. Using `iteritems()` on a DataFrame will result in an AttributeError.
What should I use instead of ‘iteritems()’ for row iteration in a DataFrame?
To iterate over rows in a DataFrame, use the `iterrows()` method. This method returns an iterator yielding index and row data as Series.
Is there a way to convert a DataFrame to a dictionary format?
Yes, you can convert a DataFrame to a dictionary using the `to_dict()` method. This method allows you to specify the orientation of the resulting dictionary.
How can I check the available methods for a pandas DataFrame?
You can check the available methods for a pandas DataFrame by using the `dir()` function in Python. For example, `dir(pd.DataFrame)` will list all attributes and methods associated with the DataFrame class.
The error message “‘dataframe’ object has no attribute ‘iteritems'” typically arises in Python when working with pandas DataFrames. This issue indicates that the code is attempting to use the `iteritems()` method, which is not available for DataFrame objects. Instead, `iteritems()` is a method associated with pandas Series. Understanding the distinction between Series and DataFrames is crucial for effectively utilizing the pandas library.
To resolve this error, it is essential to use the appropriate method for iterating over DataFrames. The correct alternatives include `iterrows()`, which allows iteration over DataFrame rows as (index, Series) pairs, or `items()`, which can be used to iterate over the columns of a DataFrame. By applying these methods, users can achieve the desired iteration without encountering the aforementioned error.
In summary, being aware of the attributes and methods associated with different pandas objects is vital for effective data manipulation. Familiarity with the pandas library’s structure will not only help in avoiding common pitfalls like the ‘iteritems’ error but also enhance overall coding efficiency. Developers should regularly consult the official pandas documentation to stay updated on best practices and available functionalities.
Author Profile
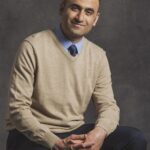
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?