Why Am I Seeing ‘DataFrame Object is Not Callable’ Error in Python?
In the world of data analysis and manipulation, Python’s Pandas library stands as a cornerstone for many developers and data scientists. Its powerful DataFrame object allows users to handle large datasets with ease, providing a versatile structure for data operations. However, as with any robust tool, users can encounter perplexing errors that can halt their progress. One such error that often leaves newcomers scratching their heads is the infamous “dataframe object is not callable.” Understanding this error is crucial for anyone looking to streamline their data workflows and avoid common pitfalls in their coding journey.
This article delves into the intricacies of this error, exploring its causes and implications within the context of Pandas. We will unpack the scenarios that lead to this frustrating message and provide insight into the underlying principles of DataFrame operations. By the end, readers will not only grasp why this error occurs but also how to effectively troubleshoot and prevent it in their own coding practices. Whether you’re a seasoned programmer or just starting your journey in data science, understanding this error will enhance your ability to work efficiently with data in Python.
Join us as we navigate the complexities of DataFrame usage, equipping you with the knowledge to tackle this common error head-on and elevate your data manipulation skills.
Understanding the Error
The error message “dataframe object is not callable” typically occurs in Python when attempting to call a pandas DataFrame object as if it were a function. This can happen due to a misunderstanding of the syntax or accidentally overwriting the DataFrame object. Understanding the underlying causes can help prevent this issue.
When you see this error, it usually indicates one of the following scenarios:
- Overwriting the DataFrame: If you assign a DataFrame to a variable name that shadows the DataFrame’s methods or attributes, you may inadvertently try to call it as a function.
- Misusing Parentheses: Using parentheses after a DataFrame variable name can signal Python to treat it as a callable object, which leads to this error.
Common Causes
To better illustrate the common causes of this error, consider the following examples:
“`python
import pandas as pd
Creating a DataFrame
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
Example of overwriting
df = 5 Now df is an integer, and calling df() will raise the error
Example of misuse
df() This will also raise the error
“`
The above examples highlight how variable reassignment and incorrect syntax can lead to confusion and errors in code execution.
How to Fix the Error
To resolve the “dataframe object is not callable” error, consider the following strategies:
- Check Variable Names: Ensure that you are not using a variable name that conflicts with the DataFrame methods. For instance, avoid naming your DataFrame as `df` when it shadows other functions or variables.
- Use Correct Syntax: When accessing DataFrame methods, ensure you are using square brackets `[]` for indexing rather than parentheses `()`.
Here are some quick tips to avoid the error:
- Always use descriptive variable names for DataFrames.
- Double-check your code for any accidental reassignment of DataFrame variables.
- Use the `.loc[]` or `.iloc[]` methods for data access instead of calling the DataFrame directly.
Example of Correct Usage
Below is an example that demonstrates the correct usage of a pandas DataFrame without invoking the callable error:
“`python
import pandas as pd
Creating a DataFrame
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
Correctly accessing data
value = df[‘A’][0] Accessing the first element in column ‘A’
print(value) Output: 1
“`
This code snippet shows how to create and access data from a DataFrame properly, avoiding the common pitfalls that lead to the “dataframe object is not callable” error.
Debugging Tips
If you continue to encounter this error, consider the following debugging tips:
- Print the Type: Use `print(type(df))` to confirm that the variable is indeed a DataFrame.
- Check the Stack Trace: Examine the error traceback to identify where the issue originates in your code.
- Use Python’s Interactive Shell: Running your code in an interactive shell can help you immediately see the output and diagnose issues in real-time.
Error Scenario | Example Code | Solution |
---|---|---|
Overwriting DataFrame | df = 5 | Use a different variable name |
Misusing Parentheses | df() | Use df[‘column_name’] instead |
By following these guidelines, you can effectively prevent and troubleshoot the “dataframe object is not callable” error in your pandas-based projects.
Understanding the Error
The error message “dataframe object is not callable” typically occurs in Python when you attempt to use parentheses to call a DataFrame object as if it were a function. This can happen due to several reasons, primarily related to syntax errors or naming conflicts. Below are common scenarios that lead to this error:
- Misnamed Variables: You may have inadvertently overwritten a method or function with a DataFrame object.
- Incorrect Syntax: Using parentheses instead of square brackets when trying to access data.
- Function Calls: Attempting to call a DataFrame like a function.
Common Causes
Identifying the root cause is crucial for resolving this error. Here are some of the most frequent causes:
Cause | Description |
---|---|
Variable Shadowing | A DataFrame variable name matches a built-in function or method name, leading to confusion. |
Incorrect Access Syntax | Using parentheses instead of brackets to access rows or columns. |
Function Assignment | Assigning a DataFrame to a variable that was previously a function. |
Examples of the Error
To illustrate the error, consider the following examples:
- Variable Shadowing Example:
“`python
import pandas as pd
Assume df is a DataFrame
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
Shadowing the DataFrame with the same variable name
def df():
return “This is a function”
This will raise the error
df() Attempting to call the DataFrame as a function
“`
- Incorrect Access Syntax Example:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
Incorrectly using parentheses
value = df(0, ‘A’) Raises “dataframe object is not callable”
“`
How to Fix the Error
To resolve this error, follow these guidelines:
- Check for Variable Shadowing:
- Rename variables to avoid conflict with built-in functions or methods.
- Use Correct Syntax:
- Always use square brackets for accessing DataFrame elements.
Example:
“`python
value = df.loc[0, ‘A’] Correct way to access DataFrame elements
“`
- Debugging Tips:
- Use `print()` statements to check variable types.
- Use the `type()` function to verify if a variable is a DataFrame.
Best Practices
Implementing best practices can prevent encountering this error in the future:
- Naming Conventions: Use distinct and descriptive names for variables to avoid shadowing.
- Consistent Syntax: Familiarize yourself with DataFrame syntax for accessing data.
- Code Reviews: Conduct code reviews to catch potential errors before execution.
By adhering to these practices, you can minimize the occurrence of the “dataframe object is not callable” error and enhance your coding proficiency with DataFrames.
Understanding the “Dataframe Object is Not Callable” Error
Dr. Emily Carter (Data Science Consultant, Analytics Insights). “The ‘dataframe object is not callable’ error typically arises when a user attempts to invoke a DataFrame as if it were a function. This often occurs due to mistakenly using parentheses instead of brackets when accessing a DataFrame’s columns. It is essential to differentiate between calling methods and accessing data.”
Michael Chen (Senior Software Engineer, DataTech Solutions). “This error can also be a result of variable name conflicts. If a DataFrame is assigned to a variable name that shadows a built-in function or method, it can lead to confusion. Always ensure that variable names are unique and descriptive to avoid such issues.”
Sarah Patel (Lead Data Analyst, Insight Analytics). “To troubleshoot the ‘dataframe object is not callable’ error, I recommend checking the code for any accidental reassignment of the DataFrame variable. Additionally, using debugging tools or print statements can help identify the exact line causing the issue, facilitating a quicker resolution.”
Frequently Asked Questions (FAQs)
What does the error “dataframe object is not callable” mean?
This error indicates that you are attempting to call a DataFrame object as if it were a function. In Python, this typically occurs when you mistakenly use parentheses after a DataFrame variable name.
How can I resolve the “dataframe object is not callable” error?
To resolve this error, check your code for instances where you use parentheses after a DataFrame object. Ensure that you are accessing DataFrame methods or attributes correctly without using parentheses unless calling a function.
What are common scenarios that lead to this error?
Common scenarios include mistakenly using parentheses when trying to access a DataFrame column or when you have overridden the DataFrame variable name with a function or method.
Can this error occur due to incorrect imports?
Yes, if you import a DataFrame incorrectly or overwrite the DataFrame class with a variable name, it can lead to this error. Always ensure that your variable names do not conflict with library functions or classes.
Is “dataframe object is not callable” specific to any programming language?
This error is primarily associated with Python, particularly when using the Pandas library. However, similar errors can occur in other programming languages when attempting to call non-callable objects.
How can I debug this error effectively?
To debug this error, review the traceback provided by Python to identify the line of code causing the issue. Inspect variable names and ensure they are not conflicting with function or class names.
The error message “dataframe object is not callable” typically arises in Python when a user attempts to call a DataFrame object as if it were a function. This often occurs due to naming conflicts, where a variable name shadows the DataFrame class or when parentheses are mistakenly used after a DataFrame variable. Understanding the context in which this error appears is crucial for effective debugging and resolution.
One common scenario leading to this error is when a DataFrame variable is assigned a name that conflicts with a method or function, such as `pd.DataFrame`. This can mislead the interpreter, causing it to treat the DataFrame as a callable function. Additionally, using parentheses after a DataFrame variable without intending to invoke a method can also trigger this error. Therefore, it is essential to review variable names and ensure they do not clash with built-in functions or methods.
To resolve the “dataframe object is not callable” error, users should first check their variable assignments and ensure that no DataFrame is being misnamed. If the variable name is appropriate, examining the code for any unintended parentheses is advisable. By following these steps, users can effectively troubleshoot and eliminate the error, allowing for smoother data manipulation and analysis within the Pandas library.
Author Profile
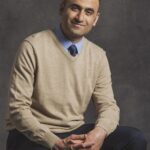
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?