How Can You Clear Filters Programmatically in MUI DataGrid?
In the world of web development, creating dynamic and user-friendly interfaces is paramount, and the Material-UI (MUI) library has emerged as a go-to solution for many developers. Among its various components, the DataGrid stands out for its powerful features that facilitate the display and manipulation of large datasets. However, as users interact with data, the need to reset or clear filters becomes essential for maintaining a seamless user experience. This article delves into the intricacies of managing filters within the MUI DataGrid, specifically focusing on how to clear them programmatically.
Understanding how to manipulate filters in the MUI DataGrid can significantly enhance the functionality of your application. Whether you’re building a complex data management tool or a simple dashboard, knowing how to clear filters in code allows for greater control over the user interface. This capability not only improves accessibility but also empowers users to navigate through data effortlessly.
As we explore the methods available for clearing filters in the MUI DataGrid, we will cover essential concepts and best practices. From leveraging built-in functionalities to implementing custom solutions, this guide will equip you with the knowledge needed to enhance your applications. Join us as we uncover the techniques that will help you create a more intuitive and responsive data experience for your users.
Implementing Clear Filters in MUI DataGrid
To clear filters programmatically in a Material-UI (MUI) DataGrid, you can utilize the `filterModel` property. This allows you to define and manage the filters applied to the grid. You can reset the filters by setting the `filterModel` back to an empty state.
Here’s how you can implement this functionality:
- Initialize the `filterModel` state in your component.
- Create a function to clear the filters by setting the `filterModel` to an empty object.
- Pass this state and function to the DataGrid.
Here’s an example code snippet:
“`javascript
import React, { useState } from ‘react’;
import { DataGrid } from ‘@mui/x-data-grid’;
const MyDataGrid = () => {
const [filterModel, setFilterModel] = useState({ items: [] });
const clearFilters = () => {
setFilterModel({ items: [] });
};
const columns = [
{ field: ‘id’, headerName: ‘ID’, width: 90 },
{ field: ‘name’, headerName: ‘Name’, width: 150 },
{ field: ‘age’, headerName: ‘Age’, width: 110 },
];
const rows = [
{ id: 1, name: ‘Alice’, age: 25 },
{ id: 2, name: ‘Bob’, age: 30 },
{ id: 3, name: ‘Charlie’, age: 35 },
];
return (
autoHeight
/>
);
};
export default MyDataGrid;
“`
In this example, the `clearFilters` function resets the `filterModel` to an empty array, effectively removing all active filters from the DataGrid.
Understanding the Filter Model
The `filterModel` property in MUI DataGrid consists of several key attributes:
- items: An array of filter items applied to the columns.
- linkOperator: Determines how the filters are combined (e.g., “AND” or “OR”).
- quickFilterLogicOperator: Used for quick filter searches.
Here’s a simple table outlining the attributes of the `filterModel`:
Attribute | Description |
---|---|
items | An array of objects representing the filters applied to the grid columns. |
linkOperator | Defines how multiple filters should be combined (AND/OR). |
quickFilterLogicOperator | Sets the logic for quick filtering operations. |
Utilizing the `filterModel` effectively allows for dynamic filtering capabilities, enhancing the user experience while interacting with data in the grid. By resetting the filters through the `clearFilters` function, you can provide users with an easy way to revert their selections without having to refresh the entire grid.
Clearing Filters Programmatically in MUI DataGrid
In Material-UI (MUI), the DataGrid component provides a robust way to display and manipulate tabular data. One common requirement is to clear applied filters programmatically. This can enhance user experience by allowing users to reset the view with a single action.
Using the `apiRef` to Clear Filters
MUI DataGrid offers an `apiRef`, which is a reference to the internal API of the DataGrid. This API can be utilized to manipulate the grid’s state, including clearing filters. Here’s how to do it:
- Create a Reference:
Use the `useGridApiRef` hook to create an API reference for your DataGrid component.
“`javascript
import { DataGrid, useGridApiRef } from ‘@mui/x-data-grid’;
const apiRef = useGridApiRef();
“`
- Attach the API Reference:
Attach the API reference to the DataGrid component.
“`javascript
“`
- **Clearing Filters**:
To clear filters, call the `setFilterModel` method with an empty filter model.
“`javascript
const clearFilters = () => {
apiRef.current.setFilterModel({ items: [] });
};
“`
- Button for Clearing Filters:
A button can be added to trigger the `clearFilters` function.
“`javascript
“`
Example Implementation
Here’s a complete example demonstrating how to implement the filter clearing functionality:
“`javascript
import React from ‘react’;
import { DataGrid, useGridApiRef } from ‘@mui/x-data-grid’;
const MyDataGridComponent = () => {
const apiRef = useGridApiRef();
const rows = [/* your row data */];
const columns = [/* your column definitions */];
const clearFilters = () => {
apiRef.current.setFilterModel({ items: [] });
};
return (
);
};
export default MyDataGridComponent;
“`
Considerations
When implementing filter clearing functionality, consider the following:
- User Experience: Provide visual feedback when filters are cleared, such as a toast notification.
- Performance: Frequent updates to the DataGrid may impact performance, especially with large datasets.
- State Management: Ensure that the filter state is synchronized with other UI elements, such as search inputs or dropdowns.
Advanced Filtering Techniques
For more complex applications, combining filter clearing with other state management techniques may be necessary:
- Integration with External State: Use a state management library (like Redux) to manage filters globally.
- Dynamic Filter Models: Create a dynamic filter model that adapts based on user input or other interactions.
These strategies can help maintain a clean and efficient user interface while providing advanced data manipulation capabilities.
Expert Insights on Clearing Filters in MUI DataGrid
Dr. Emily Carter (Senior Frontend Developer, Tech Innovations Inc.). “To effectively clear filters in a Material-UI DataGrid programmatically, one can utilize the `setFilterModel` method. By setting the filter model to an empty object, you can reset all applied filters, ensuring that the grid displays all available data without any restrictions.”
Michael Chen (UI/UX Engineer, Design Systems Group). “Implementing a clear filters button in a DataGrid can enhance user experience significantly. By binding a button to a function that resets the filter model, users can quickly revert to the default view. This is particularly useful in applications where data exploration is key.”
Sarah Thompson (Software Architect, CodeCraft Solutions). “When working with MUI DataGrid, it is essential to maintain state management effectively. Using a state management library like Redux can simplify the process of clearing filters, as you can manage the filter state centrally and dispatch actions to reset filters when needed.”
Frequently Asked Questions (FAQs)
How can I programmatically clear filters in a MUI DataGrid?
You can clear filters in a MUI DataGrid by using the `setFilterModel` method. Pass an empty filter model to it, like this: `setFilterModel({ items: [] });`. This will reset all applied filters.
Is there a built-in method to reset filters in MUI DataGrid?
MUI DataGrid does not provide a specific built-in method for resetting filters. However, you can achieve this by manipulating the filter model through the `setFilterModel` function.
Can I clear filters based on specific columns in MUI DataGrid?
Yes, you can clear filters for specific columns by updating the filter model to exclude the filters for those columns. Modify the `items` array in the filter model accordingly.
What is the filter model structure in MUI DataGrid?
The filter model in MUI DataGrid consists of an array of filter items, where each item contains properties such as `columnField`, `operatorValue`, and `value`. This structure allows for complex filtering capabilities.
How do I trigger a filter reset on a button click in MUI DataGrid?
To reset filters on a button click, create a function that calls `setFilterModel` with an empty array. Attach this function to the button’s `onClick` event to clear the filters when the button is pressed.
Are there performance considerations when clearing filters in MUI DataGrid?
Clearing filters in MUI DataGrid is generally efficient, but performance may vary with large datasets. It is advisable to test the performance impact in your specific use case, especially if filters are frequently changed or reset.
The implementation of clearing filters in a DataGrid using Material-UI (MUI) is an essential feature for enhancing user experience. Users often need to reset their views to the original dataset after applying various filters. MUI provides straightforward methods to manage this functionality programmatically, allowing developers to maintain control over the data displayed in the grid.
To clear filters in a MUI DataGrid, developers can utilize the `setFilterModel` method, which allows for the manipulation of the filter model directly. By setting the filter model to an empty state, developers can effectively reset all applied filters. This approach not only simplifies the code but also ensures that the grid reflects the unfiltered data, thereby improving usability.
Moreover, it is important to consider the user interface design when implementing filter clearing options. Providing a clear button or similar UI element can enhance user interaction, allowing users to quickly return to the original dataset without navigating through multiple filters. Overall, the ability to clear filters programmatically in MUI DataGrid is a valuable feature that contributes to a more intuitive and efficient data management experience.
Author Profile
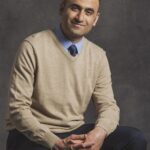
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?